C++ Tutorial - Template Specialization - 2020
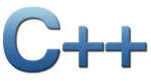
This tutorial will give the idea of the template specialization, but will be limited to just the basic.
We use templates when we need functions/classes that apply the same algorithm to a several types. So we can use the same function/class regardless of the types of the argument or result.
In other words, a template is a mechanism that allows a programmer to use types as parameters for a class or a function. The compiler then generates a specific class or function when we later provide specific types as arguments. In a sense, templates provide static (compile-time) polymorphism, as opposed to dynamic (run-time) polymorphism. A function/class defined using template is called a generic function/class, and the ability to use and create generic functions/classes is one of the key features of C++.
However, sometimes a template cannot (or should not) be applied to a certain types of data.
In the example below, we have add() function which takes two parameter and returns the same type of data after adding the two args.
#include <iostream> using namespace std; template <typename T> T add(T x, T y) { cout << "Normal template\n"; return x+y; } // specialized function template<> char add<char>(char x, char y) { cout << "Specialized template\n"; int i = x-'0'; int j = y-'0'; return i+j; } int main() { int a = 1, b = 2; cout << add(a, b) << endl; double c = 3.0, d = 5.5; cout << add(c,d) << endl; char e='e', f='f'; cout << add(e,f); return 0; }
When we designed the function add(T x, T y), the meaning was clear: add the two numbers. But when the user feeds characters into the parameter, the meaning is not obvious. So, if the intention of the design is different from the initial one, we may want to redefine the operation in a separate template. In other words, we do specialize the function.
#include <iostream> using namespace std; // Normal class template <typename T> class A { public: A(){ cout << "A()\n"; } T add(T x, T y); }; template <typename T> T A<T>::add(T x, T y) { return x+y; } // Specialized class template <> class A <char> { public: A() { cout << "Special A()\n"; } char add(char x, char y); }; // template <> <= this is not needed if defined outside of class char A<char>::add(char x, char y) { int i = x-'0'; int j = y-'0'; return i+j; } int main() { A<int> intA; A<double> doubleA; A<char> charA; int a = 1, b = 2; cout << intA.add(a,b) << endl; double c = 3.0, d = 5.5; cout << doubleA.add(c,d) << endl; char e='e', f='f'; cout << charA.add(e,f); return 0; }
Output:
A() A() Special A() 3 8.5 k
Same story as was in the previous section.
But we need to look at syntax carefully. For example, the template <> is not needed if we define a method of a class outside.
For more on template specialization, please visit Templates.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization