Design Patterns
- Facade Pattern
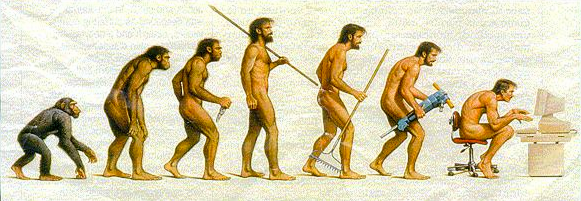
bogotobogo.com site search:
Facade Pattern
Intent
Provide a unified interface to a set of interfaces in a subsystem. Facade defines a higher-level interface that makes the subsystem easier to use.
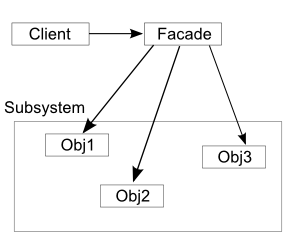
Façade pattern is different from adapter pattern because façade simplifies a class structure while adapter keeps the same class structure.
The Façade pattern is a way to structure our API into subsystems to reduce ever growing size and complexity of interfaces of API.
Here is an example code:
#include <iostream> // Subsystem 1 class SubSystemOne { public: void MethodOne(){ std::cout << "SubSystem 1" << std::endl; }; }; // Subsystem 2 class SubSystemTwo { public: void MethodTwo(){ std::cout << "SubSystem 2" << std::endl; }; }; // Subsystem 3 class SubSystemThree { public: void MethodThree(){ std::cout << "SubSystem 3" << std::endl; } }; // Facade class Facade { public: Facade() { pOne = new SubSystemOne(); pTwo = new SubSystemTwo(); pThree = new SubSystemThree(); } void MethodA() { std::cout << "Facade::MethodA" << std::endl; pOne->MethodOne(); pTwo->MethodTwo(); } void MethodB() { std::cout << "Facade::MethodB" << std::endl; pTwo->MethodTwo(); pThree->MethodThree(); } private: SubSystemOne *pOne; SubSystemTwo *pTwo; SubSystemThree *pThree; }; int main() { Facade *pFacade = new Facade(); pFacade->MethodA(); pFacade->MethodB(); return 0; }
Output is:
Facade::MethodA SubSystemOne: 1 SubSystem 2 Facade::MethodB SubSystem 2 SubSystem 3
Bogotobogo's contents
To see more items, click left or right arrow.
Bogotobogo Image / Video Processing
Computer Vision & Machine Learning
with OpenCV, MATLAB, FFmpeg, and scikit-learn.
Bogotobogo's Video Streaming Technology
with FFmpeg, HLS, MPEG-DASH, H.265 (HEVC)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization