Coding Questions IX - 2024
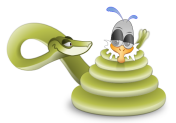
Given a square matrix with dimensions N. Find the determinant using NumPy module's linear algebra calculations.
Note: round the answer to 2 places after the decimal.Input Format: The first line contains the integer which is N. The next lines contains the space separated elements of array.
2 1.1 1.1 1.1 1.1
Here is the code:
import numpy N = input() print(f"N = {N}") a = [] for _ in range(int(N)): row = list(map(float, input().split())) print(f"row = {row}") a.append(row) det = numpy.linalg.det(a) print(round(det,2))
Output:
0.11
What is the revenue from the sale of shoes based on the given inventory (shoe_size list) and demand (demand tuple, where each element represents a pair of shoe size and corresponding price)?
shoe_size = [2, 3, 4, 5, 6, 8, 7, 6, 5, 18] demand = ((6, 55), (6, 45), (6, 55), (4, 40), (8, 60), (10, 50)) # (shoe_size, price)
Code:
from collections import Counter shoe_size = [2, 3, 4, 5, 6, 8, 7, 6, 5, 18] demand = ((6, 55), (6, 45), (6, 55), (4, 40), (8, 60), (10, 50)) # (shoe_size, price) shoe_dict = {k:v for k,v in Counter(shoe_size).items()} # (shoe_size, shoe_count) print(f"shoes_dict = {shoe_dict}") revenue = 0 for d in demand: size, price = d if size in shoe_dict.keys() and shoe_dict[size] > 0: revenue += price shoe_dict[size] -= 1 print(f"revenue = {revenue}")
Output:
shoes_dict = {2: 1, 3: 1, 4: 1, 5: 2, 6: 2, 8: 1, 7: 1, 18: 1} revenue = 200
Write a code defines a function rangoli
that takes an integer N as input
and prints a rangoli pattern of size N. The rangoli is created using lowercase alphabet characters.
The output will be symmetric and resemble a traditional rangoli pattern.
import string def rangoli(size): alphabet = string.ascii_lowercase lines = [] i = 0 print(alphabet[size-1:i:-1]) for i in range(size): # Create the left half of the line left_half = "-".join(alphabet[size-1:i:-1] + alphabet[i:size]) # Center the left half and duplicate it to create the full line full_line = (left_half.center(size * 4 - 3, "-")) # Append the line to the list lines.append(full_line) print(f"lines = {lines}") for line in lines[::-1]: print(line) for line in lines[1:size]: print(line) if __name__ == '__main__': n = int(input()) rangoli(n)
Output for an input of 5:
lines = ['e-d-c-b-a-b-c-d-e', '--e-d-c-b-c-d-e--', '----e-d-c-d-e----', '------e-d-e------', '--------e--------'] --------e-------- ------e-d-e------ ----e-d-c-d-e---- --e-d-c-b-c-d-e-- e-d-c-b-a-b-c-d-e --e-d-c-b-c-d-e-- ----e-d-c-d-e---- ------e-d-e------ --------e--------
Write a function unique_chars() that takes two parameters, a string s and an integer k. The function divides the input string s into k groups and then iterates through each group, removing consecutive repeating characters by keeping only the first occurrence.
def unique_chars(s, k): # divide s by k groups count = 0 groups = [] st = '' for i in range(len(s)): st += s[i] count += 1 if count % k == 0: groups.append(st) count = 0 st = '' print(f"groups = {groups}") # Iterate through each group, removing consecutive repeating characters by keeping only the first occurrence for g in groups: prev = g[0] st = prev for i in range(1,len(g)): if g[i] != prev: prev = g[i] st += g[i] print(f"{st}", end=" ") print() if __name__ == '__main__': st = 'AABCAAADA' k = 3 t = (st,k) unique_chars(*t) # AB, CA, AD st = 'AAABCADDEA' k = 3 t = (st,k) unique_chars(*t) # A, BCA, DE st = 'AAABCAFGHIKKNNHUKLOO' k = 5 t = (st, k) unique_chars(*t) # ABC, AFGHI, KNH, UKLO
Note that the first part of the code divides the input string s into k groups. The second part processes each group, creating a modified string st where consecutive repeating characters are removed by keeping only the first occurrence. The output includes the divided groups and the modified strings for each group.
Output:
groups = ['AAB', 'CAA', 'ADA'] AB CA ADA groups = ['AAA', 'BCA', 'DDE'] A BCA DE groups = ['AAABC', 'AFGHI', 'KKNNH', 'UKLOO'] ABC AFGHI KNH UKLO
''' UID must contain at least 2 uppercase. UID must contain at least 3 digits. UID should only contain alphanumeric. No character of UID should repeat. There must be exactly 10 characters in a valid UID. ''' def isValid(s): upper = 0 digits = 0 alphanumeric = 1 if len(s) != 10: return False, f"len > 10" for c in s: if c.isupper(): upper += 1 elif c.isdigit(): digits += 1 else: return False, f"non-alphanumeric" if s.count(c) > 1: return False, f"repeated chars: {c}" if digits < 3: return False, f"digits count = {digits}" if upper < 2: return False, f"upper count = {upper}" return True if __name__ == '__main__': s = "B1CD102354" v = isValid(s) # invalid (False) print(s,v) s = "B1CDEF2354" v = isValid(s) # valid (True) print(s,v) s = "BQWAILU471" v = isValid(s) # valid print(s,v) s = "115DEF2354" v = isValid(s) # invalid print(s,v) s = "74jK6yO6Ee" v = isValid(s) # invalid print(s,v) s = "6LfK3X35w4" v =isValid(s) # invalid print(s,v)
Output:
B1CD102354 (False, 'repeated chars: 1') B1CDEF2354 True BQWAILU471 True 115DEF2354 (False, 'repeated chars: 1') 74jK6yO6Ee (False, 'non-alphanumeric') 6LfK3X35w4 (False, 'non-alphanumeric')
Given a string, write a code that prints out the permutations of size k using itertools.permutations(iterable[, r])
.
from itertools import permutations input_string, k = input().split() k = int(k) sorted_input_string = ''.join(sorted(input_string)) print(f"sorted_input_string = {sorted_input_string}") print(f"len(sorted_input_string) = {len(sorted_input_string)}") p = list(permutations(sorted_input_string,k)) print(f"p = {p}") result = [''.join(x) for x in p] print(f"result = {result}")
Output:
hack 2 sorted_input_string = achk len(sorted_input_string) = 4 p = [('a', 'c'), ('a', 'h'), ('a', 'k'), ('c', 'a'), ('c', 'h'), ('c', 'k'), ('h', 'a'), ('h', 'c'), ('h', 'k'), ('k', 'a'), ('k', 'c'), ('k', 'h')] result = ['ac', 'ah', 'ak', 'ca', 'ch', 'ck', 'ha', 'hc', 'hk', 'ka', 'kc', 'kh']
We have a student list, students = [['Harry', 3.4], ['Sally', 3.7], ['Kelly', 3.41], ['Tommy', 3.41], ['July', 3.9]]. We need to find who has the 2nd lowest score.
students = [['Harry', 3.4], ['Sally', 3.7], ['Kelly', 3.41], ['Tommy', 3.41], ['July', 3.9]] scores = [x[1] for x in students] sorted_scores = sorted(set(scores)) # Loop through students and get the students who have the 2nd lowest score (sorted_scores[1]) answer = [s[0] for s in students if s[1] == sorted_scores[1]] print(f"answer = {answer}")
Output:
answer = ['Kelly', 'Tommy']
OrderedDict is a dictionary subclass in Python's collections module that maintains the order of the keys based on their insertion order. This means that when we iterate over the keys of an OrderedDict, they will be in the order in which they were added.
We want to modify the code so that if an item is added to the dictionary and it already exists, the new value should be added to the existing value, we can use the defaultdict from the collections module. The defaultdict allows we to set a default value type for new keys, and we can use it to initialize the values as float with a default of 0.0
from collections import defaultdict, OrderedDict my_dict = defaultdict(float) my_dict['Banana'] += 1.5 my_dict['Pomegranate'] += 13.5 my_dict['Banana'] += 0.99 my_dict['Apple'] += 3.2 my_dict['Blueberry'] += 3.2 my_dict['Apple'] += 2.8 my_dict['Apple'] += 5.8 print(f"my_dict = {my_dict}") ordered_dict = OrderedDict(my_dict) for k,v in ordered_dict.items(): print(k,v)
Output:
my_dict = defaultdict(<class 'float'>, {'Banana': 2.49, 'Pomegranate': 13.5, 'Apple': 11.8, 'Blueberry': 3.2}) Banana 2.49 Pomegranate 3.5 Apple 11.8 Blueberry 3.2
We want to count consecutive occurrences of a character in the input string and print the count along with the character.
# 1222311 => [(1, 1) (3, 2) (1, 3) (2, 1)] # [(count, digit), ....] def consecutive_digit(s): result = [] count = 1 for i in range(1,len(s)): if s[i] == s[i-1]: count += 1 else: result.append((count, int(s[i-1]))) count = 1 result.append((count, int(s[-1]))) return result if __name__ == '__main__': s = "1222311" result = consecutive_digit(s) print(f"{s} => {result}") s = "9" result = consecutive_digit(s) print(f"{s} => {result}")
Output:
1222311 => [(1, 1), (3, 2), (1, 3), (2, 1)] 9 => [(1, 9)]
Find a unique element in a collection where all other elements occur more than once:
from collections import Counter a = "1 2 3 6 5 4 4 2 5 3 6 1 6 5 3 2 4 1 2 5 1 4 3 6 8 4 3 1 5 6 2" b = a.split() d = dict(Counter(b)) print(f"d = {d}") for k,v in d.items(): if v == 1: print(k)
Output:
d = {'1': 5, '2': 5, '3': 5, '6': 5, '5': 5, '4': 5, '8': 1} 8
The array arr is a two-dimensional array or a matrix. It is represented as a list of lists. Each inner list represents a row of the matrix, and the elements within each inner list represent the columns.
Sort the array by each attribute.
from operator import itemgetter arr = [[10, 2, 5], [7, 1, 0], [9, 9, 9], [1, 23, 12], [6, 5, 9]] print(f"arr = {arr}") arr_0 = sorted(arr, key = lambda x:x[0]) print(f"arr_0 = {arr_0}") arr_1 = sorted(arr, key = itemgetter(1)) print(f"arr_1 = {arr_1}") arr_2 = sorted(arr, key = itemgetter(2)) print(f"arr_2 = {arr_2}")
Output:
arr = [[10, 2, 5], [7, 1, 0], [9, 9, 9], [1, 23, 12], [6, 5, 9]] arr_0 = [[1, 23, 12], [6, 5, 9], [7, 1, 0], [9, 9, 9], [10, 2, 5]] arr_1 = [[7, 1, 0], [10, 2, 5], [6, 5, 9], [9, 9, 9], [1, 23, 12]] arr_2 = [[7, 1, 0], [10, 2, 5], [9, 9, 9], [6, 5, 9], [1, 23, 12]]
s = "reverse me" s1 = s[::-1] print(f"s1 = {s1}") s2 = ''.join([s[len(s)-i-1] for i in range(len(s)) ]) print(f"s2 = {s2}") s3 = ''.join([s[i] for i in range(len(s)-1, -1, -1) ]) print(f"s3 = {s3}")
Output:
s1 = em esrever s2 = em esrever s3 = em esrever
Write a code to generate a list of 10 random odd numbers in 1-100 range using random.randrange()
:
import random n = 10 a = [] for i in range(10): a.append(random.randrange(1,100,2)) print(a)
In the random.randrange(start, stop, step)
function, the step parameter represents the step or interval between values.
Output:
[25, 73, 55, 93, 5, 11, 19, 49, 35, 17]
The difference between deepcopy and shallow copy lies in how they handle nested objects (objects within objects) when copying.
A shallow copy creates a new object, but instead of copying the elements of the original object, it copies references to the nested (child) objects. Therefore, changes made to nested objects inside the copy will affect the original object and vice versa.
shallow_copy.py:
import copy original_list = [1, [2, 3, 4], 5] shallow_copied_list = copy.copy(original_list) # Modify a nested list in the copy shallow_copied_list[1][0] = 'X' # Original list is also affected print("Original List:", original_list) # Output: [1, ['X', 3, 4], 5] print("Shallow Copied List:", shallow_copied_list) # Output: [1, ['X', 3, 4], 5]
A deep copy, on the other hand, creates a completely independent copy of the object and all its nested objects. Changes to the nested objects in the copy will not affect the original object and vice versa.
deep_copy.py:
import copy original_list = [1, [2, 3, 4], 5] deep_copied_list = copy.deepcopy(original_list) # Modify a nested list in the copy deep_copied_list[1][0] = 'Y' # Original list remains unchanged print("Original List:", original_list) # Output: [1, [2, 3, 4], 5] print("Deep Copied List:", deep_copied_list) # Output: [1, ['Y', 3, 4], 5]
In NumPy, we can use the numpy.transpose()
function or the T
attribute to transpose a matrix.
import numpy as np matrix = np.array( [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] ) transposed_1 = np.transpose(matrix) transposed_2 = matrix.T print(f"matrix = \n{matrix}") print(f"transposed_1 = \n{transposed_1}") print(f"transposed_2 = \n{transposed_2}")
Note that we need to convert a regular Python list to a NumPy array first.
Output:
matrix = [[1 2 3] [4 5 6] [7 8 9]] transposed_1 = [[1 4 7] [2 5 8] [3 6 9]] transposed_2 = [[1 4 7] [2 5 8] [3 6 9]]
Arguments are passed in python by reference. This means that any changes made within a function are reflected in the original object.
We want to check it using regex:
import re result = bool(re.match('[A-Za-z0-9]+$', 'A1a')) print(f"result = {result}") # True result = bool(re.match('[A-Za-z0-9]+$', 'A1a%')) print(f"result = {result}") # False
re.match('[A-Za-z0-9]+$': The addition of + requires the presence of at least one character, and the addition of $ requires that the entire string consists of one or more alphanumeric characters.
Of course we could have used isalnum()
:
result = 'A1a'.isalnum() print(f"result = {result}") # True result = 'A1a%'.isalnum() print(f"result = {result}") # False
I have a file, 'content.txt':
line 1 line 2 line 3
Write a code creating a new file, 'new_content.txt' with the lines reversed:
line 3 line 2 line 1
The code:
lines = [] with open('content.txt','r') as f: for line in f: lines.append(line.rstrip()) print(f"lines = {lines}") with open('new_content.txt','w') as f: for line in reversed(lines): f.write(line + '\n')
This is one of the simplest and most widely known encryption techniques (Caesar's cipher. It is based on a simple shift of each letter in a message by a certain number of positions down the given alphabet.
ALPHABET = "ABCDEFGHIJKLMNOPQRSTUVWXYZ" alphabet = "abcdefghijklmnopqrstuvwxyz" def encrypt(s, key): result = '' for char in s.lower(): idx = (alphabet.index(char) + key) % len(alphabet) result = result + alphabet[idx] return result def decrypt(s, key): result = '' for char in s.lower(): idx = (alphabet.index(char) - key) % len(alphabet) result = result + alphabet[idx] return result # Check the encryption function with the shift equals to 10 s = "bogotobogo" key = 1 print(f"s = {s}, key = {key}") encrypted = encrypt(s, key) print(f"encrypted = {encrypted}") decrypted = decrypt(encrypted,key) print(f"decrypted = {decrypted}")
Output:
s = bogotobogo, key = 1 encrypted = cphpupcphp decrypted = bogotobogo
"San FrancisCo's golDen Gate Bridge OFFERS breathTAKING viEWs of THE BAy" => "SAN francisco's GOLDEN gate BRIDGE offers BREATHTAKING views OF the BAY"
s_1 = "San FrancisCo's golDen Gate Bridge OFFERS breathTAKING viEWs of THE BAy" words_1 = s_1.split() words_2 = [] for i,w in enumerate(words_1): if i % 2 == 0: words_2.append(w.upper()) else: words_2.append(w.lower()) s_2 = ' '.join(words_2) print(s_2)
import re def isValidEmail(email): email_regex = r'^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-]' pattern = re.compile(email_regex) match = pattern.match(email) if match: return True else: return False if __name__ == '__main__': email_addresses = ["user_123@example-2.com", "john.doe@gmail.com", "invalid_email"] for email in email_addresses: print(f"{email}: {isValidEmail(email)}")
Note that by using r we ensure that backslashes in the pattern are treated as literal backslashes, preserving their intended meaning within the regular expression syntax.
user@example.com: True john.doe@gmail.com: True invalid_email: False
text = '''Create a regular expression matching a valid temperature represented either in Celsius or Fahrenheit scale (e.g. '+23.5 C', '-32 F', '0.0 C', '98.6 F') and to extract all the temperatures from the given string text. Positive temperatures can be with or without the + prefix (e.g. '212 F', '+212 F'). Negative temperatures must be prefixed with -. Zero temperature can be used with a prefix or without.''' import re # Define a pattern to search for valid temperatures in text temp_regex = r'[+-]?\d+(?:\.\d+)?\s[CF]' pattern = re.compile(temp_regex) # Print the temperatures out print(re.findall(pattern, text))
Output:
['+23.5 C', '-32 F', '0.0 C', '98.6 F', '212 F', '+212 F']
Note that the regex we used, temp_regex = r'[+-]?\d+(?:\.\d+)?\s[CF]' :
[+-]?
: Matches an optional plus or minus sign for positive, negative, or zero temperatures.\d+
: Matches one or more digits for the integer part of the temperature.(?:\.\d+)?
: Non-capturing group that matches an optional decimal part, consisting of a dot followed by one or more digits. The non-capturing group means it groups the dot and the digits but doesn't capture them separately. This also makes the decimal part optional. We may want to use(\.\d+)?
which is a capturing group that includes the dot and the digits after it, making it optional. This means that the decimal part is captured as a group. In practice, if we don't need to capture the decimal part separately, using a non-capturing group is often preferred for better performance. Therefore, both versions are valid, and we can choose the one that fits our specific needs. If we're only interested in the whole temperature, including the decimal part if present, we want to use non-capturing group. If we only need the integer part and don't want to capture the decimal part, we can use the capturing pattern.\s
: Matches a single whitespace character (space or tab) separating the value from the scale.[CF]
: Matches either a C or an F for the Celsius or Fahrenheit scale.
We have the following movie list, movies = ['Interstellar, 2014, Christopher Nolan', 'The Shawshank Redemption, 1994, Frank Darabont', 'Inception, 2010, Christopher Nolan']
We want to retrieve from each element of the list its name and the director using regex, re.
import re movies = ['Interstellar, 2014, Christopher Nolan', 'The Shawshank Redemption, 1994, Frank Darabont', 'Inception, 2010, Christopher Nolan'] # Compile a regular expression pattern = re.compile(r', \d+, ') movies_without_year = [] for movie in movies: # Retrieve a movie name and its director split_result = re.split(pattern, movie) # Create a new string with a movie name and its director movie_and_director = ', '.join(split_result) # Append the resulting string to movies_without_year movies_without_year.append(movie_and_director) for movie in movies_without_year: print(movie)
re.compile()
function compiles a regular expression pattern into a regex object, which can be used for matching operations
and the pattern r', \d+, '
is designed to match a comma followed by one or more digits, followed by another comma, with spaces around the commas.
Output:
Interstellar, Christopher Nolan The Shawshank Redemption, Frank Darabont Inception, Christopher Nolan
How can you merge the following two dictionaries: dict1 = {'a': 1, 'b': 2} dict2 = {'b': 3, 'c': 4}?
dict1 = {'a': 1, 'b': 2} dict2 = {'b': 3, 'c': 4} merged_dict = {**dict1, **dict2} print(f"merged_dict = {merged_dict}")
Output:
merged_dict = {'a': 1, 'b': 3, 'c': 4}
{**dict1}
unpacks the key-value pairs from dict1 into the new dictionary.
{**dict2}
unpacks the key-value pairs from dict2 into the same new dictionary.
As a result, we get a new dictionary (merged_dict) that contains the combined key-value pairs from both dict1 and dict2.
If there are any common keys between the dictionaries, the values from dict2 will overwrite the values from dict1.
In contrast to the previous section, where the value for the same key is set as the value of the later dictionary, in this case, we aim to add the values of two dictionaries if their keys are the same.
dict1 = {'a': 10, 'b': 20, 'c': 30} dict2 = {'b': 5, 'c': 15, 'd': 25} print(f"dict1 = {dict1}") print(f"dict2 = {dict2}") merged = {**dict1, **dict2} print(f"merged = {merged}") combined = {} for k,v in dict1.items(): combined[k] = combined.get(k,0) + v for k,v in dict2.items(): combined[k] = combined.get(k,0) + v print(f"combined = {combined}")
Note that we used dict.get()
method is used to safely access the value for a key.
If the key is not present in the dictionary, it returns the default value (0 in this case).
Output:
dict1 = {'a': 10, 'b': 20, 'c': 30} dict2 = {'b': 5, 'c': 15, 'd': 25} merged = {'a': 10, 'b': 5, 'c': 15, 'd': 25} combined = {'a': 10, 'b': 25, 'c': 45, 'd': 25}
Lists are suitable when we need random access to elements, when the entire sequence needs to be modified, or when we want to create a finite sequence of elements. Generator are suitable when you need to iterate over a potentially large or infinite sequence of elements without loading them all into memory. They are often used in scenarios where we only need to access elements sequentially and don't need the entire sequence at once.
mylist = [1,2,3,4,5] generator = (k**2 for k in mylist) for g in generator: print(g, end = ' ') while True: try: item = next(generator) # Get the next item print(item) except StopIteration: break # Exit the loop when there are no more items iterator = iter(mylist) for _ in mylist: print(next(iterator)**2, end = ' ')
Output:
1 4 9 16 25 1 4 9 16 25
def find_pairs_with_sum(arr, target): pairs = [] seen = set() for n in arr: find = target - n if find in seen: pairs.append((find,n)) seen.add(n) return pairs a = {1,2,3,4,5,6,7,8,9} target = 9 pairs = find_pairs_with_sum(a, target) print(f"pairs for target {target} are {pairs}")
The find_pairs_with_sum()
function takes an array arr
and a target sum target
.
It uses a set (seen
) to keep track of the numbers encountered while iterating through the array.
For each number in the array, it calculates the complement needed to reach the target sum.
If the complement is found in the set, a pair is added to the result.
The function returns a list of pairs whose sum is equal to the target value.
Output:
pairs for target 9 are [(4, 5), (3, 6), (2, 7), (1, 8)]
def add_without_plus(a, b): # The bitwise XOR (^) gives the sum without carry # The bitwise AND (&) with shifted bits gives the carry while b != 0: # Calculate the sum without carry sum_without_carry = a ^ b # Calculate the carry and shift it to the left carry = (a & b) << 1 # Update a with the sum without carry a = sum_without_carry # Update b with the carry b = carry return a def add_without_plus_binary(a,b): # Convert integers to binary strings bin_a = bin(a)[2:] # bin(a) = 0b1100 => bin(a)[2:] = 1100 bin_b = bin(b)[2:] # bin(b) = 0b101 => bin(b)[2:] = 101 nfill = len(bin_a) if len(bin_a) > len(bin_b) else len(bin_b) nfill += 1 # for a possible carry a_fill = bin_a.zfill(nfill) # 01100 b_fill = bin_b.zfill(nfill) # 00101 # Initialize the result string result = '' # Initialize the carry carry = 0 for bit_a, bit_b in zip(a_fill[::-1],b_fill[::-1]): sum_bit = int(bit_a) ^ int(bit_b) ^ carry carry = (int(bit_a) & int(bit_b)) | (carry & (int(bit_a) ^ int(bit_b))) result = str(sum_bit) + result #print(f"result = {result}") return int(result, 2) # Example usage: num1 = 12 num2 = 5 result1 = add_without_plus(num1, num2) print(f"add_without_plus(): the sum of {num1} and {num2} is {result1}") result2 = add_without_plus_binary(num1, num2) print(f"add_without_plus_binary(): the sum of {num1} and {num2} is {result2}")
Output:
add_without_plus(): the sum of 12 and 5 is 17 add_without_plus_binary(): the sum of 12 and 5 is 17
Both isinstance()
and type()
are used for type checking, but they have subtle differences in their behavior and use cases.
- type():
The
type()
function is used to get the type of an object. It returns the actual type of the object. Here's an example:a = 101 print(type(a)) # <class 'int'>
- isinstance():
The
isinstance()
function, on the other hand, is used to check whether an object is an instance of a particular class or a tuple of classes. It also considers inheritance, which means if the object is an instance of a subclass,isinstance()
will return True. Here's an example:class Animal: pass class Dog(Animal): pass a = Dog() # Using type print(type(a) == Animal) # Output: False # Using isinstance print(isinstance(a, Animal)) # Output: True
While type()
only checks the exact type of an object and does not consider inheritance,
isinstance()
checks both the exact type and any class in the inheritance hierarchy.
Use type()
when we need to get the exact type of an object.
Use isinstance()
when we need to check if an object is an instance of a specific class or any of its subclasses.
Decorators in Python are a powerful way to modify or extend the behavior of functions or methods.
Write an example demonstrates how decorators can be used to add functionality to a function, such as measuring execution time, without modifying the original function (called slow_function())'s code. Decorators provide a clean and reusable way to extend the behavior of functions in a modular fashion.
import time def my_decorator(fnc): def my_wrapper(*args, **kargs): start_time = time.time() fnc(*args, **kargs) end_time = time.time() execution_time = end_time - start_time print(f"{fnc.__name__} took {execution_time:.5f} seconds to execute.") return my_wrapper @my_decorator def slow_function(): # Simulating a time-consuming task time.sleep(2) print(f"slow_function() executed") slow_function()
- The
my_decorator
function takes another function (fnc
) as an argument. - It defines a new function
my_wrapper
that measures the time taken to execute the original function. - The original function (
fnc
) is called within themy_wrapper
function. - The
my_wrapper
function prints the execution time and returns the result.
Here's a breakdown of how it works:
- The
my_decorator
is applied to theslow_function
. - When
slow_function(2)
is called, the argument 2 is passed to the decorated function through the *args parameter in themy_wrapper
function. - The decorated function simulates a time-consuming task by sleeping for the specified duration (2 seconds in this case).
- The execution time is then measured and printed.
Output:
slow_function() executed slow_function took 2.00461 seconds to execute.
Explain it with my_list = [0, 10, 20, 30, 40, 50, 60, 70, 80, 90, 100].
my_list = [0, 10, 20, 30, 40, 50, 60, 70, 80, 90, 100] print(my_list[-3:2:-2])
Output:
[80, 60, 40]
my_list[-3:2:-2]: This syntax combines a negative start index, a negative step, and stop index of 2:
- -3 (start index): starts at the third element from the end (index 8, which is 80).
- :2: (stop index): includes elements down to the index 2 (element 20) of the list from the end.
- -2 (step): moves backward with a step of 2, selecting every other element.
# file : char_freq_sample.txt ''' The origins of life cannot be dated as precisely, but there is evidence that bacteria-like organisms lived on Earth 3.5 billion years ago, and they may have existed even earlier, when the first solid crust formed, almost 4 billion years ago. These early organisms must have been simpler than the organisms living today. ''' import collections import operator # Using collections.Counter() with open('char_freq_sample.txt','r') as f: count_dict = dict(collections.Counter(f.read())) sorted_count_dict = sorted(count_dict.items(), key = lambda x: x[1], reverse = True) print(f"#1 sorted_count_dict = {sorted_count_dict}") # Using string.count() d = collections.defaultdict(int) with open('char_freq_sample.txt','r') as f: string = f.read() for c in string: count_dict[c] = string.count(c) count_dict = dict(count_dict) sorted_count_dict = sorted(count_dict.items(), key = operator.itemgetter(1), reverse = True) print(f"#2 sorted_count_dict = {sorted_count_dict}") # Not using collections.Counter() nor string.count() d = collections.defaultdict(int) with open('char_freq_sample.txt','r') as f: string = f.read() for c in string: d[c] += 1 sorted_count_dict = sorted(count_dict.items(), key = operator.itemgetter(1), reverse = True) print(f"#3 sorted_count_dict = {sorted_count_dict}")
Output:
#1 sorted_count_dict = [(' ', 52), ('e', 35), ('i', 23), ('a', 23), ('s', 20), ('t', 18), ('r', 17), ('n', 16), ('o', 15), ('l', 14), ('h', 12), ('d', 9), ('m', 8), ('g', 7), ('y', 7), ('b', 6), ('v', 6), ('c', 5), ('f', 4), (',', 4), ('\n', 4), ('u', 3), ('.', 3), ('T', 2), ('p', 2), ('-', 1), ('k', 1), ('E', 1), ('3', 1), ('5', 1), ('x', 1), ('w', 1), ('4', 1)] #2 sorted_count_dict = [(' ', 52), ('e', 35), ('i', 23), ('a', 23), ('s', 20), ('t', 18), ('r', 17), ('n', 16), ('o', 15), ('l', 14), ('h', 12), ('d', 9), ('m', 8), ('g', 7), ('y', 7), ('b', 6), ('v', 6), ('c', 5), ('f', 4), (',', 4), ('\n', 4), ('u', 3), ('.', 3), ('T', 2), ('p', 2), ('-', 1), ('k', 1), ('E', 1), ('3', 1), ('5', 1), ('x', 1), ('w', 1), ('4', 1)] #3 sorted_count_dict = [(' ', 52), ('e', 35), ('i', 23), ('a', 23), ('s', 20), ('t', 18), ('r', 17), ('n', 16), ('o', 15), ('l', 14), ('h', 12), ('d', 9), ('m', 8), ('g', 7), ('y', 7), ('b', 6), ('v', 6), ('c', 5), ('f', 4), (',', 4), ('\n', 4), ('u', 3), ('.', 3), ('T', 2), ('p', 2), ('-', 1), ('k', 1), ('E', 1), ('3', 1), ('5', 1), ('x', 1), ('w', 1), ('4', 1)]
Write a code defines a function transform_date_format(date)
that takes a date in the format "YYYY-MM-DD"
and transforms it into the format "DD-MM-YYYY" using the re.sub()
function from the re
module
to perform a regular expression-based substitution.
import re def transform_date_format(date): '''takes a date in the format "YYYY-MM-DD" and transforms it into the format "DD-MM-YYYY." ''' return re.sub(r'(\d{4})-(\d{1,2})-(\d{1,2})', '\\3-\\2-\\1', date) date = "2024-02-13" new_date = transform_date_format(date) print(f" {date} => {new_date}")
Output:
2024-02-13 => 13-02-2024
Replace the newlines in csv file with tabs.
Given a CSV file where each row contains the name of a city and its state separated by a comma, we want to replace the newlines in the file with tabs as demonstrated in the following sample:
Input: Albany, N.Y. Albuquerque, N.M. Anchorage, Alaska Output: Albany, N.Y. Albuquerque, N.M. Anchorage, Alaska
Code:
import csv import os filename = "city.csv" new_filename = "city_tab_separated.csv" # Name of the new file with tab-separated lines # Check if the file exists if os.path.exists(filename): # print("File exists") data = [] # Read the original CSV file with open(filename, 'r') as csvfile: csvreader = csv.reader(csvfile) for row in csvreader: data.append(row) # Convert the data to tab-separated lines tab_separated_data = "\t".join([",".join(row) for row in data]) # Write the tab-separated data to a new file with open(new_filename, 'w') as new_file: new_file.write(tab_separated_data) print(f"Data has been written to {new_filename} with tab-separated lines.") else: print("File does not exist")
Input, city.csv:
Albany, N.Y. Albuquerque, N.M. Anchorage, Alaska
Output, city_tab_separated.csv:
Albany, N.Y. Albuquerque, N.M. Anchorage, Alaska
import pandas as pd data_2022 = {'Product': ['A', 'B', 'C'], 'Sales_2022': [100, 150, 200]} data_2023 = {'Product': ['A', 'B', 'D'], 'Sales_2023': [120, 180, 250]} # Convert dictionaries to Pandas DataFrames df_2022 = pd.DataFrame(data_2022) df_2023 = pd.DataFrame(data_2023) print(f"df_2022 = \n{df_2022}") print(f"df_2023 = \n{df_2023}") # Merge DataFrames on the 'Product' column merged_df = pd.merge(df_2022, df_2023, on='Product', how='outer') print(f"merged_df = \n{merged_df}") # Fill NaN values with 0 merged_df = merged_df.fillna(0) print(f"merged_df = \n{merged_df}") # Add a new column for total sales merged_df['Total_Sales'] = merged_df['Sales_2022'] + merged_df['Sales_2023'] print(f"merged_df = \n{merged_df}")
The pd.merge()
function in the Pandas
library is used to merge two DataFrames
based on a common column or index.
Here are the main arguments for the pd.merge()
:
pd.merge(left, right, how='inner', on=None, left_on=None, right_on=None, left_index=False, right_index=False, suffixes=('_x', '_y'), sort=False)
- left: The left DataFrame to be merged.
- right: The right DataFrame to be merged.
- how: The type of merge to be performed. It can take values like 'left', 'right', 'outer', and 'inner'. The default is 'inner'.
- on: The column or index on which to merge the DataFrames. This can be a column name or a list of column names if the keys are different in the left and right DataFrames.
- left_on: Columns from the left DataFrame to use as keys for merging.
- right_on: Columns from the right DataFrame to use as keys for merging.
- left_index: If True, use the index of the left DataFrame as the merge key(s).
- right_index: If True, use the index of the right DataFrame as the merge key(s).
- suffixes: A tuple of suffixes to apply to overlapping column names, to distinguish them in the result DataFrame. The default is ('_x', '_y').
- sort: Whether to sort the result DataFrame by the merge keys. The default is False.
Output:
df_2022 = Product Sales_2022 0 A 100 1 B 150 2 C 200 df_2023 = Product Sales_2023 0 A 120 1 B 180 2 D 250 merged_df = Product Sales_2022 Sales_2023 0 A 100.0 120.0 1 B 150.0 180.0 2 C 200.0 NaN 3 D NaN 250.0 merged_df = Product Sales_2022 Sales_2023 0 A 100.0 120.0 1 B 150.0 180.0 2 C 200.0 0.0 3 D 0.0 250.0 merged_df = Product Sales_2022 Sales_2023 Total_Sales 0 A 100.0 120.0 220.0 1 B 150.0 180.0 330.0 2 C 200.0 0.0 200.0 3 D 0.0 250.0 250.0
my_strings = ['BBABB', 'CCAABBCC', 'DDAAABBCCCDD'] print(f"my_strings = {my_strings}") for string in my_strings: print() # method 1 new_str_1 = "".join(dict.fromkeys(string)) print(f"new_str_1 = {new_str_1}") # method 2 seen = set() new_chars = [] for c in string: if c not in seen: new_chars.append(c) seen.add(c) new_str_2 = "".join(new_chars) print(f"new_str_2 = {new_str_2}")
Output:
my_strings = ['BBABB', 'CCAABBCC', 'DDAAABBCCCDD'] new_str_1 = BA new_str_2 = BA new_str_1 = CAB new_str_2 = CAB new_str_1 = DABC new_str_2 = DABC
class ComplexNumber: def __init__(self, real, img): self._real = real self._img = img def __str__(self): return f"{self._real} + {self._img}i" def __abs__(self): return (self._real**2 + self._img**2)**0.5 def __add__(self, other): return ComplexNumber(self._real + other._real, self._img + other._img) def __sub__(self, other): return ComplexNumber(self._real - other._real, self._img - other._img) def __mul__(self, other): return ComplexNumber(self._real * other._real - self._img * other._img, self._real * other._img + self._img * other._real ) def __truediv__(self, other): denom = other._real**2 + other._img**2 return ComplexNumber((self._real * other._real + self._img * other._img) / denom, (self._img * other._real - self._real * other._img) / denom ) def conjugate(self): return ComplexNumber(self._real, - self._img) # Example usage: c1 = ComplexNumber(3, 2) c2 = ComplexNumber(1, 7) print(f"c1 = {c1}") print(f"c2 = {c2}") print(f"Addition: {c1 + c2}") print(f"Subtraction: {c1 - c2}") print(f"Multiplication: {c1 * c2}") print(f"Division: {c1 / c2}") print(f"Conjugate of c1: {c1.conjugate()}") print(f"Absolute value of c1: {abs(c1)}")
Output:
c1 = 3 + 2i c2 = 1 + 7i Addition: 4 + 9i Subtraction: 2 + -5i Multiplication: -11 + 23i Division: 0.34 + -0.38i Conjugate of c1: 3 + -2i Absolute value of c1: 3.605551275463989
Note that when we write c1 + c2, Python interprets this as c1.__add__(c2). Here's how it works:
- c1 is the object on the left side of the + operator, so it becomes the self argument in the __add__ method.
- c2 is the object on the right side of the + operator, so it becomes the other argument in the __add__ method.
import collections words = ['bcdef','abcdefg','bcde','bcdef'] words_dict = dict.fromkeys(words,0) for w in words: words_dict[w] += 1 print(f"words_dict = {words_dict}") words_dict_2 = collections.defaultdict(int) for w in words: words_dict_2[w] += 1 print(f"words_dict_2= {dict(words_dict_2)}") words_dict_3 = dict(collections.Counter(words)) print(f"words_dict_3= {words_dict_3}")
Output:
words_dict = {'bcdef': 2, 'abcdefg': 1, 'bcde': 1} words_dict_2= {'bcdef': 2, 'abcdefg': 1, 'bcde': 1} words_dict_3= {'bcdef': 2, 'abcdefg': 1, 'bcde': 1}
- Python Coding Questions I
- Python Coding Questions II
- Python Coding Questions III
- Python Coding Questions IV
- Python Coding Questions V
- Python Coding Questions VI
- Python Coding Questions VII
- Python Coding Questions VIII
- Python Coding Questions IX
- Python Coding Questions X
List of codes Q & A
- Merging two sorted list
- Get word frequency - initializing dictionary
- Initializing dictionary with list
- map, filter, and reduce
- Write a function f() - yield
- What is __init__.py?
- Build a string with the numbers from 0 to 100, "0123456789101112..."
- Basic file processing: Printing contents of a file - "with open"
- How can we get home directory using '~' in Python?
- The usage of os.path.dirname() & os.path.basename() - os.path
- Default Libraries
- range vs xrange
- Iterators
- Generators
- Manipulating functions as first-class objects
- docstrings vs comments
- using lambdda
- classmethod vs staticmethod
- Making a list with unique element from a list with duplicate elements
- What is map?
- What is filter and reduce?
- *args and **kwargs
- mutable vs immutable
- Difference between remove, del and pop on lists
- Join with new line
- Hamming distance
- Floor operation on integers
- Fetching every other item in the list
- Python type() - function
- Dictionary Comprehension
- Sum
- Truncating division
- Python 2 vs Python 3
- len(set)
- Print a list of file in a directory
- Count occurrence of a character in a Python string
- Make a prime number list from (1,100)
- Reversing a string - Recursive
- Reversing a string - Iterative
- Reverse a number
- Output?
- Merging overlapped range
- Conditional expressions (ternary operator)
- Packing Unpacking
- Function args
- Unpacking args
- Finding the 1st revision with a bug
- Which one has higher precedence in Python? - NOT, AND , OR
- Decorator(@) - with dollar sign($)
- Multi-line coding
- Recursive binary search
- Iterative binary search
- Pass by reference
- Simple calculator
- iterator class that returns network interfaces
- Converting domain to ip
- How to count the number of instances
- Python profilers - cProfile
- Calling a base class method from a child class that overrides it
- How do we find the current module name?
- Why did changing list 'newL' also change list 'L'?
- Constructing dictionary - {key:[]}
- Colon separated sequence
- Converting binary to integer
- 9+99+999+9999+...
- Calculating balance
- Regular expression - findall
- Chickens and pigs
- Highest possible product
- Implement a queue with a limited size
- Copy an object
- Filter
- Products
- Pickle
- Overlapped Rectangles
- __dict__
- Fibonacci I - iterative, recursive, and via generator
- Fibonacci II - which method?
- Fibonacci III - find last two digits of Nth Fibonacci number
- Write a Stack class returning Max item at const time A
- Write a Stack class returning Max item at const time B
- Finding duplicate integers from a list - 1
- Finding duplicate integers from a list - 2
- Finding duplicate integers from a list - 3
- Reversing words 1
- Parenthesis, a lot of them
- Palindrome / Permutations
- Constructing new string after removing white spaces
- Removing duplicate list items
- Dictionary exercise
- printing numbers in Z-shape
- Factorial
- lambda
- lambda with map/filter/reduce
- Number of integer pairs whose difference is K
- iterator vs generator
- Recursive printing files in a given directory
- Bubble sort
- What is GIL (Global Interpreter Lock)?
- Word count using collections
- Pig Latin
- List of anagrams from a list of words
- lamda with map, filer and reduce functions
- Write a code sending an email using gmail
- histogram 1 : the frequency of characters
- histogram 2 : the frequency of ip-address
- Creating a dictionary using tuples
- Getting the index from a list
- Looping through two lists side by side
- Dictionary sort with two keys : primary / secondary keys
- Writing a file downloaded from the web
- Sorting csv data
- Reading json file
- Sorting class objects
- Parsing Brackets
- Printing full path
- str() vs repr()
- Missing integer from a sequence
- Polymorphism
- Product of every integer except the integer at that index
- What are accessors, mutators, and @property?
- N-th to last element in a linked list
- Implementing linked list
- Removing duplicate element from a list
- List comprehension
- .py vs .pyc
- Binary Tree
- Print 'c' N-times without a loop
- Quicksort
- Dictionary of list
- Creating r x c matrix
- Transpose of a matrix
- str.isalpha() & str.isdigit()
- Regular expression
- What is Hashable? Immutable?
- Convert a list to a string
- Convert a list to a dictionary
- List - append vs extend vs concatenate
- Use sorted(list) to keep the original list
- list.count()
- zip(list,list) - join elements of two lists
- zip(list,list) - weighted average with two lists
- Intersection of two lists
- Dictionary sort by value
- Counting the number of characters of a file as One-Liner
- Find Armstrong numbers from 100-999
- Find GCF (Greatest common divisor)
- Find LCM (Least common multiple)
- Draws 5 cards from a shuffled deck
- Dictionary order by value or by key
- Regular expression - re.split()
- Regular expression : re.match() vs. re.search()
- Regular expression : re.match() - password check
- Regular expression : re.search() - group capturing
- Regular expression : re.findall() - group capturin
- Prime factors : n = products of prime numbers
- Valid IPv4 address
- Sum of strings
- List rotation - left/right
- shallow/deep copy
- Converting integer to binary number
- Creating a directory and a file
- Creating a file if not exists
- Invoking a python file from another
- Sorting IP addresses
- Word Frequency
- Printing spiral pattern from a 2D array - I. Clock-wise
- Printing spiral pattern from a 2D array - II. Counter-Clock-wise
- Find a minimum integer not in the input list
- I. Find longest sequence of zeros in binary representation of an integer
- II. Find longest sequence of zeros in binary representation of an integer - should be surrounded with 1
- Find a missing element from a list of integers
- Find an unpaired element from a list of integers
- Prefix sum : Passing cars
- Prefix sum : count the number of integers divisible by k in range [A,B]
- Can make a triangle?
- Dominant element of a list
- Minimum perimeter
- MinAbsSumOfTwo
- Ceiling - Jump Frog
- Brackets - Nested parentheses
- Brackets - Nested parentheses of multiple types
- Left rotation - list shift
- MaxProfit
- Stack - Fish
- Stack - Stonewall
- Factors or Divisors
- String replace in files 1
- String replace in files 2
- Using list as the default_factory for defaultdict
- Leap year
- Capitalize
- Log Parsing
- Getting status_code for a site
- 2D-Array - Max hourglass sum
- New Year Chaos - list
- List (array) manipulation - list
- Hash Tables: Ransom Note
- Count Triplets with geometric progression
- Strings: Check if two strings are anagrams
- Strings: Making Anagrams
- Strings: Alternating Characters
- Special (substring) Palindrome
- String with the same frequency of characters
- Common Child
- Fraudulent Activity Notifications
- Maximum number of toys
- Min Max Riddle
- Poisonous Plants with Pesticides
- Common elements of 2 lists - Complexity
- Get execution time using decorator(@)
- Conver a string to lower case and split using decorator(@)
- Python assignment and memory location
- shallow copy vs deep copy for compound objects (such as a list)
- Generator with Fibonacci
- Iterator with list
- Second smallest element of a list
- *args, **kargs, and positional args
- Write a function, fn('x','y',3) that returns ['x1', 'y1', 'x2', 'y2', 'x3', 'y3']
- sublist or not
- any(), all()
- Flattening a list
- Select an element from a list
- Circularly identical lists
- Difference between two lists
- Reverse a list
- Split a list with a step
- Break a list and make chunks of size n
- Remove duplicate consecutive elements from a list
- Combination of elements from two lists
- Adding a sublist
- Replace the first occurence of a value
- Sort the values of the first list using the second list
- Transpose of a matrix (nested list)
- Binary Gap
- Powerset
- Round Robin
- Fixed-length chunks or blocks
- Accumulate
- Dropwhile
- Groupby
- Simple product
- Simple permutation
- starmap(fn, iterable)
- zip_longest(*iterables, fillvalue=None)
- What is the correct way to write a doctest?
- enumerate(iterable, start=0)
- collections.defaultdict - grouping a sequence of key-value pairs into a dictionary of lists
- What is the purpose of the 'self' keyword when defining or calling instance methods?
- collections.namedtuple(typename, field_names, *, rename=False, defaults=None, module=None)
- zipped
- What is key difference between a set and a list?
- What does a class's init() method do?
- Class methods
- Permutations and combinations of ['A','B','C']
- Sort list of dictionaries by values
- Return a list of unique words
- hashlib
- encode('utf-8')
- Reading in CSV file
- Count capital letters in a file
- is vs ==
- Create a matrix : [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
- Binary to integer and check if it's the power of 2
- urllib.request.urlopen() and requests
- Game statistics
- Chess - pawn race
- Decoding a string
- Determinant of a matrix - using numpy.linalg.det()
- Revenue from shoe sales - using collections.Counter()
- Rangoli
- Unique characters
- Valid UID
- Permutations of a string in lexicographic sorted order
- Nested list
- Consecutive digit count
- Find a number that occurs only once
- Sorting a two-dimensional array
- Reverse a string
- Generate random odd numbers in a range
- Shallow vs Deep copy
- Transpose matrix
- Are Arguments in Python Passed by Value or by Reference?
- re: Is a string alphanumeric?
- reversed()
- Caesar's cipher, or shift cipher, Caesar's code, or Caesar shift
- Every other words
- re: How can we check if an email address is valid or not?
- re: How to capture temperatures of a text
- re.split(): How to split a text.
- How can we merge two dictionaries?
- How can we combine two dictionaries?
- What is the difference between a generator and a list?
- Pairs of a given array A whose sum value is equal to a target value N
- Adding two integers without plus
- isinstance() vs type()
- What is a decorator?
- In Python slicing, what does my_list[-3:2:-2] slice do?
- Revisit sorting dict - counting chars in a text file
- re: Transforming a date format using re.sub
- How to replace the newlines in csv file with tabs?
- pandas.merge
- How to remove duplicate charaters from a string?
- Implement a class called ComplexNumber
- Find a word frequency
- Get the top 3 most frequent characters of a string
- Just seen and ever seen
- Capitalizing the full name
- Counting Consequitive Characters
- Calculate Product of a List of Integers Provided using input()
- How many times a substring appears in a string
- Hello, first_name last_name
- String validators
- Finding indices that a char occurs in a list
- itertools combinations
Python tutorial
Python Home
Introduction
Running Python Programs (os, sys, import)
Modules and IDLE (Import, Reload, exec)
Object Types - Numbers, Strings, and None
Strings - Escape Sequence, Raw String, and Slicing
Strings - Methods
Formatting Strings - expressions and method calls
Files and os.path
Traversing directories recursively
Subprocess Module
Regular Expressions with Python
Regular Expressions Cheat Sheet
Object Types - Lists
Object Types - Dictionaries and Tuples
Functions def, *args, **kargs
Functions lambda
Built-in Functions
map, filter, and reduce
Decorators
List Comprehension
Sets (union/intersection) and itertools - Jaccard coefficient and shingling to check plagiarism
Hashing (Hash tables and hashlib)
Dictionary Comprehension with zip
The yield keyword
Generator Functions and Expressions
generator.send() method
Iterators
Classes and Instances (__init__, __call__, etc.)
if__name__ == '__main__'
argparse
Exceptions
@static method vs class method
Private attributes and private methods
bits, bytes, bitstring, and constBitStream
json.dump(s) and json.load(s)
Python Object Serialization - pickle and json
Python Object Serialization - yaml and json
Priority queue and heap queue data structure
Graph data structure
Dijkstra's shortest path algorithm
Prim's spanning tree algorithm
Closure
Functional programming in Python
Remote running a local file using ssh
SQLite 3 - A. Connecting to DB, create/drop table, and insert data into a table
SQLite 3 - B. Selecting, updating and deleting data
MongoDB with PyMongo I - Installing MongoDB ...
Python HTTP Web Services - urllib, httplib2
Web scraping with Selenium for checking domain availability
REST API : Http Requests for Humans with Flask
Blog app with Tornado
Multithreading ...
Python Network Programming I - Basic Server / Client : A Basics
Python Network Programming I - Basic Server / Client : B File Transfer
Python Network Programming II - Chat Server / Client
Python Network Programming III - Echo Server using socketserver network framework
Python Network Programming IV - Asynchronous Request Handling : ThreadingMixIn and ForkingMixIn
Python Coding Questions I
Python Coding Questions II
Python Coding Questions III
Python Coding Questions IV
Python Coding Questions V
Python Coding Questions VI
Python Coding Questions VII
Python Coding Questions VIII
Python Coding Questions IX
Python Coding Questions X
Image processing with Python image library Pillow
Python and C++ with SIP
PyDev with Eclipse
Matplotlib
Redis with Python
NumPy array basics A
NumPy Matrix and Linear Algebra
Pandas with NumPy and Matplotlib
Celluar Automata
Batch gradient descent algorithm
Longest Common Substring Algorithm
Python Unit Test - TDD using unittest.TestCase class
Simple tool - Google page ranking by keywords
Google App Hello World
Google App webapp2 and WSGI
Uploading Google App Hello World
Python 2 vs Python 3
virtualenv and virtualenvwrapper
Uploading a big file to AWS S3 using boto module
Scheduled stopping and starting an AWS instance
Cloudera CDH5 - Scheduled stopping and starting services
Removing Cloud Files - Rackspace API with curl and subprocess
Checking if a process is running/hanging and stop/run a scheduled task on Windows
Apache Spark 1.3 with PySpark (Spark Python API) Shell
Apache Spark 1.2 Streaming
bottle 0.12.7 - Fast and simple WSGI-micro framework for small web-applications ...
Flask app with Apache WSGI on Ubuntu14/CentOS7 ...
Fabric - streamlining the use of SSH for application deployment
Ansible Quick Preview - Setting up web servers with Nginx, configure enviroments, and deploy an App
Neural Networks with backpropagation for XOR using one hidden layer
NLP - NLTK (Natural Language Toolkit) ...
RabbitMQ(Message broker server) and Celery(Task queue) ...
OpenCV3 and Matplotlib ...
Simple tool - Concatenating slides using FFmpeg ...
iPython - Signal Processing with NumPy
iPython and Jupyter - Install Jupyter, iPython Notebook, drawing with Matplotlib, and publishing it to Github
iPython and Jupyter Notebook with Embedded D3.js
Downloading YouTube videos using youtube-dl embedded with Python
Machine Learning : scikit-learn ...
Django 1.6/1.8 Web Framework ...
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization