Image - OpenCV BGR : Matplotlib RGB

In my python tutorial, I briefly introduced the Matplotlib, NumPy, and SciPy. But it did not address images at all.
So, I'm writing here to show how we handle images with Matplotlib in python. Once again, briefly.
Unlike the example of the previous chapter which loads color image as a grayscale image, this code will load color image and then convert it to grayscale.
We'll play with the picture below:
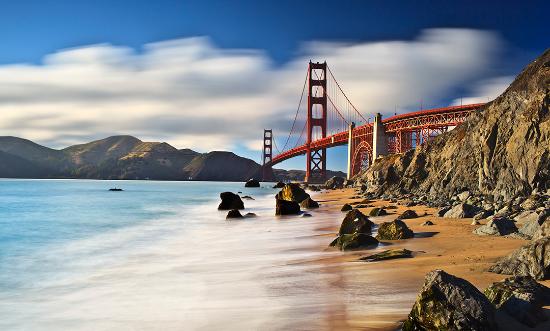
Here is the code:
import cv2 import numpy as np import matplotlib.pyplot as plt bgr_img = cv2.imread('images/san_francisco.jpg') gray_img = cv2.cvtColor(bgr_img, cv2.COLOR_BGR2GRAY) cv2.imwrite('san_francisco_grayscale.jpg',gray_img) plt.imshow(gray_img, cmap = plt.get_cmap('gray')) plt.xticks([]), plt.yticks([]) # to hide tick values on X and Y axis plt.show() while True: k = cv2.waitKey(0) & 0xFF # 0xFF? To get the lowest byte. if k == 27: break # Code for the ESC key cv2.destroyAllWindows()
The image plotted by Matplotlib:
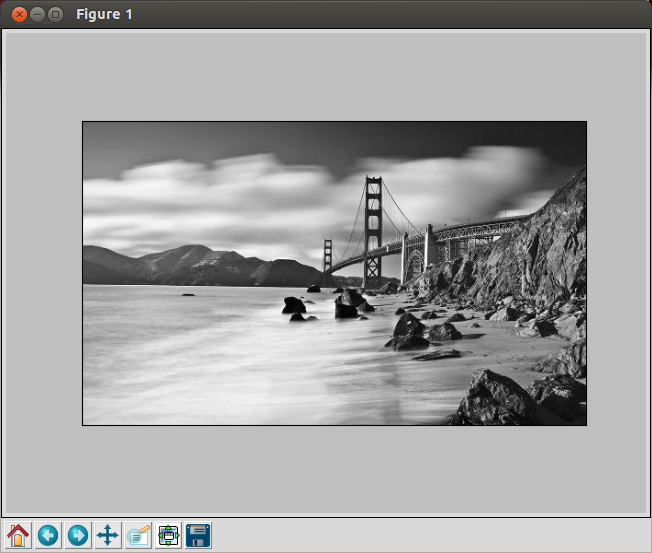
There is a difference in pixel ordering in OpenCV and Matplotlib. OpenCV follows BGR order, while matplotlib likely follows RGB order.
Therefore, when we display an image loaded in OpenCV using matplotlib functions, we may want to convert it into RGB mode.
Source code looks like this:
import cv2 import numpy as np import matplotlib.pyplot as plt bgr_img = cv2.imread('images/san_francisco.jpg') b,g,r = cv2.split(bgr_img) # get b,g,r rgb_img = cv2.merge([r,g,b]) # switch it to rgb plt.imshow(rgb_img) plt.xticks([]), plt.yticks([]) # to hide tick values on X and Y axis plt.show() while True: k = cv2.waitKey(0) & 0xFF # 0xFF? To get the lowest byte. if k == 27: break # Code for the ESC key cv2.destroyAllWindows()
Here, we pixel order switched to rgb from brg. We got the following picture:
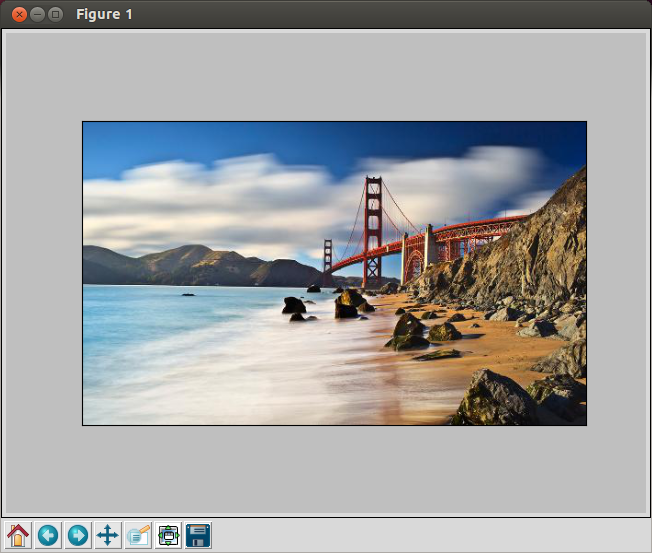
If we did not switch brg order, we get rgb inverted picture.
import cv2 import numpy as np import matplotlib.pyplot as plt bgr_img = cv2.imread('images/san_francisco.jpg') # b,g,r = cv2.split(bgr_img) # get b,g,r # rgb_img = cv2.merge([r,g,b]) # switch it to rgb plt.imshow(bgr_img) plt.xticks([]), plt.yticks([]) # to hide tick values on X and Y axis plt.show() while True: k = cv2.waitKey(0) & 0xFF # 0xFF? To get the lowest byte. if k == 27: break # Code for the ESC key cv2.destroyAllWindows()
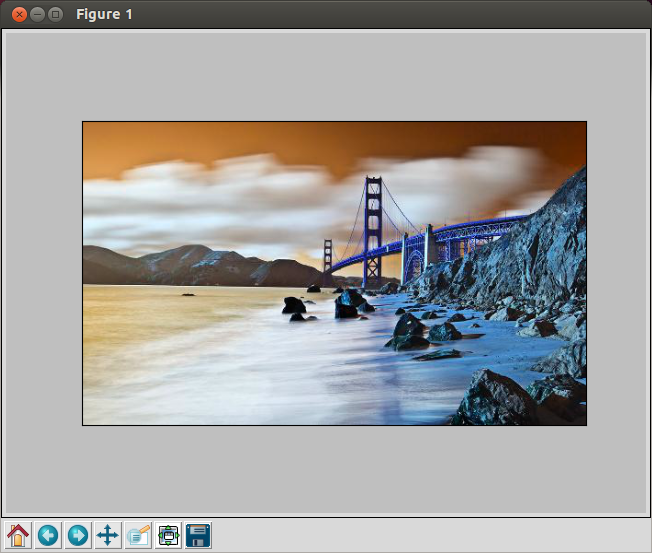
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization