C++ Tutorial Home - 2020
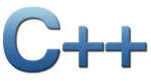
bogotobogo.com site search:
References
This tutorial is a sort of compilation work from various resources listed below, and I tried to add as many samples as possible:
- Introduction to Algorithms by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, Clifford Stein
- Bundle of Algorithms in C++, Parts 1-5: Fundamentals, Data Structures, Sorting, Searching, and Graph Algorithms (3rd Edition) (Pts. 1-5)
- Clean Code: A Handbook of Agile Software Craftsmanship, by Robert C. Martin
- Programming Language Pragmatics, Third Edition, by Michael L. Scott
- The C++ Standard Library: A Tutorial and Reference, by Nicolai M. Josuttis
- Accelerated C++: Practical Programming by Example, by Andrew Koenig, Barbara E. Moo
- C++ Primer (4th Edition) by Stanley B. Lippman, Josee Lajoie, Barbara E. Moo
- Programming: Principles and Practice Using C++ by Bjarne Stroustrup
- Code Complete: A Practical Handbook of Software Construction, Second Edition by Steve McConnell
- Exceptional C++ Style: 40 New Engineering Puzzles, Programming Problems, and Solutions by Herb Sutter
- Algorithms in a Nutshell (In a Nutshell (O'Reilly))
- Effective STL: 50 Specific Ways to Improve Your Use of the Standard Template Library by Scott Meyers
- More Effective C++: 35 New Ways to Improve Your Programs and Designs by Scott Meyers
- Effective C++: 55 Specific Ways to Improve Your Programs and Designs (3rd Edition) by Scott Meyers
- Modern Multithreading : Implementing, Testing, and Debugging Multithreaded Java and C++/Pthreads/Win32 Programs by Richard H. Carver, Kuo-Chung Tai
- Design Patterns: Elements of Reusable Object-Oriented Software by Erich Gamma, Richard Helm, Ralph Johnson, John Vlissides
- Hacking: The Art of Exploitation, 2nd Edition by Jon Erickson
- Multicore Appliction Programming for Windows, Linux, and Oracle Solaris, by Darryl Gove
- The C++ Programming Language: Special Edition by Bjarne Stroustrup
- API Design for C++ by Martin Reddy
- C++ Primer Plus, Fifth Edition, by Stephen Prata
- Beginning C++ through game programming, second edition, by Michael Dawson
- C++ GUI programming with Qt4, Second edition, by Jasmin Blanchette and Mark Summerfield
- Programming Interviews Exposed, 2nd ed., by John Mongan, et al.
- The C Programming Language, 2nd ed., by K & R
- Cracking the coding interview, Fourth edition, by Gayle Laakmann
- C++ Concurrency in Action Practical Multithreading by Anthony Williams - 2012
- The Linux Programming Interface: A Linux and UNIX System Programming Handbook by Michael Kerrisk
- Others...
Downloadable references
Thinking In C++ - Volume I (pdf)
Thinking In C++ - Volume II (pdf)
Presentation Materials: Effective C++ in an Embedded Environment (pdf) by Scott Meyers, 2012
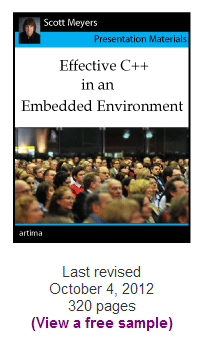
http://www.artima.com/shop/effective_cpp_in_an_embedded_environment.
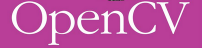
The OpenCV Tutorials Release 2.4.3 - opencv documentation (pdf)
or
Learning OpenCV: Computer Vision in C++ with the OpenCV Library by Gary Bradski and Adrian Kaehler (2008 pdf)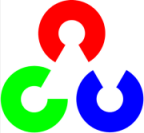
The OpenCV Reference Manual, Release 2.4.3(pdf)
Also, I started to put some info on OpenCV, here.
Meet Computer Vision professionals from OpenCV.org at LinkedIn OpenCV.org
An Effective C++11/14 Sampler
Scott Meyers - "An Effective C++11/14 Sampler" published Sept 7, 2013.
C++11/C++14 Thread Tutorials
C++11 1. Creating Threads
C++11 2. Debugging with Visual Studio 2013
C++11 3. Threading with Lambda Function
C++11 4. Rvalue and Lvalue
C++11 5. Move semantics and Rvalue Reference
C++11 5B. Move semantics - more samples for move constructor
C++11 6. Thread with Move Semantics and Rvalue Reference
C++11 7. Threads - Sharing Memory and Mutex
C++11 8. Threads - Race Conditions
C++11 9. Threads - Deadlock
C++11 10. Threads - Condition Variables
C++11 11. Threads - unique futures (std::future<>) and shared futures (std::shared_future<>).
C++11 12. Threads - std::promise
C++11/C++14 New Features
initializer_list
Uniform initialization
Type Inference (auto) and Range-based for loop
The nullptr and strongly typed enumerations
Static assertions and Constructor delegation
override and final
default and delete specifier
constexpr and string literals
Lambda functions and expressions
std::array container
Rvalue and Lvalue (from C++11 Thread tutorial)
Move semantics and Rvalue Reference (from C++11 Thread tutorial)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization