Basic Image Operations - pixel access

To load an image, we can use imread() function. It loads an image from the specified file and returns it. Actually, it reads an image as an array of RGB values. If the image cannot be read (because of missing file, improper permissions, unsupported or invalid format), the function returns an empty matrix ( Mat::data==NULL ). Even if the image path is wrong, it won't throw any error, but print image will give us None.
Here are the file formats that are currently supported:
- Windows bitmaps - *.bmp, *.dib
- JPEG files - *.jpeg, *.jpg, *.jpe
- JPEG 2000 files - *.jp2
- Portable Network Graphics - *.png
- Portable image format - *.pbm, *.pgm, *.ppm
- Sun rasters - *.sr, *.ras
- TIFF files - *.tiff, *.tif
CloudyGoldenGate.jpg:
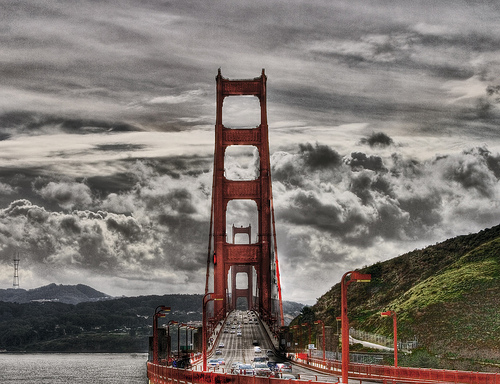
CloudyGoldenGate_grayscale.jpg:
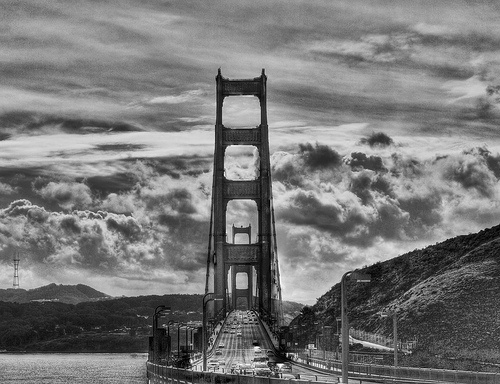
Here is a simple python code for image loading:
import cv2 import numpy as np img = cv2.imread('images/CloudyGoldenGate.jpg')
The syntax for the imread() looks like this:
cv2.imread(filename[, flags])
The flags is to specify the color type of a loaded image:
- CV_LOAD_IMAGE_ANYDEPTH - If set, return 16-bit/32-bit image when the input has the corresponding depth, otherwise convert it to 8-bit.
- CV_LOAD_IMAGE_COLOR - If set, always convert image to the color one.
- CV_LOAD_IMAGE_GRAYSCALE - If set, always convert image to the grayscale one.
- >0 Return a 3-channel color image.
- =0 Return a grayscale image.
- <0 Return the loaded image as is (with alpha channel).
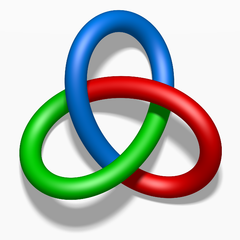
Image properties include number of rows, columns and channels, type of image data, number of pixels etc.
Shape of image is accessed by img.shape. It returns a tuple of number of rows, columns and channels. If image is grayscale, tuple returned contains only number of rows and columns. So it is a good method to check if loaded image is grayscale or color image:
import cv2 import numpy as np img_file = 'images/TriColor.png' img = cv2.imread(img_file, cv2.IMREAD_COLOR) # rgb alpha_img = cv2.imread(img_file, cv2.IMREAD_UNCHANGED) # rgba gray_img = cv2.imread(img_file, cv2.IMREAD_GRAYSCALE) # grayscale print type(img) print 'RGB shape: ', img.shape # Rows, cols, channels print 'ARGB shape:', alpha_img.shape print 'Gray shape:', gray_img.shape print 'img.dtype: ', img.dtype print 'img.size: ', img.size
Output:
<type 'numpy.ndarray'> RGB shape: (240, 240, 3) ARGB shape: (240, 240, 4) Gray shape: (240, 240) img.dtype: uint8 img.size: 172800
- img.dtype (usually, dtype=np.uint8) is very important while debugging because a large number of errors in OpenCV-Python code is caused by invalid datatype.
- If image is grayscale, tuple returned does not contain any channels.
- The number of channels for ARGB = 4.
We can access a pixel value by its row and column coordinates. For BGR image, it returns an array of Blue, Green, Red values. For grayscale image, corresponding intensity is returned.
We get BGR value from the color image:
img[45, 90] = [200 106 5] # mostly blue img[173, 25] = [ 0 111 0] # green img[145, 208] = [ 0 0 177] # red
We can also access the alpha value (transparent = 0, opaque = 255) and grayscale value (intensity) as well.
alpha_img[173, 25] = [ 0 111 0 255] # opaque gray_img[173, 25] = 87 # intensity for grayscale
If we specify what we want to get. Here we specified 5x5 pixels:
alpha_img[170:175, 25:30, 0] = [[0 0 0 0 0] # blue [1 0 0 0 0] [2 0 0 0 0] [0 1 0 0 0] [0 2 0 0 0]] alpha_img[170:175, 25:30, 1] = [[137 150 161 170 178] # green [130 143 155 165 173] [122 136 148 159 168] [111 128 140 152 162] [ 98 120 132 145 155]] alpha_img[170:175, 25:30, 2] = [[0 0 0 0 0] # red [1 0 0 0 0] [2 0 0 0 0] [0 1 0 0 0] [0 2 0 0 0]] alpha_img[170:175, 25:30, 3] = [[255 255 255 255 255] # alpha [255 255 255 255 255] [255 255 255 255 255] [255 255 255 255 255] [255 255 255 255 255]] gray_img[170:175, 25:30] = [[107 117 126 133 140] # intensity [102 111 120 129 135] [ 95 106 116 124 131] [ 87 99 109 119 127] [ 76 93 103 114 120]]
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization