Standard Template Library II
- Set - 2020
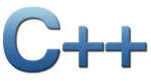
bogotobogo.com site search:
Standard Template Library (STL) II - Associative Containers: Set
Sets and multisets sort their elements automatically. Sets do not allow duplicates while multisets allow duplicates.
#include <iostream> #include <set> using namespace std; struct Compare { bool operator()(const int &a;, const int &b;) const { return a % 10 < b % 10; } }; int main() { const int a[] = {8, 2, 24, 9, 7, 3, 4, 6, 15, 2}; const int count = sizeof(a) / sizeof(a[0]); for(int i = 0; i < count; i++) cout << a[i] << " "; cout << endl; set<int> s(a, a + count); set<int>::iterator it; for(it = s.begin(); it != s.end(); ++it) cout << *it << " "; cout << endl; set<int, Compare>::iterator sit; set<int, Compare> new_s(s.begin(), s.end()); for(sit = new_s.begin(); sit != new_s.end(); ++sit) cout << *sit << " "; return 0; }
Output:
8 2 24 9 7 3 4 6 15 2 2 3 4 6 7 8 9 15 24 2 3 4 15 6 7 8 9
As we see from the output of the second line, set srots its element automatically and removes any duplicates. The third line was sorted with the last digits.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization