A quick example using ng-view, ngRoute, routeProvider, and templateUrl
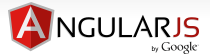
In this example, we'll have two views.
First, view index.html and with home.html inserted. Then, when we click "View galaxies", we get another view which listing all galaxies.
Both cases, the new view (when we switch them) will be placed within ng-view directive.
So, here is our default file: index.html:
<!DOCTYPE html> <html> <head lang="en"> <meta charset="utf-8"> <title>ngRoute / ng-view sample</title> </head> <body> <div ng-app="mainApp"> <h2>Welcome to Bogo Planetarium!</h2> <p><b>Header</b> <br /> This message will stay throughout all pages. <br /> Replaceable view will be within ng-view directive. <br /> ================== ng-view starts ==================</p> <br> <div ng-view></div> <br> <p>=============== ng-view ends ==================</p> <p><b>Footer</b></p> </div> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular.min.js"></script> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.4.8/angular-route.min.js"></script> <script type="text/javascript" src="main.js"></script> </body> </html>
As we can see from the index.html, we need javascript file main.js which defines controllers and routes:
var app = angular.module("mainApp", ['ngRoute']); app.config(['$routeProvider', function($routeProvider) { $routeProvider .when('/home', { templateUrl: 'home.html', controller: 'homeController' }) .when('/viewGalaxies', { templateUrl: 'viewGalaxies.html', controller: 'viewGalaxiesController' }) .otherwise({ redirectTo: '/home' }); }); app.controller('homeController', function($scope) { $scope.message = "$scope.message : from homeController"; }); app.controller('viewGalaxiesController', function($scope) { $scope.galaxies = [ {'name': 'Milkyway', 'distance':'27,000 Light years'}, {'name': 'Andromeda', 'distance':'2,560,000 Light years'}, {'name': 'Sagittarius Dwarf', 'distance':'3.400,000 Light years'} ]; $scope.add = function() { d = {} d['name'] = $scope.name; d['distance'] = $scope.distance; $scope.message = $scope.name + " " + $scope.distance $scope.galaxies.push(d); $scope.name = ""; $scope.distance = ""; $scope.message = $scope.galaxies; }; });
When we first run the app, the index.html requires home.html by the routes defined in main.js. So, here is our home.html:
<div class="container"> <h3> Home page (home.html) </h3> <p>{{message}}</p> <a href="#/viewGalaxies"> View Galaxies List</a> </div>
Finally, our 2nd view is needed when we request /viewGalaxies. The file is viewGalaxies.html and it looks like this:
<div class="container"> <h2> View Galaxies </h2> - viewGalaxies.html <ul> <li ng-repeat="galaxy in galaxies | filter:name">{{galaxy.name}} , {{galaxy.distance}} <br> <img src="{{galaxy.name}}.png" alt="{{galaxy.name}}" height="200" width="200"> <br> </li> </ul> <br /> <p>Add a new galaxy in the input box:</p> <form ng-submit="add()"> Name: <input type="text" ng-model="name" placeholder="Enter name here"> <br> Distance: <input type="text" ng-model="distance" placeholder="Enter distance here"> <br> A new galaxy: {{name}} {{distance}} <br> <input type="submit" value="Add"/> </form> <br /> <a href="#/home">Back to Home</a> </div>
AngularJS
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization