Apache Maven 3 : Compile, Build, and install a Maven project - 2020
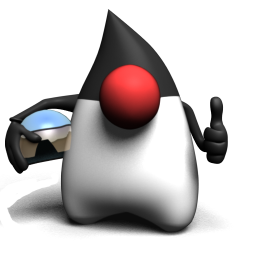
Continuing from Apache Maven 3 - Setting up and creating a project.
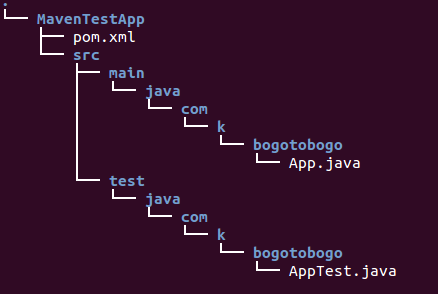
To compile a Maven project, we need to go to the project base directory:
k@laptop:~/java/apache-maven-3.2.5/bin/myapp$ cd MavenTestApp/ k@laptop:~/java/apache-maven-3.2.5/bin/myapp/MavenTestApp$ ls pom.xml src
Let's compile the project:
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/MavenTestApp$ mvn compile [INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building MavenTestApp 1.0-SNAPSHOT [INFO] ------------------------------------------------------------------------ Downloading: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-resources-plugin/2.3/maven-resources-plugin-2.3.pom Downloaded: http://repo.maven.apache.org/maven2/org/apache/maven/plugins/maven-resources-plugin/2.3/maven-resources-plugin-2.3.pom (5 KB at 6.7 KB/sec) ... [INFO] --- maven-resources-plugin:2.3:resources (default-resources) @ MavenTestApp --- ... [INFO] Using 'UTF-8' encoding to copy filtered resources. [INFO] skip non existing resourceDirectory /home/k/java/apache-maven-3.2.5/bin/myapp/MavenTestApp/src/main/resources [INFO] [INFO] --- maven-compiler-plugin:2.0.2:compile (default-compile) @ MavenTestApp ---[INFO] Compiling 1 source file to /home/k/java/apache-maven-3.2.5/bin/myapp/MavenTestApp/target/classes [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 10.414s [INFO] Finished at: Sun Feb 01 07:37:37 PST 2015 [INFO] Final Memory: 15M/101M [INFO] ------------------------------------------------------------------------ k@laptop:~/java/apache-maven-3.2.5/bin/myapp/MavenTestApp$ ...
When a phase is given, Maven will execute every phase in the sequence up to and including the one defined. For example, since we executed the compile phase, the phases that actually get executed are:
- validate
- generate-sources
- process-sources
- generate-resources
- process-resources
- compile
The mvn package will produce jar file and may download some plugins during the process:
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/MavenTestApp$ mvn package ... [INFO] Building jar: /home/k/java/apache-maven-3.2.5/bin/myapp/MavenTestApp/target/MavenTestApp-1.0-SNAPSHOT.jar [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 15.990s [INFO] Finished at: Sun Feb 01 07:57:59 PST 2015 [INFO] Final Memory: 14M/161M [INFO] ------------------------------------------------------------------------ k@laptop:~/java/apache-maven-3.2.5/bin/myapp/MavenTestApp$
As we can see from the output, it generated MavenTestApp-1.0-SNAPSHOT.jar files under the target folder.
Note that the mvn package also ran the test case:
/home/k/java/apache-maven-3.2.5/bin/myapp/MavenTestApp/src/test/java/com/k/bogotobogo/AppTest.java
package com.k.bogotobogo; import junit.framework.Test; import junit.framework.TestCase; import junit.framework.TestSuite; /** * Unit test for simple App. */ public class AppTest extends TestCase { /** * Create the test case * * @param testName name of the test case */ public AppTest( String testName ) { super( testName ); } /** * @return the suite of tests being tested */ public static Test suite() { return new TestSuite( AppTest.class ); } /** * Rigourous Test :-) */ public void testApp() { assertTrue( true ); } }
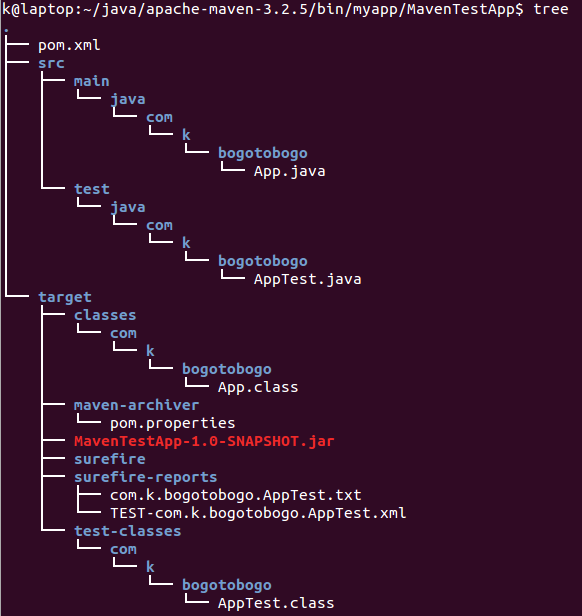
You may test the newly compiled and packaged jar with the following command:
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/MavenTestApp$ java -cp target/MavenTestApp-1.0-SNAPSHOT.jar com.k.bogotobogo.App Hello World!
Maven defines 3 lifecycles in META-INF/plexus/components.xml:
- Default Lifecycle : defined without any associated plugin. Plugin bindings for this lifecycle are defined separately for every packaging:
- Clean Lifecycle : lifecycle is defined directly with its plugin bindings.
- Site Lifecycle : defined directly with its plugin bindings.
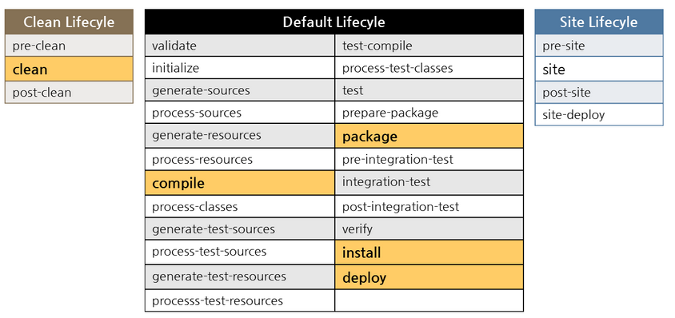
When we use mvn install, Maven goes through all the necessary build phases of Maven again.
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/MavenTestApp$ mvn install ... [INFO] --- maven-install-plugin:2.3:install (default-install) @ MavenTestApp --- [INFO] Installing /home/k/java/apache-maven-3.2.5/bin/myapp/MavenTestApp/target/MavenTestApp-1.0-SNAPSHOT.jar to /home/k/.m2/repository/com/k/bogotobogo/MavenTestApp/1.0-SNAPSHOT/MavenTestApp-1.0-SNAPSHOT.jar [INFO] Installing /home/k/java/apache-maven-3.2.5/bin/myapp/MavenTestApp/pom.xml to /home/k/.m2/repository/com/k/bogotobogo/MavenTestApp/1.0-SNAPSHOT/MavenTestApp-1.0-SNAPSHOT.pom [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 9.892s [INFO] Finished at: Sun Feb 01 10:01:13 PST 2015 [INFO] Final Memory: 7M/99M [INFO] ------------------------------------------------------------------------
We see the jar file, MavenTestApp-1.0-SNAPSHOT.jar and pom file, MavenTestApp-1.0-SNAPSHOT.pom have been installed into /home/k/.m2/repository/.
k@laptop:~/.m2/repository$ pwd /home/k/.m2/repository k@laptop:~/.m2/repository$ ls antlr commons-codec dom4j plexus aopalliance commons-collections javax slide asm commons-configuration jaxen sslext avalon-framework commons-digester jdiff tomcat backport-util-concurrent commons-el jdom wsdl4j biz commons-httpclient jline xerces bouncycastle commons-io jtidy xml-apis bsh commons-lang junit xmlenc classworlds commons-logging log4j xml-resolver com commons-net logkit xom commons-beanutils commons-pool net xpp3 commons-chain commons-validator org commons-cli de oro
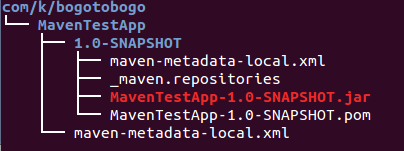
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization