C++11/C++14 7. Threads with Shared Memory and Mutex - 2020
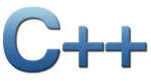
"If you're an experienced C++ programmer and are anything like me, you initially
approached C++11 thinking, "Yes, yes, I get it. It's C++, only more so." But as you
learned more, you were surprised by the scope of the changes. auto declarations,
range-based for loops, lambda expressions, and rvalue references change the face of
C++, to say nothing of the new concurrency features. And then there are the
idiomatic changes. 0 and typedefs are out, nullptr and alias declarations are in.
Enums should now be scoped. Smart pointers are now preferable to built-in ones.
Moving objects is normally better than copying them.
- Effective Modern C++ by Scott Meyers
Let's look at the example we used a while ago. This seemingly innocent short code has already an issue of race condition for a resource, cout.:
#include <iostream> #include <thread> void thread_function() { std::cout << "thread function\n"; } int main() { std::thread t(&thread_function); std::cout << "main thread\n"; t.join(); return 0; }
If we add for loop for both couts from main() and thread_function, we can clearly see that there is a resource race:
#include <iostream> #include <thread> void thread_function() { for (int i = -100; i < 0; i++) std::cout << "thread function: " << i << "\n"; } int main() { std::thread t(&thread_function); for (int i = 0; i < 100; i++) std::cout << "main thread: " << i << "\n"; t.join(); return 0; }
thread function: main thread: 0 main thread: 1 main thread: 2 main thread: 3 -100 thread function: -99 thread function: -98 thread function: -97 thread function: -96 thread function: -95 thread function: -94 thread function: -93 thread function: -92 thread function: -91 thread function: -90 thread function: -89 thread function: -88 thread function: -87 thread function: -86 thread function: -85 thread function: -84 thread function: -83 thread function: -82main thread: 4 main thread: 5 main thread: 6
As we can see from the output, the two threads get the cout resource in a ramdom fashion. To have a deterministic access, the code below is using mutex: lock before accessing cout, and then unlock after using it:
#include <iostream> #include <thread> #include <string> #include <mutex> std::mutex mu; void shared_cout(std::string msg, int id) { mu.lock(); std::cout << msg << ":" << id << std::endl; mu.unlock(); } void thread_function() { for (int i = -100; i < 0; i++) shared_cout("thread function", i); } int main() { std::thread t(&thread_function); for (int i = 100; i > 0; i--) shared_cout("main thread", i); t.join(); return 0; }
Now the output becomes more in order:
... thread function:-12 main thread:12 thread function:-11 main thread:11 thread function:-10 main thread:10 thread function:-9 main thread:9 thread function:-8 main thread:8 thread function:-7 main thread:7 thread function:-6 main thread:6 thread function:-5 main thread:5 thread function:-4 main thread:4 thread function:-3 main thread:3 thread function:-2 main thread:2 thread function:-1 main thread:1
The sections below will discuss data race and then present a way of preventing the data race condition using mutex and lock_guard().
The issued of sharing data between threads are mostly due to the consequences of modifying data.
If the data we share is read-only data, there will be no problem, because the data read by one thread is unaffected by whether or not another thread is reading the same data. However, once data is shared between threads, and one or more threads start modifying the data, and that's the start of problems. In this case, we must take care to make sure that everything works out fine.
Some of the key words used in chapter are explained in Multi-Threaded Programming - Terminology, such as race Condition, invariants, mutex, and deadlock , etc.
Here is an example of three threads attempting to access the same list, myList:
#include <iostream> #include <thread> #include <list> #include <algorithm> using namespace std; // a global variable std::list<int>myList; void addToList(int max, int interval) { for (int i = 0; i < max; i++) { if( (i % interval) == 0) myList.push_back(i); } } void printList() { for (auto itr = myList.begin(), end_itr = myList.end(); itr != end_itr; ++itr ) { cout << *itr << ","; } } int main() { int max = 100; std::thread t1(addToList, max, 1); std::thread t2(addToList, max, 10); std::thread t3(printList); t1.join(); t2.join(); t3.join(); return 0; }
One of the possible output looks like this:
0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,26,27,28,29, 30,31,32,33,34,0,10,20,30,40,50,60,70,80,90,42,43,44,45,46,47,48,49,50,51,52,53, 54,55,56,57,58,59,60,61,62,63,64,65,66,67,68,69,70,71,72,73,74,75,76,77,78,79,80 ,81,82,83,84,85,86,87,88,89,90,91,92,93,94,95,96,97,98,99,
In the process of adding elements to the list, the thread t1 that puts every integer from 0 to 99 competes with another thread t2 that puts every 10th elements. Also, there is another competing thread, t3 that accessed the same list to print out the list. As we see from the results, the list failed adding elements in order. This is due to the three threads accessed the list at the same time. So, to get the right list, we need some protection mechanism such as mutex.
Though mutexes are probably the most widely used data-protection mechanism in C++, it's important to structure our code to protect the right data and avoid race conditions inherent in our interfaces. Mutexes also come with their own problems, in the form of a deadlock and protecting either too much or too little data.
In C++, we create a mutex by constructing an instance of std::mutex, lock it with a call to the member function lock(), and unlock it with a call to the member function unlock(). However, it is not a good practice to call the member functions directly, because this means that we have to remember to call unlock() on every code path out of a function, including those due to exceptions.
In other words, if we have an exception in the code after the lock() but before the unlock(), then we'll have a problem. The resources are in locked state!
So, the Standard C++ Library provides the std::lock_guard class template, which implements that RAII idiom for a mutex. It locks the supplied mutex on construction and unlocks it on destruction, thus ensuring a locked mutex is always correctly unlocked.
The example below shows how to protect a list that can be accessed by multiple threads using a std::mutex, along with std::lock_guard. Both of these are declared in the <mutex> header.
#include <iostream> #include <thread> #include <list> #include <algorithm> #include <mutex> using namespace std; // a global variable std::list<int>myList; // a global instance of std::mutex to protect global variable std::mutex myMutex; void addToList(int max, int interval) { // the access to this function is mutually exclusive std::lock_guard<std::mutex> guard(myMutex); for (int i = 0; i < max; i++) { if( (i % interval) == 0) myList.push_back(i); } } void printList() { // the access to this function is mutually exclusive std::lock_guard<std::mutex> guard(myMutex); for (auto itr = myList.begin(), end_itr = myList.end(); itr != end_itr; ++itr ) { cout << *itr << ","; } } int main() { int max = 100; std::thread t1(addToList, max, 1); std::thread t2(addToList, max, 10); std::thread t3(printList); t1.join(); t2.join(); t3.join(); return 0; }
The new output shows that we got the right process working on the list.
0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,26,27,28,29, 30,31,32,33,34,35,36,37,38,39,40,41,42,43,44,45,46,47,48,49,50,51,52,53,54,55,56 ,57,58,59,60,61,62,63,64,65,66,67,68,69,70,71,72,73,74,75,76,77,78,79,80,81,82,8 3,84,85,86,87,88,89,90,91,92,93,94,95,96,97,98,99,0,10,20,30,40,50,60,70,80,90,
C++11/C++14 Thread Tutorials
C++11 1. Creating Threads
C++11 2. Debugging with Visual Studio 2013
C++11 3. Threading with Lambda Function
C++11 4. Rvalue and Lvalue
C++11 5. Move semantics and Rvalue Reference
C++11 5B. Move semantics - more samples for move constructor
C++11 6. Thread with Move Semantics and Rvalue Reference
C++11 7. Threads - Sharing Memory and Mutex
C++11 8. Threads - Race Conditions
C++11 9. Threads - Deadlock
C++11 10. Threads - Condition Variables
C++11 11. Threads - unique futures (std::future<>) and shared futures (std::shared_future<>).
C++11 12. Threads - std::promise
C++11/C++14 New Features
initializer_list
Uniform initialization
Type Inference (auto) and Range-based for loop
The nullptr and strongly typed enumerations
Static assertions and Constructor delegation
override and final
default and delete specifier
constexpr and string literals
Lambda functions and expressions
std::array container
Rvalue and Lvalue (from C++11 Thread tutorial)
Move semantics and Rvalue Reference (from C++11 Thread tutorial)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization