AngularJS Framework : Expressions
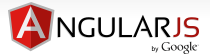
AngularJS expression is written inside double braces: {{ expression }}. It binds data to HTML the same way as the ng-bind directive. It is much like JavaScript expressions: They can contain literals, operators, and variables.
{{ 1 + 2 }}or
{{ first + " " + second }}
<!DOCTYPE html> <html> <body> <div ng-app=""> <p>AngularJS expression: {{ 1 + 2 }}</p> </div> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </body> </html>
The output looks like this:
If we remove the ng-app directive, HTML will display the expression as it is, without solving it:
<!DOCTYPE html> <html> <body> <div> <p>AngularJS expression: {{ 1 + 2 }}</p> </div> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </body> </html>
AngularJS numbers are similar to JavaScript numbers:
<!DOCTYPE html> <html> <body> <div ng-app="" ng-init="one=1;two=2"> <p>AngularJS numbers: {{ one / two }}</p> </div> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </body> </html>
We can use ng-bind:
<!DOCTYPE html> <html> <body> <div ng-app="" ng-init="one=1;two=2"> <p>AngularJS numbers: <span ng-bind="one / two"></span></p> </div> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </body> </html>
String works the same way as the numbers:
<!DOCTYPE html> <html> <body> <div ng-app="" ng-init="one='one';two='two'"> <p>AngularJS strings: <span ng-bind="one + ' ' + two"></span></p> </div> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </body> </html>
AngularJS objects are not different from JavaScript objects:
<!DOCTYPE html> <html> <body> <div ng-app="" ng-init="composers={firstName:'Claude',lastName:'Debussy'}"> <p>The name of the composer is {{ composers.firstName }} {{ composers.lastName }}</p> </div> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.jsjs"></script> </body> </html>
<!DOCTYPE html> <html> <body> <div ng-app="" ng-init="odds=[1,3,5,7]"> <p>The odd numbers in the array are {{ odds[0] }}, {{ odds[1] }}, {{ odds[2] }}, {{ odds[3] }}</p> </div> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </body> </html>
Another example of array:
<!DOCTYPE html> <html> <head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </head> <body> <div ng-app="" ng-init="capital_country=[ {capital:'Nassau',country:'Bahamas'}, {capital:'Lima',country:'Peru'}, {capital:'Kiev',country:'Ukraine'}]"> <ul> <li ng-repeat="x in capital_country"> {{ x.capital + ', ' + x.country }} </li> </ul> </div> </body> </html>
If we want to add or remove, we can do it with this:
<html ng-app> <head> <title>Hello scope and controller</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </head> <body> <div ng-controller="nameController"> Name: <input type="text" ng-model="newNames"/> <button ng-click="add()">Add</button> <button ng-click="remove()">Remove</button> <h2>Capitals</h2> <ul> <li ng-repeat="name in names"> {{ name }} </li> </ul> </div> <script> function nameController($scope) { $scope.names = ["Nassau", "Lima", "Kiev"]; $scope.add = function() { $scope.names.push($scope.newNames); $scope.newNames = ""; }; $scope.remove = function(name) { var n = $scope.names.indexOf(name); $scope.names.splice(n,1); }; } </script> </body> </html>
If we want to keep the dictionay key-value, we can do it with this code:
<html ng-app> <head> <title>Hello scope and controller</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </head> <body> <div ng-controller="nameController"> Capital: <input type="text" ng-model="newCapital"/> Country: <input type="text" ng-model="newCountry"/> <button ng-click="add()">Add</button> <button ng-click="remove()">Remove</button> <h2>Capitals</h2> <ul> <li ng-repeat="name in names"> {{ name.capital + ', ' + name.country }} </li> </ul> </div> <script> function nameController($scope) { $scope.names = [ {capital:"Nassau", country:"Bahamas"}, {capital:"Lima", country:"Peru"}, {capital:"Kiev",country:"Ukraine"} ]; $scope.add = function() { $scope.names.push({capital:$scope.newCapital, country:$scope.newCountry}); $scope.newNames = ""; }; $scope.remove = function(name) { var n = $scope.names.indexOf(name); $scope.names.splice(n,1); }; } </script> </body> </html>
AngularJS
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization