Enums (Enumerations) - 2020
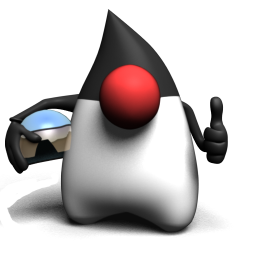
Though we can create our own constants by marking a variable static final, sometimes we want to create a set of constant values to represent the only valid values for a variable. This set of valid values is commonly known as enums.
Using enums can help reduce the bugs in our code. For instance, in our wine shop application we might want to restrict our size selections to SMALL, MEDIUM, and BIG. If we let an order for a LARGE or HUGE slip in, it might cause an error. With the following declaration, we can be sure that the compiler will stop us from assigning anything to a WineSize except SMALL, MEDIUM, and BIG:
enum WineSize {SMALL, MEDIUM, BIG};With that, the only way to get a WineSize should look like this:
WineSize wSize = WineSize.MEDIUM;
Enums can be declared as their own separate class, or as a class member and shouldn't be declared within a method.
Outside a class:
enum WineSize {SMALL, MEDIUM, BIG}; class Wine { WineSize size; } public class WineTest { public static void main (String[] args) { Wine w = new Wine(); w.size = WineSize.SMALL; System.out.println("Wine size = " + w.size); } }
With an output:
Wine size = SMALL
Here is the other example with an enum insde a class:
class Wine { enum WineSize {SMALL, MEDIUM, BIG}; WineSize size; } public class WineTest { public static void main (String[] args) { Wine w = new Wine(); w.size = Wine.WineSize.SMALL; System.out.println("Wine size = " + w.size); } }
As we can see from the two examples, enums can be declared as their own class or enclosed in another class. The syntax for accessing an enum's member depends on where the enum was declared.
But enums can't be declared with a method and the following example is illegal.
public class WineTest { public static void main (String[] args) { enum WineSize {SMALL, MEDIUM, BIG}; Wine w = new Wine(); w.size = WineSize.SMALL; System.out.println("Wine size = " + w.size); } }
Because an enum is a kind of class, we can do more than just listing the constant values. It can have constructors, instance variables, and methods.
Why do we need more in our enum?
It's useful in case we want to match the size and the weight of the wine as in the example below.
enum WineSize { SMALL(10), MEDIUM(20), BIG(30); WineSize(int ounces) { this.ounces = ounces; } public int getWeight() { return ounces; } private int ounces; } public class Wine{ WineSize size; public static void main (String[] args) { Wine ws = new Wine(); ws.size = WineSize.SMALL; Wine wm = new Wine(); wm.size = WineSize.MEDIUM; Wine wb = new Wine(); wb.size = WineSize.BIG; System.out.println(ws.size.getWeight()); System.out.println(wm.size.getWeight()); System.out.println(wb.size.getWeight()); for(WineSize wsize: WineSize.values()) System.out.println(wsize + " " + wsize.getWeight()); } }
With an output:
10 20 30 SMALL 10 MEDIUM 20 BIG 30
In the example above, we treat our enum values (SMALL, MEDIUM, BIG) as objects that can each has its own instance variables. Then we can assign those values at the time the enums are initialized, by passing a value to the enum constructor.
We can never invoke an enum constructor directly. The enum constructor is invoked automatically, with the arguments we define after the constant value. For instance, BIG(30) invokes the WineSize constructor that takes an int, passing the int literal 30 to the constructor.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization