Terraform Tutorial - output variables
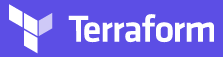
We can use output variables to organize data to be easily queried and shown back to the Terraform user.
While Terraform stores hundreds or thousands of attribute values for all our resources, we are more likely to be interested in a few values of importance, such as a load balancer IP, VPN address, etc.
Outputs are a way to tell Terraform what data is important. This data is outputted when apply
is called, and can be queried using the terraform output
command.
Ref - https://www.terraform.io/intro/getting-started/outputs.html
Let's define an output to show us the public IP address of the elastic IP address that we create. Add this to our ec2-instance.tf file:
We can peek the output via terraform console
:
$ terraform console > "${aws_instance.my-instance.*.public_ip}" [ "54.210.176.62", ] >
Here we uses '*' to get ips of all the instances. (note that we use count loop).
provider.tf:
provider "aws" { region = "${var.aws_region}" }
ec2-instance.tf:
resource "aws_key_pair" "terraform-demo" { key_name = "terraform-demo" public_key = "${file("terraform-demo.pub")}" } resource "aws_instance" "import_example" { ami = "${lookup(var.ami,var.aws_region)}" instance_type = "${var.instance_type}" key_name = "${aws_key_pair.terraform-demo.key_name}" } resource "aws_instance" "my-instance" { count = "${var.instance_count}" ami = "${lookup(var.ami,var.aws_region)}" instance_type = "${var.instance_type}" key_name = "${aws_key_pair.terraform-demo.key_name}" user_data = "${file("install_apache.sh")}" tags = { Name = "${element(var.instance_tags, count.index)}" Batch = "5AM" } } output "ip" { value = "${aws_instance.my-instance.*.public_ip}" }
vars.tf:
variable "ami" { type = "map" default = { "us-east-1" = "ami-04169656fea786776" "us-west-1" = "ami-006fce2a9625b177f" } } variable "instance_count" { default = "1" } variable "instance_tags" { type = "list" default = ["Terraform-1", "Terraform-2"] } variable "instance_type" { default = "t2.nano" } variable "aws_region" { default = "us-east-1" }
Run terraform apply
to populate the output. This only needs to be done once after the output is defined. The apply output should change slightly. At the end we should see this:
$ terraform apply ... Apply complete! Resources: 1 added, 0 changed, 1 destroyed. Outputs: ip = [ 54.210.176.62 ]
We can also query the outputs after apply-time using terraform output
:
$ terraform output ip 54.210.176.62
This command is useful for scripts to extract outputs.
Another example of using the terraform output
with a child module is available from
Terraform Tutorial - Modules which is using Terraform 12.
Terraform
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization