Image Noise Reduction : Non-local Means Denoising Algorothm

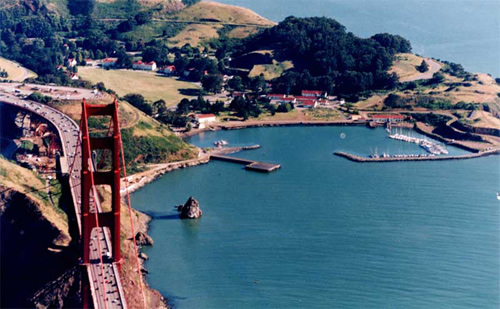
I added some noise to the picture above, and it's shown in the picture below:
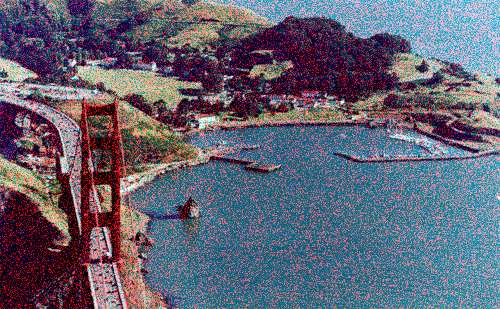
Let's see how OpenCV fares.
One of the fundamental challenges in image processing and computer vision is image denoising. What denoising does is to estimate the original image by suppressing noise from the image. Image noise may be caused by different sources ( from sensor or from environment) which are often not possible to avoid in practical situations. Therefore, image denoising plays an important role in a wide range of applications such as image restoration, visual tracking, image registration, and image segmentation. While many algorithms have been proposed for the purpose of image denoising, the problem of image noise suppression remains an open challenge, especially in situations where the images are acquired under poor conditions where the noise level is very high.
There are two main types of noise in the image:
- salt and pepper noise : It has sparse light and dark disturbances.
"Pixels in the image are very different in color or intensity from their surrounding pixels; the defining characteristic is that the value of a noisy pixel bears no relation to the color of surrounding pixels. Generally this type of noise will only affect a small number of image pixels. When viewed, the image contains dark and white dots, hence the term salt and pepper noise." - wiki - Noise reduction.
- Gaussian noise: "Each pixel in the image will be changed from its original value by a (usually) small amount. A histogram, a plot of the amount of distortion of a pixel value against the frequency with which it occurs, shows a normal distribution of noise. While other distributions are possible, the Gaussian (normal) distribution is usually a good model, due to the central limit theorem that says that the sum of different noises tends to approach a Gaussian distribution."- wiki - Noise reduction.
"Principal sources of Gaussian noise in digital images arise during acquisition eg. sensor noise caused by poor illumination and/or high temperature, and/or transmission eg. electronic circuit noise." wiki - Gaussian_noise.
This is the type we're going to work on with OpenCV in this chapter!
In this section, we'll use cv2.fastNlMeansDenoisingColored() function which is the implementation of Non-local Means Denoising algorithm. It is defined like this:
cv2.fastNlMeansDenoisingColored(src[, dst[, h[, hColor[, templateWindowSize[, searchWindowSize]]]]])
The parameters are:
- src : Input 8-bit 3-channel image.
- dst : Output image with the same size and type as src .
- h : Parameter regulating filter strength for luminance component. Bigger h value perfectly removes noise but also removes image details, smaller h value preserves details but also preserves some noise.
- templateWindowSize : Size in pixels of the template patch that is used to compute weights. Should be odd. Recommended value 7 pixels
- searchWindowSize : Size in pixels of the window that is used to compute weighted average for given pixel. Should be odd. Affect performance linearly: greater searchWindowsSize - greater denoising time. Recommended value 21 pixels
Note: The function converts image to CIELAB colorspace and then separately denoise L and AB components with given h parameters using fastNlMeansDenoising function.
Here is the code to remove the Gaussian noise from a color image using the Non-local Means Denoising algorithm:
import numpy as np import cv2 from matplotlib import pyplot as plt img = cv2.imread('DiscoveryMuseum_NoiseAdded.jpg') b,g,r = cv2.split(img) # get b,g,r rgb_img = cv2.merge([r,g,b]) # switch it to rgb # Denoising dst = cv2.fastNlMeansDenoisingColored(img,None,10,10,7,21) b,g,r = cv2.split(dst) # get b,g,r rgb_dst = cv2.merge([r,g,b]) # switch it to rgb plt.subplot(211),plt.imshow(rgb_img) plt.subplot(212),plt.imshow(rgb_dst) plt.show()
The pictures below are before and after the denoising:
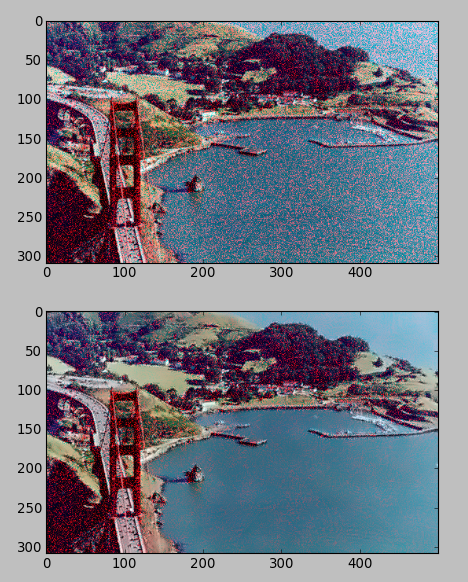
Denoising seems to be working!
If we apply the cv2.fastNlMeansDenoisingColored(img,None,10,10,7,21) function to the wiki's Gaussian sample above:
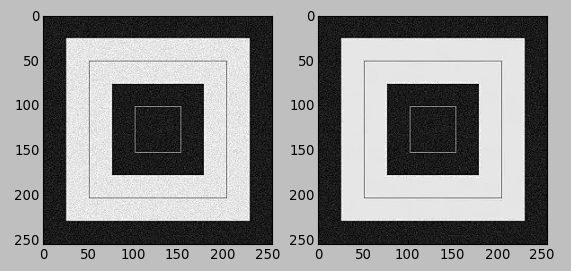
It's not quite there yet.
So, when we apply gray scale function, cv2.fastNlMeansDenoising(img,None,10,7,21), it seems to be working better denoising black and white picture with Gaussian noise:
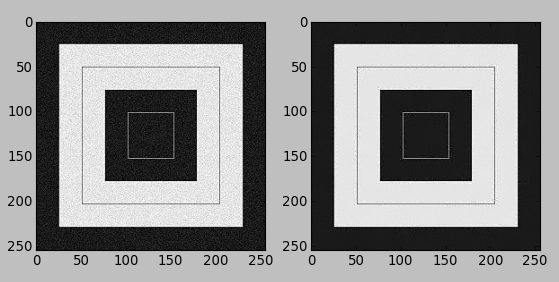
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization