Apache Maven 3 : Plugins (compiler) - 2020
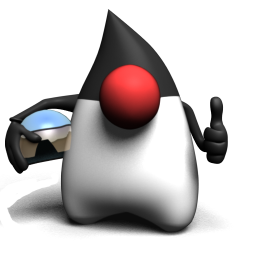
Continuing from Apache Maven 3 - Web Application.
In this chapter, we'll be focused on plugin features of Maven while we're handling plugins for compiler.
As we saw in the previous chapter, the compiler in Maven is actually a plugin. In the web application example, we set the behavior, especially, the configuration for the compiler:
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>2.0.2</version> <configuration> <source>1.4</source> <target>1.4</target> </configuration> </plugin> </plugins> </build>
The Compiler Plugin is used to compile the sources of our project. Since 3.0, the default compiler is javax.tools.JavaCompiler (if we are using java 1.6) and is used to compile Java sources. If we want to force the plugin using javac, we must configure the plugin option forceJavacCompilerUse.
Also note that at present the default source setting is 1.5 and the default target setting is 1.5, independently of the JDK we run Maven with.
In the example we used, the settings are 1.4 for both source and target because we chose to use J2EE 1.4 web application archetype.
In this section, we'll trigger compile error by using generics which in not supported 1.4 but works for 1.5. First, we added a new class in our src:
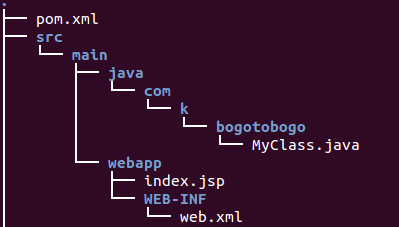
Our new class (MyCLass.java) looks like this:
package com.k.bogotobogo; import java.util.*; /** * Testing compiler * */ public class MyClass { public static void main( String[] args ) { List<String> stringList = new ArrayList<String>(); } }
When we compile it, it won't work:
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/mywebapp$ mvn compile [INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building mywebapp 1.0-SNAPSHOT [INFO] ------------------------------------------------------------------------ [INFO] [INFO] --- maven-resources-plugin:2.3:resources (default-resources) @ mywebapp --- [WARNING] Using platform encoding (UTF-8 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory /home/k/java/apache-maven-3.2.5/bin/myapp/mywebapp/src/main/resources [INFO] [INFO] --- maven-compiler-plugin:2.0.2:compile (default-compile) @ mywebapp --- [INFO] Compiling 1 source file to /home/k/java/apache-maven-3.2.5/bin/myapp/mywebapp/target/classes [INFO] ------------------------------------------------------------------------ [INFO] BUILD FAILURE [INFO] ------------------------------------------------------------------------ [INFO] Total time: 5.685s [INFO] Finished at: Sun Feb 01 16:32:22 PST 2015 [INFO] Final Memory: 7M/100M [INFO] ------------------------------------------------------------------------ [ERROR] Failed to execute goal org.apache.maven.plugins:maven-compiler-plugin:2.0.2:compile (default-compile) on project mywebapp: Compilation failure [ERROR] /home/k/java/apache-maven-3.2.5/bin/myapp/mywebapp/src/main/java/com/k/bogotobogo/MyClass.java:[13,12] error: generics are not supported in -source 1.4 [ERROR] -> [Help 1] [ERROR] [ERROR] To see the full stack trace of the errors, re-run Maven with the -e switch. [ERROR] Re-run Maven using the -X switch to enable full debug logging. [ERROR] [ERROR] For more information about the errors and possible solutions, please read the following articles: [ERROR] [Help 1] http://cwiki.apache.org/confluence/display/MAVEN/MojoFailureException
As we can see from the output, 1.4 compiler does not support generics, and we get an error:
[ERROR] /home/k/java/apache-maven-3.2.5/bin/myapp/mywebapp/src/main/java/com/k/bogotobogo/MyClass.java:[13,12] error: generics are not supported in -source 1.4
Let's switch 1.4 to 1.5 for the compiler configuration in our pom.xml:
<build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>2.0.2</version> <configuration> <source>1.5</source> <target>1.5</target> </configuration> </plugin> </plugins> </build>
Now, we compile it again:
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/mywebapp$ mvn compile [INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building mywebapp 1.0-SNAPSHOT [INFO] ------------------------------------------------------------------------ [INFO] [INFO] --- maven-resources-plugin:2.3:resources (default-resources) @ mywebapp --- [WARNING] Using platform encoding (UTF-8 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory /home/k/java/apache-maven-3.2.5/bin/myapp/mywebapp/src/main/resources [INFO] [INFO] --- maven-compiler-plugin:2.0.2:compile (default-compile) @ mywebapp --- [INFO] Compiling 1 source file to /home/k/java/apache-maven-3.2.5/bin/myapp/mywebapp/target/classes [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 5.297s [INFO] Finished at: Sun Feb 01 17:03:56 PST 2015 [INFO] Final Memory: 13M/97M [INFO] ------------------------------------------------------------------------
Now it's been compiled successfully.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization