3. Hello World - urls & views
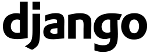
Everybody starts with Hello World. I was not an exception, either. It takes time to learn something, especially, it's not a primary everyday task at work place.
Django was my side kick and it took me several years to get used to it. Still, I consider myself as a novice.
It's been very hard to set aside a time slot for Django. But I managed to start something real such as this:
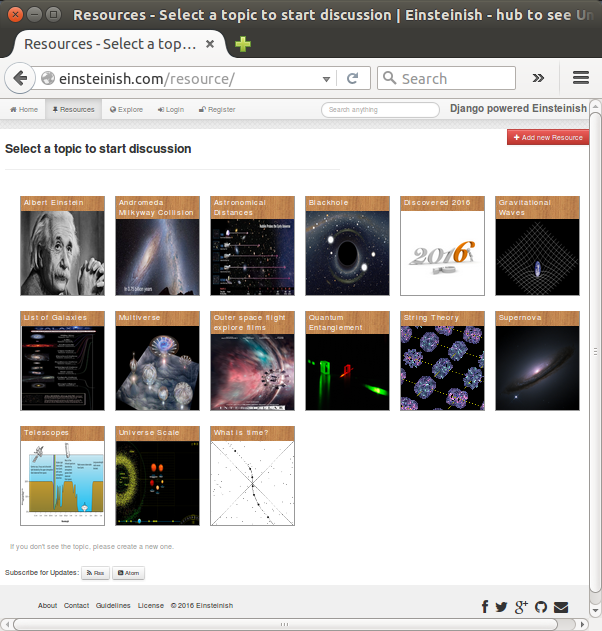
I've been exposed to couple of web frameworks, and currently managing my sites with RubyOnRails, Angular/Node, Laravel, and Django. I prefer Django over others. Probably, not just because I like Python but also Python has several promising areas including NLTK/Scikit/Spark and also graphics tool like Matplotlib which seems to be overcoming once the hot one like D3.js.
So, if you're new to Django, I encourage you to move forward and learn it.
If you're new to REST, then you can try lighter ones such as Bottle or Flask (actually, I haven't tried Flask).
In the previous chapters, we've learned how to setup project and made a polling app utilizing SQL. However, it's a little bit tougher than this "Hello World" example that I'll show in this chapter. So, let's pause the polling app here, instead do a simpler one first. We'll resume the polling project in later chapters.
In this chapter, we'll take much simpler project as a review what we've learned so far.
Let's create a HelloWorld project:
$ django-admin.py startproject HelloWorld $ cd HelloWorld $ ls HelloWorld manage.py
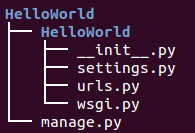
Note that we have manage.py file and HelloWorld folder inside HelloWorld project.
- __init__.py: An empty file that tells Python that this directory should be considered a Python package.
- settings.py: Settings/configuration for this Django project. Django settings will tell us all about how settings work. In other words, this file will hold all apps, database settings information.
- urls.py: The url declarations for this Django project; a "table of contents" of our Django-powered site. This is a file to hold the urls of our website such as "http://localhost/HelloWorldApp". In order to use /HelloWorldApp in our HelloWorld project we have to mention this in urls.py.
- wsgi.py: An entry-point for WSGI-compatible web servers to serve our project. This file handles our requests/responses to/from django development server.
Inside the HelloWorld project directory, we need to run the development server:
$ python manage.py runserver Validating models... 0 errors found ... Django version 1.6.5, using settings 'HelloWorld.settings' Starting development server at http://127.0.0.1:8000/ Quit the server with CONTROL-C.
Open a browser and put "http://127.0.0.1:8000/" into url box, then we get the default page:
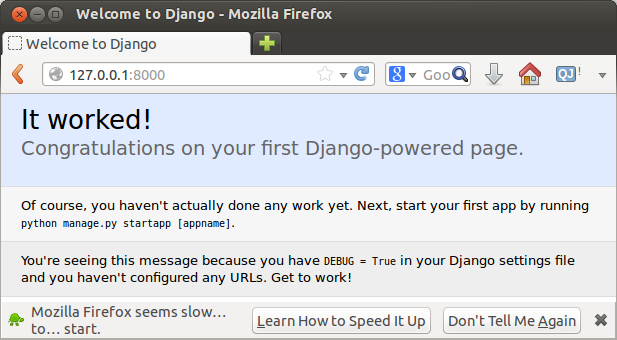
To create an app (inside HelloWorld project directory), we need to run the following command:
$ django-admin startapp HelloWorldApp $ ls HelloWorld HelloWorldApp manage.py
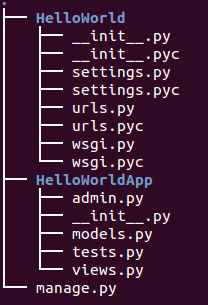
As we can see from the layout in picture above, the django-admin startapp app created files:
- __init__.py - this file indicates our app as python package.
- models.py - file to hold our database informations.
- views.py - our functions to hold requests and logics.
- tests.py - for testing.
We need to edit settings.py under HelloWorld project directory to add our application HelloWorldApp as shown below:
# Application definition INSTALLED_APPS = ( 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', 'HelloWorldApp', )
How does Django know what view to send a particular request?
Django uses a mapping file called urls.py which maps html addresses to views, using regular expressions. In other words, Django has a way to map a requested url to a view which is needed for a response via regular expressions.
Let's modify the urls.py which is under the project directory, HelloWorld:
from django.conf.urls import patterns, include, url from HelloWorldApp.views import foo #from django.contrib import admin #admin.autodiscover() urlpatterns = patterns('', # Examples: # url(r'^$', 'HelloWorld.views.home', name='home'), # url(r'^blog/', include('blog.urls')), #url(r'^admin/', include(admin.site.urls)), url(r'HelloWorldApp/$', foo), )
In the commented lines, we have ^$. Since the caret(^) means the start and the dollar($) means the end, ^$ indicates we got nothing. The r in "url(r..) is not a part of regex but it's a python indicating "raw" to prevent any character in regex from being parsed in python's way. In other words, it tells python that a string is "raw" - that nothing in the string should be escaped.
The url() is a function call that builds url patterns and it takes five arguments, most of which are optional:
url(regex, view, kwargs=None, name=None, prefix='')
The foo is the Python import string to get to a view.
Visit django.conf.urls utility functions for more information.
As a next step, we'll modify views.py under app directory, HelloWorldApp:
# Create your views here. from django.http import HttpResponse def foo(request): return HttpResponse("Hello World!")
This views.py takes a request object and returns a response object.
Note that we first import the HttpResponse object from the django.http module. Each view exists within the views.py file as a series of individual functions. In our case, we only created one view - called foo. Each view takes in at least one argument - a HttpRequest object, which also lives in the django.http module. Each view must return a HttpResponse object. A simple HttpResponse object takes a string parameter representing the content of the page we wish to send to the client which is requesting the view.
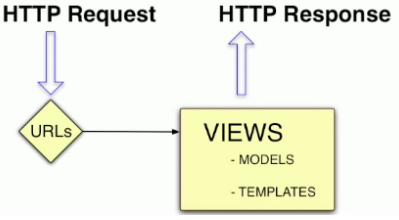
$ python manage.py runserver
If we type in http://localhost:8000/HelloWorldApp/, we get the successful "Hello World!":
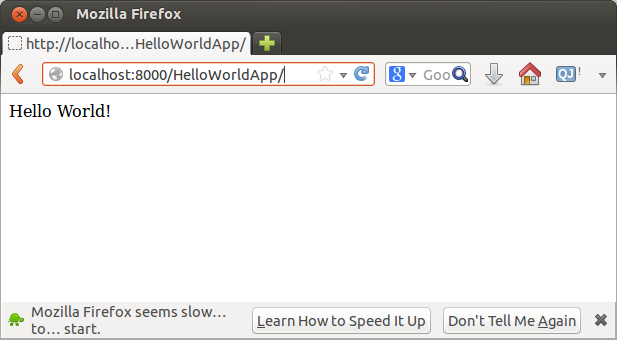
- When we type localhost:8000/HelloWorldApp into the browser, this will send request to Django. Django framework provides a way to map HelloWorldApp to a code to do something as listed in the next steps.
- Django will read urls.py and look for url matching pattern.
- If it matches, it calls the associated function.
- In our case, it matches HelloWorldApp in urls.py.
- So, foo() in views.py is called.
- In views.py, we have the code to display the "Hello World!" as HttpResponse.
- Creating a new Django Project
To create the project, we need to run,django-admin.py startproject <project_name>
- Creating a new Django application
- To create a new application, we should run,
python manage.py startapp <app_name>
- Tell our Django project about the new application by adding it to the INSTALLED_APPS tuple in our project's settings.py file.
- In our project urls.py file, add a mapping to the application and this info in urls.py file will be used to direct incoming URL strings to views.
- In our application's view.py, create the required views ensuring that they return a HttpResponse object.
- To create a new application, we should run,
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization