AngularJS Framework : Routes V - details page
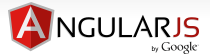
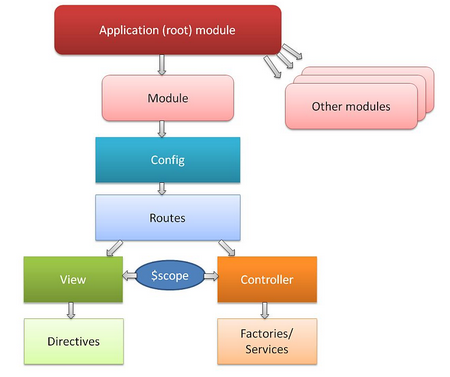
Picture from How to structure large angularJS applications
The following sections are largely based on Introduction to Angular.js in 50 Examples (part 2).
We'll start from where we left off (Routes IV - navigation between views using links). In this section, we'll be able to display more detail information about a specific country. Two of the template files are here (country-list.html and country-detail.html).
We need to change country-detail.html file, and it should look like this:
<h1> {{ country.name }} </h1> <ul> <li>Flag: <img ng-src="{{country.flagURL}}" width="100"></li> <li>Population: {{country.population | number }} </li> <li>Capital: {{country.capital}}</li> <li>GDP: {{country.gdp | currency }}</li> </ul>
Note that we used filters: to display comma, we filtered country.population with number and country.gdp with currency to put a $ sign.
Note that we used ng-src to make it easier parsing of the image upload url .
Here is our main code (route_country_detail.html):
<html ng-app="countryApp"> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular-route.min.js"></script> <script> var countryApp = angular.module('countryApp', ['ngRoute']); countryApp.config(function($routeProvider) { $routeProvider. when('/', { templateUrl: 'country-list.html', controller: 'CountryListController' }). when('/:countryName', { templateUrl: 'country-detail.html', controller: 'CountryDetailController' }). otherwise({ redirectTo: '/' }); }); countryApp.controller('CountryListController', function ($scope, $http){ $http.get('countries.json').success(function(data) { $scope.countries = data; }); }); countryApp.controller('CountryDetailController', function ($scope, $routeParams, $http){ $scope.name = $routeParams.countryName; $http.get('countries.json').success(function(data) { $scope.country = data.filter(function(entry){ return entry.name === $scope.name; })[0]; }); }); </script> </head> <body> <div ng-view></div> </body> </html>
Note that we added $http to the CountryDetailController to get json data:
countryApp.controller('CountryDetailController', function ($scope, $routeParams, $http){ $scope.name = $routeParams.countryName; $http.get('countries.json').success(function(data) { $scope.country = data.filter(function(entry){ return entry.name === $scope.name; })[0]; }); });
The root url just lists country names with links to appropriate country pages:
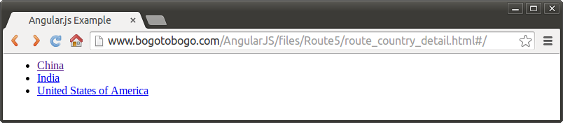
If we click 'USA', it will show detail information about USA:
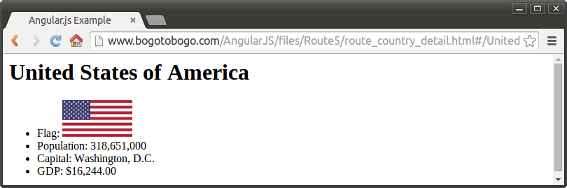
AngularJS
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization