React Starter Kit
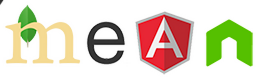
React is a Javascript library for building user interfaces.
Why React?
React is a JavaScript library for creating user interfaces by Facebook and Instagram. Many people choose to think of React as the V in MVC.
We built React to solve one problem: building large applications with data that changes over time. by Facebook and Instagram. Many people choose to think of React as the V in MVC.
We built React to solve one problem: building large applications with data that changes over time.
This page is based on react-starter-kit
To see how to install packages, see react-starter-kitGetting Started.
- Mac OS X, Windows, or Linux.
- Node.js v5.0 or newer.
- npm v3.3 or newer.
- node-gyp.
$ sudo npm install -g node-gyp
Here is the project structure:
. |-- /build/ # The folder for compiled output |-- /docs/ # Documentation files for the project |-- /node_modules/ # 3rd-party libraries and utilities |-- /src/ # The source code of the application | |--/components/ # React components | |-- /content/ # Static pages like About Us, Privacy Policy etc. | |-- /core/ # Core framework and utility functions | |-- /data/ # GraphQL server schema and data models | |-- /public/ # Static files which are copied into the /build/public folder | |-- /routes/ # Page/screen components along with the routing information | |-- /client.js # Client-side startup script | |-- /config.js # Global application settings | |-- /server.js # Server-side startup script |-- /test/ # Unit and end-to-end tests |-- /tools/ # Build automation scripts and utilities | |-- /lib/ # Library for utility snippets | |-- /build.js # Builds the project from source to output (build) folder | |-- /bundle.js # Bundles the web resources into package(s) through Webpack | |-- /clean.js # Cleans up the output (build) folder | |-- /copy.js # Copies static files to output (build) folder | |-- /deploy.js # Deploys your web application | |-- /run.js # Helper function for running build automation tasks | |-- /runServer.js # Launches (or restarts) Node.js server | |-- /start.js # Launches the development web server with "live reload" | |-- /webpack.config.js # Configurations for client-side and server-side bundles |-- package.json # The list of 3rd party libraries and utilities
Clone the latest version of React Starter Kit (pytato-react-starter-kit):
$ git clone -o react-starter-kit -b master --single-branch https://github.com/Einsteinish/pytato-react-starter-kit.git
We need to build a node-webkit specific version of sqlite3 from source rather than relying on the version direct from npm, otherwise we may get the following error:
node-webkit Error: please install sqlite3 package manually
So,
$ npm install sqlite3 --build-from-source --sqlite_libname=sqlcipher
With nvm, we can install multiple, self-contained versions of Node.js which will allow us to control our environment easier. It will give us on-demand access to the newest versions of Node.js, but will also allow us to target previous releases that our app may depend on.
We can pull down the nvm installation script via curl:
$ curl -sL https://raw.githubusercontent.com/creationix/nvm/v0.31.0/install.sh -o install_nvm.sh
Run the script with bash:
$ bash install_nvm.sh
It installs the software into ~/.nvm and add the necessary lines to ~/.profile file to use the file.
$ source ~/.profile
Now that nvm is installed, we can install isolated Node.js versions. To find out the versions of Node.js that are available for installation, we can type:
$ nvm ls-remote ... v5.12.0 v6.0.0 v6.1.0 v6.2.0 v6.2.1 v6.2.2 v6.3.0 v6.3.1 v6.4.0 v6.5.0
We can install the newest version of node (v6.5.0):
$ nvm install 6.5.0
We can specify which version of nvm to use by typing:
$ nvm use 6.5.0
We can check the version currently being used by the shell by typing:
$ which node /home/k/.nvm/versions/node/v6.5.0/bin/node $ node -v v6.5.0
Install both run-time project dependencies and developer tools listed in package.json file:
$ npm install
This command will build the app from the source files (/src) into the output /build folder. As soon as the initial build completes, it will start the Node.js server (node build/server.js).
$ npm start
To change port we can modify /src/config.js:
export const port = process.env.PORT || 3003;
If we need just to build the app (without running a dev server), we can simply run:
$ npm run build
or, for a production build:
$ npm run build -- --release
After running this command, the /build folder will contain the compiled version of the app.
For example, we can launch Node.js server normally by running node build/server.js.
For production, we may want to use pm2:
$ pm2 start build/server.js
The following configurations will forward request on port 80 to 3003.
nginx:
server { listen 80; server_name pytato.com; location / { proxy_pass http://localhost:3003; proxy_http_version 1.1; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection 'upgrade'; proxy_set_header Host $host; proxy_cache_bypass $http_upgrade; } }
Apache:
<VirtualHost *:80> ServerName www.pytato.com ServerAlias pytato.com ProxyRequests off <Proxy *> Order deny,allow Allow from all </Proxy> <Location /> ProxyPass http://localhost:3003/ ProxyPassReverse http://localhost:3003/ </Location> </VirtualHost>
Here is the site that's running Node and React with Apache proxy server:
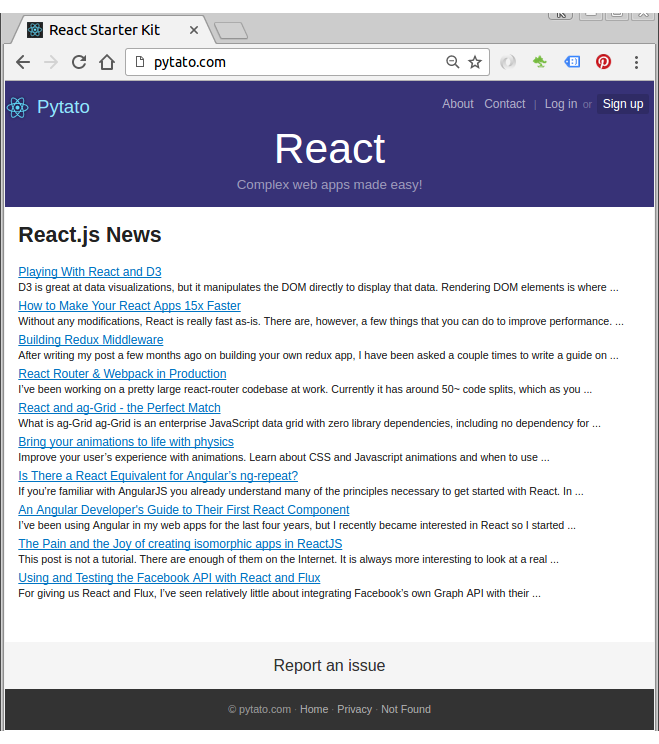
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization