Docker & Kubernetes 1 : minikube A
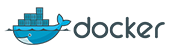
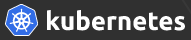
In this page, we'll use minikube to deploy apache's web, Flask app, and Django app.
Since we're already running a Docker daemon inside minikube, we should take advantage of this instead of relying on another VM so that we keep things simple.
To configure environment to use minikube’s Docker daemon, we just need to use docker-env:
$ eval $(minikube docker-env)

Let's setup a local registry, so Kubernetes can pull the image(s) from there:
$ docker run -d -p 5000:5000 --restart=always --name registry registry:2
A push or a pull accesses the Central Registry at index.docker.io. So, with the setup in the previous section, nothing has changed with the default behavior. But to push to or pull from our own registry, we just need to add the registry's location to the repository name. It will look like my.registry.address:port/repositoryname.
$ docker build -t <IMAGE_NAME>:<TAG> . $ docker build -t my-app:1.0.0 .
We should now have an image named 'my-app' locally, check by using docker images. We can then publish it to our local docker registry. To push an image to a private registry and not the central Docker registry we must tag it with the registry hostname and port (if needed):
$ docker tag my-app localhost:5000/my-app:1.0.0
Running docker images should now output the following:
$ docker images|grep my-app REPOSITORY TAG IMAGE ID CREATED SIZE my-app 1.0.0 2865bbbcfb95 3 hours ago 234MB localhost:5000/my-app 1.0.0 2865bbbcfb95 3 hours ago 234MB
$ kubectl apply -f deployment.yml
$ kubectl get deployment NAME DESIRED CURRENT UP-TO-DATE AVAILABLE AGE my-app 1 1 1 1 31s
To delete a deployment, the command is:
$ kubectl delete deployment/<DEPLOYMENT_NAME>
Each pod created via deployment has a unique IP address within the cluster. Minikube service allows us to access the pod IP address from outside of the cluster.
To create a service, the following shell command is to be executed:
$ kubectl apply -f service.yml
Though the IP address of the pod is transient and may change, the Service always routes traffic to the right pods.
To delete the service:
$ kubectl delete svc/<service-name>
We should now see our pod and our service:
$ kubectl get all
The minikube service <SERVICE_NAME> --url command will give us a url where we can access the service. In order to open the exposed service, we can use minikube service <SERVICE_NAME> command, and the service can be viewed on the browser via <minikube_ip>:<nodePort> url:
$ minikube service my-app --url http://192.168.99.100:31757

We may gather the info about the ip and port from:
$ minikube status minikube: Running cluster: Running kubectl: Correctly Configured: pointing to minikube-vm at 192.168.99.100 $ kubectl get svc NAME TYPE CLUSTER-IP EXTERNAL-IP PORT(S) AGE django-service NodePort 10.101.139.221 <none> 8000:31567/TCP 3m kubernetes ClusterIP 10.96.0.1 <none> 443/TCP 4h
Dockerfile:
FROM httpd:2.4-alpine COPY ./index.html /usr/local/apache2/htdocs/
index.html:
Hello world!
deployment.yml:
apiVersion: extensions/v1beta1 kind: Deployment metadata: labels: run: my-app name: my-app spec: replicas: 1 selector: matchLabels: run: my-app-exposed template: metadata: labels: run: my-app-exposed spec: containers: - image: localhost:5000/my-app:1.0.0 name: my-app ports: - containerPort: 80 protocol: TCP
service.yml:
apiVersion: v1 kind: Service metadata: labels: run: my-app name: my-app spec: ports: - port: 80 protocol: TCP targetPort: 80 selector: run: my-app-exposed type: NodePort
We can use Dockerhub repository instead of local repository:
apiVersion: extensions/v1beta1 kind: Deployment metadata: labels: run: my-app name: my-app spec: replicas: 1 selector: matchLabels: run: my-app-exposed template: metadata: labels: run: my-app-exposed spec: containers: - image: dockerbogo/my-app:1.0.0 name: my-app ports: - containerPort: 80 protocol: TCP
To push the image to the DockerHub repo, we may want to tag it first, and then logging in to dockerhub (username: dockerbogo):
$ docker build -t my-app:1.0.0 . $ docker tag my-app:1.0.0 dockerbogo/my-app:1.0.0 $ docker login -u dockerbogo $ docker push dockerbogo/my-app:1.0.0
$ eval $(minikube docker-env) $ docker build . -t flask-kubernetes-example:latest $ kubectl run flask-kubernetes-example --image=flask-kubernetes-example:latest --port=5000 --image-pull-policy=Never deployment.apps/flask-kubernetes-example created $ kubectl expose deployment flask-kubernetes-example --type=NodePort service/flask-kubernetes-example exposed $ minikube service flask-kubernetes-example --url http://192.168.99.100:32750

$ eval $(minikube docker-env) $ docker build -t dockerbogo/flask-hello-kubernetes:2.0.0 . $ docker push dockerbogo/flask-hello-kubernetes:2.0.0 $ kubectl apply -f deployment.yml deployment.extensions/flask-hello-kubernetes-deployment created $ kubectl get deployment NAME DESIRED CURRENT UP-TO-DATE AVAILABLE AGE flask-hello-kubernetes-deployment 1 1 1 1 9s $ kubectl apply -f service.yml service/flask-hello-kubernetes-service created $ kubectl get service NAME TYPE CLUSTER-IP EXTERNAL-IP PORT(S) AGE flask-hello-kubernetes-service LoadBalancer 10.103.44.203 <pending> 80:30148/TCP 1m $ minikube service flask-hello-kubernetes-service --url http://192.168.99.100:30148
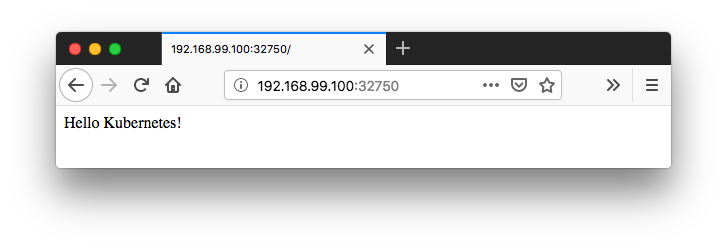
To delete the deployment and the service:
$ kubectl delete -f deployment.yml deployment.extensions "flask-hello-kubernetes-deployment" deleted $ kubectl delete -f service.yml service "flask-hello-kubernetes-service" deleted
The source files can be found here : Einsteinish/flask-hello-minikube
This app is a trimmed down version of https://cloud.google.com/python/django/kubernetes-engine dropped sql proxy and volumes.
$ docker build -t dockerbogo/django-polls:1.0.0 . $ docker push dockerbogo/django-polls:1.0.0 The push refers to repository [docker.io/dockerbogo/django-polls] $ kubectl apply -f polls.yml deployment.extensions/polls created service/polls created $ kubectl get deployment NAME DESIRED CURRENT UP-TO-DATE AVAILABLE AGE polls 3 3 3 3 10s $ kubectl get service NAME TYPE CLUSTER-IP EXTERNAL-IP PORT(S) AGE polls LoadBalancer 10.101.178.134 <pending> 80:32625/TCP 22s $ minikube service polls --url http://192.168.99.100:32625
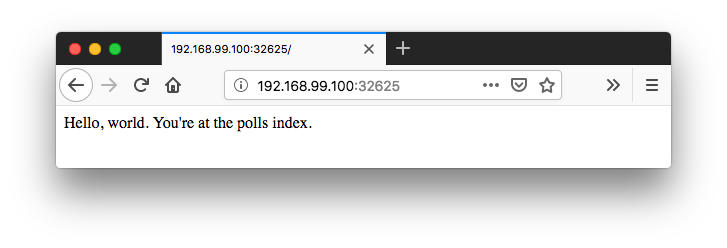
polls.yaml:
# [START kubernetes_deployment] apiVersion: extensions/v1beta1 kind: Deployment metadata: name: polls labels: app: polls spec: replicas: 3 template: metadata: labels: app: polls spec: containers: - name: polls-app # Replace with your project ID or use `make template` image: dockerbogo/django-polls:1.0.0 # This setting makes nodes pull the docker image every time before # starting the pod. This is useful when debugging, but should be turned # off in production. imagePullPolicy: Always ports: - containerPort: 8080 # [END kubernetes_deployment] --- # [START service] apiVersion: v1 kind: Service metadata: name: polls labels: app: polls spec: type: LoadBalancer ports: - port: 80 targetPort: 8080 selector: app: polls # [END service]
$ kubectl delete -f polls.yaml deployment.extensions "polls" deleted service "polls" deleted
To run the Django app on our local computer, set up a Python development environment, including Python, pip, and virtualenv.
Create an isolated Python environment, and install dependencies:
Run the Django migrations to set up your models:
Start a local web server:
In our browser, go to http://localhost:8000.
$ virtualenv venv $ source venv/bin/activate $ pip install -r requirements.txt
$ python manage.py makemigrations $ python manage.py makemigrations polls $ python manage.py migrate
$ python manage.py runserver
Docker & K8s
- Docker install on Amazon Linux AMI
- Docker install on EC2 Ubuntu 14.04
- Docker container vs Virtual Machine
- Docker install on Ubuntu 14.04
- Docker Hello World Application
- Nginx image - share/copy files, Dockerfile
- Working with Docker images : brief introduction
- Docker image and container via docker commands (search, pull, run, ps, restart, attach, and rm)
- More on docker run command (docker run -it, docker run --rm, etc.)
- Docker Networks - Bridge Driver Network
- Docker Persistent Storage
- File sharing between host and container (docker run -d -p -v)
- Linking containers and volume for datastore
- Dockerfile - Build Docker images automatically I - FROM, MAINTAINER, and build context
- Dockerfile - Build Docker images automatically II - revisiting FROM, MAINTAINER, build context, and caching
- Dockerfile - Build Docker images automatically III - RUN
- Dockerfile - Build Docker images automatically IV - CMD
- Dockerfile - Build Docker images automatically V - WORKDIR, ENV, ADD, and ENTRYPOINT
- Docker - Apache Tomcat
- Docker - NodeJS
- Docker - NodeJS with hostname
- Docker Compose - NodeJS with MongoDB
- Docker - Prometheus and Grafana with Docker-compose
- Docker - StatsD/Graphite/Grafana
- Docker - Deploying a Java EE JBoss/WildFly Application on AWS Elastic Beanstalk Using Docker Containers
- Docker : NodeJS with GCP Kubernetes Engine
- Docker : Jenkins Multibranch Pipeline with Jenkinsfile and Github
- Docker : Jenkins Master and Slave
- Docker - ELK : ElasticSearch, Logstash, and Kibana
- Docker - ELK 7.6 : Elasticsearch on Centos 7
- Docker - ELK 7.6 : Filebeat on Centos 7
- Docker - ELK 7.6 : Logstash on Centos 7
- Docker - ELK 7.6 : Kibana on Centos 7
- Docker - ELK 7.6 : Elastic Stack with Docker Compose
- Docker - Deploy Elastic Cloud on Kubernetes (ECK) via Elasticsearch operator on minikube
- Docker - Deploy Elastic Stack via Helm on minikube
- Docker Compose - A gentle introduction with WordPress
- Docker Compose - MySQL
- MEAN Stack app on Docker containers : micro services
- MEAN Stack app on Docker containers : micro services via docker-compose
- Docker Compose - Hashicorp's Vault and Consul Part A (install vault, unsealing, static secrets, and policies)
- Docker Compose - Hashicorp's Vault and Consul Part B (EaaS, dynamic secrets, leases, and revocation)
- Docker Compose - Hashicorp's Vault and Consul Part C (Consul)
- Docker Compose with two containers - Flask REST API service container and an Apache server container
- Docker compose : Nginx reverse proxy with multiple containers
- Docker & Kubernetes : Envoy - Getting started
- Docker & Kubernetes : Envoy - Front Proxy
- Docker & Kubernetes : Ambassador - Envoy API Gateway on Kubernetes
- Docker Packer
- Docker Cheat Sheet
- Docker Q & A #1
- Kubernetes Q & A - Part I
- Kubernetes Q & A - Part II
- Docker - Run a React app in a docker
- Docker - Run a React app in a docker II (snapshot app with nginx)
- Docker - NodeJS and MySQL app with React in a docker
- Docker - Step by Step NodeJS and MySQL app with React - I
- Installing LAMP via puppet on Docker
- Docker install via Puppet
- Nginx Docker install via Ansible
- Apache Hadoop CDH 5.8 Install with QuickStarts Docker
- Docker - Deploying Flask app to ECS
- Docker Compose - Deploying WordPress to AWS
- Docker - WordPress Deploy to ECS with Docker-Compose (ECS-CLI EC2 type)
- Docker - WordPress Deploy to ECS with Docker-Compose (ECS-CLI Fargate type)
- Docker - ECS Fargate
- Docker - AWS ECS service discovery with Flask and Redis
- Docker & Kubernetes : minikube
- Docker & Kubernetes 2 : minikube Django with Postgres - persistent volume
- Docker & Kubernetes 3 : minikube Django with Redis and Celery
- Docker & Kubernetes 4 : Django with RDS via AWS Kops
- Docker & Kubernetes : Kops on AWS
- Docker & Kubernetes : Ingress controller on AWS with Kops
- Docker & Kubernetes : HashiCorp's Vault and Consul on minikube
- Docker & Kubernetes : HashiCorp's Vault and Consul - Auto-unseal using Transit Secrets Engine
- Docker & Kubernetes : Persistent Volumes & Persistent Volumes Claims - hostPath and annotations
- Docker & Kubernetes : Persistent Volumes - Dynamic volume provisioning
- Docker & Kubernetes : DaemonSet
- Docker & Kubernetes : Secrets
- Docker & Kubernetes : kubectl command
- Docker & Kubernetes : Assign a Kubernetes Pod to a particular node in a Kubernetes cluster
- Docker & Kubernetes : Configure a Pod to Use a ConfigMap
- AWS : EKS (Elastic Container Service for Kubernetes)
- Docker & Kubernetes : Run a React app in a minikube
- Docker & Kubernetes : Minikube install on AWS EC2
- Docker & Kubernetes : Cassandra with a StatefulSet
- Docker & Kubernetes : Terraform and AWS EKS
- Docker & Kubernetes : Pods and Service definitions
- Docker & Kubernetes : Service IP and the Service Type
- Docker & Kubernetes : Kubernetes DNS with Pods and Services
- Docker & Kubernetes : Headless service and discovering pods
- Docker & Kubernetes : Scaling and Updating application
- Docker & Kubernetes : Horizontal pod autoscaler on minikubes
- Docker & Kubernetes : From a monolithic app to micro services on GCP Kubernetes
- Docker & Kubernetes : Rolling updates
- Docker & Kubernetes : Deployments to GKE (Rolling update, Canary and Blue-green deployments)
- Docker & Kubernetes : Slack Chat Bot with NodeJS on GCP Kubernetes
- Docker & Kubernetes : Continuous Delivery with Jenkins Multibranch Pipeline for Dev, Canary, and Production Environments on GCP Kubernetes
- Docker & Kubernetes : NodePort vs LoadBalancer vs Ingress
- Docker & Kubernetes : MongoDB / MongoExpress on Minikube
- Docker & Kubernetes : Load Testing with Locust on GCP Kubernetes
- Docker & Kubernetes : MongoDB with StatefulSets on GCP Kubernetes Engine
- Docker & Kubernetes : Nginx Ingress Controller on Minikube
- Docker & Kubernetes : Setting up Ingress with NGINX Controller on Minikube (Mac)
- Docker & Kubernetes : Nginx Ingress Controller for Dashboard service on Minikube
- Docker & Kubernetes : Nginx Ingress Controller on GCP Kubernetes
- Docker & Kubernetes : Kubernetes Ingress with AWS ALB Ingress Controller in EKS
- Docker & Kubernetes : Setting up a private cluster on GCP Kubernetes
- Docker & Kubernetes : Kubernetes Namespaces (default, kube-public, kube-system) and switching namespaces (kubens)
- Docker & Kubernetes : StatefulSets on minikube
- Docker & Kubernetes : RBAC
- Docker & Kubernetes Service Account, RBAC, and IAM
- Docker & Kubernetes - Kubernetes Service Account, RBAC, IAM with EKS ALB, Part 1
- Docker & Kubernetes : Helm Chart
- Docker & Kubernetes : My first Helm deploy
- Docker & Kubernetes : Readiness and Liveness Probes
- Docker & Kubernetes : Helm chart repository with Github pages
- Docker & Kubernetes : Deploying WordPress and MariaDB with Ingress to Minikube using Helm Chart
- Docker & Kubernetes : Deploying WordPress and MariaDB to AWS using Helm 2 Chart
- Docker & Kubernetes : Deploying WordPress and MariaDB to AWS using Helm 3 Chart
- Docker & Kubernetes : Helm Chart for Node/Express and MySQL with Ingress
- Docker & Kubernetes : Deploy Prometheus and Grafana using Helm and Prometheus Operator - Monitoring Kubernetes node resources out of the box
- Docker & Kubernetes : Deploy Prometheus and Grafana using kube-prometheus-stack Helm Chart
- Docker & Kubernetes : Istio (service mesh) sidecar proxy on GCP Kubernetes
- Docker & Kubernetes : Istio on EKS
- Docker & Kubernetes : Istio on Minikube with AWS EC2 for Bookinfo Application
- Docker & Kubernetes : Deploying .NET Core app to Kubernetes Engine and configuring its traffic managed by Istio (Part I)
- Docker & Kubernetes : Deploying .NET Core app to Kubernetes Engine and configuring its traffic managed by Istio (Part II - Prometheus, Grafana, pin a service, split traffic, and inject faults)
- Docker & Kubernetes : Helm Package Manager with MySQL on GCP Kubernetes Engine
- Docker & Kubernetes : Deploying Memcached on Kubernetes Engine
- Docker & Kubernetes : EKS Control Plane (API server) Metrics with Prometheus
- Docker & Kubernetes : Spinnaker on EKS with Halyard
- Docker & Kubernetes : Continuous Delivery Pipelines with Spinnaker and Kubernetes Engine
- Docker & Kubernetes : Multi-node Local Kubernetes cluster : Kubeadm-dind (docker-in-docker)
- Docker & Kubernetes : Multi-node Local Kubernetes cluster : Kubeadm-kind (k8s-in-docker)
- Docker & Kubernetes : nodeSelector, nodeAffinity, taints/tolerations, pod affinity and anti-affinity - Assigning Pods to Nodes
- Docker & Kubernetes : Jenkins-X on EKS
- Docker & Kubernetes : ArgoCD App of Apps with Heml on Kubernetes
- Docker & Kubernetes : ArgoCD on Kubernetes cluster
- Docker & Kubernetes : GitOps with ArgoCD for Continuous Delivery to Kubernetes clusters (minikube) - guestbook
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization