Android 4
3. Back to Hello World: Part B

This chapter
- 3.0 Back To Hello World Again
- 3.1 Eclipse IDE
- 3.2 Creating an AVD
- 3.3 Creating a New Android project
- 3.4 Constructing UI
- 3.5 Running the Application
- 3.6 Upgrade the UI to an XML Layout
- 3.7 R Class
- 3.8 Debugging a Project
- 3.9 Creating a Project without Eclipse
The "Hello, World" example we just completed uses what is called a "programmatic" UI layout.
This means that we constructed and built our application's UI directly in source code. If we've done much UI programming, we're probably familiar with how brittle that approach can sometimes be: small changes in layout can result in big source-code headaches. It's also very easy to forget to properly connect Views together, which can result in errors in our layout and wasted time debugging our code.
That's why Android provides an alternate UI construction model: XML-based layout files. The easiest way to explain this concept is to show an example. Here's an XML layout file that is identical in behavior to the programmatically-constructed example:
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/hello" /> </LinearLayout>
The general structure of an Android XML layout file is simple: it's a tree of XML elements, wherein each node is the name of a View class (this example, however, is just one View element). We can use the name of any class that extends View as an element in our XML layouts, including custom View classes we define in our own code. This structure makes it very easy to quickly build up UIs, using a more simple structure and syntax than we would use in a programmatic layout. This model is inspired by the web development model, wherein we can separate the presentation of our application (its UI) from the application logic used to fetch and fill in data.
In the above XML example, there's just one View element: the TextView, which has three XML attributes. Here's a summary of what they mean:
TABLE
Attribute | Meaning |
---|---|
xmlns:android |
This is an XML namespace declaration that tells the Android tools that we are going to refer to common attributes defined in the Android namespace. The outermost tag in every Android layout file must have this attribute. |
android:layout_width |
This attribute defines how much of the available width on the screen this View should consume.
In this case, it's the only View, so we want it to take up the entire screen, which is what a value of fill_parent means. |
android:layout_height | This is just like android:layout_width, except that it refers to available screen height. |
android:text | This sets the text that the TextView should display. In this example, we use a string resource instead of a hard-coded string value. The hello string is defined in the res/values/strings.xml file. This is the recommended practice for inserting strings to our application, because it makes the localization of our application to other languages graceful, without need to hard-code changes to the layout file. |
These XML layout files belong in the res/layout/ directory of our project. The "res" is short for "resources" and the directory contains all the non-code assets that our application requires. In addition to layout files, resources also include assets such as images, sounds, and localized strings.
The Eclipse plugin automatically creates one of these layout files for us: main.xml. In the "Hello World" application we just completed, this file was ignored and we created a layout programmatically. This was meant to teach us more about the Android framework, but we should almost always define our layout in an XML file instead of in our code. The following procedures will instruct us how to change our existing application to use an XML layout.
- In the Eclipse Package Explorer, expand the res/layout/ folder and open main.xml (once opened, we might need to click the "main.xml" tab at the bottom of the window to see the XML source). Replace the contents with the following XML:
<?xml version="1.0" encoding="utf-8"?> <TextView xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:text="@string/hello"/>
Save the file. - Inside the res/values/ folder, open strings.xml.
This is where we should save all default text strings for our user interface. If we're using Eclipse, then ADT will have started us with two strings, hello and app_name. Revise hello to something else. Perhaps "Hello, Android! I am a string resource!" The entire file should now look like this:
<?xml version="1.0" encoding="utf-8"?> <resources> <string name="hello">Hello, Android! I am a string resource!</string> <string name="app_name">Hello, Android</string> </resources>
- Now open and modify our HelloAndroid class use the XML layout. Edit the file to look like this:
package com.bogotobogo.android.first; import android.app.Activity; import android.os.Bundle; public class HelloAndroidActivity extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); } }
When we make this change, type it by hand to try the code-completion feature. As we begin typing "R.layout.main" the plugin will offer us suggestions. We'll find that it helps in a lot of situations.
Instead of passing setContentView() a View object, we give it a reference to the layout resource. The resource is identified as R.layout.main, which is actually a compiled object representation of the layout defined in /res/layout/main.xml. The Eclipse plugin automatically creates this reference for us inside the project's R.java class. If we're not using Eclipse, then the R.java class will be generated for us when we run Ant to build the application.
Now re-run our application, because we've created a launch configuration, all we need to do is click the green arrow icon to run, or select Run > Run History > Android Activity (HelloAndroid).
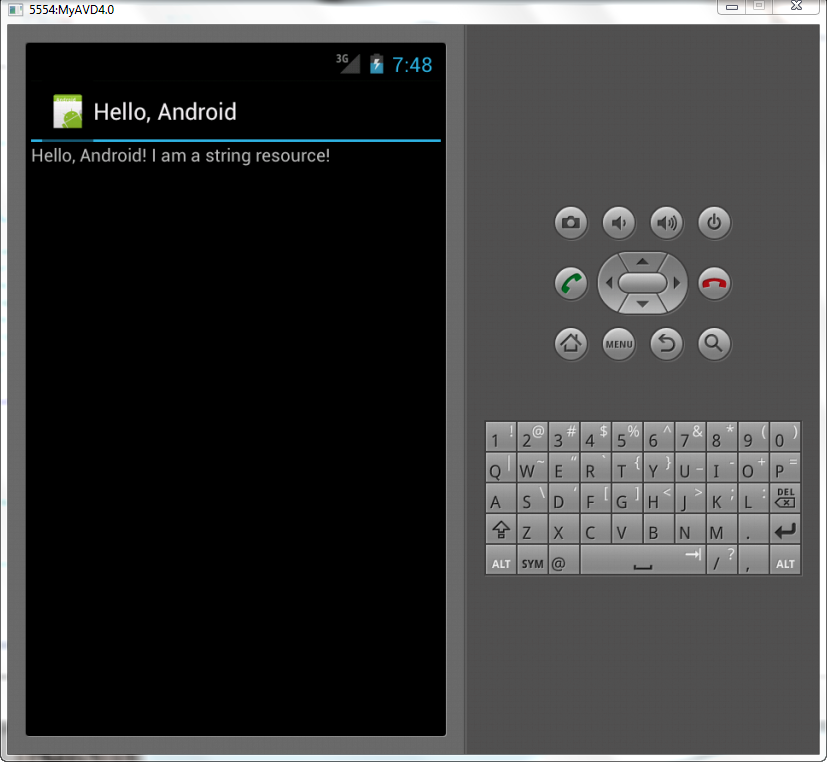
Other than the change to the TextView string, the application looks the same. After all, the point was to show that the two different layout approaches produce identical results.
So, defining our UI in XML and inflating it is the preferred way of implementing our user interfaces, as it neatly decouples our application logic from UI design.
To get access to our UI elements in code, we add identifier attributes to them in the XML definition. We can then use the findViewById() method to return a reference to each named item. The following XML snippet shows an ID attribute added to the TextView widget
android:id="@+id/myTextView"in our Hello Android templates.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" > <TextView android:id="@+id/myTextView" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="@string/hello" /> </LinearLayout>
With the modified helloandroid.java, we get the same result.
package com.bogotobogo.android.first; import android.app.Activity; import android.os.Bundle; import android.widget.TextView; public class HelloAndroidActivity extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); TextView tv = (TextView)findViewById(R.id.myTextView); setContentView(tv); } }
In Eclipse, open the file named R.java (in the gen/ [Generated Java Files] folder). It should look something like this:
/* AUTO-GENERATED FILE. DO NOT MODIFY. * * This class was automatically generated by the * aapt tool from the resource data it found. It * should not be modified by hand. */ package com.bogotobogo.android.first; public final class R { public static final class attr { } public static final class drawable { public static final int ic_launcher=0x7f020000; } public static final class id { public static final int myTextView=0x7f050000; } public static final class layout { public static final int main=0x7f030000; } public static final class string { public static final int app_name=0x7f040001; public static final int hello=0x7f040000; } }
A project's R.java file is an index into all the resources defined in the file. We use this class in our source code as a sort of short-hand way to refer to resources we've included in our project. This is particularly powerful with the code-completion features of IDEs like Eclipse because it lets us quickly and interactively locate the specific reference we're looking for.
Notice the inner class named layout, and its member field main. The Eclipse plugin noticed the XML layout file named main.xml and generated a class for it here. As we add other resources to our project (such as strings in the res/values/string.xml file or drawables inside the res/drawable/ direcory) we'll see R.java change to keep up.
When not using Eclipse, this class file will be generated for us at build time (with the Ant tool).
We should never edit this file by hand.
The Android Plugin for Eclipse also has excellent integration with the Eclipse debugger. To demonstrate this, introduce a bug into our code. Change our HelloAndroid source code to look like this:
package com.bogotobogo.android.first; import android.app.Activity; import android.os.Bundle; public class HelloAndroidActivity extends Activity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); Object o = null; o.toString(); setContentView(R.layout.main); } }
This change simply introduces a NullPointerException into our code. If we run our application again, we'll eventually see this:
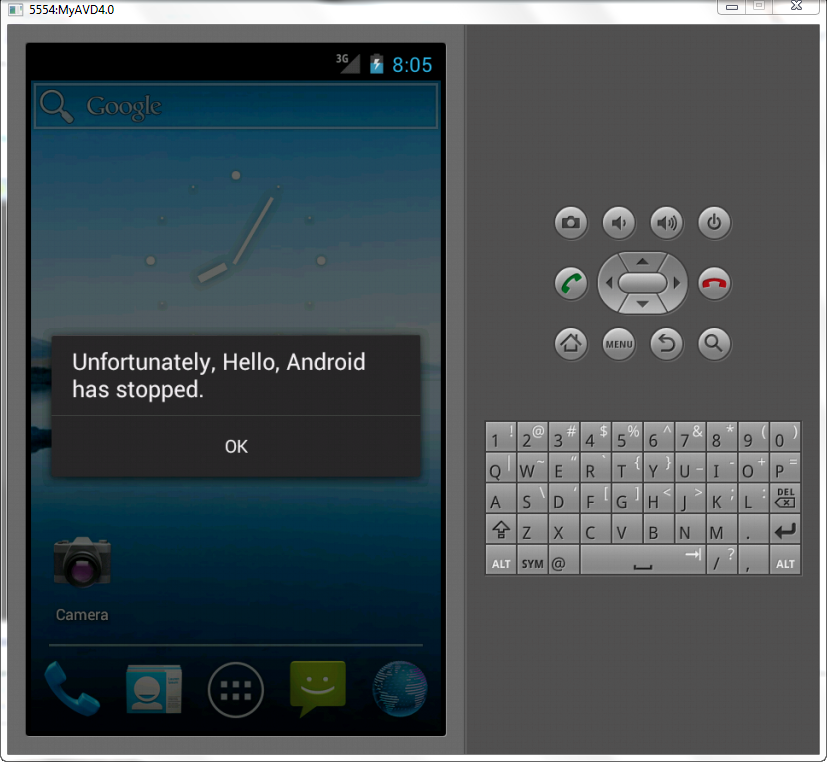
Press "Force Quit" to terminate the application and close the emulator window.
To find out more about the error, set a breakpoint in our source code on the line
Object o = null;
-double-click on the marker bar next to the source code line. Then select Run > Debug History > HelloAndroid from the menu to enter debug mode. Our app will restart in the emulator, but this time it will suspend when it reaches the breakpoint we set.
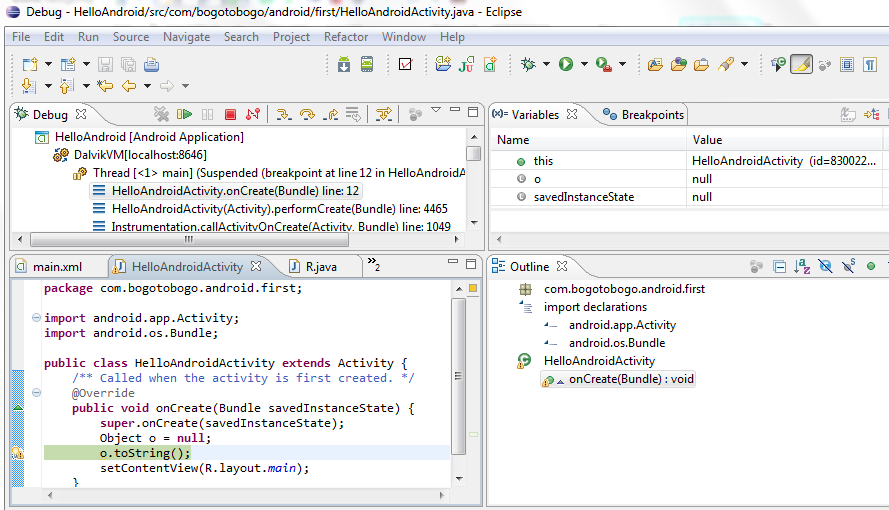
We can then step through the code in Eclipse's Debug Perspective, just as we would for any other application.
If we don't use Eclipse (such as if we prefer another IDE, or simply use text editors and command line tools) then the Eclipse plugin can't help ys. Don't worry though we don't lose any functionality just because we don't use Eclipse.
The Android Plugin for Eclipse is really just a wrapper around a set of tools included with the Android SDK. Thus, it's possible to wrap those tools with another tool, such as an 'ant' build file.
The Android SDK includes a tool named "android" that can be used to create all the source code and directory stubs for our project, as well as an ant-compatible build.xml file. This allows us to build our project from the command line, or integrate it with the IDE of our choice.
For example, to create a HelloAndroid project similar to the one created in Eclipse, use this command:
android create project \ --package com.bogotobogo.android.first \ --activity HelloAndroidActivity \ --target 9 \ --path <path-to-our-project>/HelloAndroid
This creates the required folders and files for the project at the location defined by the path.
Previous sections:
- 3.0 Back To Hello World Again
- 3.1 Eclipse IDE
- 3.2 Creating an AVD
- 3.3 Creating a New Android project
- 3.4 Constructing UI
- 3.5 Running the Application
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization