Python Introduction 2020
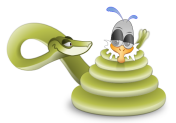
Python scripts can easily communicate with other application variety of integration mechanisms.
Python code can invoke C/C++ libraries and can be called from C/C++.
For example, integrating a C library into Python enables Python to test and launch the library's component, and embedding Python in a product enables onsite customizations to be coded without having to recompile the entire product.
It can integrate with Java and .Net components, and it can communicate over frameworks such as COM. It also can interface with devices over serial ports, and can interact over networks with interfaces like SOAP, XML-RPC, and COBRA.
Another alternative ways to script components are:
- Cython system allows coders to mix Python and C-code.
- SWIG and SIP code generators can automate much of the work needed to link compiled components into Python.
- Python's COM support.
- Iron Python .NET frameworks based implementation.
- Jython: Java-based implementation.
- COBRA toolkits for Python.
For general database, Python gives us interfaces to all RDBS - Sybase, Oracle, MySQL, PostgreSQL, and SQLite.
Python's standard pickle module provides a simple object persistence system.
Python's simplicity and rapid turnaround make it a good match for graphical user interface programming. Python comes with a object-oriented interface to the Tk GUI API called tkinter which allows Python to implement portable GUIs with native look and feel.
Python/tkinter GUIs run unchanged for different platforms.
Python scripts can:
- Communicate over sockets.
- Extract form information sent to server-side CGI scripts.
- Transfer files by FTP.
- Parse, generate, and analyze XML files.
- Send, receive, compose, and parse email.
- Fetch web pages by URLs.
- Parse the HTML and XML of fetched web pages.
- Communicate over XML-RPC, SOAP, and Telnet.
There are framework web development packages for Python:
- Django
- TurboGears
- web2py
- Pylons
- Zope
- WebWare
Python's built-in interfaces to OS services make it ideal for writing portable shell tools.
Python's standard library comes with POSIX binding and support for all the usual OS tools.
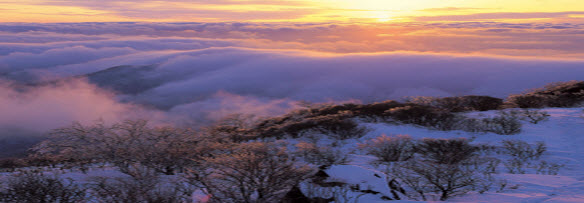
The Python code we write must always be run by the Interpreter.
To enable it, we must install a Python interpreter on our machine.
When the Python package is installed on our machine, it generates number of components:
- Interpreter
- Library
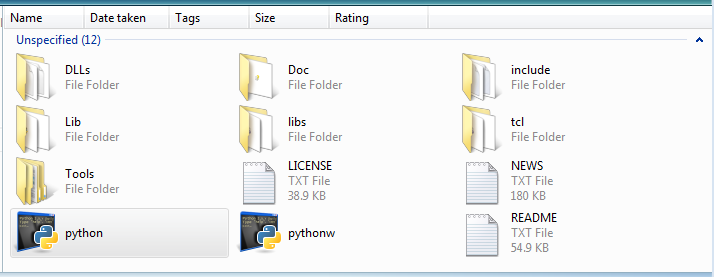
Make a file hello.py with the following line.
print("Hello world!")
The extension py is not required but for consistency, Python file usually has that extension.
If we run the file on a command prompt window:
C:\temp > python hello.py Hello World
We typed the code into text file, and we run the file through the interpreter. Simple!
But let's think about the runtime structure of Python.
When we instruct Python to run our script, there are a few steps that Python carries out before our code actually starts crunching away:
- It is compiled to bytecode.
- Then it is routed to virtual machine.
When we execute a source code, Python compiles it into a byte code. Compilation is a translation step, and the byte code is a low-level platform-independent representation of source code. Note that the Python byte code is not binary machine code (e.g., instructions for an Intel chip).
Actually, Python translate each statement of the source code into byte code instructions by decomposing them into individual steps. The byte code translation is performed to speed execution.
Byte code can be run much more quickly than the original source code statements. It has .pyc extension and it will be written if it can write to our machine.
So, next time we run the same program, Python will load the .pyc file and skip the compilation step unless it's been changed. Python automatically checks the timestamps of source and byte code files to know when it must recompile. If we resave the source code, byte code is automatically created again the next time the program is run.
If Python cannot write the byte code files to our machine, our program still works. The byte code is generated in memory and simply discarded on program exit. But because .pyc files speed startup time, we may want to make sure it has been written for larger programs.
Let's summarize what happens behind the scenes.
When a Python executes a program, Python reads the .py into memory, and parses it in order to get a bytecode, then goes on to execute. For each module that is imported by the program, Python first checks to see whether there is a precompiled bytecode version, in a .pyo or .pyc, that has a timestamp which corresponds to its .py file. Python uses the bytecode version if any. Otherwise, it parses the module's .py file, saves it into a .pyc file, and uses the bytecode it just created.
Byte code files are also one way of shipping Python codes. Python will still run a program if all it can find are .pyc files, even if the original .py source files are not there.
Once our program has been compiled into byte code, it is shipped off for execution to Python Virtual Machine (PVM). The PVM is not a separate program. It need not be installed by itself. Actually, the PVM is just a big loop that iterates through our byte code instruction, one by one, to carry out their operations. The PVM is the runtime engine of Python. It's always present as part of the Python system. It's the component that truly runs our scripts. Technically it's just the last step of what is called the Python interpreter.
Unlike other compiled languages, Python code runs immediately after it is written. Byte code is a Python-specific representation. Since byte code is not a binary machine instructions, it requires more work than CPU instructions.
On the other hand, unlike other interpreters, there is still an internal compile step - Python does not need to reanalyze and reparse each source statement repeatedly. So, Python code runs at speeds somewhere between those of a traditional compiled language and a classic interpreted language.
There is no distinction between the development and execution environments. In other words, the compiler is always right there at runtime and is part of the system that runs the program. All we have in Python is runtime. There is no initial compile-time phase at all and everything happens as the program is running.
This even includes operation such as the creation of functions and classes and the linking of modules. Such events occur before execution in more static languages, but in Python, they happen as programs execute.
This adds a more dynamic flavor to the Python - it is often very convenient for Python programs to construct and execute other Python programs at runtime. The eval and exec built-ins, for example, accept and run strings containing Python program code.
This structure is why Python lends itself to customization. Because Python code can be changed on the fly, users can modify the Python parts of a system onsite without needing to compile the entire system's code.
It is possible to turn our Python programs into true executables. This is known as frozen binaries. Frozen binaries bundles together the byte code of our program files, along with the PVM interpreter and any Python support files our program needs, into a single package, a single binary executable program like .exe file on Windows. In other words, the byte code and PVM are merged into a single component.
Frozen binaries are not the same as the output of a true compiler. They run byte code through a virtual machine. Frozen binaries are not small because they contain PVM, but they are not unusually large either.
Three primary systems are capable of generating frozen binaries:
- py2exe for Windows.
- PyInstaller is similar to py2exe but also works on Linux and Unix.
- freeze.
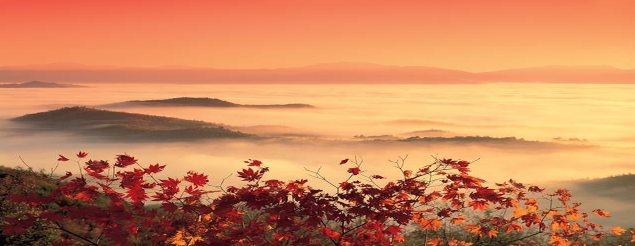
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization