AngularJS Framework : Filters
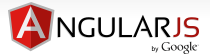
Filters can be added to expressions and directives using a pipe character.
AngularJS filters can be used to transform data:
Filter | Description |
---|---|
filter | Select a subset of items from an array. |
currency | Format a number to a currency format. |
lowercase | Format a string to lower case. |
uppercase | Format a string to upper case. |
orderBy | Orders an array by an expression. |
Uppercase & lowercase filters:
<!DOCTYPE html> <html> <head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <script src="nameController.js"></script> </head> <body> <div ng-app="" ng-controller="nameController"> <p>The name is {{ firstName | lowercase }} {{lastName | lowercase}}</p> <p>The name is {{ fullName() | uppercase }}</p> </div> </body> </html>
With nameController.js:
function nameController($scope){ $scope.firstName = 'Claude'; $scope.lastName = 'Debussy'; $scope.fullName = function() { return $scope.firstName + " " + $scope.lastName; } }
Currency filter:
The currency filter formats a number as currency:
<!DOCTYPE html> <html> <head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </head> <body> <div ng-app="" ng-controller="costController"> Number of Iterms: <input type="number" ng-model="numberOfIterms"> <br /> Price: <input type="number" ng-model="price"> <p>Total = {{ (numberOfIterms * price) | currency }}</p> </div> <script> function costController($scope) { $scope.numberOfIterms = 1; $scope.price = 99.99; } </script> </body> </html>
orderBy:
A filter can be added to a directive with a pipe character (|) and a filter. The orderBy filter orders an array by an expression:
<!DOCTYPE html> <html> <head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <script src="planetController.js"></script> </head> <body> <div ng-app="" ng-controller="planetController"> <p>Looping through planets:</p> <ul> <li ng-repeat="x in planets | orderBy:'distance'" > {{ x.name + ', ' + x.distance }} </li> </ul> </div> </body> </html>
With planetController.js:
function planetController($scope) { $scope.planets = [ {name:"Neptune",distance:30.087}, {name:"Uranus", distance:19.208}, {name:"Saturn", distance:9.523}, {name:"Jupiter", distance:5.203}, {name:"Mars", distance:1.524}, {name:"Earth", distance:1.0}, {name:"Venus", distance:0.723}, {name:"Mercury", distance:0.387} ]; }
Filtering Input:
An input filter can be added to a directive with a pipe character (|) and filter followed by a colon and a model name. The filter selects a subset of an array:
<html> <head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <script src="planetController.js"></script> </head> <body> <div ng-app="" ng-controller="planetController"> <p>Looping through planets:</p> <ul> <li ng-repeat="x in planets | filter:test | orderBy:'distance'" > {{ (x.name | uppercase) + ', ' + x.distance }} </li> </ul> </div> </body> </html>
With planetController.js:
function planetController($scope) { $scope.planets = [ {name:"Neptune",distance:30.087}, {name:"Uranus", distance:19.208}, {name:"Saturn", distance:9.523}, {name:"Jupiter", distance:5.203}, {name:"Mars", distance:1.524}, {name:"Earth", distance:1.0}, {name:"Venus", distance:0.723}, {name:"Mercury", distance:0.387} ]; }
To implement a filter, we'll start from the sample code used in the previous chapter with just two lines of midifications. Here is the code (angular_filter.html). We added search box, and it's using Angular filters:
<html ng-app="countryApp"> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <script> var countryApp = angular.module('countryApp', []); countryApp.controller('CountryController', ['$scope', '$http', function (scope, http){ http.get('http://www.bogotobogo.com/AngularJS/files/Dependency_Injection/countries.json').success(function(data) { scope.countries = data; }); }]); </script> </head> <body ng-controller="CountryController"> Search:<input ng-model="query" type="text"/> <table> <tr> <th>Country</th> <th>Population</th> </tr> <tr ng-repeat="country in countries | filter:query"> <td>{{country.name}}</td> <td align="right">{{country.population}}</td> </tr> </table> </body> </html>
As we may see, here are the lines changed from the code used in the previous chapter:
Search:<input ng-model="query" type="text"/> ... <tr ng-repeat="country in countries | filter:query">
We added query text box and added filter to ng-repeat.
We can put sorting filter in ng-repeat using orderBy, and it will display the countries with increasing population:
<tr ng-repeat="country in countries | filter:query | orderBy:'population'">
To sort the list in decreasing order, we just need '-' sign:
<tr ng-repeat="country in countries | filter:query | orderBy:'-population'">
This time, we want to sort the list by clicking columns (angular_filter_sort_by_columns.html):
<html ng-app="countryApp"> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <script> var countryApp = angular.module('countryApp', []); countryApp.controller('CountryController', ['$scope', '$http', function (scope, http){ http.get('http://www.bogotobogo.com/AngularJS/files/Dependency_Injection/countries.json').success(function(data) { scope.countries = data; }); scope.sortField = 'population'; }]); </script> </head> <body ng-controller="CountryController"> Search:<input ng-model="query" type="text"/> <table> <tr> <th><a href="" ng-click="sortField = 'name'">Country</a></th> <th><a href="" ng-click="sortField = 'population'">Population</a></th> </tr> <tr ng-repeat="country in countries | filter:query | orderBy:sortField"> <td>{{country.name}}</td> <td align="right">{{country.population}}</td> </tr> </table> </body> </html>
This time, we want to sort the list by clicking columns in reverse
(angular_filter_sort_by_columns_reverse.html):
<html ng-app="countryApp"> <head> <meta charset="utf-8"> <title>Angular.js Example</title> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <script> var countryApp = angular.module('countryApp', []); countryApp.controller('CountryController', ['$scope', '$http', function (scope, http){ http.get('http://www.bogotobogo.com/AngularJS/files/Dependency_Injection/countries.json').success(function(data) { scope.countries = data; }); scope.sortField = 'population'; scope.reverse = true; }]); </script> </head> <body ng-controller="CountryController"> Search:<input ng-model="query" type="text"/> <table> <tr> <th><a href="" ng-click="sortField ='name'; reverse = !reverse">Country</a></th> <th><a href="" ng-click="sortField = 'population'; reverse = !reverse">Population</a></th> </tr> <tr ng-repeat="country in countries | filter:query | orderBy:sortField:reverse"> <td>{{country.name}}</td> <td align="right">{{country.population}}</td> </tr> </table> </body> </html>
AngularJS
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization