C++11/C++14 Thread
3. Threading with Lambda Function 2020
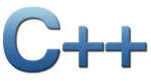
"If you're an experienced C++ programmer and are anything like me, you initially
approached C++11 thinking, "Yes, yes, I get it. It's C++, only more so." But as you
learned more, you were surprised by the scope of the changes. auto declarations,
range-based for loops, lambda expressions, and rvalue references change the face of
C++, to say nothing of the new concurrency features. And then there are the
idiomatic changes. 0 and typedefs are out, nullptr and alias declarations are in.
Enums should now be scoped. Smart pointers are now preferable to built-in ones.
Moving objects is normally better than copying them.
- Effective Modern C++ by Scott Meyers
Lambda function was briefly introduced in C++11 Thread 1. Creating Threads.
In this section, we'll see the usefulness of lambda function for multi-threading.
Let's start from where we left:
#include <iostream> #include <thread> int main() { std::thread t([](){ std::cout << "thread function\n"; }); std::cout << "main thread\n"; t.join(); return 0; }
We're going to create 5 more threads, and put those into std::vector container. For the join(), we'll use for_each():
#include <iostream> #include <thread> #include <vector> #include <algorithm> int main() { // vector container stores threads std::vector<std::thread> workers; for (int i = 0; i < 5; i++) { workers.push_back(std::thread([]() { std::cout << "thread function\n"; })); } std::cout << "main thread\n"; // Looping every thread via for_each // The 3rd argument assigns a task // It tells the compiler we're using lambda ([]) // The lambda function takes its argument as a reference to a thread, t // Then, joins one by one, and this works like barrier std::for_each(workers.begin(), workers.end(), [](std::thread &t) { t.join(); }); return 0; }
Output should look like this:
thread function thread function thread function thread function thread function main thread
The vector container workers stores 5 threads created, and after they finished the task they are joined with the main thread:
We loop through every thread via for_each(), and its 3rd argument assigns a task. The '[]' tells the compiler we're using lambda. The lambda function takes its argument as a reference to a thread, t. Then, joins one by one, and this works like barrier.
As we can see from the output, we cannot tell which thread is printing which. So, we need to put a tag on each thread. Since we're using lambda, we can utilize its capture capability. By just putting the i as in the following line, we can pass the index into the thread function:
for (int i = 0; i < 5; i++) { workers.push_back(std::thread([i]() { std::cout << "thread function " << i << "\n"; })); }
Output:
thread function thread function thread function thread function thread function main thread 4 2 1 0 3
The output could be different for each run, which demonstrates the non-deterministic nature of concurrent programming. Also, we can see from the output, even in-between the print statement, it could be preemptive, in other words, the scheduler can interrupt at any time. So, our additional effort of using the capture feature of lambda was not successful due to the nature of current programming.
C++11/C++14 Thread Tutorials
C++11 1. Creating Threads
C++11 2. Debugging with Visual Studio 2013
C++11 3. Threading with Lambda Function
C++11 4. Rvalue and Lvalue
C++11 5. Move semantics and Rvalue Reference
C++11 5B. Move semantics - more samples for move constructor
C++11 6. Thread with Move Semantics and Rvalue Reference
C++11 7. Threads - Sharing Memory and Mutex
C++11 8. Threads - Race Conditions
C++11 9. Threads - Deadlock
C++11 10. Threads - Condition Variables
C++11 11. Threads - unique futures (std::future<>) and shared futures (std::shared_future<>).
C++11 12. Threads - std::promise
C++11/C++14 New Features
initializer_list
Uniform initialization
Type Inference (auto) and Range-based for loop
The nullptr and strongly typed enumerations
Static assertions and Constructor delegation
override and final
default and delete specifier
constexpr and string literals
Lambda functions and expressions
std::array container
Rvalue and Lvalue (from C++11 Thread tutorial)
Move semantics and Rvalue Reference (from C++11 Thread tutorial)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization