AngularJS Framework : Twitter Bootstrap
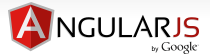
Bootstrap is the most popular HTML, CSS, and JS framework for developing responsive, mobile first projects on the web. Bootstrap makes front-end web development faster and easier. It's made for folks of all skill levels, devices of all shapes, and projects of all sizes.
Bootstrap ships with vanilla CSS, but its source code utilizes the two most popular CSS preprocessors, Less and Sass.
Quickly get started with precompiled CSS or build on the source. Bootstrap easily and efficiently scales our websites and applications with a single code base, from phones to tablets to desktops with CSS media queries.
With Bootstrap, we get extensive and beautiful documentation for common HTML elements, dozens of custom HTML and CSS components, and awesome jQuery plugins.
angular.module('myApp', []).controller('userCtrl', function($scope) { $scope.firstName = ''; $scope.lastName = ''; $scope.password1 = ''; $scope.password2 = ''; $scope.users = [ {id:1, firstName:'Claude', lastName:"Debussy" }, {id:2, firstName:'Maurice', lastName:"Ravel" }, {id:3, firstName:'Igor', lastName:"Stravinsky" }, {id:4, firstName:'Arnold', lastName:"Schoenberg" }, {id:5, firstName:'Nikolai', lastName:"Rimsky-Korsakov" } ]; $scope.edit = true; $scope.error = false; $scope.incomplete = false; $scope.editUser = function(id) { if (id == 'new') { $scope.edit = true; $scope.incomplete = true; $scope.firstName = ''; $scope.lastName = ''; } else { $scope.edit = false; $scope.firstName = $scope.users[id-1].firstName; $scope.lastName = $scope.users[id-1].lastName; } }; $scope.$watch('password1',function() {$scope.test();}); $scope.$watch('password2',function() {$scope.test();}); $scope.$watch('firstName', function() {$scope.test();}); $scope.$watch('lastName', function() {$scope.test();}); $scope.test = function() { if ($scope.password1 !== $scope.password2) { $scope.error = true; } else { $scope.error = false; } $scope.incomplete = false; if ($scope.edit && (!$scope.firstName.length || !$scope.lastName.length || !$scope.password1.length || !$scope.password2.length)) { $scope.incomplete = true; } }; });
<!DOCTYPE html> <html> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.4/css/bootstrap.min.css"> <script src= "//ajax.googleapis.com/ajax/libs/angularjs/1.3.15/angular.min.js"></script> <body ng-app="myApp" ng-controller="userCtrl"> <div class="container"> <h3>Users</h3> <table class="table table-striped"> <thead> <tr> <th>Edit</th> <th>First Name</th> <th>Last Name</th> </tr> </thead> <tbody> <tr ng-repeat="user in users"> <td> <button class="btn" ng-click="editUser(user.id)"> <span class="glyphicon glyphicon-pencil"></span> Edit </button> </td> <td>{{ user.firstName }}</td> <td>{{ user.lastName }}</td> </tr> </tbody> </table> <hr> <button class="btn btn-success" ng-click="editUser('new')"> <span class="glyphicon glyphicon-user"></span> Create New User </button> <hr> <h3 ng-show="edit">Create New User:</h3> <h3 ng-hide="edit">Edit User:</h3> <form class="form-horizontal"> <div class="form-group"> <label class="col control-label">First Name:</label> <div class="col"> <input type="text" ng-model="firstName" ng-disabled="edit" placeholder="First Name"> </div> </div> <div class="form-group"> <label class="col control-label">Last Name:</label> <div class="col"> <input type="text" ng-model="lastName" ng-disabled="edit" placeholder="Last Name"> </div> </div> <div class="form-group"> <label class="col control-label">Password:</label> <div class="col"> <input type="password" ng-model="password1" placeholder="Password"> </div> </div> <div class="form-group"> <label class="col control-label">Repeat:</label> <div class="col"> <input type="password" ng-model="password2" placeholder="Repeat Password"> </div> </div> </form> <hr> <button class="btn btn-success" ng-disabled="error || incomplete"> <span class="glyphicon glyphicon-save"></span> Save Changes </button> </div> <script src="users.js"></script> </body> </html>
AngularJS
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization