AngularJS Framework : Introduction
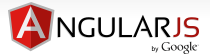
We will learn all the basics of AngularJS: directives, expressions, templates, and data binding.
What else we need to know about AngularJS?
Controllers, Modules, Events, DOM, Forms, Input, Validation, Http, and much more.
AngularJS is a JavaScript MVC framework developed by Google.
It can be added to an HTML page with a <script> tag. It enables us to create single-page applications that only require HTML, CSS, and JavaScript on the client side.
AngularJS extends HTML attributes with Directives and Data binding to HTML with Expressions.
AngularJS is quite new.
Version 1.0 was released in 2012 by Misko Hevery, a Google employee, started to work on AngularJS in 2009. The idea turned out very good, and the project is now officially backed by a Google development team.
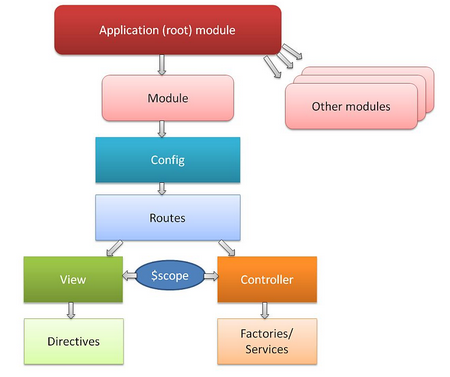
Picture from How to structure large angularJS applications
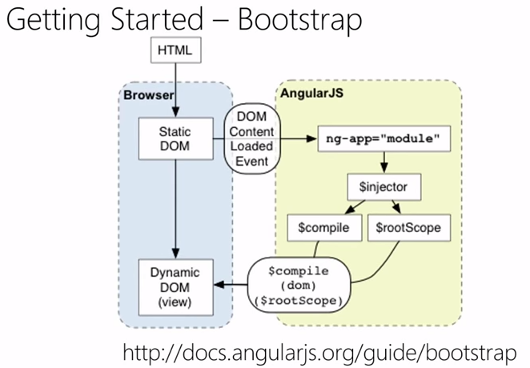
Here are some of the characteristics of AngularJS:
- Data-binding framework
Data-binding is an automatic way of updating the view whenever the model changes, as well as updating the model whenever the view changes. The HTML template is compiled in the browser.The compilation step creates pure html, upon which the browser re-renders into the live view. The step is repeated for subsequent page views.
In traditional server-side HTML programming, concepts such as controller and model interact within a server process to produce new HTML views.
In the AngularJS framework, however, the controller and model state are maintained within the client browser.
Therefore, new pages are generated without any interaction with a server - wiki - Single-page application (SPA).
As a consequence, the page is updated only the hash fragments (ULR/#) with the same URL.
- Two way data-binding
The most prominent feature that separates AngularJS from Backbone.js library is in the way models and views are synchronized.AngularJS supports two way data-binding while other frameworks such as Backbone.js relies heavily on boilerplate code to harmonize its models and views.
- AngularJS ng-directives
AngularJS ng-directives extend HTML by providing directives that add functionality to our markup and allow us to create powerful dynamic templates.It enhances HTML by attaching directives to our pages with new attributes or tags and expressions in order to define very powerful templates directly in our HTML.
We can also create our own directives, crafting reusable components that fill our needs and abstracting away all the DOM manipulation logic.
- Facilitate building single page application (SPA)
In a single page web application, we only have one "real" HTML page whose content can be changed in Javascript without having to download a new page.The new content is created programmatically in Javascript using templates.
SPA has advantages like performances (since we're not downloading a new HTML page each time as we navigate to a new page).
- Page never reloads
- No server-side page rendering
- Note: description about SPA from wiki : single-page application (SPA):
A single-page application (SPA) is a web application or web site that fits on a single web page with the goal of providing a more fluent user experience similar to a desktop application.
In a SPA, either all necessary code - HTML, JavaScript, and CSS - is retrieved with a single page load, or the appropriate resources are dynamically loaded and added to the page as necessary, usually in response to user actions.
The page does not reload at any point in the process, nor does control transfer to another page, although the location hash can be used to provide the perception and navigability of separate logical pages in the application, as can the HTML5 pushState() API.
Interaction with the single page application often involves dynamic communication with the web server behind the scenes. - Based on the Model View Controller concept.
- Provide solutions for:
- Routing - handling updates to the URL hash fragment.
- Templating - dynamically creating and updating HTML based on templates and models.
- Data binding - synchronizing the model and user interface.
- HTML is the view!
- REST APIs should provide Perfect JSON.
- Communication is one way:
Directives->HTML->Controller->Services. - The controller DOES NOT manipulate the DOM (use directives).
- Single-responsibility principle for controllers, services and directives.
From AngularJS Fundamentals for Rapid HTML5 Development
Download it from https://angularjs.org/.
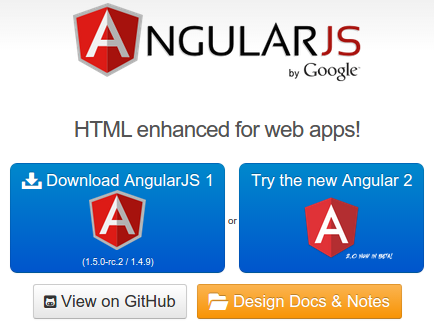
AngularJS is a JavaScript framework.
It is a library written in JavaScript.
AngularJS is distributed as a JavaScript file, and can be added to a web page with a script tag.
There are two types of angular script URLs we can point to, one for development and one for production:
- angular.js - This is the human-readable, non-minified version, suitable for web development.
- angular.min.js - This is the minified version, usually used in production.
For angular.min.js, we can use it like this:
<script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script>
AngularJS extends HTML with ng-directives:
- The ng-app directive defines an AngularJS application.
It is a directive that bootstraps AngularJS and designate the caller element as the root.
The usual place for ng-app is either <html> or <body>. - The ng-model directive binds the value of HTML controls (input, select, textarea) to application data.
- The ng-bind directive binds application data to the HTML view.
The following code demonstrates the usage of the ng-directives:
<!DOCTYPE html> <html> <head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> </head> <body> <div ng-app=""> <p>Type anything into the input box:</p> <p>Name: <input type="text" ng-model="name"></p> <p ng-bind="name"></p> </div> </body> </html>
AngularJS starts automatically when the web page has loaded:
- The ng-app directive tells AngularJS that the <div> element is the "owner" of an AngularJS application.
- The ng-model directive binds the value of the input field to the application variable name.
It is a directive that binds form elements such as input, select, checkboxes, or textarea to a property called $scope. - The ng-bind directive binds the innerHTML of the <p> element to the application variable name.
We'll get to the details in our subsequent tutorials.
This sample has basic features of AngularJS.
Other features of AngularJS come later in subsequent tutorials.
Ok, the result first!
To make the app to work, we need 4 files: index.html, main.js, home.html, and viewCustomers.html.
We'll talk about them one by one:
index.html:
<!DOCTYPE html> <html> <head lang="en"> <meta charset="utf-8"> <title>AngularJS Routing</title> </head> <body> <div ng-app="mainApp"> <div ng-view></div> </div> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular-route.min.js"></script> <script type="text/javascript" src="main.js"></script> </body> </html>
The ngView directive is used to display the HTML templates or views in the specified routes.
Every time the current route changes, the included view (from mainApp module) changes with it according to the configuration of the $route service.
The mainApp is defined in main.js file as below:
main.js:
var app = angular.module("mainApp", ['ngRoute']); app.config(function($routeProvider) { $routeProvider .when('/home', { templateUrl: 'home.html', controller: 'customerController' }) .when('/viewCustomers', { templateUrl: 'viewCustomers.html', controller: 'customerController' }) .otherwise({ redirectTo: '/home' }); }); app.controller('customerController', function($scope) { $scope.customers = [ {name: 'Jane Stewart', city:'San Francisco'}, {name: 'Sam Jenkins', city:'Moscow'}, {name: 'Mark Andrews', city:'New York'} ]; $scope.message = "Click the link to view the customers list."; });
The main.js does:
- Configures the routes via $routeProvider.
- Prepares data for our view via Controller and $scope.
The ngRoute module provides routing and deep linking services and directives for angular applications.
$routeProvider is a key service.
It sets the configuration of urls, then maps them with the corresponding html page, and attach a controller.
The when() method takes a route as parameters.
The path is a part of the URL after the # symbol.
The route contains two properties: templateUrl and controller:
- The templateUrl property tells which HTML template AngularJS should load and display inside the div with the ngView directive.
- The controller property tells which controllers should be used with the HTML template.
When the application is loaded, the path is matched against the part of the URL after the # symbol. If no route paths matches the given URL the browser will be redirected to the path specified in the otherwise() function.
home.html is the default page of our application.
In this view, we just print out the message, which we have already initialized in the customerController.
home.html:
<div class="container"> <h2> Welcome </h2> <p>{{message}}</p> <a href="#/viewCustomers"> View customers List</a> </div>
As we can see, we have a link to the viewCustomers page.
viewCustomers.html:
<div class="container"> <h2> View Customers </h2> Search: <br/> <input type="text" ng-model="name" /> <br/> <ul> <li ng-repeat="customer in customers | filter:name">{{customer.name}} , {{customer.city}}</li> </ul> <a href="#/home"> Back</a> </div>
The <div ng-view></div> in index.html (or <ng-view></ng-view>) is the placeholder of our two views: home.html and viewCustomers.html.
The ng-view tag simply creates a place holder where a corresponding view (html or ng-template view) can be placed based on the configuration.
----
This sample is not much different from the previous one which just displays items.
Let's make it more interesting:
we want to have additional feature for adding items to the list.
index.html:
<!DOCTYPE html> <html> <head lang="en"> <meta charset="utf-8"> <title>AngularJS sample app II</title> </head> <body> <div ng-app="mainApp"> <h2>Welcome to sample app II</h2> <p><b>Header</b> <br /> This message will stay throughout all pages. <br /> Replaceable view will be within ng-view directive. <br /> =================================================</p> <div ng-view></div> <p>=================================================</p> <p><b>Footer</b></p> </div> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular-route.min.js"></script> <script type="text/javascript" src="main.js"></script> </body> </html>
main.js:
var app = angular.module("mainApp", ['ngRoute']); app.config(function($routeProvider) { $routeProvider .when('/home', { templateUrl: 'home.html', controller: 'homeController' }) .when('/viewCustomers', { templateUrl: 'viewCustomers.html', controller: 'viewCustomersController' }) .otherwise({ redirectTo: '/home' }); }); app.controller('homeController', function($scope) { $scope.message = "$scope.message : from homeController"; }); app.controller('viewCustomersController', function($scope) { $scope.customers = [ {'name': 'Jane Stewart', 'city':'San Francisco'}, {'name': 'Sam Jenkins', 'city':'Moscow'}, {'name': 'Mark Andrews', 'city':'New York'} ]; $scope.add = function() { d = {} d['name'] = $scope.name; d['city'] = $scope.city; $scope.message = $scope.name + " " + $scope.city $scope.customers.push(d); $scope.name = ""; $scope.city = ""; $scope.message = $scope.customers; }; });
home.html:
<div class="container"> <h3> Home page (home.html) </h3> <p>{{message}}</p> <a href="#/viewCustomers"> View customers List</a> </div>
viewCustomers.html:
<div class="container"> <h2> View Customers </h2> - viewCustomers.html <ul> <li ng-repeat="customer in customers | filter:name">{{customer.name}} , {{customer.city}}</li> </ul> <br /> <p>Add a new customer in the input box:</p> <form ng-submit="add()"> Name: <input type="text" ng-model="name" placeholder="Enter name here"> <br> City: <input type="text" ng-model="city" placeholder="Enter city here"> <br> A new customer: {{name}} {{city}} <br> <input type="submit" value="Add"/> </form> <br /> <a href="#/home">Back to Home</a> </div>
AngularJS
- Single-page application
- Data Binding Frameworks
- Youtube - Introduction to Angular.js in 50 Examples
- Youtube - AngularJS Fundamentals In 60-ish Minutes
- AngularJS Fundamentals for Rapid HTML5 Development
- Everything you need to understand to start with AngularJS
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization