Python Modules and IDLE
(Import, Reload, exec) - 2020
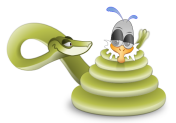
Every file of Python code with .py extension is a module. Other files can have an access to the items a module defines by importing that module. The import operations load another file and give access to that file's contents through its attributes.
Importing x module makes all of its functions and attributes available.
sys.path is a list of directory names that constitute the current search path:>>> sys.path ['', 'C:\\Python32\\Lib\\idlelib', 'C:\\Windows\\system32\\python32.zip', 'C:\\Python32\\DLLs', 'C:\\Python32\\lib', 'C:\\Python32', 'C:\\Python32\\lib\\site-packages'] >>>
Python will look through these directories for a .py file whose name matches what we're trying to import.
In fact, because not all modules are stored as .py files, and some are built-in modules, they are actually baked right into Python itself. Built-in modules behave just like regular modules, but their Python source code is not available, because they are not written in Python but written in C.
Once we import a module, we can reference any of its public functions, classes, or attributes. We access its functions with module.function.
This module-based services model turns out to be the core idea behind program architecture in Python. Larger programs have multiple module files. One of the modules is designated as the main or top-level file, and this is the one launched to start the entire program.
Import operations run the code in a file that is being loaded as a final step. Because of this, importing a file is yet another way to launch it.
For example, if we start an interactive session, we can run the script1.py file we created with a simple import.
C:\workspace> python >>> import script1.py win32 31.6385840391 blah blah blah
This works, but only once per session by default. After the first import, later imports do nothing, even if we change and save the module's source file again in another window:
C:\workspace> python >>> import script1 win32 31.6385840391 blah blah blah >>> import script1 >>> import script1 >>>
This is by design. Imports are too expensive to repeat more than once per file, per program run. If really want to force Python to run the file again in the same session without stopping and restarting the session, we need to instead call the reload function available in the imp standard library module.
>>> from imp import reload >>> reload(script1) win32 31.6385840391 blah blah blah <module 'script1' from 'script1.py'> >>>
The from copies a name out of a module. The reload function loads and runs the current version of the file, picking up changes if it's been changed and saved it in another window. This allows us to edit and pick up new code on the fly.
The reload function expects the name of an already loaded module object, so we have to have successfully imported a module once before we reload it.
The reload is a function that is called while import is a statement. So, reload expects parentheses around the module object name:
reload(script1)But import does not need any parentheses.
That's why we must pass the module name to reload as an argument in parentheses, and that's why we get back an extra output line when reloading. The last output line is just the display representation of the reload call's return value, a Python module object.
Waht's the difference between import and from in Python?
Python's import loads a Python module into its own namespace, so that you have to add the module name followed by a dot in front of references to any names from the imported module that you refer to:
import us # Access the whole module, us us_city = us.california("Sacramento") # Qualify to reference
from loads a Python module into the current namespace, so that you can refer to it without the need to mention the module name again:
from us import * # Copy name into my scope us_city = california("Sacramento") # Use class name directlyor
from us import city us_city = california("Sacramento")
Here is the summary:
- Can't I always use from?
If we are loading a lot of modules using from, we may have conflicts of names. Though from is fine for a small program but for a big project, we would hit problems. - Then, should we always use import?
No, we should use import most of the time, but we may want to use from if we want to refer to the members of a module lots of times in the calling code. In that case, by using from, we can save ourselves.
Import and reloads provide a launch option because import operations execute files as a last step. In the broader scheme of things, however, modules serve the roles of libraries of tools. Actually, a module is mostly just a package of variable names, namespace. The names within that package are called attributes. An attribute is simply a variable name that is attached to a specific object like a module.
Typically, importers gain access to all the names assigned at the top level of a module's file. These names are usually assigned to tools exported by the module such as functions, classes, variables, and so on. Those are intended to be used in other files and other programs. Externally, a module file's names can be fetched with tow Python statements, import and from, as well as the reload call.
Let's make a file called mython.py:
title = "To iterate is human, to recurse divine"
Though the file has only one line doing assignment, when imported, it will generate the module's attribute. The assignment statement creates a module attribute named title.
We can access the title attribute in other components in two different ways:
- We can load the module as a whole with an import statement, and then qualify the module name with the attribute name to fetch it:
C:\workspace> python # Start Python >>> import mython # Run file, load module as a whole >>> print mython.title # Use its attribute names: >'.' to qualify To iterate is human, to recurse divine >>>
The dot expression syntax object.attribute let is fetch any attribute attached to any object, and we used it to access the string title inside the module mython.py.
- We can fetch names out of a module with from statement:
C:\workspace> python # Start Python >>> from mython import title # Run file, copy its names >>> print title # Use name directly, no need to qualify To iterate is human, to recurse divine >>>
The from is just like an import with an extra assignment to names in the importing component. Actually, from copies a module's attributes such that they become simple variables in the recipient. So, we can simply refer to the imported string this time title variable instead of mython.title which is an attribute reference.
Whether we use import or from to invoke an import operation, the statements in the module file mython.py are executed. The importing component, interactive prompt, gain access to names assigned at the top level of the file.
Module files usually have more than one name:
a = "Edsger Dijkstra" b = "Donald Knuth" c = "Guido van Rossum" d = "John McCarthy" print(a,b,c,d)
This file, TheNames.py, assigns four variables, and so generates four attributes for the outside world. It also uses its own four variables in a print statement.
C:\workspace> python TheNames.py ('Edsger Dijkstra', 'Donald Knuth', 'Guido van Rossum', 'John McCarthy') C:\workspace>
Clients of this file that use import get a module with attributes, while client that use from get copies of the file's names:
C:\workspace> C:\workspace> python >>> import TheNames # Grab the whole module ('Edsger Dijkstra', 'Donald Knuth', 'Guido van Rossum ', 'John McCarthy') >>> >>> TheNames.b, TheNames.d ('Donald Knuth', 'John McCarthy') >>> >>> from TheNames import a,b,c,d # Copy multiple names >>> a,c ('Edsger Dijkstra', 'Guido van Rossum ') >>>
The results here are printed in parentheses because they are really tuples.
Once we start coding modules with multiple names like this, the built-in dir function starts to come in handy. We can use it to fetch a list of the names available inside a module. The following returns a Python list of strings:
('Edsger Dijkstra', 'Guido van Rossum ') >>> dir(TheNames) ['__builtins__', '__doc__', '__file__', '__name__', '__package__', 'a', 'b', 'c' , 'd'] >>>
When the dir function is called with the name of an imported module passed in parentheses like this, it returns all the attributes inside that module. Some of the names it returns have leading and trailing double underscores. These are built0in names that are always predefined by Python and that have special meaning to the interpreter. The variables of our code, a,b,c, and d show up last in the dir result.
Python programs are composed of multiple module files, linked together by import statement. Each module file is a self-contained package of variables, a namespace. One module file cannot see the names defined in another file unless it explicitly imports that other file. So, modules serve to minimize name collisions in our code. Because each file is a self-contained namespace, the names in one file cannot clash with those in another.
The exec(open('module.py').read()) built-in function call is another way to launch files from the interactive prompt without having to import and later reload. Each exec runs the current version of the file, without requiring later reloads:
C:\workspace> python >>> exec(open('script1.py').read()) win32 31.6385840391 blah blah blah ... script1.py has been changed >>> exec(open('script1.py').read()) win32 33.331666625 blah! blah! blah! blah! blah! >>>
The exec call has an effect similar to an import. But it doesn't import the module. Each time we call exec this way, it runs the file anew as though we had pasted it in at the place where exec is called. Because of that, exec does not require module reloads after file changes. It skips the normal import logic.
On the downside, because it works as if pasting code into the place where it is called, exec, like the from statement, has the potential to silently overwrite variables we may currently be using.
By contrast, the basic import statement runs the file only once per process, and it makes the file a separate module namespace so that its assignments will not change variables in our scope.
Like any other languages, Python has exceptions. Actually, exceptions are everywhere in Python. Virtually every module in the standard Python library uses them, and Python itself will raise them in a lot of different circumstances.
So, what is an exception?
In general, it's an indication of errors. Some programming languages encourage the use of error return codes, which we can check. Python encourages the use of exceptions, which we can handle.
When an error occurs in the Python Shell, it prints out some details about the exception and how it happened. We call them unhandled exception. When the exception was raised, if there was no code to explicitly notice it and deal with it, Python turns around and heads its way back up to the top level of the Python Shell, which spits out some debugging information. In the shell, that may not be a big issue at all, but if that happened while our actual Python program was running, the entire program would come to a halt if nothing handles the exception.
Python functions don't declare what kind of exceptions they might raise. It's up to us to decide what possible exceptions we need to catch.
An exception doesn't necessarily result in a complete program crash, though. Exceptions can be handled. Sometimes an exception occurs because we have a bug in our code, but sometimes an exception is something we can anticipate. For example, when we're opening a file, it might not be available for some reason. If we're importing a module, it might not be installed. If we're connecting to a database, it might be unavailable, or we might not have the correct security credentials to access it. If we know a line of code may raise an exception, we should handle the exception using a try...except block.
Python uses try...except blocks to handle exceptions, and the raise statement to generate them. In Java and C++, we use try...catch blocks to handle exceptions, and the throw statement to generate them.
The syntax for raising an exception is simple. Use the raise statement, followed by the exception name, and an optional human-readable string for debugging purposes.
#tst.py def raising_python_exception(x, y): try: z = x/y except ZeroDivisionError, e: err = e print e raising_python_exception(1,0)
If we run the code, we get:
C:\TEST>python tst.py integer division or modulo by zero
We don't need to handle an exception in the function that raises it. If one function doesn't handle it, the exception is passed to the calling function, then that function's calling function, and so on up the stack. If the exception is never handled, our program will crash, Python will print a traceback to standard error, and that's the end of it.
Maybe that's what we want. It depends on what our program is up to.
When you try to import a module and fail, then Python will raise a built-in exception, ImportError. This can occur for a various reasons, but the simplest case is when the module doesn't exist in our import search path.
We can use this to include optional features in our program. For example, the chardet library provides character encoding auto-detection. Perhaps our program wants to use this library if it exists, but continue gracefully if the user hasn't installed it. We can do this with a try...except block.
try: import chardet except ImportError: chardet = None
Later, we can check for the presence of the chardet module with a simple if statement:
if chardet: # do something else: # continue anyway
IDLE provides a graphical user interface for Python. IDLE uses a Python program that uses the tkinter GUI toolkit. It runs portably on most Python platforms.
We can start IDLE from the Start button menu for Python on Windows system. It can also be selected by right-clicking on a Python program icon.
The picture below shows the IDLE/ The Python shell window that opens initially is the main window. It runs an interactive session. This works like all interactive sessions. The code we type here is run immediately after we type it.
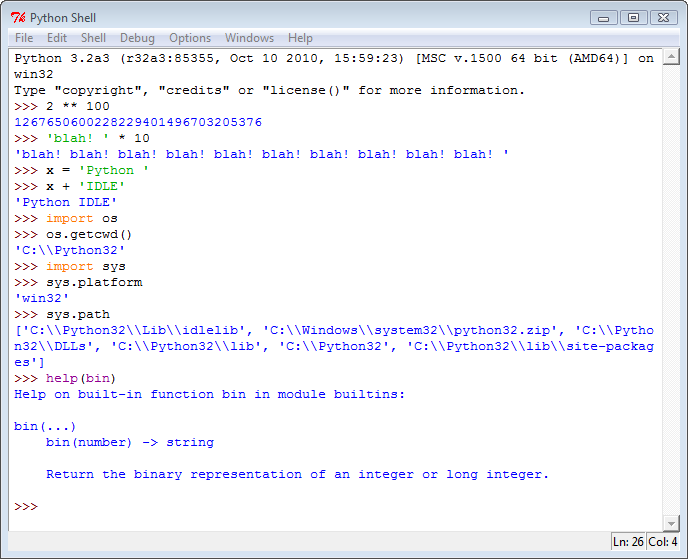
To run a file of code that we're editing in IDLE, select the file's text edit window, open that window's Run pull-down menu, and choose the Run Module option listed.
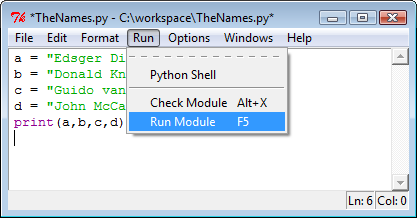
When run this way, the output of our script and any error messages it may generate show up back in the main interactive window, the Python shell window.
Python tutorial
Python Home
Introduction
Running Python Programs (os, sys, import)
Modules and IDLE (Import, Reload, exec)
Object Types - Numbers, Strings, and None
Strings - Escape Sequence, Raw String, and Slicing
Strings - Methods
Formatting Strings - expressions and method calls
Files and os.path
Traversing directories recursively
Subprocess Module
Regular Expressions with Python
Regular Expressions Cheat Sheet
Object Types - Lists
Object Types - Dictionaries and Tuples
Functions def, *args, **kargs
Functions lambda
Built-in Functions
map, filter, and reduce
Decorators
List Comprehension
Sets (union/intersection) and itertools - Jaccard coefficient and shingling to check plagiarism
Hashing (Hash tables and hashlib)
Dictionary Comprehension with zip
The yield keyword
Generator Functions and Expressions
generator.send() method
Iterators
Classes and Instances (__init__, __call__, etc.)
if__name__ == '__main__'
argparse
Exceptions
@static method vs class method
Private attributes and private methods
bits, bytes, bitstring, and constBitStream
json.dump(s) and json.load(s)
Python Object Serialization - pickle and json
Python Object Serialization - yaml and json
Priority queue and heap queue data structure
Graph data structure
Dijkstra's shortest path algorithm
Prim's spanning tree algorithm
Closure
Functional programming in Python
Remote running a local file using ssh
SQLite 3 - A. Connecting to DB, create/drop table, and insert data into a table
SQLite 3 - B. Selecting, updating and deleting data
MongoDB with PyMongo I - Installing MongoDB ...
Python HTTP Web Services - urllib, httplib2
Web scraping with Selenium for checking domain availability
REST API : Http Requests for Humans with Flask
Blog app with Tornado
Multithreading ...
Python Network Programming I - Basic Server / Client : A Basics
Python Network Programming I - Basic Server / Client : B File Transfer
Python Network Programming II - Chat Server / Client
Python Network Programming III - Echo Server using socketserver network framework
Python Network Programming IV - Asynchronous Request Handling : ThreadingMixIn and ForkingMixIn
Python Coding Questions I
Python Coding Questions II
Python Coding Questions III
Python Coding Questions IV
Python Coding Questions V
Python Coding Questions VI
Python Coding Questions VII
Python Coding Questions VIII
Python Coding Questions IX
Python Coding Questions X
Image processing with Python image library Pillow
Python and C++ with SIP
PyDev with Eclipse
Matplotlib
Redis with Python
NumPy array basics A
NumPy Matrix and Linear Algebra
Pandas with NumPy and Matplotlib
Celluar Automata
Batch gradient descent algorithm
Longest Common Substring Algorithm
Python Unit Test - TDD using unittest.TestCase class
Simple tool - Google page ranking by keywords
Google App Hello World
Google App webapp2 and WSGI
Uploading Google App Hello World
Python 2 vs Python 3
virtualenv and virtualenvwrapper
Uploading a big file to AWS S3 using boto module
Scheduled stopping and starting an AWS instance
Cloudera CDH5 - Scheduled stopping and starting services
Removing Cloud Files - Rackspace API with curl and subprocess
Checking if a process is running/hanging and stop/run a scheduled task on Windows
Apache Spark 1.3 with PySpark (Spark Python API) Shell
Apache Spark 1.2 Streaming
bottle 0.12.7 - Fast and simple WSGI-micro framework for small web-applications ...
Flask app with Apache WSGI on Ubuntu14/CentOS7 ...
Fabric - streamlining the use of SSH for application deployment
Ansible Quick Preview - Setting up web servers with Nginx, configure enviroments, and deploy an App
Neural Networks with backpropagation for XOR using one hidden layer
NLP - NLTK (Natural Language Toolkit) ...
RabbitMQ(Message broker server) and Celery(Task queue) ...
OpenCV3 and Matplotlib ...
Simple tool - Concatenating slides using FFmpeg ...
iPython - Signal Processing with NumPy
iPython and Jupyter - Install Jupyter, iPython Notebook, drawing with Matplotlib, and publishing it to Github
iPython and Jupyter Notebook with Embedded D3.js
Downloading YouTube videos using youtube-dl embedded with Python
Machine Learning : scikit-learn ...
Django 1.6/1.8 Web Framework ...
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization