Exception Handling II - 2020
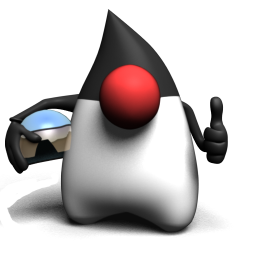
Every exception is an instance of a class that has class Exception in its inheritance hierarchy. In other words, exceptions are always a subclass of java.lang.Exceptions.
All exception classes are subtypes of class Exception which derives from the class Throwable which is derives from the class Object.
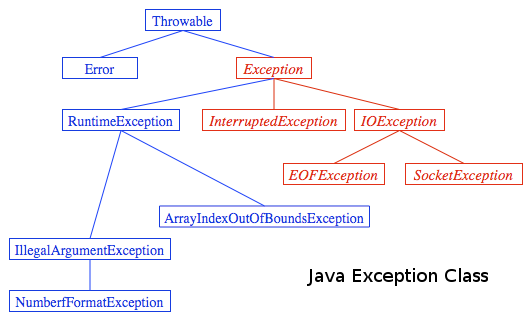
As we see, there are two subclasses that derive from Throwable: Exception and Error.
- Error
- This represents unusual situations which are not from program errors, and indicate things that would not normally happen during the execution, such as the running out of memory.
- In general, our application won't be able to recover from an Error, so we're not required to handle them.
- Errors are technically not exceptions because they do not derive from class Exception.
- Exception
- This represents something that happened not as a result of a programming error, but rather because some resource is not available or some other condition required for execution in not present.
- For example, if our application is supposed to communicate with another program that's not answering, this is an exception that is not caused by a bug.
- RuntimeExceptions are a special case because they sometimes do indicate program errors.
Except for RuntimeException, Error, and their subclasses, all exceptions are called checked exceptions. The compiler ensures that if a method can throw a checked exception, directly or indirectly, the method explicitly deal with it. The method must either catch the exception and take the appropriate action, or pass the exception onto ins caller.
Exceptions defined by Error and RuntimeException classes and their subclasses are known as unchecked exception, meaning that a method is not obliged to deal with these kinds of exceptions. They are either irrecoverable (Error class) and the program should not attempt to deal with them, or they are programming errors (RuntimeException class) and should usually be dealt with as such, and not as exceptions.
- Checked Exceptions
- Exceptions that are not RuntimeException and Error
- All Exceptions the compiler cares about are called checked exceptions which really mean compiler-checked exceptions.
- Caused by a condition that fails at runtime in ways that we cannot predict or prevent.
- We cannot guarantee the server is up.
- The method should do "handle or declare"
- Use try/catch blocks to try to recover from situations we can't guarantee will succeed.
- At the very least, print out a message to the user and a stack trace, so somebody can figure out what happened.
- Most RuntimeExceptions come from a problem in our code logic.
- We can make sure our code doesn't index off the end of an array.
- We want RuntimeExceptions to happen at development and testing time.
- We don't want to code in a try/catch and have the overhead that goes with it, to catch something that shouldn't happen in the first place.
- We can throw, catch, and declare RuntimeExceptions, but we don't have to, and the compiler won't check.
The compiler checks for everything except for RuntimeExceptions .
The compiler guarantees:
- If you throw an exception in your code you must declare it using the throw keyword in your method declaration.
- If you call a method that throws an exception, in other words, a method that declares it throws an exception, you must acknowledge that you're aware of the exception possibility. One way to satisfy the compiler is to wrap the call in a try/catch.
- If you're not prepared to handle the exception, you can still make the compiler happy by officially ducking the exception.
- ArrayIndexOutOfBoundsException
Thrown when attempting to access an array with an invalid value (either negative or beyond the length of the array. - ClassCastException
Thrown when attempting to cast a reference variable to a type that fails the IS-A test. - IllegalArgumentException
Thrown when a method receives an argument formatted differently that the method expects. - IllegalStateException
Thrown when the state of the environment doesn't match the operation being attempted, e.g., using a Scanner that's been closed. - NullPointerException
Thrown when attempting to access an object with a reference variable whose current value is null.class NullPointerEx { static String s; public static void main (String[] args) { System.out.println(s.length()); //NullPointerException } }
- NumberFormatException
Thrown when a method that converts a String to a number receives a String that it cannot convert.int parseInt(String s) throws NumberFormatException { boolean parseSuccess = false; int result = 0; // parsing if(!parseSuccess) throw new NumberFormatException(); return result; }
- AssertionError
Thrown when a statement's boolean test returns false. - ExceptionInInitializerError
Thrown when attempting to initialize a static variable or an initialization block.public class InitializationError { static int [] j = new int [4]; static { j[4] = 10 ; } // bad array index public static void main( String[] args) {} }
- StackOverflowError
Thrown when a method recurses too deeply.void callme() { callme(); }
- NoClassDefFoundError
Thrown when the JVM can't find a class it needs, because of a command-line error, a classpath issue, or a missing class file.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization