A quick example for multi / nested views using UI-router
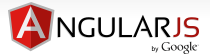
When I heard "states", I thought it's the "state" when we talk about "state/stateless". But it's not. I think it indicates the physical place or location in a page.
States | Routes |
---|---|
A place within our app | A url within our app |
Nested hierarchy | Flat hierarchy |
In this example, we'll have two views:
- Home view - this view has two nesting views, both of them will be placed at the same position. But only one view will be placed not both.
- About view has three views: column1, column2, and bottom-row. Unlike the Home view, every view has its own place. So, they can be displayed at the time. Also, we should note that they are name based.
This sample is heavily borrowed from AngularJS Routing Using UI-Router.
So, here is our default file: index.html:
<!-- index.html --> <!DOCTYPE html> <html> <head> <link href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css" rel="stylesheet"> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <script src="//cdnjs.cloudflare.com/ajax/libs/angular-ui-router/0.2.8/angular-ui-router.min.js"></script> <script src="app.js"></script> </head> <!-- apply our angular app to our site --> <body ng-app="routerApp"> <nav class="navbar navbar-default navbar-fixed-top"> <div class="navbar-header"> <a class="navbar-brand" ui-sref="#">ui-route</a> </div> <div class="navbar-collapse collapse"> <ul class="nav navbar-nav"> <li><a ui-sref="home">Home</a></li> <li><a ui-sref="about">About</a></li> </ul> </div> </nav> <div class="container"> <!-- we use ui-view instead of ng-view --> <div ui-view></div> </div> </body> </html>
This is the result of the codes in this tutorial.
I'm having some issues with iframe and navbar, so I could not spare proper space for this little toy. Please use scroll bar on the right side to see what's going on.
First, try the two buttons on home view, and then have a look at the About view by clicking the "About" button on the navbar.
Here is app.js:
// app.js var routerApp = angular.module('routerApp', ['ui.router']); routerApp.config(function($stateProvider, $urlRouterProvider) { $urlRouterProvider.otherwise('/home'); $stateProvider // nested views .state('home', { url: '/home', templateUrl: 'home.html' }) // nested list with custom controller .state('home.list', { url: '/list', templateUrl: 'list.html', controller: function($scope) { $scope.funnycitynames = ['Boring Oregon City', 'Emba rrass Minnesota', 'No Name South Dakota', 'Penistone South Yorkshire England', 'Intercourse Lancaster County, PA']; } }) // nested list with just some random string data .state('home.anything', { url: '/anything', template: 'What do you want from ui-route' }) // multiviews .state('about', { url: '/about', views: { // the main template will be placed here (relatively named) '': { templateUrl: 'about.html' }, // the child views (absolutely named) // for column #1, defines a separate controller 'column1@about': { templateUrl: 'column1.html', controller: 'column1Controller' }, // the child views (absolutely named) 'column2@about': { template: 'column #2!' }, // for bottom row, defines a separate controller shares with column #1 'bottom-row@about': { templateUrl: 'bottom.html', controller: 'column1Controller' } } }); }); // column1 controller that we call up in the about state routerApp.controller('column1Controller', function($scope) { $scope.message = 'column1'; $scope.galaxies = [ { name: 'Milkyway Galaxy', distance: '27,000' }, { name: 'Andromeda Galaxy', distance: '2,560,000' }, { name: 'Sagittarius Dwarf', distance: '3.400,000' } ]; }); // let's define the column1 controller that we call up in the about state routerApp.controller('bottom-rowController', function($scope) { $scope.message = 'bottom row'; $scope.variables = [ ]; });
Here is home.html:
<!-- home.html --> <div class="jumbotron text-center"> <h3>This is from home.html</h3> <p>nested views</p> <a ui-sref=".list" class="btn btn-primary">Funny City List</a> <a ui-sref=".anything" class="btn btn-danger">Anything</a> </div> <div ui-view></div>
Here is list.html:
<!-- list.html --> <ul> <li ng-repeat="cityname in funnycitynames">{{ cityname }}</li> </ul>
Now we're dealing with the 2nd top-menu: About:
about.html:
<!-- about.html --> <div class="jumbotron text-center"> <h3>About Page</h3> <p><span class="text-danger">Multiple</span> views and <span class="text-danger">Named</span> views!</p> </div> <div class="row"> <!-- col1 --> <div class="col-sm-6"> <div ui-view="column1"></div> </div> <!-- col2 --> <div class="col-sm-6"> <div ui-view="column2"></div> </div> <!-- bottom row --> <div class="col-sm-12 text-center"> <div ui-view="bottom-row"></div> </div> </div>
column1.html:
<!-- column1.html --> <style> table, th, td { border: 1px solid black; } th, td { padding: 10px; } </style> <h2>Nearest Galaxies (distance: Light years from the earth)</h2> <table> <tr> <th>Name</th> <th>Distance</th> </tr> <tr ng-repeat="galaxy in galaxies"> <td>{{galaxy.name}}</td> <td>{{galaxy.distance}}</td> </tr> </table>
bottom.html:
<!-- bottom.html --> <h3>Bottom row!</h3> <ul> <li ng-repeat="galaxy in galaxies | filter:name"> {{galaxy.name}} <img src="{{galaxy.name}}.png" alt="{{galaxy.name}}" height="50" width="50"> </li> </ul>
AngularJS
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization