Apache Maven 3 : Dependencies - 2020
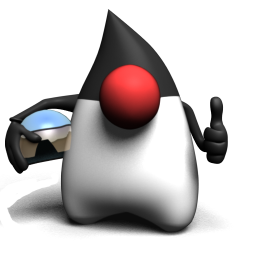
Continuing from Apache Maven 3 - Compile, build, and install a Maven project.
In this chapter, we'll add dependencies and let Maven know we have changed dependencies via pom.xml.
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/MavenTestApp$ ls pom.xml src target
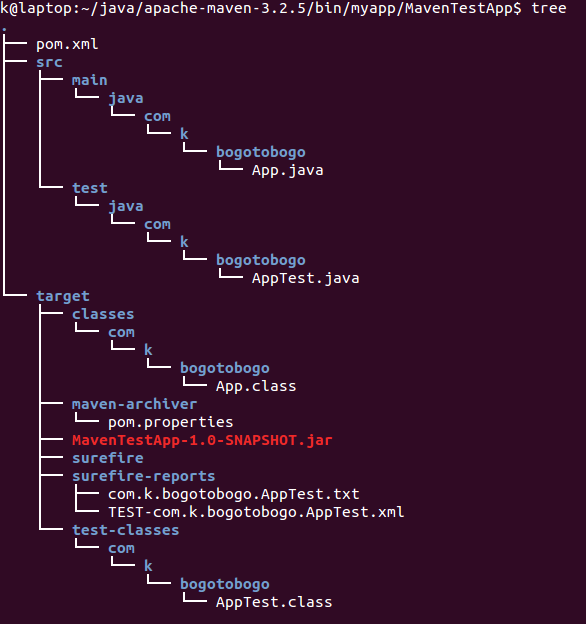
Since we've installed all the necessary components include jar files into local repository, we do not need target folder. So, we may want to delete it:
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/MavenTestApp$ mvn clean [INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building MavenTestApp 1.0-SNAPSHOT [INFO] ------------------------------------------------------------------------ [INFO] [INFO] --- maven-clean-plugin:2.5:clean (default-clean) @ MavenTestApp --- [INFO] Deleting /home/k/java/apache-maven-3.2.5/bin/myapp/MavenTestApp/target [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 1.739s [INFO] Finished at: Sun Feb 01 10:34:10 PST 2015 [INFO] Final Memory: 6M/97M [INFO] ------------------------------------------------------------------------
We can see the target folder has been deleted:
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/MavenTestApp$ ls pom.xml src
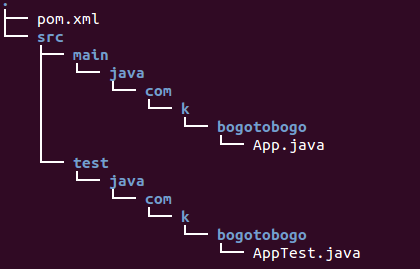
We have our source code,
/home/k/java/apache-maven-3.2.5/bin/myapp/MavenTestApp/src/main/java/com/k/bogotobogo/App.java
which looks like this:
package com.k.bogotobogo; /** * Hello world! * */ public class App { public static void main( String[] args ) { System.out.println( "Hello World!" ); } }
Now we want to print out the message to log instead of stdout, and the code modified like this:
package com.k.bogotobogo; import org.slf4j.*; /** * Hello world! * */ public class App { public static void main( String[] args ) { // System.out.println( "Hello World!" ); Logger logger = LoggerFactory.getLogger(App.class); logger.info("Hello World!"); } }
Note that we commented out the 'System.out' and using Logger. Also, we're importing a new package called org.slf4j. Here slf4j stands for Simple Logging Facade for Java.
Let's compile it:
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/MavenTestApp$ mvn compile ... [INFO] ------------------------------------------------------------------------ [INFO] BUILD FAILURE [INFO] ------------------------------------------------------------------------ ... error: package org.slf4j does not exist ...
What's missing?
We need to tell Maven to pull up the slf4j from the central repository. To do that we need to modify our pom.xml file which is in base directory of our project.
Here is our current pom.xml file:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.k.bogotobogo</groupId> <artifactId>MavenTestApp</artifactId> <version>1.0-SNAPSHOT</version> <packaging>jar</packaging> <name>MavenTestApp</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> </project>
We need to add the new dependency to the file.
Before we do that, we need information about the groupId, artifactId, and version for slf4j. We can find the info using a keyword like 'maven repository search', and search for 'slf4j':
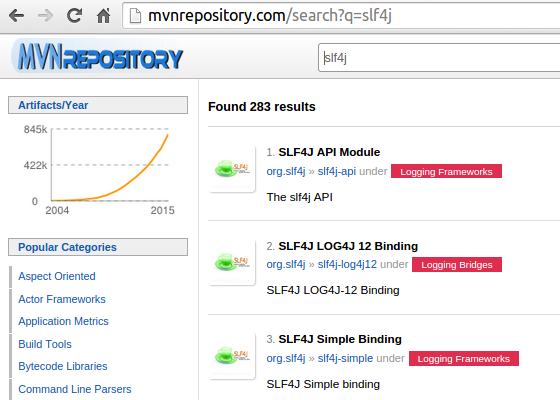
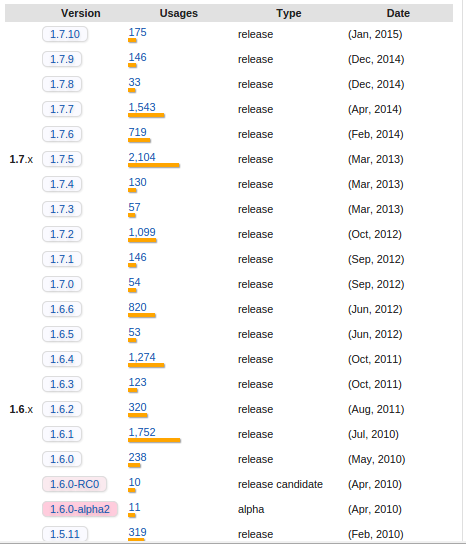
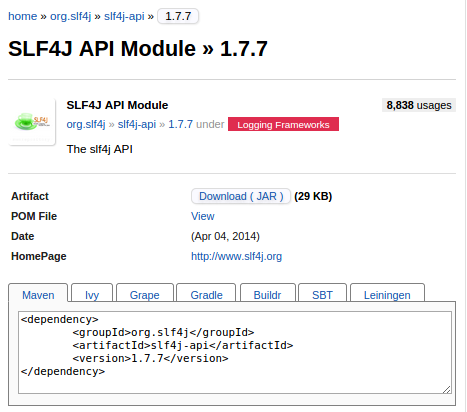
We can see it even provide what we should put into our pom.xml to tell Maven the dependency.
Here is our new version of pom.xml after adding the new dependency:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.k.bogotobogo</groupId> <artifactId>MavenTestApp</artifactId> <version>1.0-SNAPSHOT</version> <packaging>jar</packaging> <name>MavenTestApp</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>1.7.7</version> <scope>test</scope> </dependency> </dependencies> </project>
What Maven will do is to get download the slf4j jar before the compilation.
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/MavenTestApp$ mvn compile ... Downloading: http://repo.maven.apache.org/maven2/org/slf4j/slf4j-api/1.7.7/slf4j-api-1.7.7.jar Downloaded: http://repo.maven.apache.org/maven2/org/slf4j/slf4j-api/1.7.7/slf4j-api-1.7.7.jar (29 KB at 44.3 KB/sec) ... [INFO] --- maven-compiler-plugin:2.0.2:compile (default-compile) @ MavenTestApp --- [INFO] Compiling 1 source file to /home/k/java/apache-maven-3.2.5/bin/myapp/MavenTestApp/target/classes [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 4.870s [INFO] Finished at: Sun Feb 01 11:48:22 PST 2015 [INFO] Final Memory: 13M/97M [INFO] ------------------------------------------------------------------------
This time the compilation is successful.
Note that we did not put scope in our new dependency. In fact, we're using compile scope which is the default because we haven't specified any scope:
<scope>compile</scope>
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization