AngularJS Framework : ng-directives
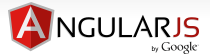
AngularJS lets us extend HTML with new attributes called Directives.
AngularJS directives attach special behavior to DOM elements. It's a marker. It makes our HTML interactive by attaching event listeners to the elements and/or transforming the DOM (sometimes a term 'compilation' is used for this process).
For example, ng-repeat repeats a specific element and ng-show conditionally shows an element. If we want to make an element draggable/droppable, we may want to create a directive for that as well.
AngularJS uses a directive to extend HTML.
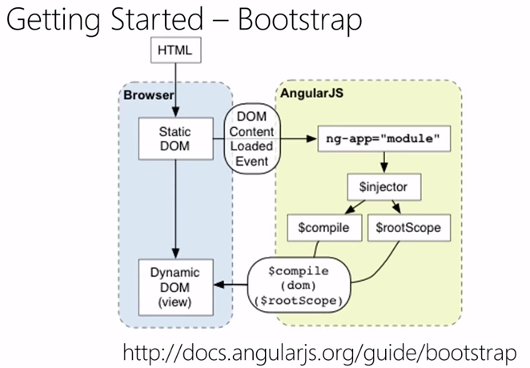
The ng-app directive defines an AngularJS application. We use this directive to auto-bootstrap an AngularJS application. The ng-app directive designates the root element of the application and is typically placed near the root element of the page - e.g. on the <body> or <html> tags.
Only one AngularJS application can be auto-bootstrapped per HTML document. The first ng-app found in the document will be used to define the root element to auto-bootstrap as an application.
AngularJS will initialize when the DOM content is loaded. If ng-app directive is found, that will become the root scope of the app.
The ng-model directive binds the value of HTML controls (input, select, textarea) to application data.
<html ng-app> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </head> <body> Name: <input ng-model="foo_name" type="text"/> <br /> <br /> Hello {{foo_name}}! </body> </html>
AngularJS starts automatically when the web page has loaded. The ng-app directive tells AngularJS that the <html> element is the "owner" of an AngularJS application.
The ng-model directive binds the value of the input field to the application variable name, foo_name, in the example above.The ng-app can be moved to <body> or we can create <div> elements and put the directive there, and tells AngularJS that the <div> element is the "owner" of the AngularJS application:
<html> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </head> <body> <div ng-app> Name: <input ng-model="foo_name" type="text"/> <br /> <br /> Hello {{foo_name}}! </div> </body> </html>
The {{foo_name}} expression, in the example above, is an AngularJS data binding expression. Data binding in AngularJS, synchronizes AngularJS expressions with AngularJS data. {{foo_name}} is synchronized with ng-model="foo_name".
<head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </head> <body> <div data-ng-app="" data-ng-init="quantity=1;price=49.99"> <h2>What's the cost?</h2> Quantity: <input type="number" ng-model="no_of_items"> Price: <input type="number" ng-model="price"> <p><b>Total in dollar:</b> {{no_of_items * price}}</p> </div> </body> </html>
ng-bind directive binds the innerHTML of the <span> element to the application variable name.
<html> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </head> <body> <div ng-app> Name: <input ng-model="foo_name" type="text"/> <br /> <br /> Hello <span ng-bind="foo_name"></span>! </div> </body> </html>
The ng-bind's attribute tells Angular to replace the text content of the specified HTML element with the value of a given expression, and to update the text content when the value of that expression changes.
The following lines do data binding with multiple text inputs:
<html> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </head> <body ng-app> First name: <input ng-model="firstName" type="text"/> <br /> Middle name: <input ng-model="middleName" type="text"/> <br /> Last name: <input ng-model="lastName" type="text"/> <br /><br /> Hello {{firstName}} {{middleName}} {{lastName}} ! </body> </html>
We can use data-ng-, instead of ng-, if we want to make our page HTML5 valid.
There is absolutely no difference between the two except that certain HTML5 validators will throw an error on a property like ng-app, but don't throw an error again anything prefixed with data-ng-app. So, we may want to use data-ng-app if we wish validating our HTML to be a bit easier.
AngularJS
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization