Type Cast Operators - 2020
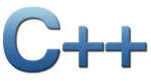
Here is the list of type-conversions.
a = dynamic_cast<T*>(p)
Try to convert p into a T*. It may return 0a = dynamic_cast<T&>(*p)
Try to convert *p into a T&. It may throw bad_casta = static_cast<T>(p)
Convert p into a T if a T can be conterted into p's type.a = reinterpret_cast<T>(p)
Convert p into a T represented by the same bit pattern.a =const_cast<T>(p)
Convert p into a T by adding or subtracting const.a = (T)v
C-style cast.a = T(v)
Functional cast.
As a preview, take the following code and guess which line compiles.
struct Foo{}; struct Bar{}; int main(int argc, char** argv) { Foo* f = new Foo; Bar* b1 = f; // (1) Bar* b2 = static_cast<Bar*>(f); // (2) Bar* b3 = dynamic_cast<Bar*>(f); // (3) Bar* b4 = reinterpret_cast<Bar*>(f); // (4) Bar* b5 = const_cast<Bar*>(f); // (5) return 0; }
Answer is only line (4) compiles without any complain. Only reinterpret_cast can be used to convert a pointer to an object to a pointer to an unrelated object type. The dynamic_cast would fail at run-time, however on most compilers it will also fail to compile because there are no virtual functions in the class of the pointer being casted.
const_cast is typically used to cast away the constness of objects. It is the only C++ style that can do this.
The syntax is:
const_cast < type-name > (expression)
The reason for this operator is that we may have a need for a value that is constant most of the time but that can be changed occasionally. In such a case, we can declare the value as const and use const_cast when we need to alter the value.
Here is a simple example:
#include <iostream> #include <cstring> using namespace std; int main () { string str("A123456789"); const char *cstr = str.c_str(); char *nonconst_cstr = const_cast<char *> (cstr) ; *nonconst_cstr ='B'; cout << nonconst_cstr << endl; return 0; }
Because we casted away the constness of the string, we were able to modify the string from "A123456789" to "B1234567889".
reinterpret_cast is intended for low-level casts that yield implementation-dependent and it would not be portable.
This cast is used for reinterpreting a bit pattern. It is not guaranteed to be portable. In fact, it is best to assume that reinterpret_cast is not portable at all. A typical example is an int-to-pointer to get a machine address into a program:
Register* a = reinterpret_cast<Register*>(0xfa);
This example is the typical use of a reinterpret_cast. We are telling the compiler that the part of memory starting with 0xfa is to be considered a Register.
It converts any pointer type to any other pointer type, even of unrelated classes. The operation result is a simple binary copy of the value from one pointer to the other. All pointer conversions are allowed: neither the content pointed nor the pointer type itself is checked.
We can cast a pointer type to an integer type that's large enough to hold the pointer representation, but we can't cast a pointer to a smaller integer type or to a floating-point type. The format in which this integer value represents a pointer is platform-specific. We can't cast a function pointer to a data pointer or vice versa.
The syntax is:
reinterpret_cast < type-name > (expression)
Here is an example code:
#include <iostream> using namespace std; struct data { short a; short b; }; int main () { long value = 0xA2345678; data* pdata = reinterpret_cast<data*> (&value;); cout << pdata->a << endl; return 0; }
Output on my machine: 22136 which is 2 bytes of value.
Another example might be:
class A {}; class B {}; int main() { A * pA = new A; B * pB = reinterpret_cast<B*>(pA); }
In the following code (b1-b5), only Bar* b5 = reinterpret_cast<Bar*> compiles.
struct Foo {}; struct Bar {}; int main() { Foo* f = new Foo; Bar* b1 = f; Bar* b2 = static_cast<Bar*>(f); Bar* b3 = dynamic_cast<Bar*>(f); Bar* b4 = const_cast<Bar*>(f); Bar* b5 = reinterpret_cast<Bar*>(f); return 0; }
Only reinterpret_cast can be used to convert a pointer to an object to a pointer to an unrelated object type. The dynamic cast would fail at run-time, however on most compilers it will also fail to compile because there are no virtual functions in the class of the pointer being casted.
Normally, the pointer comparison between different types are undefined. Here is the example of using reinterpret_cast for pointer comparison.
#include <iostream> class A{}; class B{}; class C:public A, public B{}; int main() { C d; A *a = &d; B *b = &d; bool bool1 = reinterpret_cast<char*>(a) == reinterpret_cast<char*>(&d;); bool bool2 = b == &d; bool bool3 = reinterpret_cast<char*>(a) == reinterpret_cast<char*>(b); std::cout << bool1 << bool2 << bool3 << std::endl; // 110 return 0; }
Base pointer (a and b) and the address of the derived object &d; are equal but a and b are different as expected.
Here is one of the threads related to the comparing pointers. How to compare pointers?.
static_cast can be used to force implicit conversions such as non-const object to const, int to double. It can be also be used to perform the reverse of many conversions such as void* pointers to typed pointers, base pointers to derived pointers. But it cannot cast from const to non-const object. This can only be done by const_cast operator.
The syntax is:
static_cast < type-name > (expression)
It's valid only if type_name can be converted implicitly to the same type that expression has, or vise versa. Otherwise, the type cast is an error.
class Base {}; class Derived : public Base {}; class UnrelatedClass {}; int main() { Base base; Derived derived; // #1: valid upcast Base *pBase = static_cast<Base *>(&derived;); // #2: valid downcast Derived *pDerived = static_cast<Derived *> (&base;); // #3: invalid, between unrelated classes UnrelatedClass *pUnrelated = static_cast<UnrelatedClass *> (&derived;); }
In the example, the conversion from Base to Derived and Derived to Base are valid, but a conversion from Derived to UnrelatedClass is disallowed.
The #1 conversion here is valid because an upcast can be done explicitly. The #2 conversion, from a base-class pointer to a derived-class pointer, can't be done without an explicit type conversion. But because the type cast in the other direction can be made without a type cast, it's valid to use static_cast for a downcast. However, pDerivded would point to an incomplete object of the class and could lead to runtime errors if dereferenced.
As we saw in the example, static_cast can perform conversions between pointers to related classes, not only from the derived class to its base, but also from a base class to its derived.
This ensures that at least the classes are compatible if the proper object is converted, but no safety check is performed during runtime to check if the object being converted is in fact a full object of the destination type.
Therefore, it is up to the programmer to ensure that the conversion is safe. On the other side, the overhead of the type-safety checks of dynamic_cast is avoided.
dynamic_cast is used to perform safe downcasting, i.e., to determine whether an object is of a particular type in an inheritance hierarchy. It is the only cast that may have a significant runtime cost.
Look at the dynamic_cast of C++ Tutorial - dynamic_cast.
This is from Google C++ Style Guide.
C++ introduced a different cast system from C that distinguishes the types of cast operations. Use C++ casts like static_cast<>(). Do not use other cast formats like int y = (int)x; or int y = int(x);.
- Pros
The problem with C casts is the ambiguity of the operation; sometimes we are doing a conversion (e.g., (int)3.5) and sometimes we are doing a cast (e.g., (int)"hello"); C++ casts avoid this. Additionally C++ casts are more visible when searching for them. - Cons
The syntax is nasty. - Decision
Do not use C-style casts. Instead, use these C++-style casts.- Use static_cast as the equivalent of a C-style cast that does value conversion, or when we need to explicitly up-cast a pointer from a class to its superclass.
- Use const_cast to remove the const qualifier.
- Use reinterpret_cast to do unsafe conversions of pointer types to and from integer and other pointer types. Use this only if we know what we are doing and we understand the aliasing issues.
- For dynamic_cast, visit RTTI - pros and cons.
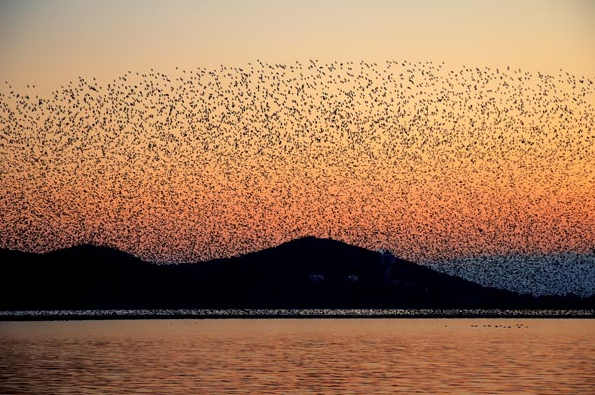
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization