Docker Cheat Sheet
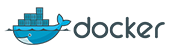
Container is runtime environments for an image. When we do docker pull <image>
, the container is not started nor created yet.
We most commonly used command is the docker run <image>
command. It downloads the image if it's not already in our local machine and starts the container once the image is ready.
- docker create: creates a writable container layer over the specified image and prepares it for running the specified command. The container ID is then printed to STDOUT. This is similar to docker run -d except the container is never started. We can then use the docker start <container_id> command to start the container at any point.
$ docker create -t -i fedora bash ... dff32a272ad4c94cd51419ee4f53c371e3c3a8cfb448a469634d4420e139d118 $ docker start -a -i dff32a272ad4c [root@dff32a272ad4 /]#
i: interactive, keep STDIN open even if not attached
t: Allocate a pseudo-TTY
a: Attach container's STDOUT and STDERR and forward all signals to the process.
- docker rename allows the container to be renamed.
- docker run creates and starts a container in one operation.
- docker rm deletes a container.
- docker update updates a container's resource limits. Example : updating multiple resource configurations for multiple containers:
$ docker rename my_container my_new_container
$ docker run -it ubuntu-ssh-k /bin/bash
$ docker rm myfedora
$ docker update --cpu-shares 512 -m 300M dff32a272ad4 happy_kare dff32a272ad4 happy_kare
- docker ps shows running containers.
- docker logs gets logs from container. (We can use a custom log driver, but logs is only available for json-file and journald in 1.10)
- docker inspect looks at all the info on a container (including IP address). To get an IP address of a running container:
- docker events gets events from container.
- docker port shows public facing port of container.
- docker top shows running processes in container.
- docker stats shows containers' resource usage statistics.
- docker diff shows changed files in the container's FS.
$ docker ps CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES e2481c1bad5d ubuntu-ssh-k:latest "/bin/bash" 10 hours ago Up 10 hours hopeful_carson
$ docker logs 839f66a78983
$ docker inspect --format '{{ .NetworkSettings.IPAddress }}' $(docker ps -q) 172.17.0.5
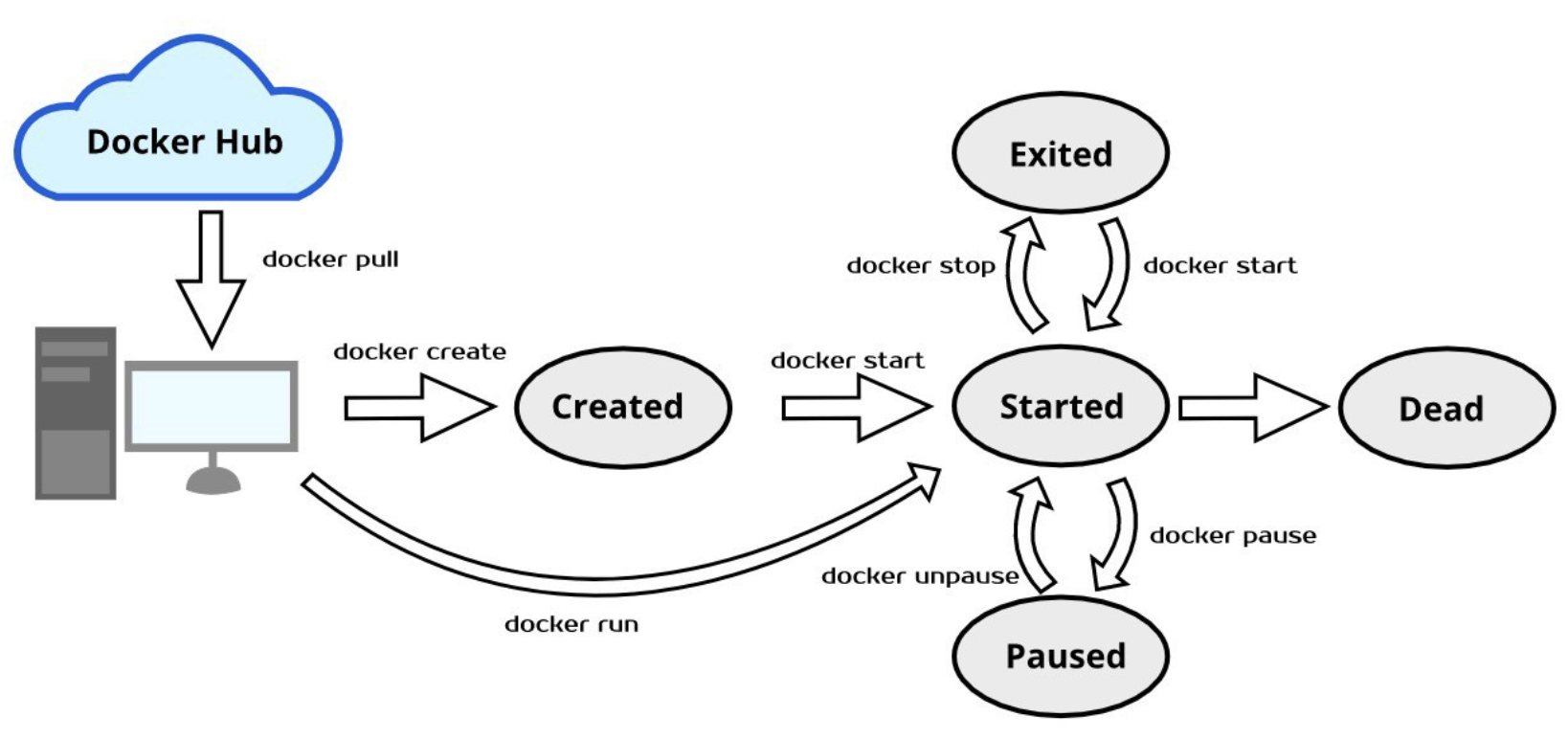
Picture credit: Get Started with Docker Lifecycle
- docker start starts a container so it is running.
- docker stop stops a running container.
- docker restart stops and starts a container.
- docker pause pauses a running container, "freezing" it in place.
- docker unpause will unpause a running container.
- docker wait blocks until running container stops.
- docker kill sends a SIGKILL to a running container.
- docker attach will connect to a running container.
Or we can use the following to get tty:$ docker exec -i -t my-nginx-1 /bin/bash
Note that we used exec to run a command in a "running" container!
- docker images : shows all images.
- docker import : creates an image from a tarball.
- docker build : creates image from Dockerfile.
- docker commit : creates image from a container, by default, the container being committed and its processes will be paused while the image is committed. This reduces the likelihood of encountering data corruption during the process of creating the commit. As an example, let's install nginx to the ubuntu container:
- -m 'added nginx start' creates a comment for this commit.
- --change='CMD ... is changing the CMD command, which is what the image will run when it is first started up. In this example, we are telling the image to run nginx in the foreground. Most base os images have CMD set to bash so we can interact with the os when attaching.
- We used "EXPOSE" to get the port exposed to the host.
- "9644a814e95a" is the name of the container we want to commit from.
- "ubuntu-nginx:version2" is our name for the new image. We appended version to the name of the container.
- docker rmi : removes (and un-tags) an image from the host node. If an image has multiple tags, using this command with the tag as a parameter only removes the tag. If the tag is the only one for the image, both the image and the tag are removed. This does not remove images from a registry. We cannot remove an image of a running container unless we use the -f option.
- docker load : loads an image from a tar archive as STDIN, including images and tags .
- docker save : saves an image to a tar archive stream to STDOUT with all parent layers, tags & versions .
$ docker run -it ubuntu /bin/bash root@bf5d24821dfa:/# apt-get update root@bf5d24821dfa:/# apt-get install nginx
While the container is running, on another terminal, we can commit the change to another image:
$ docker ps CONTAINER ID IMAGE COMMAND bf5d24821dfa ubuntu "/bin/bash" $ docker commit bf5d24821dfa ubuntu-nginx $ docker images REPOSITORY TAG IMAGE ID ubuntu-nginx latest 91cc8b745f5e $ docker run -it ubuntu-nginx root@9644a814e95a:/# $ docker ps CONTAINER ID IMAGE COMMAND 9644a814e95a ubuntu-nginx "/bin/bash" bf5d24821dfa ubuntu "/bin/bash"
Note the the new container dit not start "nginx" server. So, we can commit a container with new CMD and EXPOSE instructions:
root@9644a814e95a:/# exit $ docker commit -m 'added nginx start' --change='CMD ["nginx", "-g daemon off;"]' -c "EXPOSE 80" 9644a814e95a ubuntu-nginx:version2
We can now view our new image with the following command:
$ docker images REPOSITORY TAG IMAGE ID ubuntu-nginx version2 61918a284221
Run the new image:
$ docker run -idt ubuntu-nginx:version2 $ ps aux | grep -v grep |grep nginx root 12546 0.0 0.2 124972 9560 pts/25 Ss+ 10:21 0:00 nginx: master process nginx -g daemon off; www-data 12565 0.0 0.0 125332 3040 pts/25 S+ 10:21 0:00 nginx: worker process www-data 12566 0.0 0.0 125332 3040 pts/25 S+ 10:21 0:00 nginx: worker process $ docker inspect --format '{{ .NetworkSettings.IPAddress }}' $(docker ps -q) 172.17.0.2
We can see the index page:
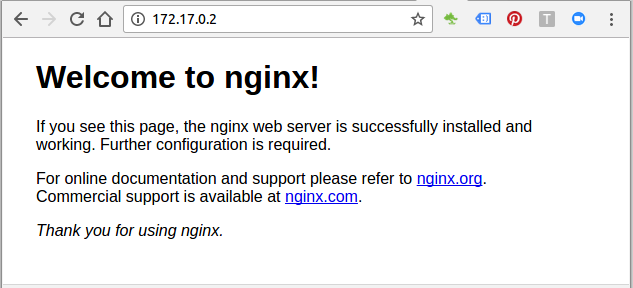
- docker history shows history of image.
- docker tag tags an image to a name (local or registry).
- docker network create
- docker network rm
- docker network ls
- docker network inspect
- docker network connect
- docker network disconnect
- docker login to login to a registry. (see docker push)
- docker logout to logout from a registry.
- docker search searches registry for image.
- docker pull pulls an image from registry to local machine.
- docker push pushes an image to the registry from local machine. First, we'll install nginx on top of ubuntu, and then commit it. After login to DockerHub, we'll tag the image and push it:
$ docker login --username=dockerbogo Password: Login Succeeded
$ docker logout Removing login credentials for https://index.docker.io/v1/
$ docker search mysql NAME DESCRIPTION STARS OFFICIAL AUTOMATED mysql MySQL is a widely used, open-source relati... 2797 [OK] mysql/mysql-server Optimized MySQL Server Docker images. Crea... 182 [OK] ...
$ docker pull ubuntu latest: Pulling from ubuntu 20ee58809289: Pull complete f905badeb558: Pull complete 119df6bf2a3a: Pull complete 94d6eea646bc: Pull complete bb4eabee84bf: Pull complete Digest: sha256:85af8b61adffea165e84e47e0034923ec237754a208501fce5dbeecbb197062c Status: Downloaded newer image for ubuntu:latest
Docker images can consist of multiple layers. In the example above, the image consists of five layers.
$ docker run -it ubuntu:latest /bin/bash root@846346c3482f:/# apt-get update root@846346c3482f:/# apt-get install nginx $ docker commit 846346c3482f ubuntu-with-nginx $ docker images REPOSITORY TAG IMAGE ID CREATED SIZE ubuntu-with-nginx latest de412f7faba5 16 minutes ago 212 MB $ docker login --username=dockerbogo Password: Login Succeeded $ docker tag de412f7faba5 dockerbogo/ubuntu-with-nginx:myfirstpush $ docker push dockerbogo/ubuntu-with-nginx:myfirstpush The push refers to a repository [docker.io/dockerbogo/ubuntu-with-nginx] 44f391d61c42: Pushed 73e5d2de6e3e: Mounted from library/ubuntu 08f405d988e4: Mounted from library/ubuntu 511ddc11cf68: Mounted from library/ubuntu a1a54d352248: Mounted from library/ubuntu 9d3227c1793b: Mounted from library/ubuntu myfirstpush: digest: sha256:a24d914b8e3a013987314054b2c2409e99c00384342b25ee7dba4c51cb1ea49a size: 1569
We can check if the push was successful:
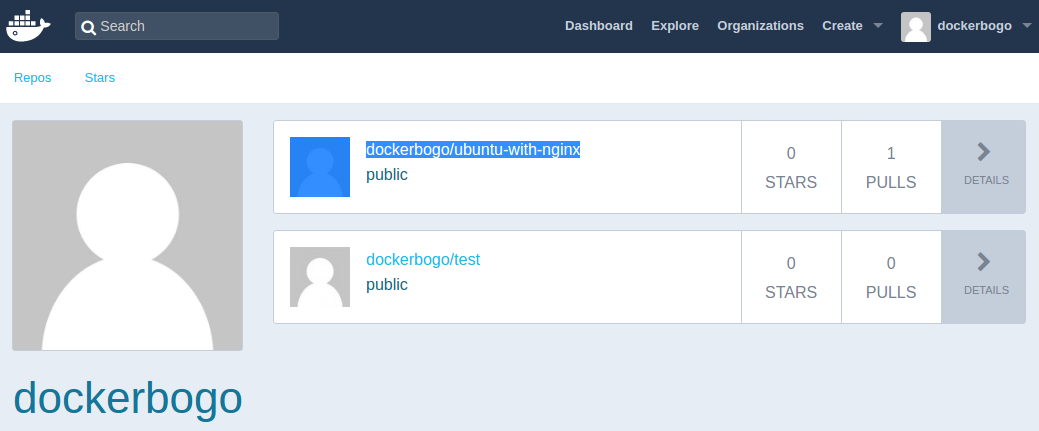
On Max, the containers are stored within the VM located at:
$ ls -la ~/Library/Containers/com.docker.docker/Data/vms/0 total 16215008 drwxr-xr-x@ 17 kihyuckhong staff 544 Dec 16 10:42 . drwxr-xr-x 3 kihyuckhong staff 96 Jul 31 15:03 .. srwxr-xr-x 1 kihyuckhong staff 0 Dec 16 10:41 00000002.000005f4 srwxr-xr-x 1 kihyuckhong staff 0 Dec 16 10:41 00000002.00001001 srwxr-xr-x 1 kihyuckhong staff 0 Dec 16 10:41 00000002.00001003 srwxr-xr-x 1 kihyuckhong staff 0 Dec 16 10:41 00000003.000005f5 srwxr-xr-x 1 kihyuckhong staff 0 Dec 16 10:41 00000003.00000948 -rw-r--r-- 1 kihyuckhong staff 8331264000 Dec 20 16:46 Docker.qcow2 -rw-r--r-- 1 kihyuckhong staff 90112 Dec 16 10:41 config.iso srwxr-xr-x 1 kihyuckhong staff 0 Dec 16 10:41 connect drwxr-xr-x 2 kihyuckhong staff 64 Aug 5 20:31 data lrwxr-xr-x 1 kihyuckhong staff 17 Dec 16 10:41 guest.000005f5 -> 00000003.000005f5 lrwxr-xr-x 1 kihyuckhong staff 17 Dec 16 10:41 guest.00000948 -> 00000003.00000948 -rw-r--r-- 1 kihyuckhong staff 2764 Dec 16 10:41 hyperkit.json -rw-r--r-- 1 kihyuckhong staff 3 Dec 16 10:41 hyperkit.pid drwxr-xr-x 2 kihyuckhong staff 64 Jul 31 15:03 log lrwxr-xr-x 1 kihyuckhong staff 12 Dec 16 10:41 tty -> /dev/ttys004
$ screen ~/Library/Containers/com.docker.docker/Data/vms/0/tty
The command will show a blank window, just hit "Enter", then we get:
docker-desktop:~# docker-desktop:~# cd /var/lib/docker docker-desktop:/var/lib/docker# ls -la total 124 drwx--x--x 15 root root 4096 Dec 16 18:42 . drwxr-xr-x 10 root root 4096 Jul 31 22:03 .. drwx------ 2 root root 4096 Jul 31 22:03 builder drwx--x--x 4 root root 4096 Jul 31 22:03 buildkit drwx------ 3 root root 4096 Jul 31 22:03 containerd drwx------ 10 root root 4096 Dec 20 20:34 containers drwx------ 3 root root 4096 Jul 31 22:03 image drwxr-x--- 3 root root 4096 Jul 31 22:03 network drwx------ 80 root root 49152 Dec 20 20:34 overlay2 drwx------ 4 root root 4096 Jul 31 22:03 plugins drwx------ 2 root root 4096 Dec 16 18:42 runtimes drwx------ 2 root root 4096 Jul 31 22:03 swarm drwx------ 2 root root 4096 Dec 16 18:42 tmp drwx------ 2 root root 4096 Jul 31 22:03 trust drwx------ 14 root root 20480 Dec 15 18:43 volumes
Additional steps with a screen command:
# disconnect that session but leave it open in background Ctrl-a d # list that session that's still running in background $ screen -ls There is a screen on: 18887.ttys005.Kihyucks-MacBook-Air (Detached) 1 Socket in /var/folders/3s/42rgkz495lj4ydjgnqhxn_400000gn/T/.screen. # reconnect to that session (don't open a new one, that won't work and 2nd tty will give you garbled screen) $ screen -r 18887.ttys005.Kihyucks-MacBook-Air [screen is terminating] # kill this session (window) and exit Ctrl-a k
https://docs.docker.com/engine/reference/commandline/docker/
Docker & K8s
- Docker install on Amazon Linux AMI
- Docker install on EC2 Ubuntu 14.04
- Docker container vs Virtual Machine
- Docker install on Ubuntu 14.04
- Docker Hello World Application
- Nginx image - share/copy files, Dockerfile
- Working with Docker images : brief introduction
- Docker image and container via docker commands (search, pull, run, ps, restart, attach, and rm)
- More on docker run command (docker run -it, docker run --rm, etc.)
- Docker Networks - Bridge Driver Network
- Docker Persistent Storage
- File sharing between host and container (docker run -d -p -v)
- Linking containers and volume for datastore
- Dockerfile - Build Docker images automatically I - FROM, MAINTAINER, and build context
- Dockerfile - Build Docker images automatically II - revisiting FROM, MAINTAINER, build context, and caching
- Dockerfile - Build Docker images automatically III - RUN
- Dockerfile - Build Docker images automatically IV - CMD
- Dockerfile - Build Docker images automatically V - WORKDIR, ENV, ADD, and ENTRYPOINT
- Docker - Apache Tomcat
- Docker - NodeJS
- Docker - NodeJS with hostname
- Docker Compose - NodeJS with MongoDB
- Docker - Prometheus and Grafana with Docker-compose
- Docker - StatsD/Graphite/Grafana
- Docker - Deploying a Java EE JBoss/WildFly Application on AWS Elastic Beanstalk Using Docker Containers
- Docker : NodeJS with GCP Kubernetes Engine
- Docker : Jenkins Multibranch Pipeline with Jenkinsfile and Github
- Docker : Jenkins Master and Slave
- Docker - ELK : ElasticSearch, Logstash, and Kibana
- Docker - ELK 7.6 : Elasticsearch on Centos 7
- Docker - ELK 7.6 : Filebeat on Centos 7
- Docker - ELK 7.6 : Logstash on Centos 7
- Docker - ELK 7.6 : Kibana on Centos 7
- Docker - ELK 7.6 : Elastic Stack with Docker Compose
- Docker - Deploy Elastic Cloud on Kubernetes (ECK) via Elasticsearch operator on minikube
- Docker - Deploy Elastic Stack via Helm on minikube
- Docker Compose - A gentle introduction with WordPress
- Docker Compose - MySQL
- MEAN Stack app on Docker containers : micro services
- MEAN Stack app on Docker containers : micro services via docker-compose
- Docker Compose - Hashicorp's Vault and Consul Part A (install vault, unsealing, static secrets, and policies)
- Docker Compose - Hashicorp's Vault and Consul Part B (EaaS, dynamic secrets, leases, and revocation)
- Docker Compose - Hashicorp's Vault and Consul Part C (Consul)
- Docker Compose with two containers - Flask REST API service container and an Apache server container
- Docker compose : Nginx reverse proxy with multiple containers
- Docker & Kubernetes : Envoy - Getting started
- Docker & Kubernetes : Envoy - Front Proxy
- Docker & Kubernetes : Ambassador - Envoy API Gateway on Kubernetes
- Docker Packer
- Docker Cheat Sheet
- Docker Q & A #1
- Kubernetes Q & A - Part I
- Kubernetes Q & A - Part II
- Docker - Run a React app in a docker
- Docker - Run a React app in a docker II (snapshot app with nginx)
- Docker - NodeJS and MySQL app with React in a docker
- Docker - Step by Step NodeJS and MySQL app with React - I
- Installing LAMP via puppet on Docker
- Docker install via Puppet
- Nginx Docker install via Ansible
- Apache Hadoop CDH 5.8 Install with QuickStarts Docker
- Docker - Deploying Flask app to ECS
- Docker Compose - Deploying WordPress to AWS
- Docker - WordPress Deploy to ECS with Docker-Compose (ECS-CLI EC2 type)
- Docker - WordPress Deploy to ECS with Docker-Compose (ECS-CLI Fargate type)
- Docker - ECS Fargate
- Docker - AWS ECS service discovery with Flask and Redis
- Docker & Kubernetes : minikube
- Docker & Kubernetes 2 : minikube Django with Postgres - persistent volume
- Docker & Kubernetes 3 : minikube Django with Redis and Celery
- Docker & Kubernetes 4 : Django with RDS via AWS Kops
- Docker & Kubernetes : Kops on AWS
- Docker & Kubernetes : Ingress controller on AWS with Kops
- Docker & Kubernetes : HashiCorp's Vault and Consul on minikube
- Docker & Kubernetes : HashiCorp's Vault and Consul - Auto-unseal using Transit Secrets Engine
- Docker & Kubernetes : Persistent Volumes & Persistent Volumes Claims - hostPath and annotations
- Docker & Kubernetes : Persistent Volumes - Dynamic volume provisioning
- Docker & Kubernetes : DaemonSet
- Docker & Kubernetes : Secrets
- Docker & Kubernetes : kubectl command
- Docker & Kubernetes : Assign a Kubernetes Pod to a particular node in a Kubernetes cluster
- Docker & Kubernetes : Configure a Pod to Use a ConfigMap
- AWS : EKS (Elastic Container Service for Kubernetes)
- Docker & Kubernetes : Run a React app in a minikube
- Docker & Kubernetes : Minikube install on AWS EC2
- Docker & Kubernetes : Cassandra with a StatefulSet
- Docker & Kubernetes : Terraform and AWS EKS
- Docker & Kubernetes : Pods and Service definitions
- Docker & Kubernetes : Service IP and the Service Type
- Docker & Kubernetes : Kubernetes DNS with Pods and Services
- Docker & Kubernetes : Headless service and discovering pods
- Docker & Kubernetes : Scaling and Updating application
- Docker & Kubernetes : Horizontal pod autoscaler on minikubes
- Docker & Kubernetes : From a monolithic app to micro services on GCP Kubernetes
- Docker & Kubernetes : Rolling updates
- Docker & Kubernetes : Deployments to GKE (Rolling update, Canary and Blue-green deployments)
- Docker & Kubernetes : Slack Chat Bot with NodeJS on GCP Kubernetes
- Docker & Kubernetes : Continuous Delivery with Jenkins Multibranch Pipeline for Dev, Canary, and Production Environments on GCP Kubernetes
- Docker & Kubernetes : NodePort vs LoadBalancer vs Ingress
- Docker & Kubernetes : MongoDB / MongoExpress on Minikube
- Docker & Kubernetes : Load Testing with Locust on GCP Kubernetes
- Docker & Kubernetes : MongoDB with StatefulSets on GCP Kubernetes Engine
- Docker & Kubernetes : Nginx Ingress Controller on Minikube
- Docker & Kubernetes : Setting up Ingress with NGINX Controller on Minikube (Mac)
- Docker & Kubernetes : Nginx Ingress Controller for Dashboard service on Minikube
- Docker & Kubernetes : Nginx Ingress Controller on GCP Kubernetes
- Docker & Kubernetes : Kubernetes Ingress with AWS ALB Ingress Controller in EKS
- Docker & Kubernetes : Setting up a private cluster on GCP Kubernetes
- Docker & Kubernetes : Kubernetes Namespaces (default, kube-public, kube-system) and switching namespaces (kubens)
- Docker & Kubernetes : StatefulSets on minikube
- Docker & Kubernetes : RBAC
- Docker & Kubernetes Service Account, RBAC, and IAM
- Docker & Kubernetes - Kubernetes Service Account, RBAC, IAM with EKS ALB, Part 1
- Docker & Kubernetes : Helm Chart
- Docker & Kubernetes : My first Helm deploy
- Docker & Kubernetes : Readiness and Liveness Probes
- Docker & Kubernetes : Helm chart repository with Github pages
- Docker & Kubernetes : Deploying WordPress and MariaDB with Ingress to Minikube using Helm Chart
- Docker & Kubernetes : Deploying WordPress and MariaDB to AWS using Helm 2 Chart
- Docker & Kubernetes : Deploying WordPress and MariaDB to AWS using Helm 3 Chart
- Docker & Kubernetes : Helm Chart for Node/Express and MySQL with Ingress
- Docker & Kubernetes : Deploy Prometheus and Grafana using Helm and Prometheus Operator - Monitoring Kubernetes node resources out of the box
- Docker & Kubernetes : Deploy Prometheus and Grafana using kube-prometheus-stack Helm Chart
- Docker & Kubernetes : Istio (service mesh) sidecar proxy on GCP Kubernetes
- Docker & Kubernetes : Istio on EKS
- Docker & Kubernetes : Istio on Minikube with AWS EC2 for Bookinfo Application
- Docker & Kubernetes : Deploying .NET Core app to Kubernetes Engine and configuring its traffic managed by Istio (Part I)
- Docker & Kubernetes : Deploying .NET Core app to Kubernetes Engine and configuring its traffic managed by Istio (Part II - Prometheus, Grafana, pin a service, split traffic, and inject faults)
- Docker & Kubernetes : Helm Package Manager with MySQL on GCP Kubernetes Engine
- Docker & Kubernetes : Deploying Memcached on Kubernetes Engine
- Docker & Kubernetes : EKS Control Plane (API server) Metrics with Prometheus
- Docker & Kubernetes : Spinnaker on EKS with Halyard
- Docker & Kubernetes : Continuous Delivery Pipelines with Spinnaker and Kubernetes Engine
- Docker & Kubernetes : Multi-node Local Kubernetes cluster : Kubeadm-dind (docker-in-docker)
- Docker & Kubernetes : Multi-node Local Kubernetes cluster : Kubeadm-kind (k8s-in-docker)
- Docker & Kubernetes : nodeSelector, nodeAffinity, taints/tolerations, pod affinity and anti-affinity - Assigning Pods to Nodes
- Docker & Kubernetes : Jenkins-X on EKS
- Docker & Kubernetes : ArgoCD App of Apps with Heml on Kubernetes
- Docker & Kubernetes : ArgoCD on Kubernetes cluster
- Docker & Kubernetes : GitOps with ArgoCD for Continuous Delivery to Kubernetes clusters (minikube) - guestbook
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization