Web Technologies
- Open APIs, SOAP, and REST 2020
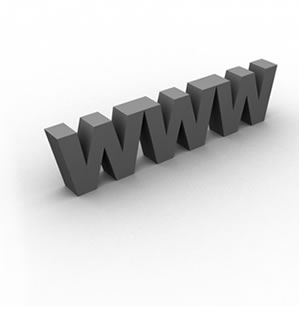
Open API (Application Programming Interface) enables websites to interact with each other by using REST, SOAP, JavaScript, XML-RPC (XML remote procedure call), and other web technologies. In other words, Open API is a system gives developers of third-party websites the opportunity of authorizing users on other site. So, it is open to anybody. But to do that we need to comply to certain rules or protocols, for example, rules described by REST. These rules are related to the authorization/authentication of requests.
The API provider opens up its data, and a developer must register his/her application. Then, the developer gets an API Key. The key allows the provider to identify the application that wants to use specific APIs. OAuth is the protocol to comply.
The API is a set of HTTP request messages either in XML or JSON (JavaScript Object Notation). The communication is done by SOAP (Simple Object Access Protocol) or REST (Representational State Transfer).
"The XML vs. JSON debate is one of the bigger topics in developer circles during the last decade. Although XML has several advantages, such as being a defined standard since 1996, JSON's lighter approach has proved popular. ProgrammableWeb's historical API data shows that XML as a data format peaked in mid-2009, but that JSON has been rising for the last eight years."
1. We need to install the following.
2. Setting up Tomcat server.
In Eclipse, Window > Preferences.
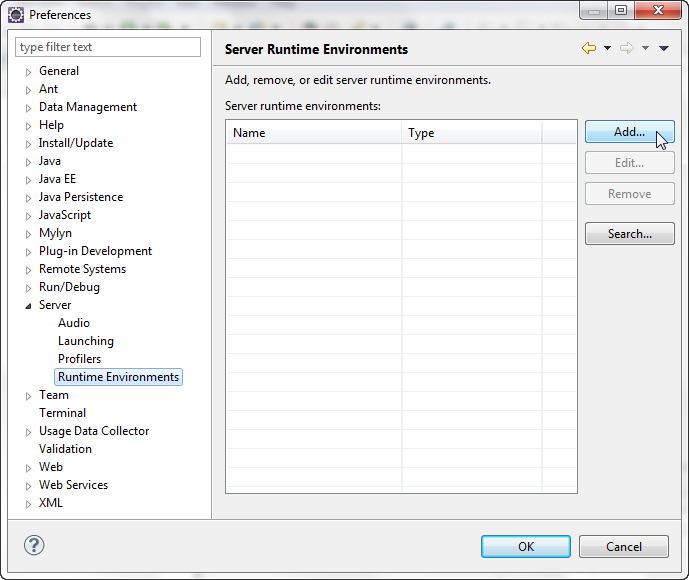
Click Add button.
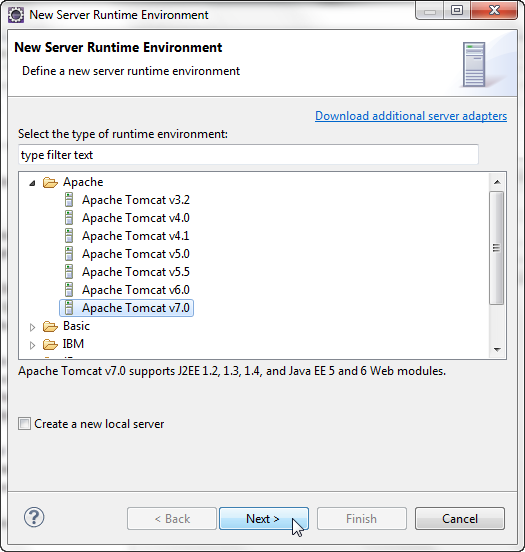
Type in root directory of Tomcat and set Java Runtime Environment to 7 (jre7).
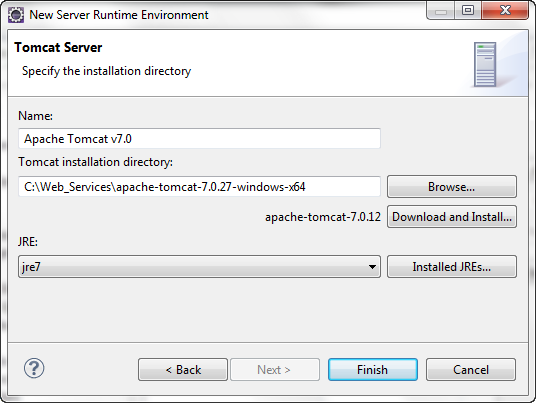
At finish, we get
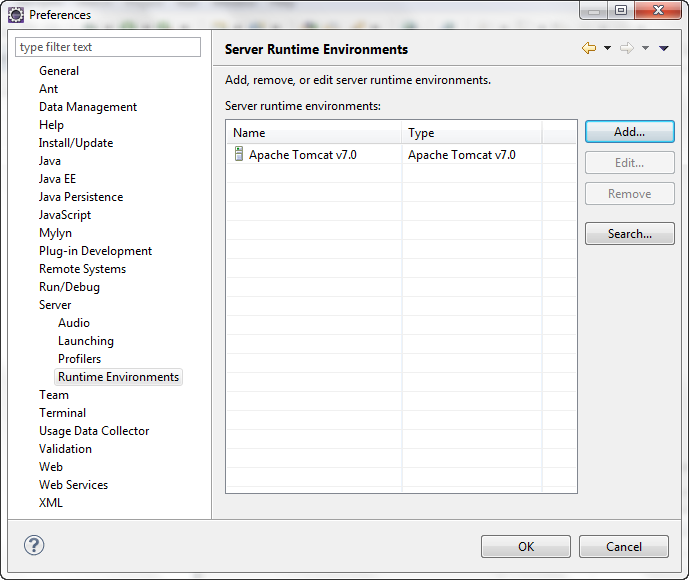
Click OK. Now, we installed Tomcat server.
3. Configuring Eclipse to use Apache CXF as the Web Service Runtime.
In Eclipse, Window > Preferences > Web Services > CXF 2.x Preferences >Add, then type in the CXF installation directory.
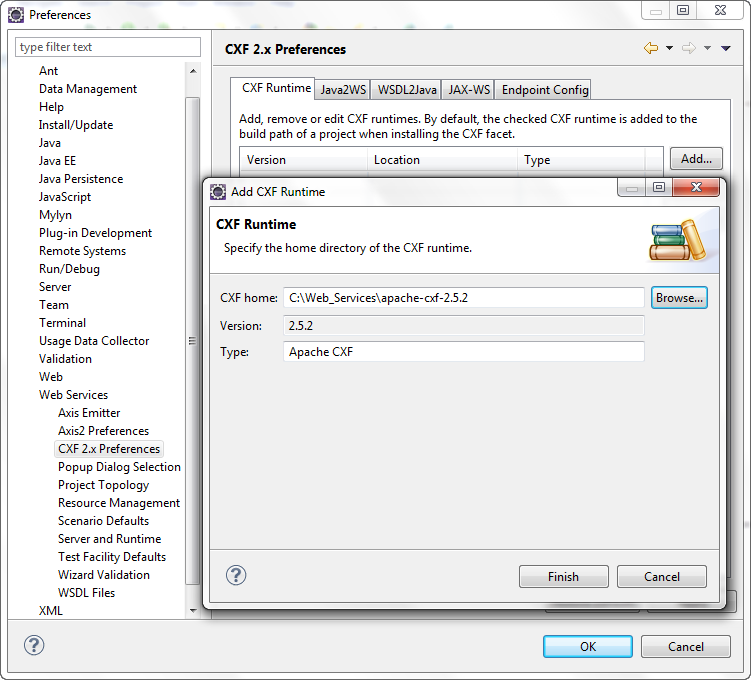
Click Finish, and then, check the box to set CXF as default.

4. Configuring Tomcat to use Apache CXF as the Web Service Runtime by default.
In Eclipse, Window > Preferences > Web Services > Server and Runtime, then select Apache CXF 2.x for Web service runtime.
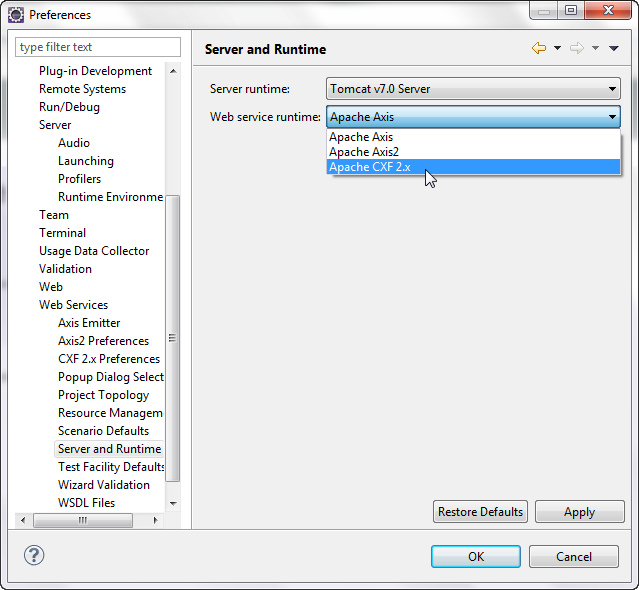
Click Finish button. Now, we completed setting up Eclipse to use Apache Tomcat as a web server and to use CXF as a runtime for Web Services. So, it is time to create a Web Service.
5. Creating a Web Service.
File > New > Dynamic Web Project, and then type in project name.
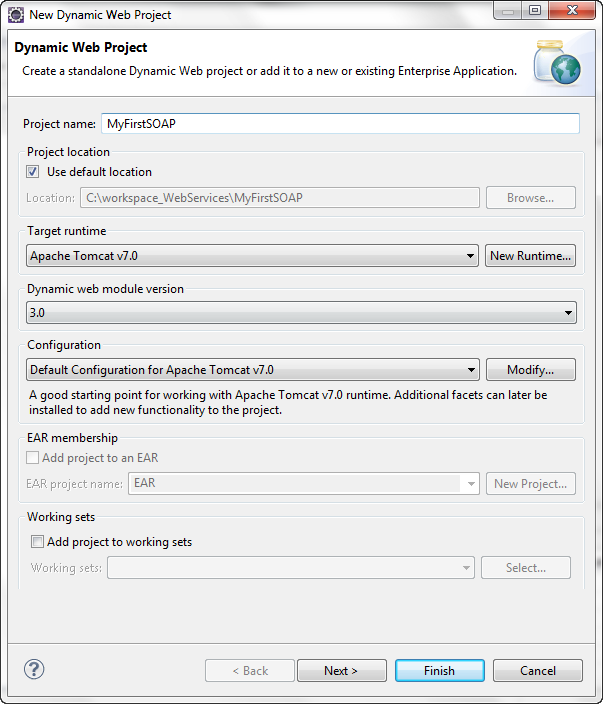
Click finish, then we have new stuffs in the Project Explorer.
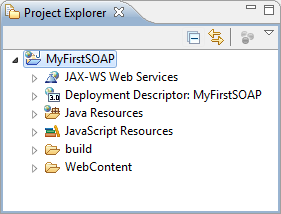
Right click on Java Resources > New > Class, then type in the class name.
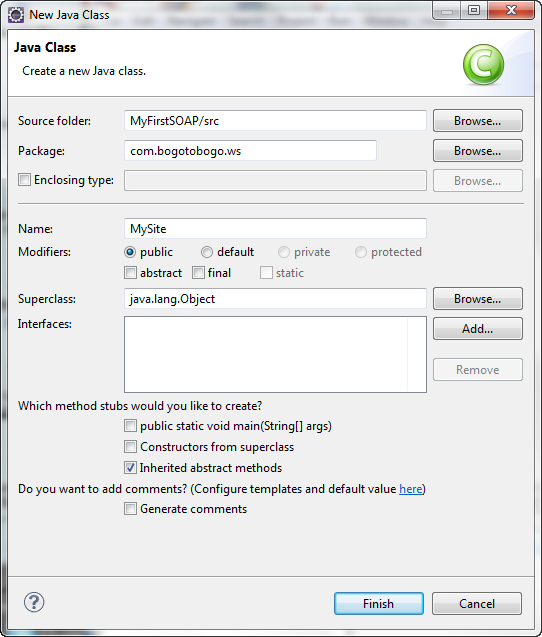
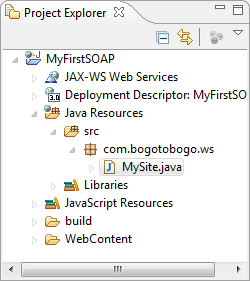
Here is the simplest code returning a string:
package com.bogotobogo.ws; public class MySite { public String WhatIsTheNameOfMySite(String site) { return "My site is " + site + ".com!"; } }
Now we want to use Web Service by wrapping around the code:
File > New > Web Service
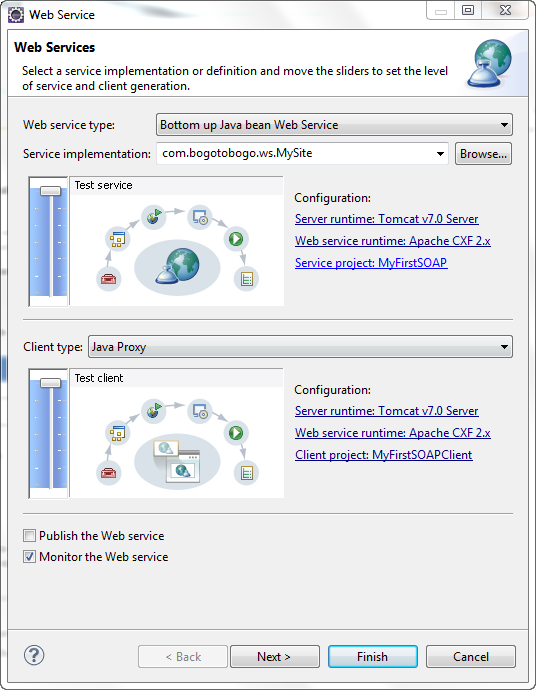
Note that we moved the slide to the top and checked "Monitor the Web Service" check box. Then, click next.
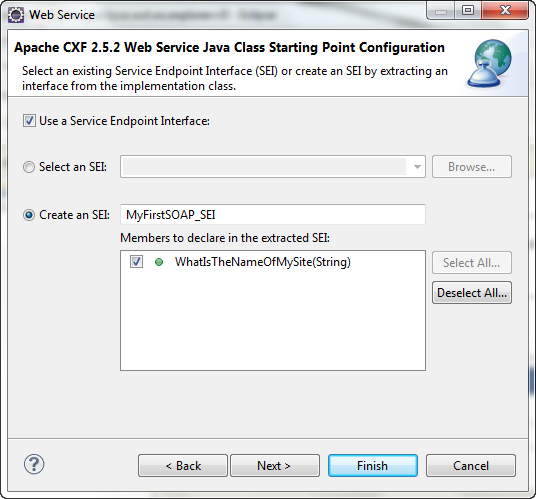
After clicking the Finish, Eclipse launches Web Services Explorer with which we can test out web services.
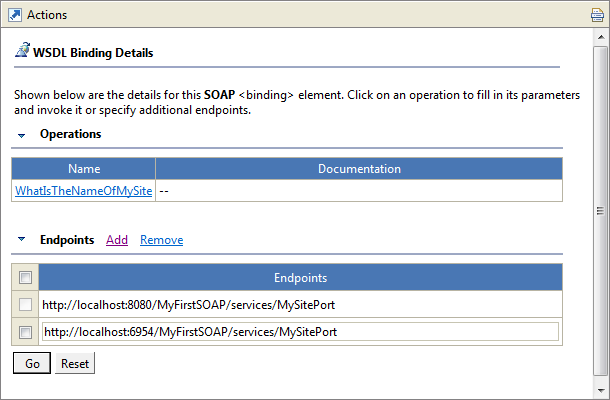
Click the method name link, and then type in arg0 value after hitting Add button.
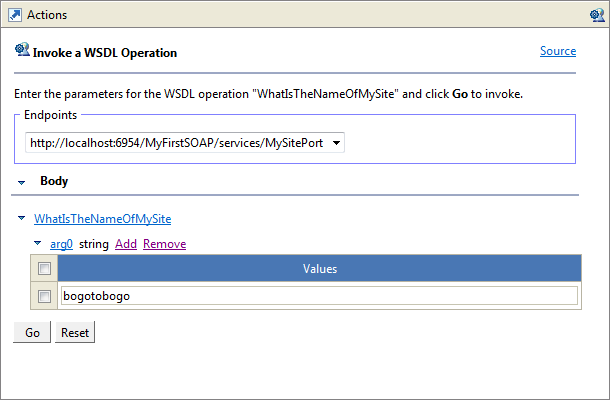
Go button will invoke Web Service, and we can investigate the results from the Console window. Indeed, we get the right answer as we expected.
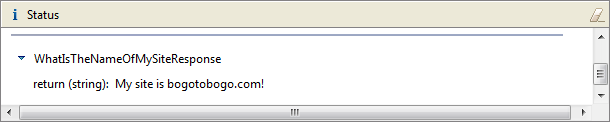
Here is the part of an output from Console window:
---------------------------- ID: 3 Address: http://localhost:8080/MyFirstSOAP/services/MySitePort?xsd=mysite_schema1.xsd Http-Method: GET Content-Type: Headers: {Accept=[text/html, image/gif, image/jpeg, *; q=.2, */*; q=.2], connection=[keep-alive], Content-Type=[null], host=[localhost:8080], user-agent=[Java/1.7.0_02]} ... ID: 4 Address: http://localhost:8080/MyFirstSOAP/services/MySitePort Encoding: UTF-8 Http-Method: POST Content-Type: text/xml; charset=utf-8 Headers: {Accept=[application/soap+xml, application/dime, multipart/related, text/*], cache-control=[no-cache], connection=[close], Content-Length=[368], content-type=[text/xml; charset=utf-8], host=[localhost:8080], pragma=[no-cache], SOAPAction=[""], user-agent=[IBM Web Services Explorer]} Payload: <soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:q0="http://ws.bogotobogo.com/" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"> <soapenv:Body> <q0:WhatIsTheNameOfMySite> <arg0>bogotobogo</arg0> </q0:WhatIsTheNameOfMySite> </soapenv:Body> </soapenv:Envelope>
6. Invoking a Web Service from the client java.
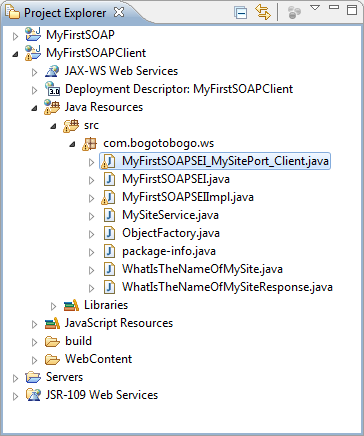
Let's modify the plain java file highlighted in the picture above.
package com.bogotobogo.ws; /** * Please modify this class to meet your needs * This class is not complete */ import java.io.File; import java.net.MalformedURLException; import java.net.URL; import javax.xml.namespace.QName; import javax.jws.WebMethod; import javax.jws.WebParam; import javax.jws.WebResult; import javax.jws.WebService; import javax.xml.bind.annotation.XmlSeeAlso; import javax.xml.ws.RequestWrapper; import javax.xml.ws.ResponseWrapper; /** * This class was generated by Apache CXF 2.5.2 * Generated source version: 2.5.2 * */ public final class MyFirstSOAPSEI_MySitePort_Client { private static final QName SERVICE_NAME = new QName("http://ws.bogotobogo.com/", "MySiteService"); private MyFirstSOAPSEI_MySitePort_Client() { } public static void main(String args[]) throws java.lang.Exception { URL wsdlURL = MySiteService.WSDL_LOCATION; if (args.length > 0 && args[0] != null && !"".equals(args[0])) { File wsdlFile = new File(args[0]); try { if (wsdlFile.exists()) { wsdlURL = wsdlFile.toURI().toURL(); } else { wsdlURL = new URL(args[0]); } } catch (MalformedURLException e) { e.printStackTrace(); } } MySiteService ss = new MySiteService(wsdlURL, SERVICE_NAME); MyFirstSOAPSEI port = ss.getMySitePort(); { System.out.println("Invoking whatIsTheNameOfMySite..."); java.lang.String _whatIsTheNameOfMySite_arg0 = "_whatIsTheNameOfMySite_arg0-16431645"; java.lang.String _whatIsTheNameOfMySite__return = port.whatIsTheNameOfMySite(_whatIsTheNameOfMySite_arg0); System.out.println("whatIsTheNameOfMySite.result=" + _whatIsTheNameOfMySite__return); } System.exit(0); } }
We can just switch the "_whatIsTheNameOfMySite_arg0-16431645" which is highlighted in red to "BOGOTOBOGO".
Then right click on the code pane, then run it. Run As > Java Application.
We the the following output in the Console window:
Invoking whatIsTheNameOfMySite... whatIsTheNameOfMySite.result=My site is BOGOTOBOGO.com!
The server is already running and the Web service already been deployed. The java code can start up the second process that will connect to the Web Service.
Frameworks using RESTful (These are my tutorials just for absolute beginners, still working on them). :
- Ruby on Rails - RESTful Web Framework
- Django - RESTful Web Framework
- Laravel Installation on Windows
Recently, I launched a couple of new sites powered by different frameworks, but they are still in their early stages. (May 2016)
- epicmath.com : AngularJS/Node, on conceptual mathematics.
- einsteinish.com : Django - on quantum physics and Universe.
- xeoman.com : Spring framework - planning to merge with AngularJS
- pygoogle.com : Ruby on Rails 4 - tapping Google services with Python
- xophist.com : Laravel 5 - lots of pictures (Amazing places on our planet)
- memonimo.com : Flask - blogging app
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization