AngularJS Framework : Module
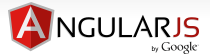
Angular uses modules to organize application code.
A module can depend on other modules:
var app = angular.module("myApp", ['ngRoute']]);
The first argument is the name of the module and the second argument is a list of module dependencies.
If we do not have any dependencies, an empty array should be provided.
Omitting an array has a behavior similar to getter: we're not creating a new module, but retrieving an existing one.
For example, when we need to reference a module in order to create a new controllers, services or directives we will simply use angular.module('myApp') without the second argument.
Again, modules are used to keep different logics separate so that each module works like a micro-services with its own potential models, controllers, services, directives, filters, etc. AngularJS modular approach enables us to keep the code clean.
A module is created by using the AngularJS function angular.module:
<div ng-app="myApp"> ... </div> <script> var app = angular.module("myApp", []); </script>
The myApp parameter refers to an HTML element in which the application will run.
We define modules in separate js files and name them as per the module.js file.
Modules define applications.
All application Controllers should belong to a Module. Modules make our application more readable, and keep the global namespace clean.
A Module can have a Config function and it could be used to define different routes. The routes are really important in SPA because if we have different views, and those views need to be loaded into the shell page, then we need a way to build a track what router on, and what view is associated with, what controller goes with that view.
How we do all those different pieces combine them together?
We need to find a route in AngularJS. We can define two things on that route to have the key things: one of the two is view and the view needs controller.
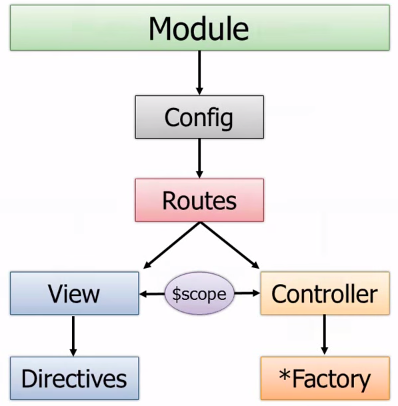
Picture source: AngularJS Fundamentals In 60-ish Minutes
Given controller and with the access to the scope object which the view will bind to. The controllers rather than having all the functionality to get the data and update data and perform CRUD operations, in the actual application, they will call out to Factories.
AngularJS has a set of built-in directives which we can use to add functionality to our application.
<!DOCTYPE html> <html> <head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.2.0/angular.min.js"></script> </head> <body> <div ng-app="" ng-controller="myCtrl"> {{ firstName + " " + lastName }} </div> <script src="myCtrl.js"></script> </body> </html>
With myCtrl.js:
function myCtrl($scope) { $scope.firstName = "Albert"; $scope.lastName = "Camus"; }
Note: for this sample, we used Angular 1.2.0: in the example, we're using function instead of app.controller.
Global values should be avoided in any applications because can easily be corrupted by other part of the code. AngularJS modules can solve, or at least reduce this problem.
The application code above does not have a name, and the controller function is global, which we may want to avoid, if possible.
The application has a name ng-app="myApp", and the controller is a property of the module:
<!DOCTYPE html> <html> <head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> </head> <body> <div ng-app="myApp" ng-controller="myCtrl"> {{ firstName + " " + lastName }} </div> <script> var app = angular.module("myApp", []); app.controller("myCtrl", function($scope) { $scope.firstName = "Albert"; $scope.lastName = "Camus"; }); </script> </body> </html>
We learned what modules are, and how they work.
Now it is time to build our application file.
Our application should have at least one module file, and one controller file for each controller.
The diagram in previous section is one of the ways of using modules but it's not a recommended way of using module. The diagram below is the way we should use a module as a container of different things:
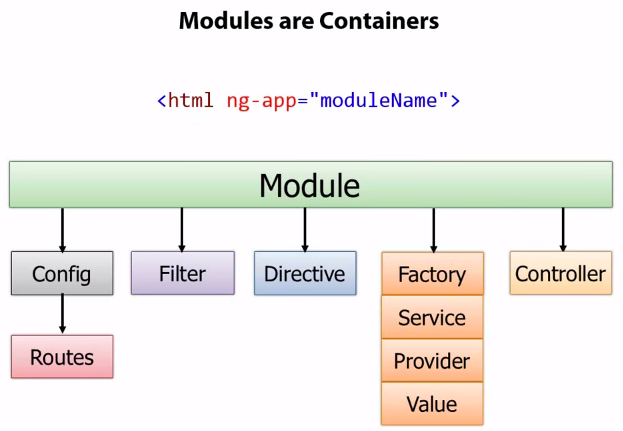
Picture source: AngularJS Fundamentals In 60-ish Minutes
- Module file, myApp.js:
var app = angular.module("myApp", []);
Note that the [] parameter in the module definition can be used to define dependent modules. This is where dependency injection comes in because our module might actually rely on other modules to get data. - Controller file(s), myCtrl.js:
app.controller("myCtrl", function($scope) { $scope.firstName = "Albert"; $scope.lastName = "Camus"; });
So, with those two files, our final AngularJS file should look like this:
<!DOCTYPE html> <html> <head> <script src="//ajax.googleapis.com/ajax/libs/angularjs/1.2.0/angular.min.js"></script> </head> <body> <div ng-app="myApp" ng-controller="myCtrl"> {{ firstName + " " + lastName }} </div> <script src="myApp.js"></script> <script src="myCtrl.js"></script> </body> </html>
This section is borrwoed from app.controller vs function in angular.js
When should we use app.controller("MyCtrl",...) and when should we use function MyCtrl($scope){...}?
app-vs-fnc.html:
<body ng-app="myApp"> <div ng-controller="FirstCtrl as app1"> <button class="btn" ng-model="app1.count" ng-click="app1.increment()"> Click to increment</button> {{ app1.count }} </div> <div ng-controller="SecondCtrl"> <button class="btn" ng-model="count" ng-click="increment()"> Click to increment</button> {{ count }} </div> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.0/angular.js"></script> <script type="text/javascript" src="example.js"></script> </body>
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization