Mat(rix) object (Image Container)
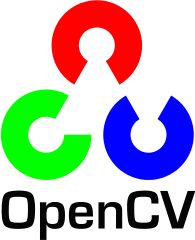
Every image is a matrix: it is stored as a matrix and it is manipulated as a matrix.
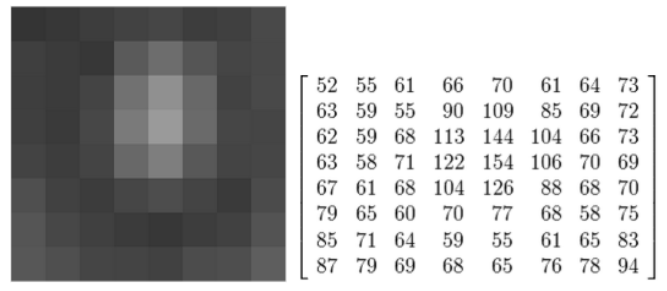
For more information about getting the images from sensors or how we process them, please visit:
OpenCV is an image processing library which contains a large collection of image processing functions. In lots of cases, we have to use multiple functions, and passing images to other functions. Those functions perform computationally heavy task. We do not want to put additional stress to the processing system which is already experiencing huge stress.
Via a reference counting system, OpenCV's Mat (matrix) object can be shared and can be passed around without expensive copies (each Mat object has its own header, however the matrix may be shared between two instance of them by having their matrix pointers point to the same address). Whenever we copy a header of a Mat object, a counter is increased for the matrix. Whenever a header is cleaned this counter is decreased. When the counter reaches zero, the matrix is freed.
Note that the Mat's pointer is pointing to the headers of large matrix, not the data itself(i.e. the copy operators will only copy the headers). So, when we create Mat object, actually it is creating a head:
Mat imageA, imageB;
When we load an image, it's allocating memory for the matrix:
imageA = imread( argv[1], 1 );
Copy or assign:
imageC(image1); // copy constructor imageB = imageA; // assignment
After those operations, in the end, all images are pointing to the same single data matrix though their header parts are different.
Note that can create headers which refer to only a subsection of the full data. For example, to create a region of interest (ROI) in an image, we just create a new header with the new boundaries:
Mat imageD (imageA, Rect(10, 10, 150, 150) ); // a rectangle Mat imageE = imageA(Range:all(), Range(2,5)); // row and column boundaries
We may want to copy the matrix itself, we can use clone() and copyTo() functions:
Mat imageB = imageA.clone(); Mat imageC; imageA.copyTo(C);
Now modifying imageB or imageC will not affect the matrix pointed by the Mat header of imageA.
Here are what we've learned so far: The Basic Image Container.
- Output image allocation for OpenCV functions is automatic (unless specified otherwise).
- You do not need to think about memory management with OpenCVs C++ interface.
- The assignment operator and the copy constructor only copies the header.
- The underlying matrix of an image may be copied using the clone() and copyTo() functions.
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization