Matlab Tutorial : For Loop
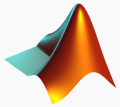
For loop's syntax looks like this:
for loop_index = vector code; end
This will iterate each element of the vector.
Let's write our first for loop:
for i = 1:3 i end
The code will print out 1, 2 and 3.
Next try another one:
v = -3:3; for i = 1:length(v) v(i) end
If we run the code, we get -3, -2, ..., 2, 3
The following code will add up each element of a vector, and display the result after ending the for loop:
v = 1:10; sum = 0; for i = 1:length(v) sum = sum + v(i); end disp(sum)
We can specify the step size of the loop:
for loop_index = start: step_size : end code; end
Suppose We want to add only odd numbers from the numbers in 1:10
sum = 0; for i = 1:2:10 sum = sum + i; end disp(sum) % 25
The vector for the loop index does not have to be in order:
a = 1:10; indx = [1 9 2 4]; sum_a = 0; for i = indx sum_a = sum_a + a(i); end disp(sum_a) % 16
The loop will access the elements of vector a starting from the 1st, 9th, 2nd and 4th.
a = 1:10; v = zeros(1,length(a)); sum_a = 0; for i = 1:length(a) sum_a = sum_a + i; v(i) = sum_a; end figure; plot(v);
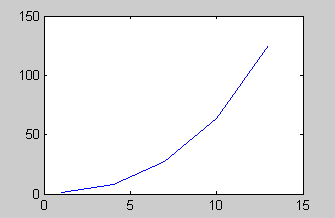
We can also check the result from the Variable window by double clicking the v on Workspace pane:
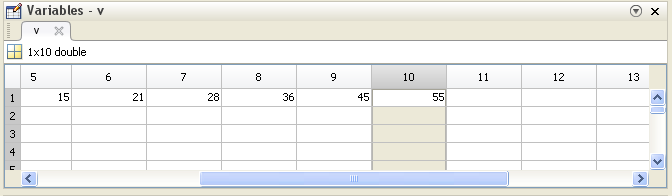
Sometime we want to have several lines be commented out or uncomment those lines. We have short-keys:
- Select the text
- Use Ctrl-R to comment
- Use Ctrl-T to uncomment
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization