REST API Http Requests for Humans with Flask
A simple, easy-to-use HTTP library written in Python.
Requests makes interacting with Web services seamless, and it overcomes most of the difficulties in urllib/urllib2.
In this tutorial, we'll learn how to make http requests such as GET and POST.
We'll use a minimal Flask wsgi server to respond to the requests.
It might be better using virtualenv with Python3. So, let's do it.
$ virtualenv -p python3 venv $ source venv/bin/activate (venv)$
Then, install Flask:
(venv)$ pip install flask
Also, we may want to install requests into our virtualenv:
(venv)$ pip install requests
Here is the code we need to return response from Flask server. We don't have to know how it works. We just run it.
from flask import Flask from flask import jsonify from flask import request app = Flask(__name__) quarks = [{'name': 'up', 'charge': '+2/3'}, {'name': 'down', 'charge': '-1/3'}, {'name': 'charm', 'charge': '+2/3'}, {'name': 'strange', 'charge': '-1/3'}] @app.route('/', methods=['GET']) def hello_world(): return jsonify({'message' : 'Hello, World!'}) @app.route('/quarks', methods=['GET']) def returnAll(): return jsonify({'quarks' : quarks}) @app.route('/quarks/<string:name>', methods=['GET']) def returnOne(name): theOne = quarks[0] for i,q in enumerate(quarks): if q['name'] == name: theOne = quarks[i] return jsonify({'quarks' : theOne}) @app.route('/quarks', methods=['POST']) def addOne(): new_quark = request.get_json() quarks.append(new_quark) return jsonify({'quarks' : quarks}) @app.route('/quarks/<string:name>', methods=['PUT']) def editOne(name): new_quark = request.get_json() for i,q in enumerate(quarks): if q['name'] == name: quarks[i] = new_quark qs = request.get_json() return jsonify({'quarks' : quarks}) @app.route('/quarks/<string:name>', methods=['DELETE']) def deleteOne(name): for i,q in enumerate(quarks): if q['name'] == name: del quarks[i] return jsonify({'quarks' : quarks}) if __name__ == "__main__": app.run(debug=True)
Well, it might be better know the basics.
Note that we have 4 routes: 3 GETs, 1 POST, 1 PUT, and 1 DELETE. All of them returns json. We'll eventually figure out how request/response works when we make requests in later section of this tutorial.
The reference for Flask :
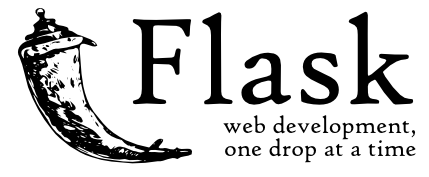
For now, let's run our Flask server.
(venv)$ $ python hello.py * Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
Ok, our server is listening on port 5000 waiting for any request.
Open up another terminal to get interactive python shell, preferably, with Python 3 virtual env.
(venv)$ python Python 3.4.3 (default, Oct 14 2015, 20:28:29) [GCC 4.8.4] on linux Type "help", "copyright", "credits" or "license" for more information. >>>
We're about to make a requests, and the requests module is available since we've installed it on our virtualenv. So, all we have to do is to import the module into our console session:
>>> import requests
Now we are ready to make a request. So, let's make the simplest one, 'root':
>>> response = requests.get("http://127.0.0.1:5000") >>> response.json() {'message': 'Hello, World!'}
Ok, we got the response for the '/' request.
Let's try another request. This time for '/quarks':
>>> response = requests.get("http://127.0.0.1:5000/quarks") >>> response.json() {'quarks': [{'charge': '+2/3', 'name': 'up'}, {'charge': '-1/3', 'name': 'down'}, {'charge': '+2/3', 'name': 'charm'}, {'charge': '-1/3', 'name': 'strange'}]}
We can convert the response to JSON object and play with it:
>>> jsonObj = response.json() >>> jsonObj {'quarks': [{'charge': '+2/3', 'name': 'up'}, {'charge': '-1/3', 'name': 'down'}, {'charge': '+2/3', 'name': 'charm'}, {'charge': '-1/3', 'name': 'strange'}]} >>> jsonObj['quarks'][0]['name'] 'up' >>> jsonObj['quarks'][1]['charge'] '-1/3'
Let's make a request just one item:
>>> response = requests.get("http://127.0.0.1:5000/quarks/up") >>> response.json() {'quarks': {'charge': '+2/3', 'name': 'up'}}
So far, we made 'GET' requests. How about 'PUT' request?
We'll add 'top' quark to our existing dictionary:
>>> response = requests.post("http://127.0.0.1:5000/quarks", json={"name":"top","charge":"+2/3"}) >>> response.json() {'quarks': [{'charge': '+2/3', 'name': 'up'}, {'charge': '-1/3', 'name': 'down'}, {'charge': '+2/3', 'name': 'charm'}, {'charge': '-1/3', 'name': 'strange'}, 'name': {'charge': '+2/3', 'name': 'top'}}]}
Note that we made a POST request to the same endpoint ("/quarks") and appended one more records.
If we switch to the server console, we can see the HTTP responses so far:
(venv)$ python hello.py * Running on http://127.0.0.1:5000/ (Press CTRL+C to quit) 127.0.0.1 - - "GET / HTTP/1.1" 200 - 127.0.0.1 - - "GET /quarks HTTP/1.1" 200 - 127.0.0.1 - - "GET /quarks/1 HTTP/1.1" 200 - 127.0.0.1 - - "POST /quarks HTTP/1.1" 200 -
From now on, we'll also use Postman in addition to our command shell.
Here is what we've got so far:
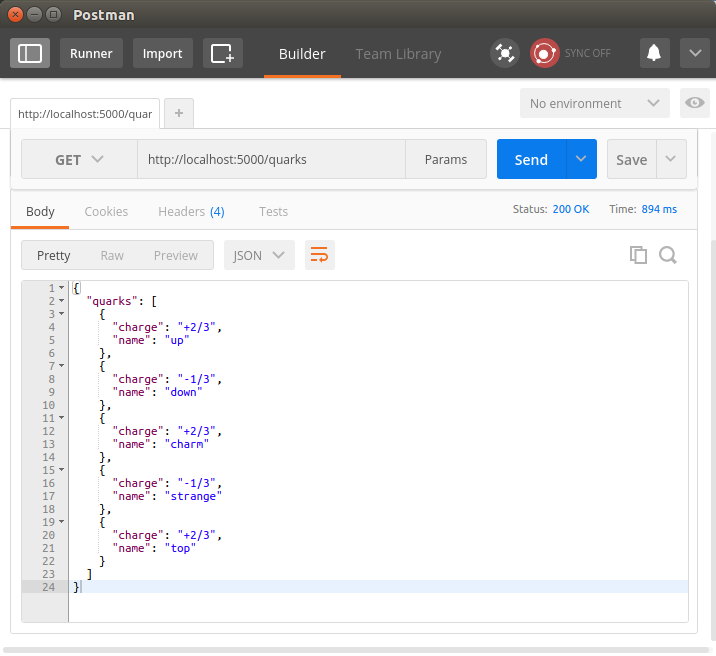
In particle physics, we have one more quark, 'bottom' with '-1/3 charge. Let's add it using Postman's POST feature:
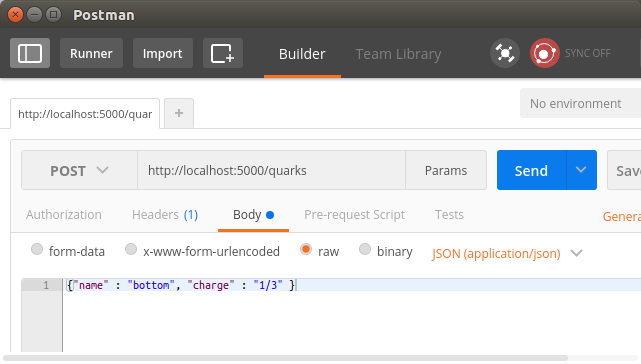
Now we have all 6 quarks:
>>> response = requests.post("http://127.0.0.1:5000/quarks") >>> response.json() {'quarks': [{'name': 'up', 'charge': '+2/3'}, {'name': 'down', 'charge': '-1/3'}, {'name': 'charm', 'charge': '+2/3'}, {'name': 'strange', 'charge': '-1/3'}, {'name': 'top', 'charge': '+2/3'}, {'name': 'bottom', 'charge': '1/3'}]}
We made a mistake for the charge of 'bottom' quark : we put positive charge of '1/3' instead of negative value of '-1/3'
Let' update the data:
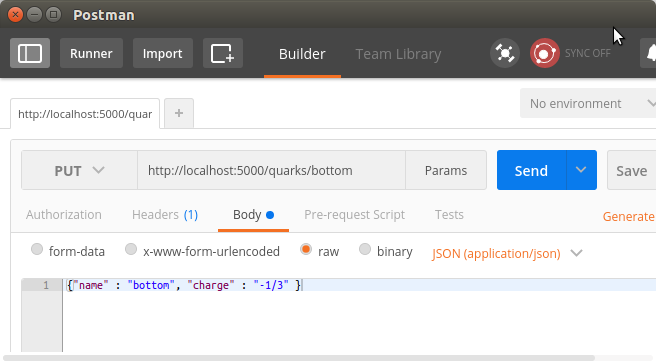
Finally, we finished the quarks list!
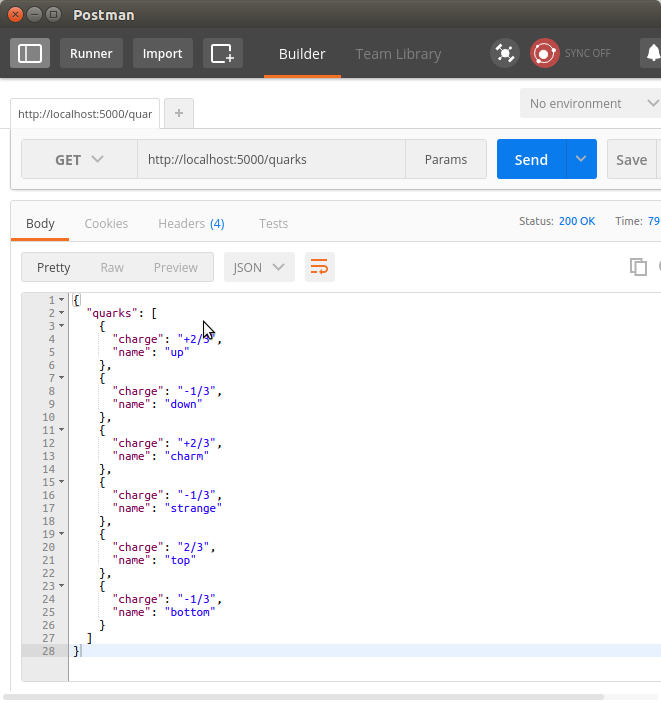
DELETE request is much similar to put request. After matching the given name, delete an item by the matching index:
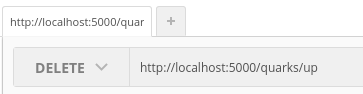