AngularJS Framework : Controllers
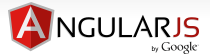
AngularJS controllers are nothing but plain JavaScript objects which are bound to a particular scope.
Angular.js controllers are code that controls certain sections containing DOM elements in which they are declared. They encapsulate the behavior via callbacks and glue $scope models with views.
Controllers are used to add logic to our views which are HTML pages. These pages simply show the data that we bind to them using two-way data binding in Angular.
Basically it is Controller's responsibility to glue the Model (data) with the View.
Here are descriptions about controllers from https://docs.angularjs.org/guide/controller
When a Controller is attached to the DOM via the ng-controller directive, Angular will instantiate a new Controller object, using the specified Controller's constructor function. A new child scope will be available as an injectable parameter to the Controller's constructor function as $scope.
Use controllers to:
- Set up the initial state of the $scope object.
- Add behavior to the $scope object.
The following sample shows the case of initializing the model using an Angular controller, defined with a global function:
<html ng-app> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <script> function nameController($scope){ $scope.firstName = 'Claude'; $scope.lastName = 'Debussy'; } </script> </head> <body ng-controller="nameController"> First name:<input ng-model="firstName" type="text"/> <br /> Last name:<input ng-model="lastName" type="text"/> <br /><br /> Hello {{firstName}} {{lastName}}! </body> </html>
The AngularJS application is defined by ng-app. The application runs inside a <div>.
The line:
<body ng-controller="nameController">
tells Angular to use "nameController" as a controller for the <body></body> portion of the code. The "nameController" function is a standard JavaScript object constructor.
AngularJS will invoke "nameController" with a $scope object.
In AngularJS, $scope is the application object (the owner of application variables and functions). The controller initializes the data, "firstName" and "lastName" to "Claude" and "Debussy".
The ng-model directives bind the input fields to the controller properties (firstName and lastName).
Note that we may put the ng-controller into other element such as "div".
However, setting controller as global is not a recommended way. So, in general, we define our controller as shown in the following section.
A controller can have methods:
<html> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <script> function nameController($scope){ $scope.firstName = 'Claude'; $scope.lastName = 'Debussy'; $scope.fullName = function() { return $scope.firstName + " " + $scope.lastName; } } </script> </head> <body> <div ng-app="" ng-controller="nameController"> First name: <input ng-model="firstName" type="text"/> <br /> Last name: <input ng-model="lastName" type="text"/> <br /><br /> Full name = {{fullName()}} </div> </body> </html>
<html> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <script src="nameController.js"></script> </head> <body> <div ng-app="" ng-controller="nameController"> First name: <input ng-model="firstName" type="text"/> <br /> Last name: <input ng-model="lastName" type="text"/> <br /><br /> Hello {{fullName()}}! </div> </body> </html>
With nameController.js:
function nameController($scope){ $scope.firstName = 'Claude'; $scope.lastName = 'Debussy'; $scope.fullName = function() { return $scope.firstName + " " + $scope.lastName; } }
<html ng-app="nameApp"> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <script> var nameApp = angular.module('nameApp', []); nameApp.controller('nameController', function ($scope){ $scope.firstName = 'Claude'; $scope.lastName = 'Debussy'; }); </script> </head> <body ng-controller="nameController"> First name:<input ng-model="firstName" type="text"/> <br /> Last name:<input ng-model="lastName" type="text"/> <br /><br /> Hello {{firstName}} {{lastName}}! </body> </html>
This is the Angular way of defining controller: rather than defining using a global function, we used API, nameApp.controller():
<script> var nameApp = angular.module('nameApp', []); nameApp.controller('nameController', function ($scope){ $scope.firstName = 'Claude'; $scope.lastName = 'Debussy'; }); </script>
Note that we do not have any dependency listed:
var nameApp = angular.module('nameApp', []);
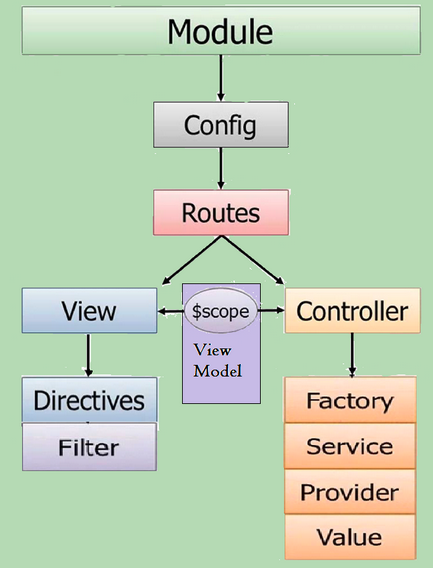
Picture from Modular Design of AngularJS Application
Note also the $scope object which is given as an argument to the anonymous function works as a glue between a controller and a view"
nameApp.controller('nameController', function ($scope){
AngularJS
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization