generator.send() with yield
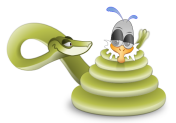
It may be difficult to understand what the following code is doing:
import random def cf(): while True: val = yield print val, def pf(c): while True: val = random.randint(1,10) c.send(val) yield if __name__ == '__main__': c = cf() c.send(None) p = pf(c) for wow in range(10): next(p)
The output looks like this:
7 5 7 3 10 10 8 2 3 10
To understand the inner workings of the code, let's go to the next section.
Let's start with the interactive python as below:
>>> def f(): ... while True: ... val = yield ... yield val*10 ... >>> g = f() >>> g.next() >>> g.send(1) 10 >>> g.next() >>> g.send(10) 100 >>> g.next() >>> g.send(0.5) 5.0 >>>
When we do g = f(), g gets the generator. Python's generator class has generator.next() and generator.send(value) methods. What the next() does is clear: the execution continues to the next yield expression. The send(value) sends a value into the generator function. The value argument becomes the result of the current yield expression.
We're getting closer!
Another worming up. What does the output look like from the code below?
def f(): yield def g(): return print 'f()=', f() print 'g()=', g()
Output:
f()=g()= None
Now, let's look into our initial code in the earlier section again:
import random def cf(): while True: val = yield print val, def pf(c): while True: val = random.randint(1,10) c.send(val) yield if __name__ == '__main__': c = cf() c.send(None) p = pf(c) for wow in range(10): next(p)
Now we can see the function cf() is returning a generator because of the yield keyword. So,
c = cf()
c gets a generator, and passing it to pf(c) where it sends a random value to c. Within p it prints out the value it's called in the for loop:
for wow in range(10): next(p)
For more information on generator or yield, please visit
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization