Formatting Strings
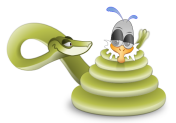
Two flavors of string formatting:
- string formatting expressions: this is based on the C type printf.
- string formatting method calls: this is newer and added since Python 2.6.
The % provides a compact way to code several string substitutions all at once.
>>> 'I am using %s %d.%d' %('Python', 3, 2) 'I am using Python 3.2'
Code | Meaning |
---|---|
b | Binary format. Outputs the number in base 2. |
c | Character. Converts the integer to the corresponding unicode character before printing. |
d | Decimal Integer. Outputs the number in base 10. Default. |
e, E | Exponent notation. Prints the number in scientific notation using the letter e/E to indicate the exponent. |
f, F | Fixed point. Displays the number as a fixed-point number. |
g | General format. For a given precision p >= 1, this rounds the number to p significant digits and then formats the result in either fixed-point format or in scientific notation, depending on its magnitude. |
G | General format. Same as g except switches to E if the number gets too large. The representations of infinity and NaN are uppercased, too. |
n | Number. This is the same as g, except that it uses the current locale setting to insert the appropriate number separator characters. |
o | Octal format. Outputs the number in base 8. |
s | String format. This is the default type for strings and may be omitted. |
x, X | Hex format. Outputs the number in base 16, using lower/upper- case letters for the digits above 9. |
% | Percentage. Multiplies the number by 100 and displays in fixed (f) format, followed by a percent sign. |
The example below is using left justification(-) and zero(0) fills.
>>> n = 6789 >>> a = "...%d...%-6d...%06d" %(n, n, n) >>> a '...6789...6789 ...006789' >>> >>> m = 9.876543210 >>> '%e | %E | %f | %g' %(m, m, m, m) '9.876543e+00 | 9.876543E+00 | 9.876543 | 9.87654' >>>
For floating point number, in the following example, we're using left justification, zero padding, numeric + signs, field width, and digits after the decimal point.
>>> '%6.2f | %05.2f | %+06.1f' %(m, m, m) ' 9.88 | 09.88 | +009.9'
We can simply convert them to string using a format expression or str built-in function:
>>> '%s' % m, str(m) ('9.87654321', '9.87654321')
In case when the sizes are unknown until runtime, we can have the width and precision computed by specifying them with a * in the format string to force their values to be taken from the next item in the inputs to the right of the %. In the example, the 4 in the tuple gives precision:
>>> '%f, %.3f, %.*f' % (9.87654321, 9.87654321, 4, 9.87654321) '9.876543, 9.877, 9.8765'
Targets on the left can refer to the keys in a dictionary on the right so that they can fetch the corresponding values. In the example below, the (m) and (w) in the format string refers to the keys in the dictionary on the right and fetch their corresponding values.
>>> '%(m)d %(w)s' %{'m':101, 'w':'Freeway'} '101 Freeway'
We can build a dictionary of values and substitute them all at once with a single formatting expression that uses key-based references. This technique can be used to generate text:
>>> greetings = ''' Hello %(name)s! Merry Christmas. I hope %(year)d will be a good year. ''' >>> replace = {'name' : 'Scala', 'year' : 2013} >>> print(greetings % replace) Hello Scala! Merry Christmas. I hope 2013 will be a good year.
As an another example, we can use it for build-in function, vars():
>>> language = 'Python' >>> version = 320 >>> vars() {'language': 'Python', '__builtins__':, '__package__': None, 'version': 320, '__name__': '__main__', '__doc__': None} >>> '%(language)s %(version)d' % vars() 'Python 320'
Unlike formatting using expressions which is based on C's printf, string formatting using method calls is regarded as more Python-specific.
This new string object's format method uses the subject string as a template and takes any number of arguments that represent values to the substituted according to the template. Within the subject string, curly braces designate substitution targets and arguments to be inserted either by position such as {1} or keyword such as language.
>>> # By position >>> t = '{0}, {1}, {2}' >>> t.format('Dec', '29', '2013') 'Dec, 29, 2013' >>> >>> # By keyword >>> t = '{Month}, {Day}, {Year}' >>> t.format(Month='Dec', Day='29', Year='2013') 'Dec, 29, 2013' >>> >>> # Mixed >>> t = '{Year}, {Month}, {0}' >>> t '{Year}, {Month}, {0}' >>> t.format('29', Year='2013', Month='Dec') '2013, Dec, 29' >>>
Arbitrary object type can be substituted from a temporary string:
>>> '{Month}, {Year}, {0}'.format('29', Month=['Nov', 'Dec'], Year='2013') "['Nov', 'Dec'], 2013, 29"
Format strings can name object attributes and dictionary keys. Square brackets name dictionary keys and dots denote object attributes of an item referenced by position or keyword.
>>> import sys >>> >>> 'My {0[Machine]} is running {1.platform}'.format({'Machine': 'notebook'}, sys) 'My notebook is running win32' >>> >>> 'My {config[Machine]} is running {sys.platform}'.format(config={'Machine':'notebook'}, sys=sys) 'My notebook is running win32'
In the example above, the first one indexes a dictionary on the key Machine and then fetches the attribute platform from the imported sys module. Though the 2nd case in the example is doing the same thing, but names the objects by keyword not by its position.
By adding extra syntax in the format string, we can achieve more specific layouts similar to the % expressions.
{fieldName!conversionFlag:formatSpecification}
For the formatting method, we can use a colon after the substitution target's identification, followed by a format specifier that can name the field size, justification, and a specific type code.
- fieldName is a number or keyword naming an argument, followed by optional .name attribute or [index] references.
- conversionFlag can be r, s, or a to call repr, str, or ascii built-in functions on the value, respectively.
- formatSpecification specifies how the value should be presented.
The alignment for the formatSpecification can be <. >, =, or ^, for left alignment, right alignment, padding after a sign character, or centered alignment, respectively.
>>> '{0:10} = {1:5}'.format(3.1415, 'pi') ' 3.1415 = pi ' >>> '{0:15} = {1:10}'.format(3.1415, 'pi') ' 3.1415 = pi ' >>> '{0:<15} = {1:>10}'.format(3.1415, 'pi') '3.1415 = pi' >>> >>> '{0.platform:>10} is the platform of my {1[item]:<10}'.format(sys, dict(item='notebook')) ' win32 is the platform of my notebook ' >>>
The following example shows the specification for floating point numbers:
>>> '{0:e},{1:3e},{2:g},{3:f},{4:.2f},{5:06.2f}'.format(3.1415,3.1415,3.1415,3.1415,3.1415,3.1415) '3.141500e+00,3.141500e+00,3.1415,3.141500,3.14,003.14' >>>
The following example is for the review of formatting we've discussed so far:
# byte.py UNITS = ['KB', 'MB', 'GB', 'TB', 'PB'] def sizeInByte(sz): if sz < 0: raise ValueError('number < 0') factor = 1024 for i in range(len(UNITS)): if sz < factor: if i == 0: return '{0:.1f} {1[0]}'.format(sz, UNITS) elif i == 1: return '{0:.1f} {1[1]}'.format(sz, UNITS) elif i == 2: return '{0:.1f} {1[2]}'.format(sz, UNITS) elif i == 3: return '{0:.1f} {1[3]}'.format(sz, UNITS) else: return '{0:.1f} {1[4]}'.format(sz, UNITS) else: sz /= factor raise ValueError('too big')
After importing the module, we get the following output from the interactive shell:
>>> from byte import sizeInByte >>> sizeInByte(10000) '9.8 MB' >>> sizeInByte(100000000) '95.4 GB' >>> sizeInByte(10000000000000) '9.1 PB' >>> sizeInByte(1000000000000000000000) Traceback (most recent call last): File "", line 1, in File "byte.py", line 23, in sizeInByte raise ValueError('too big') ValueError: too big >>>
The first specification {0:.1f} is for the sz and the second one {1[0]} is for the 'KB' in UNITS list. Note that we used index to the UNITS list to get the proper byte unit. As a foot note, I try to use the variable i for the units such as {1[i]}. But I could not make it work and got an error: "list indices must be integers."
more
Python tutorial
Python Home
Introduction
Running Python Programs (os, sys, import)
Modules and IDLE (Import, Reload, exec)
Object Types - Numbers, Strings, and None
Strings - Escape Sequence, Raw String, and Slicing
Strings - Methods
Formatting Strings - expressions and method calls
Files and os.path
Traversing directories recursively
Subprocess Module
Regular Expressions with Python
Regular Expressions Cheat Sheet
Object Types - Lists
Object Types - Dictionaries and Tuples
Functions def, *args, **kargs
Functions lambda
Built-in Functions
map, filter, and reduce
Decorators
List Comprehension
Sets (union/intersection) and itertools - Jaccard coefficient and shingling to check plagiarism
Hashing (Hash tables and hashlib)
Dictionary Comprehension with zip
The yield keyword
Generator Functions and Expressions
generator.send() method
Iterators
Classes and Instances (__init__, __call__, etc.)
if__name__ == '__main__'
argparse
Exceptions
@static method vs class method
Private attributes and private methods
bits, bytes, bitstring, and constBitStream
json.dump(s) and json.load(s)
Python Object Serialization - pickle and json
Python Object Serialization - yaml and json
Priority queue and heap queue data structure
Graph data structure
Dijkstra's shortest path algorithm
Prim's spanning tree algorithm
Closure
Functional programming in Python
Remote running a local file using ssh
SQLite 3 - A. Connecting to DB, create/drop table, and insert data into a table
SQLite 3 - B. Selecting, updating and deleting data
MongoDB with PyMongo I - Installing MongoDB ...
Python HTTP Web Services - urllib, httplib2
Web scraping with Selenium for checking domain availability
REST API : Http Requests for Humans with Flask
Blog app with Tornado
Multithreading ...
Python Network Programming I - Basic Server / Client : A Basics
Python Network Programming I - Basic Server / Client : B File Transfer
Python Network Programming II - Chat Server / Client
Python Network Programming III - Echo Server using socketserver network framework
Python Network Programming IV - Asynchronous Request Handling : ThreadingMixIn and ForkingMixIn
Python Coding Questions I
Python Coding Questions II
Python Coding Questions III
Python Coding Questions IV
Python Coding Questions V
Python Coding Questions VI
Python Coding Questions VII
Python Coding Questions VIII
Python Coding Questions IX
Python Coding Questions X
Image processing with Python image library Pillow
Python and C++ with SIP
PyDev with Eclipse
Matplotlib
Redis with Python
NumPy array basics A
NumPy Matrix and Linear Algebra
Pandas with NumPy and Matplotlib
Celluar Automata
Batch gradient descent algorithm
Longest Common Substring Algorithm
Python Unit Test - TDD using unittest.TestCase class
Simple tool - Google page ranking by keywords
Google App Hello World
Google App webapp2 and WSGI
Uploading Google App Hello World
Python 2 vs Python 3
virtualenv and virtualenvwrapper
Uploading a big file to AWS S3 using boto module
Scheduled stopping and starting an AWS instance
Cloudera CDH5 - Scheduled stopping and starting services
Removing Cloud Files - Rackspace API with curl and subprocess
Checking if a process is running/hanging and stop/run a scheduled task on Windows
Apache Spark 1.3 with PySpark (Spark Python API) Shell
Apache Spark 1.2 Streaming
bottle 0.12.7 - Fast and simple WSGI-micro framework for small web-applications ...
Flask app with Apache WSGI on Ubuntu14/CentOS7 ...
Fabric - streamlining the use of SSH for application deployment
Ansible Quick Preview - Setting up web servers with Nginx, configure enviroments, and deploy an App
Neural Networks with backpropagation for XOR using one hidden layer
NLP - NLTK (Natural Language Toolkit) ...
RabbitMQ(Message broker server) and Celery(Task queue) ...
OpenCV3 and Matplotlib ...
Simple tool - Concatenating slides using FFmpeg ...
iPython - Signal Processing with NumPy
iPython and Jupyter - Install Jupyter, iPython Notebook, drawing with Matplotlib, and publishing it to Github
iPython and Jupyter Notebook with Embedded D3.js
Downloading YouTube videos using youtube-dl embedded with Python
Machine Learning : scikit-learn ...
Django 1.6/1.8 Web Framework ...
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization