Hough transform : Circle

The classical Hough transform was developed to identify lines in the image, but later the Hough transform has been extended to identify the positions of arbitrary shapes, most commonly circles or ellipses.
"In many cases an edge detector can be used as a pre-processing stage to obtain image points or image pixels that are on the desired curve in the image space. Due to imperfections in either the image data or the edge detector, however, there may be missing points or pixels on the desired curves as well as spatial deviations between the ideal line/circle/ellipse and the noisy edge points as they are obtained from the edge detector. For these reasons, it is often non-trivial to group the extracted edge features to an appropriate set of lines, circles or ellipses. The purpose of the Hough transform is to address this problem by making it possible to perform groupings of edge points into object candidates by performing an explicit voting procedure over a set of parameterized image objects." - wiki - Hough transform.
A circle can be fully defined with three parameters: the center coordinates ($a$, $b$) and the radius ($R$):
$$x = a + R\cos\theta$$ $$y = b + R\sin\theta$$As the $\theta$ varies from $0$ to $360$, a complete circle of radius $R$ is created.
So with the Circle Hough Transform, we expect to find triplets of $(x, y, R)$ from the image. In other words, our purpose is to find those three parameters.
Therefore, we need to construct a 3D accumulator for Hough transform, which would be highly ineffective. So, OpenCV uses more trickier method, Hough Gradient Method which uses the gradient information of edges.
import cv2 import numpy as np from matplotlib import pyplot as plt bgr_img = cv2.imread('b.jpg') # read as it is if bgr_img.shape[-1] == 3: # color image b,g,r = cv2.split(bgr_img) # get b,g,r rgb_img = cv2.merge([r,g,b]) # switch it to rgb gray_img = cv2.cvtColor(bgr_img, cv2.COLOR_BGR2GRAY) else: gray_img = bgr_img img = cv2.medianBlur(gray_img, 5) cimg = cv2.cvtColor(img,cv2.COLOR_GRAY2BGR) circles = cv2.HoughCircles(img,cv2.HOUGH_GRADIENT,1,20, param1=50,param2=30,minRadius=0,maxRadius=0) circles = np.uint16(np.around(circles)) for i in circles[0,:]: # draw the outer circle cv2.circle(cimg,(i[0],i[1]),i[2],(0,255,0),2) # draw the center of the circle cv2.circle(cimg,(i[0],i[1]),2,(0,0,255),3) plt.subplot(121),plt.imshow(rgb_img) plt.title('Input Image'), plt.xticks([]), plt.yticks([]) plt.subplot(122),plt.imshow(cimg) plt.title('Hough Transform'), plt.xticks([]), plt.yticks([]) plt.show()
The function we use here is cv2.HoughCircles(). It has plenty of arguments which are well explained in the documentation. So we directly go to the code.
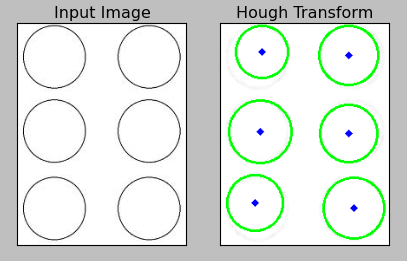
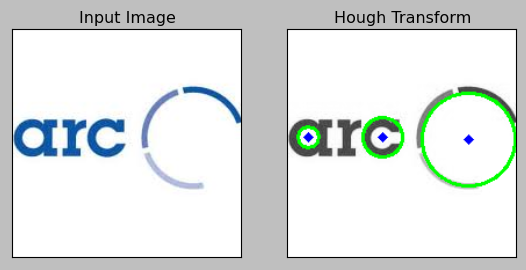
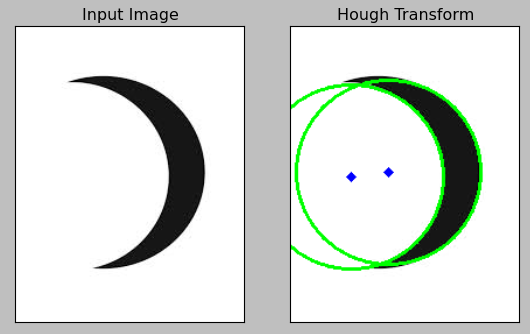
So far so good.
In the following cases, however, the Hough transform finds hidden circles which are not meant to be perceived as circles:
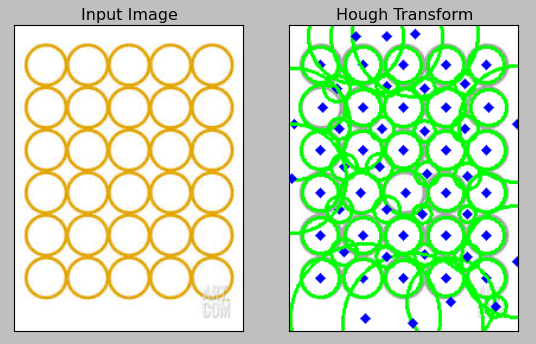
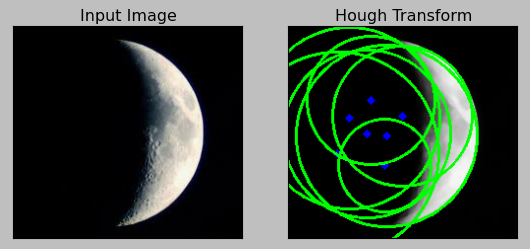
In this Crescent case, we can make it better by blurring more:
img = cv2.medianBlur(gray_img,5) => img = cv2.medianBlur(gray_img,25)
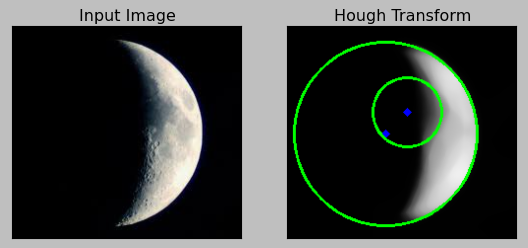
In general, without blurring, the algorithms tends to extract too many circular features. So, to be more successful, the prepocessing seems to be crucial.
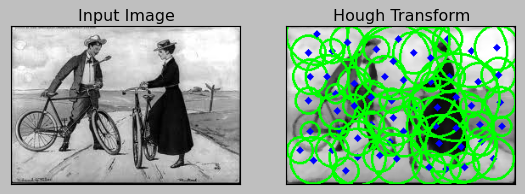
With blurring value 51:
img = cv2.medianBlur(gray_img,51)
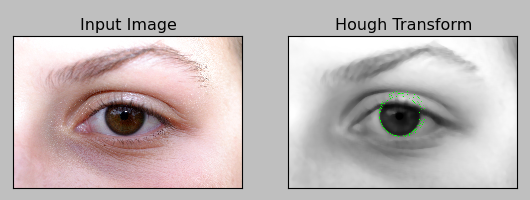
OpenCV 3 Tutorial
image & video processing
Installing on Ubuntu 13
Mat(rix) object (Image Container)
Creating Mat objects
The core : Image - load, convert, and save
Smoothing Filters A - Average, Gaussian
Smoothing Filters B - Median, Bilateral
OpenCV 3 image and video processing with Python
OpenCV 3 with Python
Image - OpenCV BGR : Matplotlib RGB
Basic image operations - pixel access
iPython - Signal Processing with NumPy
Signal Processing with NumPy I - FFT and DFT for sine, square waves, unitpulse, and random signal
Signal Processing with NumPy II - Image Fourier Transform : FFT & DFT
Inverse Fourier Transform of an Image with low pass filter: cv2.idft()
Image Histogram
Video Capture and Switching colorspaces - RGB / HSV
Adaptive Thresholding - Otsu's clustering-based image thresholding
Edge Detection - Sobel and Laplacian Kernels
Canny Edge Detection
Hough Transform - Circles
Watershed Algorithm : Marker-based Segmentation I
Watershed Algorithm : Marker-based Segmentation II
Image noise reduction : Non-local Means denoising algorithm
Image object detection : Face detection using Haar Cascade Classifiers
Image segmentation - Foreground extraction Grabcut algorithm based on graph cuts
Image Reconstruction - Inpainting (Interpolation) - Fast Marching Methods
Video : Mean shift object tracking
Machine Learning : Clustering - K-Means clustering I
Machine Learning : Clustering - K-Means clustering II
Machine Learning : Classification - k-nearest neighbors (k-NN) algorithm
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization