Virtual Destruictor and shared_ptr - 2020
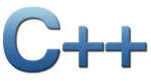
In the following example, we created a variation of an Iris as a child class which is Setosa. Then, we we tried to delete the derived object, however, the destructor of the class was not called. Only the destructor of the parent class was called. As a result, the child class object was partially destructed:
#include <iostream> using namespace std; struct Iris { ~Iris() { cout << "Iris dtor\n"; } }; struct Setosa : public Iris { ~Setosa() { cout << "Setosa dtor\n"; } }; int main() { Iris *i = new Setosa; delete i; }
Output:
Iris dtor
In the previous section, when we do delete i;, the pointer i was bound to the Iris not the derived class Setosa even though it was pointing to Setosa object (Iris *i = new Setosa;). The binding was done in compile time not in runtime. So, when the compiler saw delete i;, it knew what to do: track the pointer type not the object type it's referencing.
Therefore, the fix for the issue is doing the binding at run time polymorphically (dynamic binding). We only need virtual keyword to achieve the goal.
#include <iostream> using namespace std; struct Iris { virtual ~Iris() { cout << "Iris dtor\n"; } }; struct Setosa : public Iris { ~Setosa() { cout << "Setosa dtor\n"; } }; int main() { Iris *i = new Setosa; delete i; }
Output:
Setosa dtor Iris dtor
The destructor of a derived is called and then the parent's portion of the derived object. A complete child object destruction!
If we use boost::shared_ptr, we may not need virtual destructor since the smart point will take care of it for us:
// iris.cpp #include <boost/shared_ptr.hpp> #include <iostream> using namespace std; struct Iris { ~Iris() { cout << "Iris dtor\n"; } }; struct Setosa : public Iris { ~Setosa() { cout << "Setosa dtor\n"; } }; int main() { boost::shared_ptr<Iris> i(new Setosa); return 0; }
Compile:
c++ -I /usr/local/boost_1_55_0 iris.cpp -o iris
Output:
Setosa dtor Iris dtor
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization