CI/CD Github actions
What are GitHub Actions?
As a GitHub feature, the GitHub Actions allow us to run a CI/CD pipeline to build, test, and deploy software directly from GitHub.
In short, GitHub Actions is the platform for automating tasks, while GitHub Workflows are the specific automation processes defined within repositories using YAML files.
In this section, three files will be created: .github/workflows/main.yml and app files hello.py and requirements.txt:
.github/workflows/main.yml:
name: Python CI on: push: branches: - main # Change this branch name to match your repository's default branch jobs: build: runs-on: ubuntu-latest # You can specify a different runner if needed steps: - name: Checkout code uses: actions/checkout@v2 # This step checks out your code from the repository - name: Set up Python uses: actions/setup-python@v2 with: python-version: 3.8 # Specify Python 3.8 - name: Install dependencies run: | python -m pip install --upgrade pip pip install -r requirements.txt # Install any required Python packages - name: Run Python script run: | python your_script.py # Python script
hello.py:
def main(): print("Hello, GitHub Actions!") if __name__ == "__main__": main()
requirements.txt (actually, we don't need this file):
requests==2.26.0
Then, we just push the codes:
$ git add .github requirements.txt hello.py $ git commit -m "initial commit" [main be38bf4] initial commit 3 files changed, 31 insertions(+) create mode 100644 .github/workflows/main.yml create mode 100644 hello.py create mode 100644 requirements.txt $ git remote set-url origin https://Einsteinish:ghp_*****Ebc@github.com/Einsteinish/github-actions-dummy.git $ git push Enumerating objects: 8, done. Counting objects: 100% (8/8), done. Delta compression using up to 4 threads Compressing objects: 100% (4/4), done. Writing objects: 100% (7/7), 898 bytes | 449.00 KiB/s, done. Total 7 (delta 0), reused 0 (delta 0), pack-reused 0 To https://github.com/Einsteinish/github-actions-dummy.git f37ad9d..be38bf4 main -> main
We can see the actions by Click on the "Actions" Tab of the GitHub repository.
Then, we'll see a list of our workflows. Each workflow will be listed by name, and we'll also see information about the most recent workflow runs, including their status and when they were triggered.
To see more details about a specific workflow, click on its name in the list. This will take us to the workflow's page, where we can see a history of runs and their statuses. If we click on a specific workflow run, we can view detailed logs and information about the steps that were executed during that run.

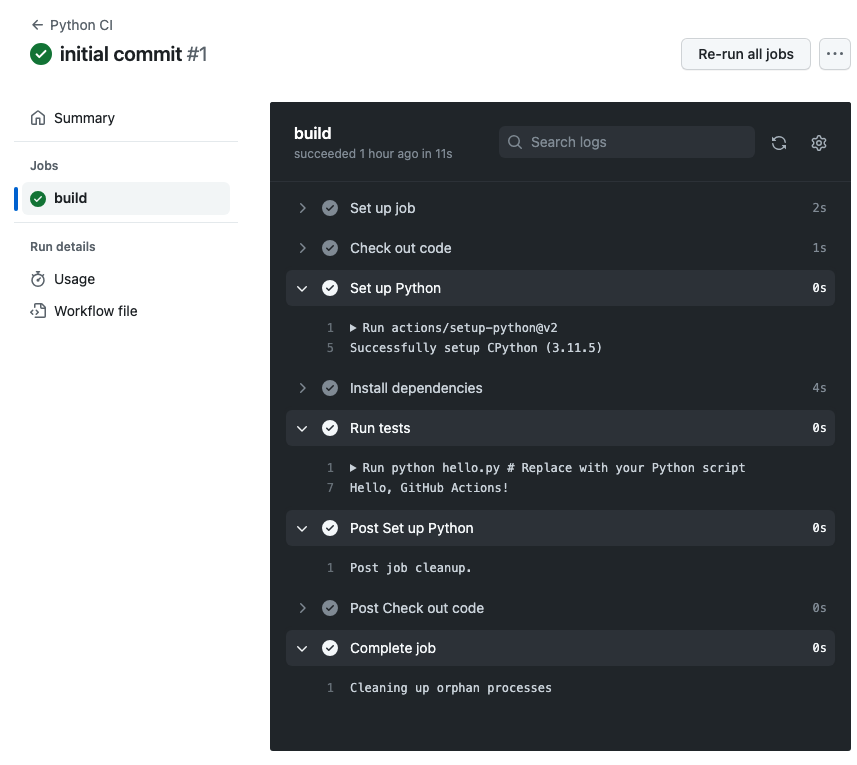
The following code repo: https://github.com/Einsteinish/github-actions has all the code including the workflow yaml file:
# This workflow uses actions that are not certified by GitHub. # They are provided by a third-party and are governed by # separate terms of service, privacy policy, and support # documentation. # This workflow will build a Java project with Gradle and cache/restore any dependencies to improve the workflow execution time # For more information see: https://docs.github.com/en/actions/automating-builds-and-tests/building-and-testing-java-with-gradle name: Java CI with Gradle on: push: branches: [ "main" ] pull_request: branches: [ "main" ] permissions: contents: read jobs: build: runs-on: ubuntu-latest steps: - uses: actions/checkout@v3 - name: Set up JDK 11 uses: actions/setup-java@v3 with: java-version: '11' distribution: 'temurin' - name: add x permission to gradlew run: chmod +x gradlew - name: Build with Gradle uses: gradle/gradle-build-action@67421db6bd0bf253fb4bd25b31ebb98943c375e1 with: arguments: build - name: Build & push Docker image uses: mr-smithers-excellent/docker-build-push@v5 with: image: dockerbogo/github-actions-gradle registry: docker.io username: ${{ secrets.DOCKER_USERNAME }} password: ${{ secrets.DOCKER_PASSWORD }}


Here is a sample of Github Actions when there is either a pull request from a branch or direct push to main branch:
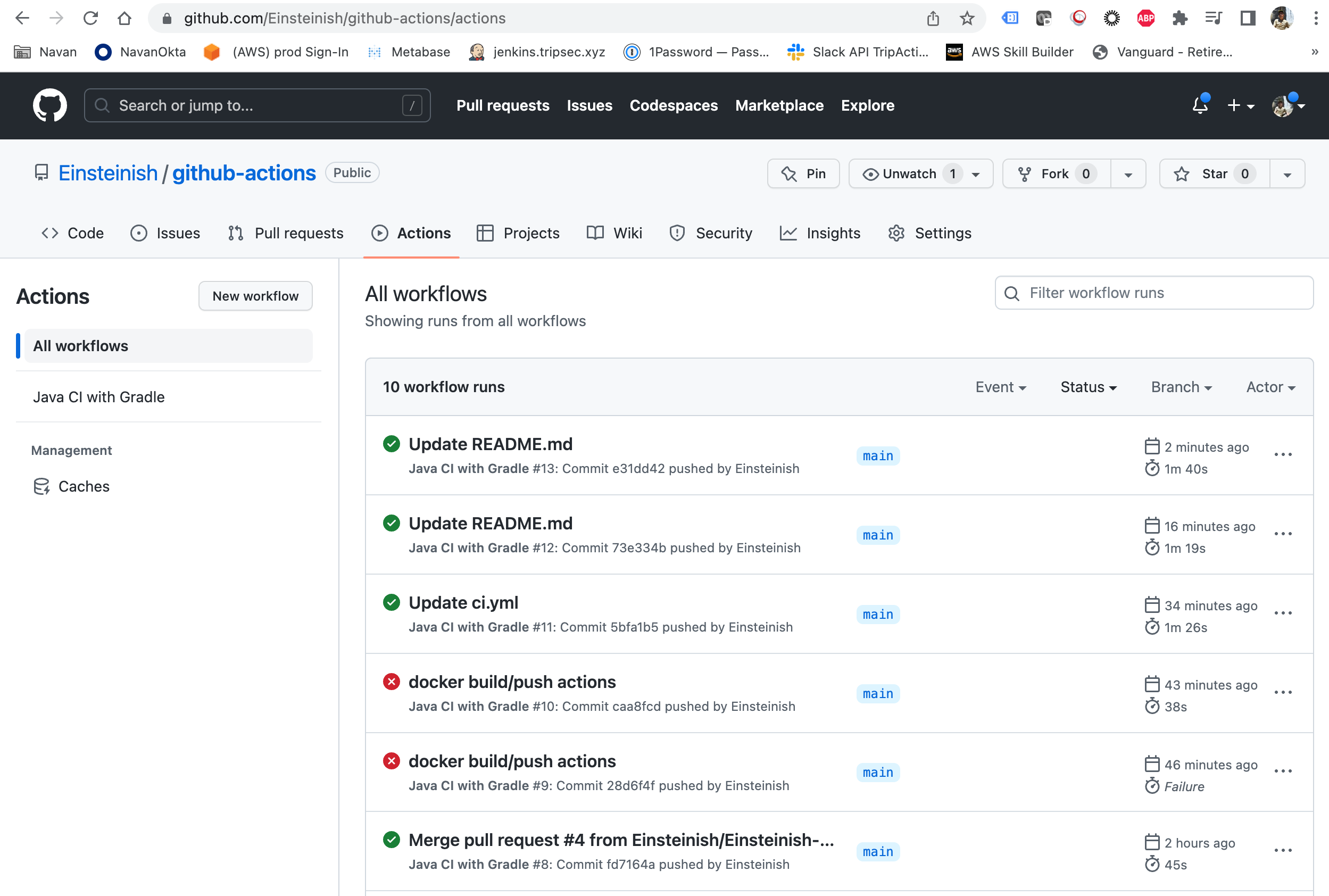
Also, a successfully pushed img in DockerHub:

DevOps
DevOps / Sys Admin Q & A
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization