Apache Maven 3 : Installing and creating a project - 2020
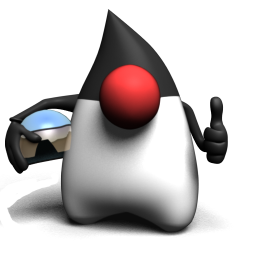
This will be very brief tutorial. We'll install Maven 3 plugin and then create a Maven Project on Ubuntu 14.04.
Let's download the Maven from Download Apache Maven 3.2.5:
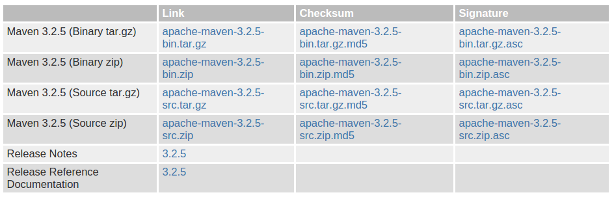
Here are the downloaded Maven files:
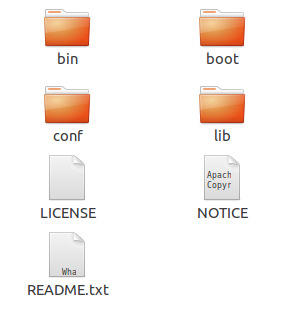
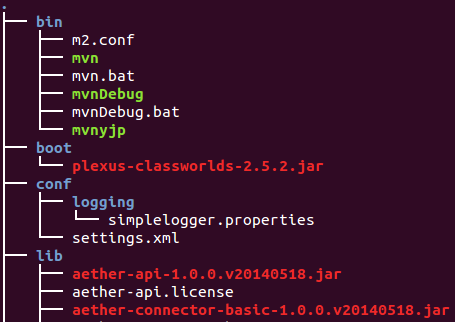
We need to set environment variables. First one is M2_HOME:
$ export M2_HOME=/home/k/java/apache-maven-3.2.5
Then, the path to bin so that we can execute the several commands in the bin folder:
$ export PATH=/home/k/java/apache-maven-3.2.5/bin:${PATH}
To check the version of Maven:
$ mvn --version Apache Maven 3.2.5 (12a6b3acb947671f09b81f49094c53f426d8cea1; 2014-12-14T09:29:23-08:00) Maven home: /home/k/java/apache-maven-3.2.5 Java version: 1.7.0_65, vendor: Oracle Corporation Java home: /usr/lib/jvm/java-7-openjdk-amd64/jre Default locale: en_US, platform encoding: UTF-8 OS name: "linux", version: "3.13.0-40-generic", arch: "amd64", family: "unix"
An archetype is a very simple artifact, that contains the project prototype we wish to create. To create a project, we'll use mvn archetype:generate command. In other words, we're creating a project from an existing template.
When we run the command, maven does following things (maven archetype:generate):
- Downloads maven-archetype-plugin's latest version.
- Lists all archetype's that can be used to create a project from. If we defined an archetype while calling the command, maven jumps to step 4.
- By default, maven chooses maven-archetype-quickstart archetype which basically creates a maven Hello World project with source and test classes. If we want to create a simple project, we can just press enter to continue. If we want to create a specific type of application, we should find the archetype matching our needs and enter the number of that archetype, then press enter.
- Since archetypes are templates and they intend to reflect current best practices, they can evolve in time, thus they have their own versions. Maven will ask us which version of the archetype we want to use. By default, maven chooses latest version for us. So, if we agree to use the latest version of an archetype, we just press Enter.
- Every maven project (and module) has its groupId, artifactId and version:
- groupId: Identifier of the organization or group that is responsible for this project. For exmple, org.hibernate, log4j, and org.springframework.boot.
- artifactId: Identifier of the artifact being generated by the project. This must be unique among the projects using the same groupId. For example, hibernate-tools, log4j, spring-core, and so on.
- version: Indicates the version number of the project. For example, 1.0.0, 2.3.1-SNAPSHOT, and 4.3.6.Final.
- Finally, maven will ask us the package structure for our code. A best practice is to create our folder structure that reflects the groupId, thus Maven sets this as default.
Now that we learned what is the mvn archetype, let's run the command:
First we may want to make a folder myapp:
$ mkdir myapp $ cd myapp
Then, issue the command.
$ mvn archetype:generate [INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building Maven Stub Project (No POM) 1 [INFO] ------------------------------------------------------------------------ [INFO] ... 1240: remote -> us.fatehi:schemacrawler-archetype-plugin-dbconnector (-) 1241: remote -> us.fatehi:schemacrawler-archetype-plugin-lint (-) Choose a number or apply filter (format: [groupId:]artifactId, case sensitive contains): 523: Choose org.apache.maven.archetypes:maven-archetype-quickstart version: 1: 1.0-alpha-1 2: 1.0-alpha-2 3: 1.0-alpha-3 4: 1.0-alpha-4 5: 1.0 6: 1.1 Choose a number: 6:
So, far we chose default and the latest version which is 1.1.
Then, it will ask couple of properties such as groupId, artifactId, and version:
Define value for property 'groupId': : com.k.bogotobogo Define value for property 'artifactId': : MavenTestApp version: 1.0-SNAPSHOT package: com.k.bogotobogo Y: : y [INFO] ---------------------------------------------------------------------------- [INFO] Using following parameters for creating project from Old (1.x) Archetype: maven-archetype-quickstart:1.1 [INFO] ---------------------------------------------------------------------------- [INFO] Parameter: groupId, Value: com.k.bogotobogo [INFO] Parameter: packageName, Value: com.k.bogotobogo [INFO] Parameter: package, Value: com.k.bogotobogo [INFO] Parameter: artifactId, Value: MavenTestApp [INFO] Parameter: basedir, Value: /home/k/java/apache-maven-3.2.5/bin/myapp [INFO] Parameter: version, Value: 1.0-SNAPSHOT [INFO] project created from Old (1.x) Archetype in dir: /home/k/java/apache-maven-3.2.5/bin/myapp/MavenTestApp [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 3:24.271s [INFO] Finished at: Sat Jan 31 20:40:16 PST 2015 [INFO] Final Memory: 18M/164M [INFO] ------------------------------------------------------------------------
Now that Maven's done, let's see what's in the newly created app directory:
k@laptop:~/java/apache-maven-3.2.5/bin/myapp$ ls MavenTestApp k@laptop:~/java/apache-maven-3.2.5/bin/myapp$ cd .. k@laptop:~/java/apache-maven-3.2.5/bin$ ls m2.conf mvn mvn.bat mvnDebug mvnDebug.bat mvnyjp myapp
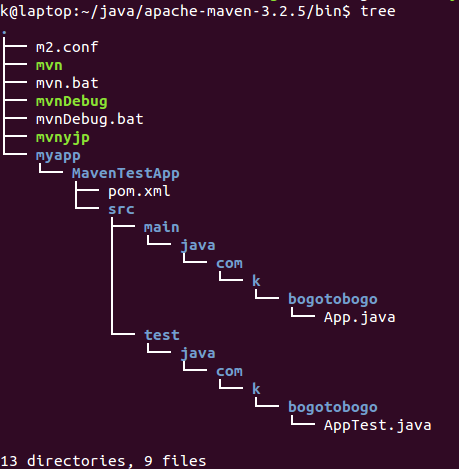
As we saw in the previous section, under MavenTestApp we have pom.xml file.
pom stands for Project Object Model. It is the basic unit in Maven. It always resides in the base directory of the project as pom.xml. The pom contains information about the project and various configuration detail used by Maven to build the project(s) as well as the goals and plugins. While executing a task or goal, Maven looks for the pom in the current directory. It reads the pom, gets the configuration information, then executes the goal.
Here is our new pom file:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.k.bogotobogo</groupId> <artifactId>MavenTestApp</artifactId> <version>1.0-SNAPSHOT</version> <packaging>jar</packaging> <name>MavenTestApp</name> <url>http://maven.apache.org</url> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> </properties> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> </dependencies> </project>
The pom.xml file is the core of a project's configuration in Maven. It is a single configuration file that contains the majority of information required to build a project in just the way we want. The pom is huge and can be daunting in its complexity, but it is not necessary to understand all of the intricacies just yet to use it effectively.
Note that the pom has also the dependency section. In our simple case, it only depends on junit 3.8.
Dependencies declared in our project's pom.xml file often have their own dependencies called transitive dependencies.
The picture below provides an example of transitive dependencies. Notice that transitive dependencies can have their own dependencies. As we might imagine, this can quickly get complex, especially when multiple direct dependencies pull different versions of the same JAR file.
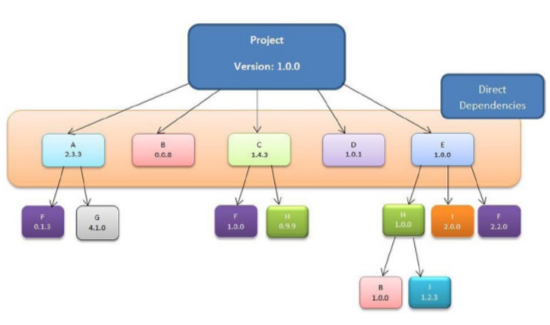
Picture source : Introducing Maven
Maven uses a technique known as dependency mediation to resolve version conflicts.
Simply stated, dependency mediation allows Maven to pull the dependency that is closest to the project in the tree. In the figure, there are two versions of dependency B: 0.0.8 and 1.0.0. In this scenario, version 0.0.8 of dependency B is included in the project, because it is a direct dependency and closest to the tree. Now look at the three versions of dependency F: 0.1.3, 1.0.0, and 2.2.0. All three dependencies are at the same depth. In this scenario, Maven will use the first-found dependency, which would be 0.1.3, and not the latest 2.2.0 version.
Let's look into the src folder. Maven generated two folders for us: main and test.
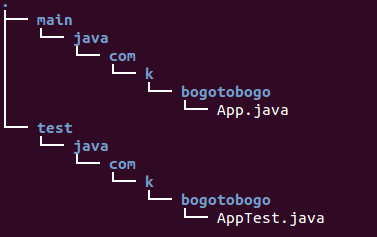
Maven places Java source code in the src\main\java folder. This makes it easier to understand and navigate any Maven project.
Here is the generated "Hello World!" java source code ():
package com.k.bogotobogo; /** * Hello world! * */ public class App { public static void main( String[] args ) { System.out.println( "Hello World!" ); } }
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization