Docker Hello World Application
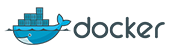
Docker allows us to run applications inside containers. Running an application inside a container takes a single command: docker run
or docker container run
Prior to docker 1.13 the docker run command was only available. The cli commands were then refactored to have the formdocker COMMAND SUBCOMMAND
, where in this case the COMMAND is container and the SUBCOMMAND is run. This was done to have a more inituitive grouping of commands since the number of commands at the time has grown substantially.
https://stackoverflow.com/questions/51247609/what-is-the-difference-between-docker-run-and-docker-container-run
Ref : Dockerizing applications: A "Hello world"
$ docker run [OPTIONS] IMAGE[:TAG|@DIGEST] [COMMAND] [ARG...]
Or
With Docker 1.13 release, the Docker commands are given a structure where a command has three parts (with a few exceptions) in addition to other options or arguments as needed -
$ docker <Management Command> <Sub-Command <Opts/Args>
Here is the list of all (Management) commands available in the current docker version (Docker version 18.06.1-ce):
checkpoint Manage checkpoints config Manage Docker configs container Manage containers image Manage images network Manage networks node Manage Swarm nodes plugin Manage plugins secret Manage Docker secrets service Manage services stack Manage Docker stacks swarm Manage Swarm system Manage Docker trust Manage trust on Docker images volume Manage volumes
The docker container run command runs a process in a new container. It starts a process with its own file system, its own networking, and its own isolated process tree.
The IMAGE which starts the process may define defaults related to the process that will be run in the container, the networking to expose, and more, but docker container run gives final control to the operator or administrator who starts the container from the image. For that reason, docker container run has more options than any other Docker command.If the IMAGE is not already loaded, then docker container run will pull the IMAGE, and all image dependencies, from the repository in the same way running docker pull IMAGE, before it starts the container from that image.
We need to have a disk image to make the virtualization work. The disk image represents the system we're running on and they are the basis of containers. Docker registry is a registry of already existing images that we can use to run and create containerized applications.
$ docker container run ubuntu:16.04 /bin/echo 'Hello world' Hello world
Note that we just launched our first container!
Let's check what the docker container run command did.
- First, we specified the docker binary and the command we wanted to execute, container + run. The container run combination runs containers.
- Next we specified an image: ubuntu:16.04. This is the source of the container we ran. Docker calls this an image. In this case, we used an Ubuntu 16.04 operating system image. When we specify an image, Docker looks first for the image on our Docker host. If it can't find the image, then it downloads the image from the public image registry: Docker Hub.
- Next, we told Docker what command to run inside our new container:
/bin/echo 'Hello world'
When our container was launched, Docker created a new Ubuntu 16.04 environment, and then executed the /bin/echo command inside it. We saw the result on the command line:Hello world
Docker containers only run as long as the command we specify is active. So, as soon as the "Hello world" was echoed, the container stopped. It's not running anymore.
We can check if there any running containers using docker container ps:
$ docker container ps CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
We see no containers are running!
Let's try the docker container run command again. But this time, let's specify a new command to run in our container.
$ docker container run -t -i ubuntu:16.04 /bin/bash root@7c5c3139f14f:/#
Here, as we did before, we issued the docker container run command, and launched an ubuntu:16.04 image.
We used two flags: -t and -i.
- The -t flag assigns a pseudo-tty or terminal inside our new container
- The -i flag allows us to make an interactive connection by grabbing the standard input (STDIN) of the container.
We have a new command for our container to run: /bin/bash which launches a Bash shell inside our container.
So, when our container is launched, we can see what we've got: a command prompt inside it!
root@7c5c3139f14f:/#
We can play around inside this container. When we're done, we can use the exit command or enter Ctrl-D to finish.
root@7c5c3139f14f:/# exit exit
Once the Bash shell process has finished, the container is stopped.
Let's create a container that runs as a daemon (detached), and we can do this with the docker container run command:
$ docker container run -d ubuntu:16.04 /bin/sh -c "while true; do echo hello world; sleep 1; done" 7a7fa9cc294d9ef7b32d5fe6c8a9da5b82d4500ab0710013d96bea26dbadbd6d
Where's our "Hello world" output? Let's look at what we've run here. We ran docker container run but this time we used -d. The -d flag tells Docker to run the container and put it in the background, to daemonize it.
Note that we also used the same image: ubuntu:16.04 as before.
Then, we run the following command:
/bin/sh -c "while true; do echo hello world; sleep 1; done"
This is our daemon: a shell script that echoes "hello world" forever.
However, we cannot see any "hello world", instead, Docker has returned a really long string:
54c0e4b98a6e57e7c0fd4c4f9b1250c6ddf71b1e3e77175a70f58150a71ad540
This is a container ID, and it uniquely identifies a container. So, we can play with it. Actually, we can use this container ID to see what's happening with our "hello world" daemon.
First, let's make sure our container is running. We can do that with the docker ps or docker container pscommand. The docker container ps command queries the Docker daemon for information about all the containers it knows about.
$ docker container ps CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 54c0e4b98a6e ubuntu:16.04 "/bin/sh -c 'while t 16 minutes ago Up 16 minutes nostalgic_bassi
Here we can see our daemonized container. The docker container ps has returned some useful information about it, starting with a shorter variant of its container ID: 7a7fa9cc294d. We can also see the image we used to build it, ubuntu:16.04, the command it is running, its status and an automatically assigned name, nostalgic_bassi.
$ docker logs nostalgic_bassi hello world hello world hello world ...
The docker logs command looks inside the container and returns its standard output: in this case, the output of our command is "hello world".
Now we can see our daemon is working, and we've just created our first Docker application!
How can we stop our daemonized container?
docker stop!
The docker container stop command tells Docker to stop the running container. If it succeeds, it will return the name of the container it has just stopped:
$ docker container stop nostalgic_bassi nostalgic_bassi
Let's check if it really stopped. We can do it with the docker container ps command:
$ docker container ps CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
No docker container is running now!
Docker & K8s
- Docker install on Amazon Linux AMI
- Docker install on EC2 Ubuntu 14.04
- Docker container vs Virtual Machine
- Docker install on Ubuntu 14.04
- Docker Hello World Application
- Nginx image - share/copy files, Dockerfile
- Working with Docker images : brief introduction
- Docker image and container via docker commands (search, pull, run, ps, restart, attach, and rm)
- More on docker run command (docker run -it, docker run --rm, etc.)
- Docker Networks - Bridge Driver Network
- Docker Persistent Storage
- File sharing between host and container (docker run -d -p -v)
- Linking containers and volume for datastore
- Dockerfile - Build Docker images automatically I - FROM, MAINTAINER, and build context
- Dockerfile - Build Docker images automatically II - revisiting FROM, MAINTAINER, build context, and caching
- Dockerfile - Build Docker images automatically III - RUN
- Dockerfile - Build Docker images automatically IV - CMD
- Dockerfile - Build Docker images automatically V - WORKDIR, ENV, ADD, and ENTRYPOINT
- Docker - Apache Tomcat
- Docker - NodeJS
- Docker - NodeJS with hostname
- Docker Compose - NodeJS with MongoDB
- Docker - Prometheus and Grafana with Docker-compose
- Docker - StatsD/Graphite/Grafana
- Docker - Deploying a Java EE JBoss/WildFly Application on AWS Elastic Beanstalk Using Docker Containers
- Docker : NodeJS with GCP Kubernetes Engine
- Docker : Jenkins Multibranch Pipeline with Jenkinsfile and Github
- Docker : Jenkins Master and Slave
- Docker - ELK : ElasticSearch, Logstash, and Kibana
- Docker - ELK 7.6 : Elasticsearch on Centos 7
- Docker - ELK 7.6 : Filebeat on Centos 7
- Docker - ELK 7.6 : Logstash on Centos 7
- Docker - ELK 7.6 : Kibana on Centos 7
- Docker - ELK 7.6 : Elastic Stack with Docker Compose
- Docker - Deploy Elastic Cloud on Kubernetes (ECK) via Elasticsearch operator on minikube
- Docker - Deploy Elastic Stack via Helm on minikube
- Docker Compose - A gentle introduction with WordPress
- Docker Compose - MySQL
- MEAN Stack app on Docker containers : micro services
- MEAN Stack app on Docker containers : micro services via docker-compose
- Docker Compose - Hashicorp's Vault and Consul Part A (install vault, unsealing, static secrets, and policies)
- Docker Compose - Hashicorp's Vault and Consul Part B (EaaS, dynamic secrets, leases, and revocation)
- Docker Compose - Hashicorp's Vault and Consul Part C (Consul)
- Docker Compose with two containers - Flask REST API service container and an Apache server container
- Docker compose : Nginx reverse proxy with multiple containers
- Docker & Kubernetes : Envoy - Getting started
- Docker & Kubernetes : Envoy - Front Proxy
- Docker & Kubernetes : Ambassador - Envoy API Gateway on Kubernetes
- Docker Packer
- Docker Cheat Sheet
- Docker Q & A #1
- Kubernetes Q & A - Part I
- Kubernetes Q & A - Part II
- Docker - Run a React app in a docker
- Docker - Run a React app in a docker II (snapshot app with nginx)
- Docker - NodeJS and MySQL app with React in a docker
- Docker - Step by Step NodeJS and MySQL app with React - I
- Installing LAMP via puppet on Docker
- Docker install via Puppet
- Nginx Docker install via Ansible
- Apache Hadoop CDH 5.8 Install with QuickStarts Docker
- Docker - Deploying Flask app to ECS
- Docker Compose - Deploying WordPress to AWS
- Docker - WordPress Deploy to ECS with Docker-Compose (ECS-CLI EC2 type)
- Docker - WordPress Deploy to ECS with Docker-Compose (ECS-CLI Fargate type)
- Docker - ECS Fargate
- Docker - AWS ECS service discovery with Flask and Redis
- Docker & Kubernetes : minikube
- Docker & Kubernetes 2 : minikube Django with Postgres - persistent volume
- Docker & Kubernetes 3 : minikube Django with Redis and Celery
- Docker & Kubernetes 4 : Django with RDS via AWS Kops
- Docker & Kubernetes : Kops on AWS
- Docker & Kubernetes : Ingress controller on AWS with Kops
- Docker & Kubernetes : HashiCorp's Vault and Consul on minikube
- Docker & Kubernetes : HashiCorp's Vault and Consul - Auto-unseal using Transit Secrets Engine
- Docker & Kubernetes : Persistent Volumes & Persistent Volumes Claims - hostPath and annotations
- Docker & Kubernetes : Persistent Volumes - Dynamic volume provisioning
- Docker & Kubernetes : DaemonSet
- Docker & Kubernetes : Secrets
- Docker & Kubernetes : kubectl command
- Docker & Kubernetes : Assign a Kubernetes Pod to a particular node in a Kubernetes cluster
- Docker & Kubernetes : Configure a Pod to Use a ConfigMap
- AWS : EKS (Elastic Container Service for Kubernetes)
- Docker & Kubernetes : Run a React app in a minikube
- Docker & Kubernetes : Minikube install on AWS EC2
- Docker & Kubernetes : Cassandra with a StatefulSet
- Docker & Kubernetes : Terraform and AWS EKS
- Docker & Kubernetes : Pods and Service definitions
- Docker & Kubernetes : Service IP and the Service Type
- Docker & Kubernetes : Kubernetes DNS with Pods and Services
- Docker & Kubernetes : Headless service and discovering pods
- Docker & Kubernetes : Scaling and Updating application
- Docker & Kubernetes : Horizontal pod autoscaler on minikubes
- Docker & Kubernetes : From a monolithic app to micro services on GCP Kubernetes
- Docker & Kubernetes : Rolling updates
- Docker & Kubernetes : Deployments to GKE (Rolling update, Canary and Blue-green deployments)
- Docker & Kubernetes : Slack Chat Bot with NodeJS on GCP Kubernetes
- Docker & Kubernetes : Continuous Delivery with Jenkins Multibranch Pipeline for Dev, Canary, and Production Environments on GCP Kubernetes
- Docker & Kubernetes : NodePort vs LoadBalancer vs Ingress
- Docker & Kubernetes : MongoDB / MongoExpress on Minikube
- Docker & Kubernetes : Load Testing with Locust on GCP Kubernetes
- Docker & Kubernetes : MongoDB with StatefulSets on GCP Kubernetes Engine
- Docker & Kubernetes : Nginx Ingress Controller on Minikube
- Docker & Kubernetes : Setting up Ingress with NGINX Controller on Minikube (Mac)
- Docker & Kubernetes : Nginx Ingress Controller for Dashboard service on Minikube
- Docker & Kubernetes : Nginx Ingress Controller on GCP Kubernetes
- Docker & Kubernetes : Kubernetes Ingress with AWS ALB Ingress Controller in EKS
- Docker & Kubernetes : Setting up a private cluster on GCP Kubernetes
- Docker & Kubernetes : Kubernetes Namespaces (default, kube-public, kube-system) and switching namespaces (kubens)
- Docker & Kubernetes : StatefulSets on minikube
- Docker & Kubernetes : RBAC
- Docker & Kubernetes Service Account, RBAC, and IAM
- Docker & Kubernetes - Kubernetes Service Account, RBAC, IAM with EKS ALB, Part 1
- Docker & Kubernetes : Helm Chart
- Docker & Kubernetes : My first Helm deploy
- Docker & Kubernetes : Readiness and Liveness Probes
- Docker & Kubernetes : Helm chart repository with Github pages
- Docker & Kubernetes : Deploying WordPress and MariaDB with Ingress to Minikube using Helm Chart
- Docker & Kubernetes : Deploying WordPress and MariaDB to AWS using Helm 2 Chart
- Docker & Kubernetes : Deploying WordPress and MariaDB to AWS using Helm 3 Chart
- Docker & Kubernetes : Helm Chart for Node/Express and MySQL with Ingress
- Docker & Kubernetes : Deploy Prometheus and Grafana using Helm and Prometheus Operator - Monitoring Kubernetes node resources out of the box
- Docker & Kubernetes : Deploy Prometheus and Grafana using kube-prometheus-stack Helm Chart
- Docker & Kubernetes : Istio (service mesh) sidecar proxy on GCP Kubernetes
- Docker & Kubernetes : Istio on EKS
- Docker & Kubernetes : Istio on Minikube with AWS EC2 for Bookinfo Application
- Docker & Kubernetes : Deploying .NET Core app to Kubernetes Engine and configuring its traffic managed by Istio (Part I)
- Docker & Kubernetes : Deploying .NET Core app to Kubernetes Engine and configuring its traffic managed by Istio (Part II - Prometheus, Grafana, pin a service, split traffic, and inject faults)
- Docker & Kubernetes : Helm Package Manager with MySQL on GCP Kubernetes Engine
- Docker & Kubernetes : Deploying Memcached on Kubernetes Engine
- Docker & Kubernetes : EKS Control Plane (API server) Metrics with Prometheus
- Docker & Kubernetes : Spinnaker on EKS with Halyard
- Docker & Kubernetes : Continuous Delivery Pipelines with Spinnaker and Kubernetes Engine
- Docker & Kubernetes : Multi-node Local Kubernetes cluster : Kubeadm-dind (docker-in-docker)
- Docker & Kubernetes : Multi-node Local Kubernetes cluster : Kubeadm-kind (k8s-in-docker)
- Docker & Kubernetes : nodeSelector, nodeAffinity, taints/tolerations, pod affinity and anti-affinity - Assigning Pods to Nodes
- Docker & Kubernetes : Jenkins-X on EKS
- Docker & Kubernetes : ArgoCD App of Apps with Heml on Kubernetes
- Docker & Kubernetes : ArgoCD on Kubernetes cluster
- Docker & Kubernetes : GitOps with ArgoCD for Continuous Delivery to Kubernetes clusters (minikube) - guestbook
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization