Design Patterns
- Delegation
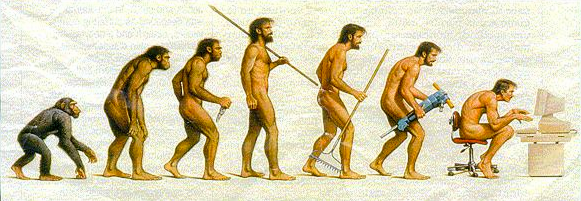
Delegation is used in several design patterns: state, strategy, visitor or http://www.bogotobogo.com/CSharp/csharp_delegates.php in C#.
Conceptually, a delegate is nothing more than a type-safe method reference. A delegate allows us to delegate the act of calling a method to somebody else.
A delegate also can be thought of as an object that contains an ordered list of methods with the same signature and return type.
Using delegation can make a design more flexible as shown in the example below. The RemoteHost does not have to refer to RemoteHostA or RemoteHostB in any way because the switching of delegation is abstracted from RemoteHost.
The RemoteHost class can delegate to RemoteHostA or RemoteHostB.
"Delegation is a good design choice only when it simplifies more than it complicates. It isn't easy to give rules that tell you exactly when to use delegation, because how effective it will be depends on the context and on how much experience you have with it. Delegation works best when it's used in highly stylized ways-that is, in standard patterns." - from Design Pattern - Elements of Resuable Object-Oriented Software by Eirch Gamma et al.
Here is an example code:
#include <iostream> using namespace std; class Host { public: virtual void f() = 0; }; class RemoteHostA : public Host { public: void f() { cout << "A::f()" << endl; } }; class RemoteHostB : public Host { public: void f() { cout << "B::f()" << endl; } }; // This class has a delegate switch to // either RemoteHostA or RemoteHostB class RemoteHost : public Host { public: explicit RemoteHost() { mHost = new RemoteHostA;} void f() { mHost->f(); } void connectA() { mHost = new RemoteHostA(); } void connectB() { mHost = new RemoteHostB(); } private: Host *mHost; }; int main() { RemoteHost *remote = new RemoteHost(); remote->f(); // A::f() remote->connectB(); remote->f(); // B::f() remote->connectA(); remote->f(); // A::f() return 0; }
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization