AngularJS Framework : Routes II - separate url template files
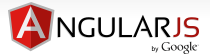
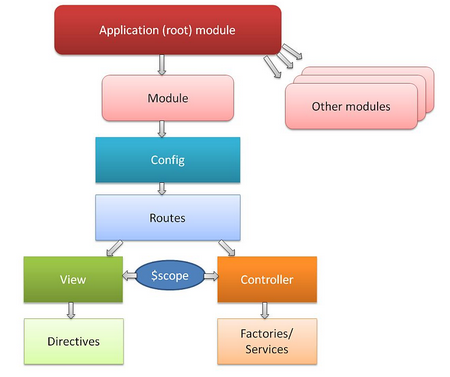
Picture from How to structure large angularJS applications
The following sections are largely based on Introduction to Angular.js in 50 Examples (part 2).
We'll start from where we left off (Routes I). In this section, we'll use template files for the routes. (country-list.html and country-detail.html). Here is the new code (route_separate_file.html)
In the previous section (Routes I - introduction), here is the snippet of the html file:
var countryApp = angular.module('countryApp', ['ngRoute']); countryApp.config(function($routeProvider) { $routeProvider. when('/', { template: '<ul><li ng-repeat="country in countries">{{country.name}}</li><ul>', controller: 'CountryListController' }). when('/:countryName', { template: '<h1>TODO create country detail view</h1>', controller: 'CountryDetailController' }). otherwise({ redirectTo: '/' }); }); ...
We modified template portions of the code and put them into separate html files:
<html ng-app="countryApp"> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular-route.min.js"></script> <script> var countryApp = angular.module('countryApp', ['ngRoute']); countryApp.config(function($routeProvider) { $routeProvider. when('/', { templateUrl: 'country-list.html', controller: 'CountryListController' }). when('/:countryName', { templateUrl: 'country-detail.html', controller: 'CountryDetailController' }). otherwise({ redirectTo: '/' }); }); countryApp.controller('CountryListController', function ($scope, $http){ $http.get('http://www.bogotobogo.com/AngularJS/files/Route2/countries.json').success(function(data) { $scope.countries = data; }); }); countryApp.controller('CountryDetailController', function ($scope, $routeParams){ console.log($routeParams); }); </script> </head> <body> <div ng-view></div> </body> </html>
It is rendered like this when we types in a country name after '#/':
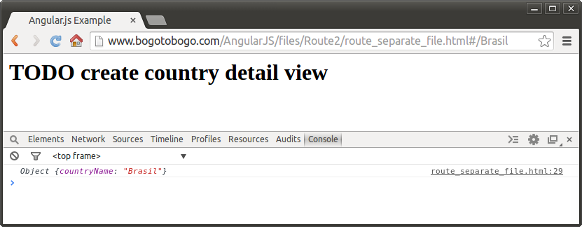
Note that we used the injected $http to get the data from a remote resource (see Dependency Injection).
Note that we only put the string into files:
when('/', { templateUrl: 'country-list.html', controller: 'CountryListController' }). when('/:countryName', { templateUrl: 'country-detail.html', controller: 'CountryDetailController' }).
Also, we switched from template to templateUrl.
The when() method takes a path and a route as parameters. The path is a part of the URL after the # symbol. The route contains two properties named templateUrl and controller. The templateUrl property tells which HTML template AngularJS should load and display inside the div with the ngView directive. The controller property tells which controllers should be used with the HTML template.
Continued at Routes III - extracting and using parameters from routes.
AngularJS
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization