Spring-Boot / Spring Security with AngularJS : Part I - 2020
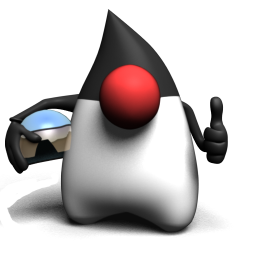
This post is based on Spring Security and Angular JS.
In this Part I, we'll put basis for our AngularJS SPA with Spring-boot and Spring Security. Then, in Part II, we'll move on to real AngularJS portion.
Though we can start from scratch, to head start the process, we may want to get the code as a .zip file from the Spring Boot Initializr.
We need to select dependencies "Web" and "Security", then click on "Generate Project". The set of files we get is not a complete set of codes but just a scaffold.
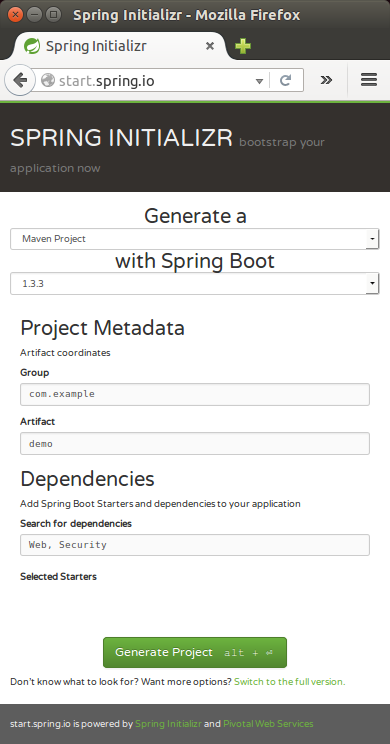
The .zip file contains a standard Maven or Gradle project in the root directory:
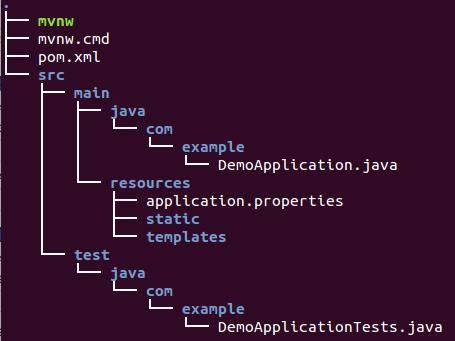
Keep in mind we're making SPA, so the index.html is the very core. We'll create in src/main/resources/static though we can create it in src/main/resources/public.
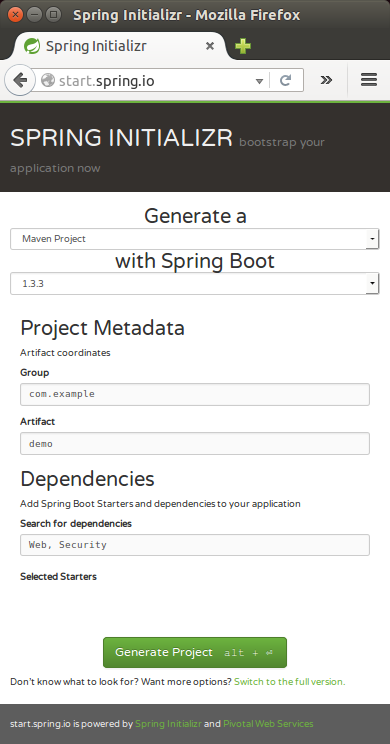
Here is the index.html:
<!doctype html> <html> <head> <title>Hello AngularJS</title> <link href="css/angular-bootstrap.css" rel="stylesheet"> <style type="text/css"> [ng\:cloak], [ng-cloak], .ng-cloak { display: none !important; } </style> </head> <body ng-app="hello"> <div class="container"> <h1>Greeting</h1> <div ng-controller="home" ng-cloak class="ng-cloak"> <p>The ID is {{greeting.id}}</p> <p>The content is {{greeting.content}}</p> </div> </div> <script src="js/angular-bootstrap.js" type="text/javascript"></script> <script src="js/hello.js"></script> </body> </html>
The app is not that interesting since it's just a "hello world" app. But it's worth the effort in that it's the first time we integrate AngularJS into Spring.
Before we run the app, let's make one more file, src/main/resources/application.yml. This is because when we load the home page we get a browser dialog asking for username and password. It looks like this:
security: user: password: password
Now that we're ready, let's run our simple app:
$ mvn spring-boot:run
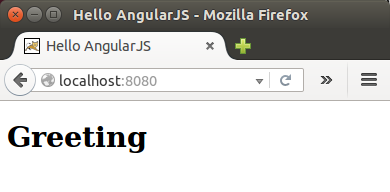
We may want to consolidate scripts such as angular-bootstrap.css. We are going to use wro4j, which is a Java-based toolchain for preprocessing and packaging front end assets. In other words, we are going to build static resource files and bundle them in our application JAR.
To create static resources at build time, we want to add some magic to the Maven pom.xml:
<build> <resources> <resource> <directory>${project.basedir}/src/main/resources</directory> </resource> <resource> <directory>${project.build.directory}/generated-resources</directory> </resource> </resources> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> <plugin> <artifactId>maven-resources-plugin</artifactId> <executions> <execution> <!-- Serves *only* to filter the wro.xml so it can get an absolute path for the project --> <id>copy-resources</id> <phase>validate</phase> <goals> <goal>copy-resources</goal> </goals> <configuration> <outputDirectory>${basedir}/target/wro</outputDirectory> <resources> <resource> <directory>src/main/wro</directory> <filtering>true</filtering> </resource> </resources> </configuration> </execution> </executions> </plugin> <plugin> <groupId>ro.isdc.wro4j</groupId> <artifactId>wro4j-maven-plugin</artifactId> <version>1.7.6</version> <executions> <execution> <phase>generate-resources</phase> <goals> <goal>run</goal> </goals> </execution> </executions> <configuration> <wroManagerFactory>ro.isdc.wro.maven.plugin.manager.factory.ConfigurableWroManagerFactory</wroManagerFactory> <cssDestinationFolder>${project.build.directory}/generated-resources/static/css</cssDestinationFolder> <jsDestinationFolder>${project.build.directory}/generated-resources/static/js</jsDestinationFolder> <wroFile>${project.build.directory}/wro/wro.xml</wroFile> <extraConfigFile>${basedir}/src/main/wro/wro.properties</extraConfigFile> <contextFolder>${basedir}/src/main/wro</contextFolder> </configuration> <dependencies> <dependency> <groupId>org.webjars</groupId> <artifactId>jquery</artifactId> <version>2.1.1</version> </dependency> <dependency> <groupId>org.webjars</groupId> <artifactId>angularjs</artifactId> <version>1.3.8</version> </dependency> <dependency> <groupId>org.webjars</groupId> <artifactId>bootstrap</artifactId> <version>3.2.0</version> </dependency> </dependencies> </plugin> </plugins> </build>
We need 3 files regarding the wro4j process. We can get it from
tut-spring-security-and-angular-js/basic/src/main/wro/.
- wro.properties is a configuration file for the preprocessing and rendering engine in wro4j.
- wro.xml declares a single "group" of resources called "angular-bootstrap", and this ends up being the base name of the static resources that are generated.
- main.less is empty in the sample code, but could be used to customise the look and feel. We could add a single line.
preProcessors=lessCssImport postProcessors=less4j,jsMin
<groups xmlns="http://www.isdc.ro/wro"> <group name="angular-bootstrap"> <css>webjar:bootstrap/3.2.0/less/bootstrap.less</css> <css>file:${project.basedir}/src/main/wro/main.less</css> <js>webjar:jquery/2.1.1/jquery.min.js</js> <js>webjar:angularjs/1.3.8/angular.min.js</js> </group> </groups>
@brand-primary: #de8579;
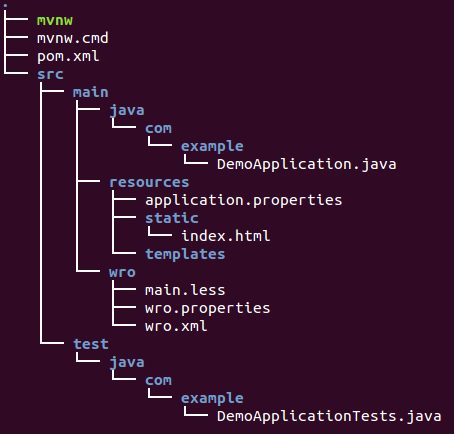
Let's run "mvn package":
$ mvn package
The generated resources will go in "target/generated-resources":
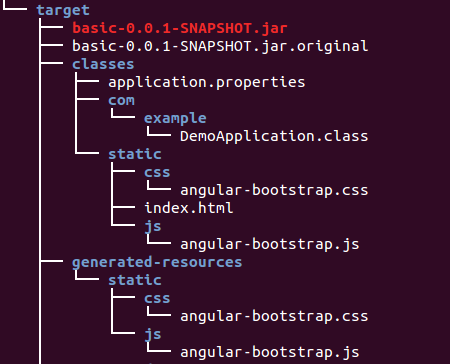
Because being declared in the <resources/> section, they will be packaged in the output JAR from the project. So, should also see the "bootstrap-angular.*" resources show up in our JAR file:
$ jar tf target/demo-0.0.1-SNAPSHOT.jar ... application.properties com/example/DemoApplication.class static/js/angular-bootstrap.js static/css/angular-bootstrap.css static/index.html META-INF/maven/ ...
Let's run the app now:
$ mvn spring-boot:run
We should see the CSS take effect while the business logic and navigation is still missing.
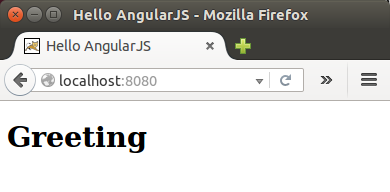
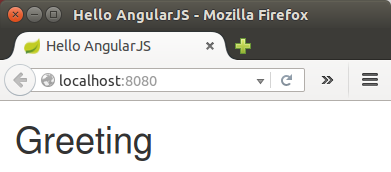
Hard to tell the difference since there not many stuff in the page. But we can see the text style has been changed from the one on the top to the bottom one.
Continued to Spring-Boot / Spring Security with AngularJS - Part II (Dynamic resource load from Angular)
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization