Apache Maven 3 : Web Application - 2020
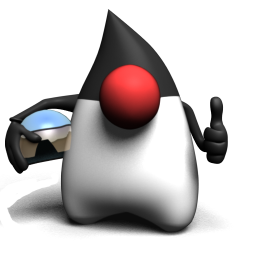
bogotobogo.com site search:
mvn archetype:generate
Continuing from Apache Maven 3 - Dependencies.
In this chapter, we'll create a new web application which provides us *.war file and additional dependencies.
While we're running mvn archetype:generate, we'll choose
764: remote -> org.codehaus.mojo.archetypes:webapp-j2ee14 (J2EE 1.4 web application archetype)
as our archetype.
k@laptop:~/java/apache-maven-3.2.5/bin/myapp$ mvn archetype:generate ... Choose a number or apply filter (format: [groupId:]artifactId, case sensitive contains): 525: 764 Choose org.codehaus.mojo.archetypes:webapp-j2ee14 version: 1: 1.0 2: 1.0.1 3: 1.1 4: 1.2 5: 1.3 Choose a number: 5: Downloading: http://repo.maven.apache.org/maven2/org/codehaus/mojo/archetypes/webapp-j2ee14/1.3/webapp-j2ee14-1.3.jar Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/mojo/archetypes/webapp-j2ee14/1.3/webapp-j2ee14-1.3.jar (2 KB at 10.7 KB/sec) Downloading: http://repo.maven.apache.org/maven2/org/codehaus/mojo/archetypes/webapp-j2ee14/1.3/webapp-j2ee14-1.3.pom Downloaded: http://repo.maven.apache.org/maven2/org/codehaus/mojo/archetypes/webapp-j2ee14/1.3/webapp-j2ee14-1.3.pom (911 B at 9.1 KB/sec) Define value for property 'groupId': : com.k.bogotobogo Define value for property 'artifactId': : mywebapp Define value for property 'version': 1.0-SNAPSHOT: : Define value for property 'package': com.k.bogotobogo: : Confirm properties configuration: groupId: com.k.bogotobogo artifactId: mywebapp version: 1.0-SNAPSHOT package: com.k.bogotobogo Y: : [INFO] ---------------------------------------------------------------------------- [INFO] Using following parameters for creating project from Archetype: webapp-j2ee14:1.3 [INFO] ---------------------------------------------------------------------------- [INFO] Parameter: groupId, Value: com.k.bogotobogo [INFO] Parameter: artifactId, Value: mywebapp [INFO] Parameter: version, Value: 1.0-SNAPSHOT [INFO] Parameter: package, Value: com.k.bogotobogo [INFO] Parameter: packageInPathFormat, Value: com/k/bogotobogo [INFO] Parameter: package, Value: com.k.bogotobogo [INFO] Parameter: version, Value: 1.0-SNAPSHOT [INFO] Parameter: groupId, Value: com.k.bogotobogo [INFO] Parameter: artifactId, Value: mywebapp [INFO] project created from Archetype in dir: /home/k/java/apache-maven-3.2.5/bin/myapp/mywebapp [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 59.347s [INFO] Finished at: Sun Feb 01 14:07:12 PST 2015 [INFO] Final Memory: 18M/163M [INFO] ------------------------------------------------------------------------ k@laptop:~/java/apache-maven-3.2.5/bin/myapp/mywebapp$ ls pom.xml src
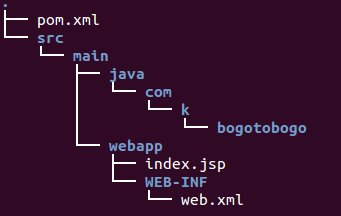
pom.xml
Here is our new pom.xml which Maven has generated for us:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.k.bogotobogo</groupId> <artifactId>mywebapp</artifactId> <version>1.0-SNAPSHOT</version> <packaging>war</packaging> <name>mywebapp</name> <url>http://maven.apache.org</url> <dependencies> <dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <version>2.4</version> <scope>provided</scope> </dependency> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.1</version> <scope>provided</scope> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>2.0.2</version> <configuration> <source>1.4</source> <target>1.4</target> </configuration> </plugin> </plugins> </build> </project>
Three things are worth mentioning:
- We have war package instead of jar package since it's a web application.
<packaging>war</packaging>
- We have servlet and jsp dependencies.
<dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <version>2.4</version> <scope>provided</scope> </dependency> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.1</version> <scope>provided</scope> </dependency>
- The compiler in Maven is actually a plugin. We set the behavior, especially, the configuration:
<plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>2.0.2</version> <configuration> <source>1.4</source> <target>1.4</target> </configuration> </plugin>
mvn compile
When we compile it, Maven will download all packages that our project is dependent on:
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/mywebapp$ mvn compile [INFO] Scanning for projects... [INFO] [INFO] ------------------------------------------------------------------------ [INFO] Building mywebapp 1.0-SNAPSHOT [INFO] ------------------------------------------------------------------------ Downloading: http://repo.maven.apache.org/maven2/javax/servlet/servlet-api/2.4/servlet-api-2.4.pom Downloaded: http://repo.maven.apache.org/maven2/javax/servlet/servlet-api/2.4/servlet-api-2.4.pom (156 B at 0.2 KB/sec) Downloading: http://repo.maven.apache.org/maven2/javax/servlet/servlet-api/2.4/servlet-api-2.4.jar Downloaded: http://repo.maven.apache.org/maven2/javax/servlet/servlet-api/2.4/servlet-api-2.4.jar (96 KB at 165.9 KB/sec) [INFO] [INFO] --- maven-resources-plugin:2.3:resources (default-resources) @ mywebapp --- [WARNING] Using platform encoding (UTF-8 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory /home/k/java/apache-maven-3.2.5/bin/myapp/mywebapp/src/main/resources [INFO] [INFO] --- maven-compiler-plugin:2.0.2:compile (default-compile) @ mywebapp --- [INFO] Nothing to compile - all classes are up to date [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 6.477s [INFO] Finished at: Sun Feb 01 15:15:00 PST 2015 [INFO] Final Memory: 11M/97M [INFO] ------------------------------------------------------------------------ k@laptop:~/java/apache-maven-3.2.5/bin/myapp/mywebapp$
mvn package
mvn package:
k@laptop:~/java/apache-maven-3.2.5/bin/myapp/mywebapp$ mvn package ... [INFO] [INFO] --- maven-war-plugin:2.1.1:war (default-war) @ mywebapp --- [INFO] Packaging webapp [INFO] Assembling webapp [mywebapp] in [/home/k/java/apache-maven-3.2.5/bin/myapp/mywebapp/target/mywebapp-1.0-SNAPSHOT] [INFO] Processing war project [INFO] Copying webapp resources [/home/k/java/apache-maven-3.2.5/bin/myapp/mywebapp/src/main/webapp] [INFO] Webapp assembled in [98 msecs] [INFO] Building war: /home/k/java/apache-maven-3.2.5/bin/myapp/mywebapp/target/mywebapp-1.0-SNAPSHOT.war [INFO] WEB-INF/web.xml already added, skipping [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 10.686s [INFO] Finished at: Sun Feb 01 15:16:40 PST 2015 [INFO] Final Memory: 12M/161M [INFO] ------------------------------------------------------------------------
generated files
Let's look at what's been generated so far:
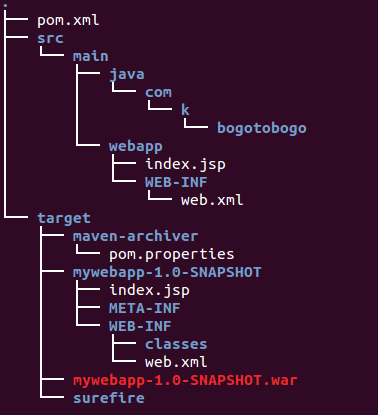
- We see our package file mywebapp-1.0-SNAPSHOT.war in target folder.
- When we look into the src directory, we have nothing under src/main/java but we have index.jsp under the src/main/webapp folder:
<%@page contentType="text/html" pageEncoding="UTF-8"%> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8"> <title>JSP Page</title> </head> <body> <h1>Hello World!</h1> </body> </html>
The web.xml should look like this:<?xml version="1.0" encoding="UTF-8"?> <web-app version="2.4" xmlns="http://java.sun.com/xml/ns/j2ee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> <display-name>mywebapp</display-name> <session-config> <session-timeout> 30 </session-timeout> </session-config> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> </web-app>
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization