Coding Questions II - 2024
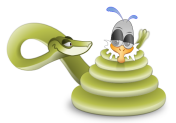
In information theory, the Hamming distance between two strings of equal length is the number of positions at which the corresponding symbols are different:
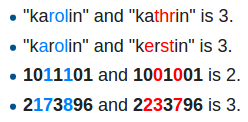
Here is the code for the Hamming distance:
def hamming(s1,s2): if len(s1) != len(s2): raise ValueError("Not defined - unequal lenght sequences") return sum(c1 != c2 for c1,c2 in zip(s1,s2)) if __name__ == '__main__': print(hamming("karolin", "kathrin")) # 3 print(hamming("karolin", "kerstin")) # 3 print(hamming("1011101", "1001001")) # 2 print(hamming("2173896", "2233796")) # 3 print(hamming("00000", "11111")) # 5
The first zip
will produce [('k', 'k'), ('a', 'a'), ('r', 't'), ('o', 'h'), ('l', 'r'), ('i', 'i'), ('n', 'n')].
and the sum()
counts the number of False
, for example 3, because the input for sum()
should look like this:
[False, False, True, True, True, False, False].
Write a floor division function without using '/' or '%' operator. For example, f(5,2) = 5/2 = 2, f(-5,2) = -5/2 = -3.
def floor(a,b): count = 0 sign = 1 if a < 0: sign = -1 while True: if b == 1: return a # positive if a >= 0: a -= b if a < 0: break # negative else: a = -a - b a = -a if a > 0: count += 1 break count += 1 return count*sign from random import randint n = 20 while n > 0: a, b = randint(-20,20), randint(1,10) print '%s/%s = %s' %(a,b, floor(a,b)) n -= 1
Output:
11/4 = 2 3/5 = 0 11/5 = 2 10/1 = 10 -6/9 = -1 14/2 = 7 -4/7 = -1 8/6 = 1 7/1 = 7 -7/1 = -7 -20/5 = -4 11/4 = 2 16/10 = 1 -9/7 = -2 -7/6 = -2 -8/2 = -4 13/7 = 1 -3/2 = -2 -10/2 = -5 -6/1 = -6
Q1: How do you fetch every other item in the list?
Suppose we have a list like this:
>>> L = [x*10 for x in range(10)] >>> L [0, 10, 20, 30, 40, 50, 60, 70, 80, 90]
- We can use enumerate(L) to get indexed series:
>>> for i,v in enumerate(L): ... if i % 2 == 0: ... print v, ... 0 20 40 60 80
- This one may be the simplest solution:
L2 = L[::2] >>> L2 [0, 20, 40, 60, 80]
What's get printed?
def f(): pass print type(f()) print type(1J) print 1j*1j print type(lambda:None)
Answer:
<type 'NoneType'> <type 'complex'> (-1+0j) <type 'function'>
- The argument to the type() call is a return value of a function call, which returns None.
- 'lambda arguments: expression' yields a function object.
- An imaginary literal yields a complex number with a real part of 0.0.
Q2: What's the list comprehension? How about dictionary comprehension?
Construct x^3 from a list of integers, L = [0,1,2,3,4,5]
List comprehension:
>>> L2 = [x**3 for x in L] >>> L2 [0, 1, 8, 27, 64, 125]
Or:
>>> L2 = [x**3 for x in range(6)] >>> L2 [0, 1, 8, 27, 64, 125]
Dictionary comprehension:
>>> D1 = dict((k,k**3) for k in L) >>> D1 {0: 0, 1: 1, 2: 8, 3: 27, 4: 64, 5: 125}
Or for Python 3:
>>> D2 = {k:k**3 for k in L} >>> D2 {1: 1, 2: 8, 3: 27}
Q3: Sum of all elements in a list ([1,2,3,...,100] with one line of code.
>>> Ans = sum(range(101)) >>> Ans 5050
Q3.b: How about summing only odd elements?
>>> sum(range(1,101,2))
Or:
>>> Ans = sum(x for x in range(101) if x % 2 != 0) >>> Ans 2500
Or:
>>> Ans = reduce(lambda x,y: x+y, filter(lambda x: x % 2 != 0, range(1,101))) >>> Ans 2500
Or
>>> sum(filter(lambda x: x % 2, range(1,101)))
Two divisions - '/' and '//':
>>> 5.5/2 2.75 >>> 5.5//2 2.0
Q: What are the differences between Python 2 and Python 3?
Please visit Python 2 vs Python 3.
What's the output?
>>> len(set([1,1,2,3,3,3,4]))
Output:
4
set() only retains unique values.
We want to print a list of files in a directory including the sub-directories. We may want to do it recursively.
import os def file_list(dir): basedir = dir subdir_list = [] for item in os.listdir(dir): fullpath = os.path.join(basedir,item) if os.path.isdir(fullpath): subdir_list.append(fullpath) else: print fullpath for d in subdir_list: file_list(d) file_list('/dir')
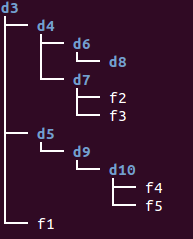
Output:
./d3/f1 ./d3/d4/d7/f2 ./d3/d4/d7/f3 ./d3/d5/d9/d10/f4 ./d3/d5/d9/d10/f5
Here is simpler code:
import os path = './d3' file_list = [] for root, dir_names, file_names in os.walk(path): for f in file_names: file_list.append(os.path.join(root,f)) print(file_list)
Output:
['./d3/f1', './d3/d4/d7/f3', './d3/d4/d7/f2', './d3/d5/d9/d10/f4', './d3/d5/d9/d10/f5']
For a given sentence, for example, "The Mississippi River", count the occurrence of a character in the string.
sentence='The Mississippi River' def count_chars(s): s=s.lower() count=list(map(s.count,s)) return (max(count)) print count_chars(sentence)
Let's look into the code, count=list(map(s.count,s))
>>> count = map(s.count,s) >>> count [1, 1, 2, 2, 1, 5, 4, 4, 5, 4, 4, 5, 2, 2, 5, 2, 1, 5, 1, 2, 1]
Actually, the each item in the list output represents the occurrence of each character including space:
T h e M i s s i s s i p p i R i v e r [1, 1, 2, 2, 1, 5, 4, 4, 5, 4, 4, 5, 2, 2, 5, 2, 1, 5, 1, 2, 1]
Answer = 5
In the line, map(func, sequence), the s.count counts the occurrence of a character while it iterates the character sequence of s.
Or
sentence='The Mississippi River' def count_chars(s): s=s.lower() L = [s.count(c) for c in s] return max(L) print (count_chars(sentence))
Or we can use dictionary:
sentence='The Mississippi River' sl = sentence.lower() d = {}.fromkeys([x for x in sl],0) for char in sl: d[char] += 1 print d
Output:
{' ': 2, 'e': 2, 'i': 5, 'h': 1, 'm': 1, 'p': 2, 's': 4, 'r': 2, 't': 1, 'v': 1}
Or we can use dict.get(key,None) method:
sentence='The Mississippi River' sl = sentence.lower() d = {} for c in sl: d[c] = d.get(c,0) + 1 print d
Output:
{' ': 2, 'e': 2, 'i': 5, 'h': 1, 'm': 1, 'p': 2, 's': 4, 'r': 2, 't': 1, 'v': 1}
Or we can use dictionary comprehension:
sentence = "The Mississippi River" ans = dict((c,sentence.count(c)) for c in sentence) print ans
Output:
{' ': 2, 'e': 2, 'i': 5, 'h': 1, 'M': 1, 'p': 2, 's': 4, 'R': 1, 'T': 1, 'v': 1, 'r': 1}
import math def isPrime(n): if n <= 1: return False if n == 2: return True if n % 2 == 0: return False for t in range(3, int(math.sqrt(n)+1),2): if n % t == 0: return False return True print [n for n in range(100) if isPrime(n)]
Output:
[2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97]
A simpler code:
def isPrime(n): if n == 1: return False for t in range(2,n): if n % t == 0: return False return True print [n for n in range(1,100) if isPrime(n)]
Not much but a little bit of tweak: generate a list with the first 100 primes:
import math def isPrime(n): if n == 1: return False elif n == 2: return True elif n % 2 == 0: return False else: for t in range(3, int(math.sqrt(n)+1), 2): if ( n % t == 0 ): return False return True count = 1 n = 1 primes = [] while (count <= 100): if isPrime(n): primes.append(n) count += 1 n += 1 print primes
Run it:
$ python prime2.py python prime.py [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241, 251, 257, 263, 269, 271, 277, 281, 283, 293, 307, 311, 313, 317, 331, 337, 347, 349, 353, 359, 367, 373, 379, 383, 389, 397, 401, 409, 419, 421, 431, 433, 439, 443, 449, 457, 461, 463, 467, 479, 487, 491, 499, 503, 509, 521, 523, 541]
def isPrime(n): if n == 1: return False if n == 2: return True for i in range(2,n): if n % i == 0: return False return True MaxCount = 10 c = 0 n = 2 primes = [] while (c <= MaxCount): if isPrime(n): primes.append(n) c += 1 n += 1
Output for MaxCount = 10:
primes = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31]
When it comes to reversing a string in python, we do have s[::-1], boring!
In the following recursive code, at every call, the first string will go to the end and stored in stack. So, when there is only one character left as an input string, the code starts rewind and retrieve the character one by one. The net effect is it gets the character in reverse. It becomes obvious when we see the printed output below:
def reverse(input): print input if len(input) <= 1: return input return reverse(input[1:]) + input[0] s = 'reverse' print(reverse(s))
Output:
reverse everse verse erse rse se e esrever
See also Reversing words
In the code below, we use range index operation, putting the characters into the list in reverse order. Than we pack them using join():
def reverse(input): return ''.join([input[i] for i in range(len(input)-1, -1, -1)]) # or return ''.join(input[-i-1] for i in range(len(input))) # or return input[::-1] s = 'reverse' print(reverse(s))
In the range() function, we used "-1" as a "stop" index and another "-1" as a "step" size.
Output:
esrever
See also Reversing words
Return a given Number say 12345, in reverse order. So, it should return 54321:
# operates on a number directly def rev1(n): r = 0 for i in range(len(str(n))): r += n % 10 n = n / 10 # promote digits except the last digit if (n > 0): r = r * 10 return r # operates on a converted string def rev2(n): sn = str(n) r = ''.join(sn[-i-1] for i in range(len(sn))) return r # operates on a converted string - simplest def rev3(n): return str(n)[::-1] n = 12345 print(rev1(n)) print(rev2(n)) print(rev3(n))
Output:
54321 54321 54321
Q: What's the output from the following code?
def f(): yield def g(): return print 'f()=', f() print 'g()=', g()
You can find the answer from generator.send() method.
Q: We have a list of tuples where each tuple is a (start, end). We want to merge all overlapping ranges and return a list of distinct ranges. For example:
[(1, 5), (2, 4), (3, 6)] ---> [(1, 6)] [(1, 3), (2, 4), (5, 6)] ---> [(1, 4), (5, 6)]
Write a code to produce a list of tuples with distinct ranges. Try with this data:
L = [(4,9), (20, 22), (1, 3), (24, 32), (23, 31), (40,50), (12, 15), (8,13)]
Here is the code:
L = [(4,9), (20, 22), (1, 3), (24, 32), (23, 31), (40,50), (12, 15), (8,13)] # L = [(1, 5), (2, 4), (3, 6)] # L = [(1, 3), (2, 4), (5, 6)] L = sorted(L) print L Lnew = [] st = L[0][0] end = L[0][1] for item in L[1:]: if end >= item[0]: if end < item[1]: end = item[1] else: Lnew.append((st,end)) st = item[0] end = item[1] Lnew.append((st,end)) print Lnew
Output:
[(1, 3), (4, 9), (8, 13), (12, 15), (20, 22), (23, 31), (24, 32), (40, 50)] [(1, 3), (4, 15), (20, 22), (23, 32), (40, 50)]
Q: Outputs the result using ternary operator. 'pass' when the 'expected' is the same as 'returned', otherwise outputs 'fail'. Assume 'expected' = 1, 'returned' = 0.
expected = 1 returned = 0 result = 'pass' if expected == returned else 'fail' print result
In Python, packing and unpacking refer to operations involving the grouping and unpacking of values in data structures. This often involves tuples, lists, and iterable objects. Let's look at both concepts:
- Packing: the process of combining multiple values into a single variable or data structure. Tuples are commonly used for packing
# Packing values into a tuple packed_tuple = 1, 'apple', 3.14 # Packed tuple print(packed_tuple) # Output: (1, 'apple', 3.14)
- Unpacking: the reverse process of extracting values from a data structure (like a tuple or a list) and assigning them to individual variables.
# Packing values into a tuple packed_tuple = 1, 'apple', 3.14 # Packed tuple print(packed_tuple) # Output: (1, 'apple', 3.14)
We can also use the * operator for variable-length unpacking, where one variable gets assigned the remaining elements as a list:packed_tuple = 1, 'apple', 3.14 first, *rest = packed_tuple print(first) # Output: 1 print(rest) # Output: ['apple', 3.14]
- Packing and Unpacking in Function Arguments:
Packing and unpacking are often used in function arguments.
# Packing arguments into a tuple def example_function(*args): print(args) example_function(1, 'apple', 3.14) # Output: (1, 'apple', 3.14) # Unpacking arguments from a tuple def another_function(a, b, c): print(a, b, c) arguments = (1, 'apple', 3.14) another_function(*arguments) # Output: 1 apple 3.14
In this example, the *arguments syntax unpacks the values from the tuple and passes them as separate arguments to the function. Packing and unpacking are versatile techniques that enhance the flexibility and readability of our code, especially when working with functions or dealing with multiple values.
What will be printed?
def getThem(arg1, *arg2): print type(arg2), arg2 getThem('uno','dos','tres','cuatro','cinco')
arg2 aggregates the rest of args into a tuple. So, the output should look like this:
<type 'tuple'> ('dos', 'tres', 'cuatro', 'cinco')
How about this one:
def getThem(arg1, **arg2): print type(arg2), arg2 getThem('numbers',one='uno',two='dos',three='tres',four='cuatro',five='cinco')
arg2 aggregates the remaining parameters into a dictionary. So, the output is:
<type 'dict'> {'four': 'cuatro', 'three': 'tres', 'five': 'cinco', 'two': 'dos', 'one': 'uno'}
Will the code below work?
def getThem(a,b,c,d,e): print a,b,c,d,e mList = ['uno','dos','tres','cuatro','cinco'] getThem(mList)
Answer: No.
We'll get an error:
TypeError: getThem() takes exactly 5 arguments (1 given)
So, the code should be changed like this:
getThem(*mList)
*mList will unpack the list into individual elements to be passed to the function.
The unpacking a list can also be used as this:
>>> a, *b, c = [1,2,3,4,5,6,7,8,9,10] >>> print(b) [2, 3, 4, 5, 6, 7, 8, 9] >>> a, *b, c, d = [1,2,3,4,5,6,7,8,9,10] >>> print(b) [2, 3, 4, 5, 6, 7, 8]
We have (oth-7th) revisions committed to Github, and due to a testing flaw, at the 7th revision we found that a bug has been introduced. We want to find when it was committed:
# r0 r1 r2 r3 r4 r5 r6 r7 # G ? ? ? ? ? ? B (G: good, B: buggy)
We may want to use binary search: if found bug, move backwards (previous rev.), if not, mode forwards (later rev.). In the code sample below, we're testing 6 cases:
def hasBug(i): if rev[i] == 'B': return True return False def find_bug(good, bad): st = good end = bad ret = -1 mid = (st + end) / 2 while (mid > good and mid < bad): # backwards if hasBug(mid): end = mid ret = mid if mid == good + 1: break # forwards else: st = mid if mid == end - 1: break mid = (st + end ) / 2 return ret if __name__ == "__main__": rev = ['G','B','B','B','B','B','B','B'] print 1 == find_bug(0,7) rev = ['G','G','B','B','B','B','B','B'] print 2 == find_bug(0,7) rev = ['G','G','G','B','B','B','B','B'] print 3 == find_bug(0,7) rev = ['G','G','G','G','B','B','B','B'] print 4 == find_bug(0,7) rev = ['G','G','G','G','G','B','B','B'] print 5 == find_bug(0,7) rev = ['G','G','G','G','G','G','B','B'] print 6 == find_bug(0,7)
Note that the find_bug() function returns the first revision # that introduced a bug.
Output:
True True True True
What will be printed out?
>>> True or False and False
Since AND is higher precedence than OR, AND will be evaluated first, "True" will be printed out.
Then, how about this one?
>>> not True or False or not False and True True
NOT has first precedence, then AND, then OR.
We have a function that returns the amount with tax added. We want to prefix the amount with a dollar($) using decorator:
@dollar def price(amount, tax_rate): return amount + amount*tax_rate
How do we want to implement the decorator function, dollar()?
Here is the code:
def dollar(fn): def new(*args): return '$' + str(fn(*args)) return new @dollar def price(amount, tax_rate): return amount + amount*tax_rate print price(100,0.1)
Output:
$110
Note that we did not modify the decorated function, price(), at all.
This example is not much different from the previous one.
We have a function returning squared number:
def oldFnc(n): return n*n
Write a code doubling the return value from oldFnc() just by adding a decorator like this:
@doubleIt def oldFnc(n): return n*n
Here is a code:
def doubleIt(myfnc): def doubleItInside(*args): return myfnc(*args)*2 return doubleItInside @doubleIt def oldFnc(n): return n*n #oldFnc = doubleIt(oldFnc) print oldFnc(5)
For more information about decorators and the examples, please check Decorators
The following code won't print out properly. What's wrong with the code?
days = ['Monday', 'Tuesday', 'Wednesday'] months = ['Jan', \ 'Feb', \ 'Mar'] print "DAYS: %s, MONTHS %s" % (days, months)
days is OK because expressions in parentheses, square brackets or curly braces can be split over more than one physical line without using backslashes. The months is also OK because backslashes are used to join physical lines, even though they are not required in this case. The print statement will not print the data because 2 logical lines are used without a backslash to join them into a logical line.
So, correct code should look like this:
print "DAYS: %s, MONTHS %s" %\ (days, months)
Recursive binary serach:
# returns True if found the item # otherwise, returns False def bSearch(a, item): if len(a) == 0: return False mid = len(a)/2 if a[mid] == item: return True else: if a[mid] < item: return bSearch(a[mid+1:], item) else: return bSearch(a[:mid], item) if __name__ =="__main__": a = [1,3,4,5,8,9,17,22,36,40] print bSearch(a, 9) print bSearch(a, 10) print bSearch(a, 36)
Output:
True False True
Iterative binary serach:
# returns True if found the item # otherwise, returns False def bSearch(a, item): st = 0 end = len(a)-1 mid = len(a)/2 left = st right = end while True: # found if a[mid] == item: return True # upper elif a[mid] < item: left = mid + 1 # lower else: right = mid - 1 mid = (left+right)/2 if mid < st or mid > end: break if left == right and a[mid] != item: break return False if __name__ =="__main__": a = [1,3,4,5,8,9,17,22,36,40] print bSearch(a, 9) print bSearch(a, 10) print bSearch(a, 36) print bSearch(a, 5) print bSearch(a, 22)
Output:
True False True True True
List is passed by reference to the function and modifications to the function parameter also effect the original list.
What's the size of the list, (len(mList)).
def addItem(l): l += [0] mList = [1, 2, 3, 4] addItem(mList) print len(mList)
Answer: 5
This is not a real calculator. It's a practice of stack implementation by manipulating list's pop() and append().
The code should pass the following test:
test = {"11++":-1, "1+2": -1, "12+":3, "12+4*":12, "12+4*5+":17, "42-5*7-":3}
The keys are the question strings, and the values are the correct answers.
Note the following:
- We should have at least two items in the list whenever we see '+'/'-'/'*' operators. Otherwise, it should issue -1 and exit.
- For '-' operation, we need to take care of the the order of pop() operation. In the code, we prepend '-' for the last item in the list and then pops the 2nd to the last after that.
Here is the code:
def sol(input): a = [] print "input=",input for c in input: if c.isdigit(): a.append(c) elif c == '+': if len(a) < 2: return -1 a.append(int(a.pop())+int(a.pop())) elif c == '-': if len(a) < 2: return -1 a.append(-int(a.pop())+int(a.pop())) elif c == '*': if len(a) < 2: return -1 a.append(int(a.pop())*int(a.pop())) else: pass return a[0] test = {"11++":-1, "1+2": -1, "12+":3, "12+4*":12, "12+4*5+":17, "42-5*7-":3} for k,v in test.items(): if sol(k) == v: print k, v, 'Passed' print else: print k, v, 'Error' print
Output:
input= 12+4* 12+4* 12 Passed input= 42-5*7- 42-5*7- 3 Passed input= 11++ 11++ -1 Passed input= 12+ 12+ 3 Passed input= 12+4*5+ 12+4*5+ 17 Passed input= 1+2 1+2 -1 Passed
Write a class that returns network interfaces (eth0, wlan0, ...).
Use subprocess and ip link show command.
import subprocess class interfaces: def __init__(self): self.intf = [] proc = subprocess.Popen(['ip', 'link', 'show'], stdout=subprocess.PIPE) line_count = 0 while True: line = proc.stdout.readline() if line != '': if line_count % 2 == 0: self.intf.append(line) else: break line_count += 1 self.counter = -1 self.max = len(self.intf) def __iter__(self): return self def next(self): if self.counter >= self.max-1: raise StopIteration self.counter += 1 return self.intf[self.counter] f = interfaces() for i in f: print i
Note that we used next() instead of __next__() because we're using Python 2.
Output:
1: lo:mtu 65536 qdisc noqueue state UNKNOWN mode DEFAULT group default 2: eth0: mtu 1500 qdisc pfifo_fast state DOWN mode DEFAULT group default qlen 1000 3: wlan0: mtu 1500 qdisc mq state UP mode DORMANT group default qlen 1000 4: lxcbr0: mtu 1500 qdisc noqueue state UNKNOWN mode DEFAULT group default 5: docker0: mtu 1500 qdisc noqueue state DOWN mode DEFAULT group default 8: vmnet1: mtu 1500 qdisc pfifo_fast state UNKNOWN mode DEFAULT group default qlen 1000 9: vmnet8: mtu 1500 qdisc pfifo_fast state UNKNOWN mode DEFAULT group default qlen 1000
We have a file that lists domain names. We want to write a Python function that takes the file and returns a dictionary that has a key for the domain and a value for ip.
For example, the input file ('domains.txt') looks like this:
google.com yahoo.com
Output is:
{'yahoo.com': '98.139.183.24', 'google.com': '216.58.192.14'}
Hint: we may want to use dig command, and to make the output simpler (only want to get ANSWER section), we can use it with additional options: +noall and +answer.
Here is the code:
import subprocess def getIPs(file): ips = {} with open(file) as f: for line in f: domain = line.strip() out = subprocess.check_output(["dig","+noall","+answer", domain]) ips[domain] = out.split()[4] return ips print getIPs('domains.txt')
We have a class A, and we want to count the number of A instances.
Hint : use staticmethod
class A: total = 0 def __init__(self, name): self.name = name A.total += 1 def status(): print "Total number of instance of A : ", A.total status = staticmethod(status) a1 = A("A1") a2 = A("A2") a3 = A("A3") a4 = A("A4") A.status()
Output:
Total number of instance of A : 4
We can use decorator (@staticmethod) like this:
@staticmethod def status(): print "Total number of instance of A : ", A.total
Ref : https://docs.python.org/2/library/profile.html
cProfile and profile provide deterministic profiling of Python programs. A profile is a set of statistics that describes how often and for how long various parts of the program executed.
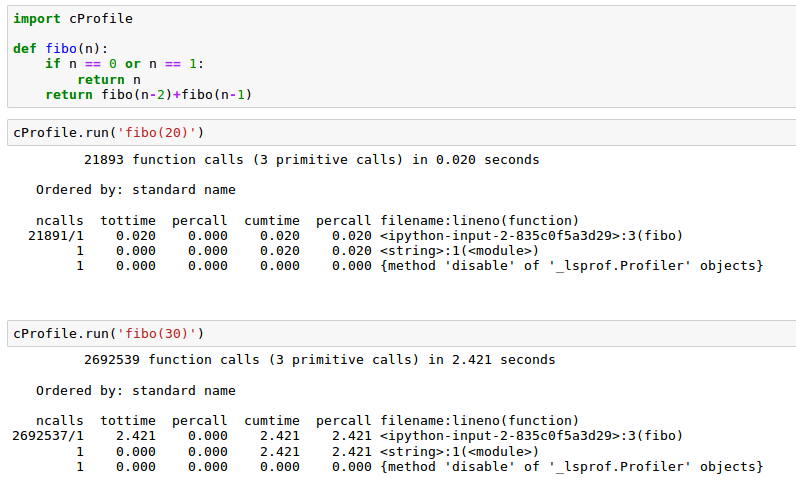
We can find the explanation of the output from the reference:
In the output from fibo(10), the first line indicates that 21893 calls were monitored. Of those calls, 3 were primitive, meaning that the call was not induced via recursion. The next line: Ordered by: standard name, indicates that the text string in the far right column was used to sort the output. The column headings include:
- ncalls
for the number of calls - tottime
for the total time spent in the given function (and excluding time made in calls to sub-functions) - percall
is the quotient of tottime divided by ncalls - cumtime
is the cumulative time spent in this and all subfunctions (from invocation till exit). This figure is accurate even for recursive functions. - percall
is the quotient of cumtime divided by primitive calls - filename:lineno(function)
provides the respective data of each function
When there are two numbers in the first column (for example 21891/1), it means that the function recursed. The second value is the number of primitive calls and the former is the total number of calls. Note that when the function does not recurse, these two values are the same, and only the single figure is printed.
The super() can be used when the root class inherits from the object class, and here is the code of calling a base class method from a child class that overrides it:
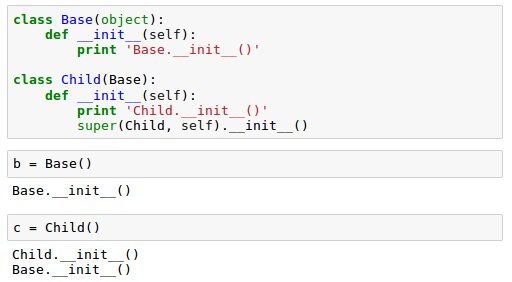
A module can find out its own module name by looking at the predefined global variable __name__. If this has the value '__main__', the program is running as a script.
Many modules that are usually used by importing them also provide a command-line interface or a self-test, and only execute this code after checking __name__:
# t.py def f(): print(f"module name is {__name__}") if __name__ == '__main__': f()
We have a simple Python module t.py with a function f() and a conditional statement that checks if the module is being run as the main program. If it is, it calls the f() function.
- The function f() prints the name of the module using the __name__ variable. When a Python script is run, the __name__ variable is set to '__main__'. If the module is imported into another script, __name__ is set to the name of the module.
- The conditional statement if __name__ == '__main__': ensures that the f() function is only called if the script is executed directly, not if it is imported as a module in another script.
- If we run this script directly (not imported as a module), it will print "module name is main" to the console. However, if we import it as a module in another script, the f() function won't be called automatically.
Run it as a script directly:
$ python t.py module name is __main__
If we import the t.py, the module name will be t:
# s.py import t
$ python s.py module name is t
Note that if want the f() to be called when imported the conditionals should be commented out:
# t.py def f(): print(f"module name is {__name__}") # if __name__ == '__main__': # f() f()
Now, when we import t in another script, the f() function will be executed automatically. Keep in mind that this approach makes the f() function run every time the module is imported, which may or may not be desired depending on the use case.
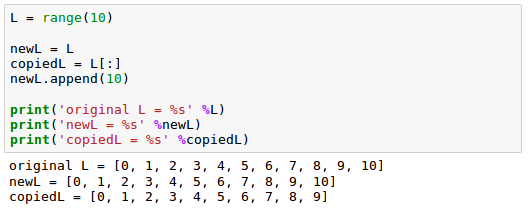
We might be wondering why appending an element to newL changed L too.
There are two factors that produce this result:
- Variables are simply names that refer to objects. Doing newL = L doesn't create a copy of the list - it creates a new variable newL that refers to the same object L refers to. This means that there is only one object (the list), and both L and newL refer to it.
- Lists are mutable, which means that we can change their content.
Please check: Copy an object
We have a list of numbers, and we want to group them by the number of digits:
numbers = [1,256,64,65536,186000,1024,8,16,1905] d = {} for n in numbers: d.setdefault(len(str(n)),[]).append(n) print d
Or:
numbers = [1,256,64,65536,186000,1024,8,16,1905] d = {len(str(i)):[] for i in numbers} print(f"group_by_digit = {d}") for x in numbers: d[len(str(x))].append(x) print(f"group_by_digit = {d}")
Output:
{1: [1, 8], 2: [64, 16], 3: [256], 4: [1024, 1905], 5: [65536], 6: [186000]}
Write a program which will find all such numbers which are divisible by 7 but are not a multiple of 3, between 1 and 100 (both included). The numbers obtained should be printed in a colon(:)-separated sequence on a single line as shown below:
7:14:28:35:49:56:70:77:91:98
The code should look like this:
l = [] for n in range(1,101): if n % 7 == 0 and n % 3 != 0: l.append(str(n)) print ':'.join(l)
We want to convert binary numbers to integers:
"0100,0011,1010,1001" => [4, 3, 10, 9]
Here is the code:
s1 = "0100,0011,1010,1001" s2 = s1.split(',') num = [] for s in s2: n = 0 for i,v in enumerate(s[::-1]): # reverse 0100->0010 n += (2**int(i)) * int(v) num.append(n) print num
We want to write a code that computes the value of a+aa+aaa+aaaa with a given `digit` as the value of `a`. Suppose the following input is supplied to the program:
N = 9 DIGIT = 4
Then, the output should be:
9+99+999+9999 = 11106
Here is the code:
N = 9 DIGIT = 4 s = 0 for i in range(1,DIGIT+1): s += int(str(N)*i) # '9'*3 = '999' print s
We have a file that has deposit(D) and withdrawal(W) records:
D 500 D 300 W 100 D 400 W 100
We want to calculate the balance after reading the bank records, and it should be 1,000. The code looks like this:
f = open("bank.txt") balance = 0 for line in f: item = line.rstrip().split() if item[0] == 'D': balance += int(item[1]) else: balance -= int(item[1]) print balance f.close()
We can take only the words composed of digits from a given string using re.findall():
s = """Solar system: Planets 8 Dwarf Planets: 5 Moons: Known = 149 | Provisional = 24 | Total = 173 Comets: More than 3400 Asteroids: More than 715000""" import re d = re.findall('\d+',s) print d
Output:
['8', '5', '149', '24', '173', '3400', '715000']
We have a count of 35 heads and 94 legs among the chickens and pigs in a farm. How many pigs and how many chickens do we have?
# 35 heads and 94 legs among the chickens and pigs # c + p = 35 # 2*c + 4*p = 94 heads = 35 legs = 94 for c in range(heads): p = 35-c if 2*c + 4*p == 94: print c,p
The answer is:
23 12
Find the highest possible product that we can get by multiplying any 3 numbers from an input array:
def mx3(a): b = sorted(a) m0 = b[0] m1 = b[1] x1 = b[-1] x2 = b[-2] x3 = b[-3] if m0 < 0 and m1 < 0: return max(m0*m1*x1,x1*x2*x3) else: return x1*x2*x3 l1 = [10,20,5,2,7,9,3,4] l2 = [10,-20,5,2,7,9,3,4] l3 = [-10,-20,5,2,7,9,-3,4] l4 = [10,-20,5,2,7,9,-3,4] print mx3(l1) print mx3(l2) print mx3(l3) print mx3(l4)
Output:
1800 630 1800 630
Implement a queue with a size limit of 3.
class Queue: def __init__(self): self.items = [] def put(self, k,v): if len(self.items) >= 3: self.items.pop(0) self.items.append((k,v)) def get(self,k): for x in self.items: if k == x[0]: return x[1] return -1 def __repr__(self): return str(self.items) q = Queue() q.put(1,111), print(q) q.put(2,222), print(q) q.put(3,333), print(q) q.put(4,444), print(q) print(q.get(4)) print(q.get(10))
Output:
[(1, 111)] [(1, 111), (2, 222)] [(1, 111), (2, 222), (3, 333)] [(2, 222), (3, 333), (4, 444)] 444 -1
- Python Coding Questions I
- Python Coding Questions II
- Python Coding Questions III
- Python Coding Questions IV
- Python Coding Questions V
- Python Coding Questions VI
- Python Coding Questions VII
- Python Coding Questions VIII
- Python Coding Questions IX
- Python Coding Questions X
List of codes Q & A
- Merging two sorted list
- Get word frequency - initializing dictionary
- Initializing dictionary with list
- map, filter, and reduce
- Write a function f() - yield
- What is __init__.py?
- Build a string with the numbers from 0 to 100, "0123456789101112..."
- Basic file processing: Printing contents of a file - "with open"
- How can we get home directory using '~' in Python?
- The usage of os.path.dirname() & os.path.basename() - os.path
- Default Libraries
- range vs xrange
- Iterators
- Generators
- Manipulating functions as first-class objects
- docstrings vs comments
- using lambdda
- classmethod vs staticmethod
- Making a list with unique element from a list with duplicate elements
- What is map?
- What is filter and reduce?
- *args and **kwargs
- mutable vs immutable
- Difference between remove, del and pop on lists
- Join with new line
- Hamming distance
- Floor operation on integers
- Fetching every other item in the list
- Python type() - function
- Dictionary Comprehension
- Sum
- Truncating division
- Python 2 vs Python 3
- len(set)
- Print a list of file in a directory
- Count occurrence of a character in a Python string
- Make a prime number list from (1,100)
- Reversing a string - Recursive
- Reversing a string - Iterative
- Reverse a number
- Output?
- Merging overlapped range
- Conditional expressions (ternary operator)
- Packing Unpacking
- Function args
- Unpacking args
- Finding the 1st revision with a bug
- Which one has higher precedence in Python? - NOT, AND , OR
- Decorator(@) - with dollar sign($)
- Multi-line coding
- Recursive binary search
- Iterative binary search
- Pass by reference
- Simple calculator
- iterator class that returns network interfaces
- Converting domain to ip
- How to count the number of instances
- Python profilers - cProfile
- Calling a base class method from a child class that overrides it
- How do we find the current module name?
- Why did changing list 'newL' also change list 'L'?
- Constructing dictionary - {key:[]}
- Colon separated sequence
- Converting binary to integer
- 9+99+999+9999+...
- Calculating balance
- Regular expression - findall
- Chickens and pigs
- Highest possible product
- Implement a queue with a limited size
- Copy an object
- Filter
- Products
- Pickle
- Overlapped Rectangles
- __dict__
- Fibonacci I - iterative, recursive, and via generator
- Fibonacci II - which method?
- Fibonacci III - find last two digits of Nth Fibonacci number
- Write a Stack class returning Max item at const time A
- Write a Stack class returning Max item at const time B
- Finding duplicate integers from a list - 1
- Finding duplicate integers from a list - 2
- Finding duplicate integers from a list - 3
- Reversing words 1
- Parenthesis, a lot of them
- Palindrome / Permutations
- Constructing new string after removing white spaces
- Removing duplicate list items
- Dictionary exercise
- printing numbers in Z-shape
- Factorial
- lambda
- lambda with map/filter/reduce
- Number of integer pairs whose difference is K
- iterator vs generator
- Recursive printing files in a given directory
- Bubble sort
- What is GIL (Global Interpreter Lock)?
- Word count using collections
- Pig Latin
- List of anagrams from a list of words
- lamda with map, filer and reduce functions
- Write a code sending an email using gmail
- histogram 1 : the frequency of characters
- histogram 2 : the frequency of ip-address
- Creating a dictionary using tuples
- Getting the index from a list
- Looping through two lists side by side
- Dictionary sort with two keys : primary / secondary keys
- Writing a file downloaded from the web
- Sorting csv data
- Reading json file
- Sorting class objects
- Parsing Brackets
- Printing full path
- str() vs repr()
- Missing integer from a sequence
- Polymorphism
- Product of every integer except the integer at that index
- What are accessors, mutators, and @property?
- N-th to last element in a linked list
- Implementing linked list
- Removing duplicate element from a list
- List comprehension
- .py vs .pyc
- Binary Tree
- Print 'c' N-times without a loop
- Quicksort
- Dictionary of list
- Creating r x c matrix
- Transpose of a matrix
- str.isalpha() & str.isdigit()
- Regular expression
- What is Hashable? Immutable?
- Convert a list to a string
- Convert a list to a dictionary
- List - append vs extend vs concatenate
- Use sorted(list) to keep the original list
- list.count()
- zip(list,list) - join elements of two lists
- zip(list,list) - weighted average with two lists
- Intersection of two lists
- Dictionary sort by value
- Counting the number of characters of a file as One-Liner
- Find Armstrong numbers from 100-999
- Find GCF (Greatest common divisor)
- Find LCM (Least common multiple)
- Draws 5 cards from a shuffled deck
- Dictionary order by value or by key
- Regular expression - re.split()
- Regular expression : re.match() vs. re.search()
- Regular expression : re.match() - password check
- Regular expression : re.search() - group capturing
- Regular expression : re.findall() - group capturin
- Prime factors : n = products of prime numbers
- Valid IPv4 address
- Sum of strings
- List rotation - left/right
- shallow/deep copy
- Converting integer to binary number
- Creating a directory and a file
- Creating a file if not exists
- Invoking a python file from another
- Sorting IP addresses
- Word Frequency
- Printing spiral pattern from a 2D array - I. Clock-wise
- Printing spiral pattern from a 2D array - II. Counter-Clock-wise
- Find a minimum integer not in the input list
- I. Find longest sequence of zeros in binary representation of an integer
- II. Find longest sequence of zeros in binary representation of an integer - should be surrounded with 1
- Find a missing element from a list of integers
- Find an unpaired element from a list of integers
- Prefix sum : Passing cars
- Prefix sum : count the number of integers divisible by k in range [A,B]
- Can make a triangle?
- Dominant element of a list
- Minimum perimeter
- MinAbsSumOfTwo
- Ceiling - Jump Frog
- Brackets - Nested parentheses
- Brackets - Nested parentheses of multiple types
- Left rotation - list shift
- MaxProfit
- Stack - Fish
- Stack - Stonewall
- Factors or Divisors
- String replace in files 1
- String replace in files 2
- Using list as the default_factory for defaultdict
- Leap year
- Capitalize
- Log Parsing
- Getting status_code for a site
- 2D-Array - Max hourglass sum
- New Year Chaos - list
- List (array) manipulation - list
- Hash Tables: Ransom Note
- Count Triplets with geometric progression
- Strings: Check if two strings are anagrams
- Strings: Making Anagrams
- Strings: Alternating Characters
- Special (substring) Palindrome
- String with the same frequency of characters
- Common Child
- Fraudulent Activity Notifications
- Maximum number of toys
- Min Max Riddle
- Poisonous Plants with Pesticides
- Common elements of 2 lists - Complexity
- Get execution time using decorator(@)
- Conver a string to lower case and split using decorator(@)
- Python assignment and memory location
- shallow copy vs deep copy for compound objects (such as a list)
- Generator with Fibonacci
- Iterator with list
- Second smallest element of a list
- *args, **kargs, and positional args
- Write a function, fn('x','y',3) that returns ['x1', 'y1', 'x2', 'y2', 'x3', 'y3']
- sublist or not
- any(), all()
- Flattening a list
- Select an element from a list
- Circularly identical lists
- Difference between two lists
- Reverse a list
- Split a list with a step
- Break a list and make chunks of size n
- Remove duplicate consecutive elements from a list
- Combination of elements from two lists
- Adding a sublist
- Replace the first occurence of a value
- Sort the values of the first list using the second list
- Transpose of a matrix (nested list)
- Binary Gap
- Powerset
- Round Robin
- Fixed-length chunks or blocks
- Accumulate
- Dropwhile
- Groupby
- Simple product
- Simple permutation
- starmap(fn, iterable)
- zip_longest(*iterables, fillvalue=None)
- What is the correct way to write a doctest?
- enumerate(iterable, start=0)
- collections.defaultdict - grouping a sequence of key-value pairs into a dictionary of lists
- What is the purpose of the 'self' keyword when defining or calling instance methods?
- collections.namedtuple(typename, field_names, *, rename=False, defaults=None, module=None)
- zipped
- What is key difference between a set and a list?
- What does a class's init() method do?
- Class methods
- Permutations and combinations of ['A','B','C']
- Sort list of dictionaries by values
- Return a list of unique words
- hashlib
- encode('utf-8')
- Reading in CSV file
- Count capital letters in a file
- is vs ==
- Create a matrix : [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
- Binary to integer and check if it's the power of 2
- urllib.request.urlopen() and requests
- Game statistics
- Chess - pawn race
- Decoding a string
- Determinant of a matrix - using numpy.linalg.det()
- Revenue from shoe sales - using collections.Counter()
- Rangoli
- Unique characters
- Valid UID
- Permutations of a string in lexicographic sorted order
- Nested list
- Consecutive digit count
- Find a number that occurs only once
- Sorting a two-dimensional array
- Reverse a string
- Generate random odd numbers in a range
- Shallow vs Deep copy
- Transpose matrix
- Are Arguments in Python Passed by Value or by Reference?
- re: Is a string alphanumeric?
- reversed()
- Caesar's cipher, or shift cipher, Caesar's code, or Caesar shift
- Every other words
- re: How can we check if an email address is valid or not?
- re: How to capture temperatures of a text
- re.split(): How to split a text.
- How can we merge two dictionaries?
- How can we combine two dictionaries?
- What is the difference between a generator and a list?
- Pairs of a given array A whose sum value is equal to a target value N
- Adding two integers without plus
- isinstance() vs type()
- What is a decorator?
- In Python slicing, what does my_list[-3:2:-2] slice do?
- Revisit sorting dict - counting chars in a text file
- re: Transforming a date format using re.sub
- How to replace the newlines in csv file with tabs?
- pandas.merge
- How to remove duplicate charaters from a string?
- Implement a class called ComplexNumber
- Find a word frequency
- Get the top 3 most frequent characters of a string
- Just seen and ever seen
- Capitalizing the full name
- Counting Consequitive Characters
- Calculate Product of a List of Integers Provided using input()
- How many times a substring appears in a string
- Hello, first_name last_name
- String validators
- Finding indices that a char occurs in a list
- itertools combinations
Python tutorial
Python Home
Introduction
Running Python Programs (os, sys, import)
Modules and IDLE (Import, Reload, exec)
Object Types - Numbers, Strings, and None
Strings - Escape Sequence, Raw String, and Slicing
Strings - Methods
Formatting Strings - expressions and method calls
Files and os.path
Traversing directories recursively
Subprocess Module
Regular Expressions with Python
Regular Expressions Cheat Sheet
Object Types - Lists
Object Types - Dictionaries and Tuples
Functions def, *args, **kargs
Functions lambda
Built-in Functions
map, filter, and reduce
Decorators
List Comprehension
Sets (union/intersection) and itertools - Jaccard coefficient and shingling to check plagiarism
Hashing (Hash tables and hashlib)
Dictionary Comprehension with zip
The yield keyword
Generator Functions and Expressions
generator.send() method
Iterators
Classes and Instances (__init__, __call__, etc.)
if__name__ == '__main__'
argparse
Exceptions
@static method vs class method
Private attributes and private methods
bits, bytes, bitstring, and constBitStream
json.dump(s) and json.load(s)
Python Object Serialization - pickle and json
Python Object Serialization - yaml and json
Priority queue and heap queue data structure
Graph data structure
Dijkstra's shortest path algorithm
Prim's spanning tree algorithm
Closure
Functional programming in Python
Remote running a local file using ssh
SQLite 3 - A. Connecting to DB, create/drop table, and insert data into a table
SQLite 3 - B. Selecting, updating and deleting data
MongoDB with PyMongo I - Installing MongoDB ...
Python HTTP Web Services - urllib, httplib2
Web scraping with Selenium for checking domain availability
REST API : Http Requests for Humans with Flask
Blog app with Tornado
Multithreading ...
Python Network Programming I - Basic Server / Client : A Basics
Python Network Programming I - Basic Server / Client : B File Transfer
Python Network Programming II - Chat Server / Client
Python Network Programming III - Echo Server using socketserver network framework
Python Network Programming IV - Asynchronous Request Handling : ThreadingMixIn and ForkingMixIn
Python Coding Questions I
Python Coding Questions II
Python Coding Questions III
Python Coding Questions IV
Python Coding Questions V
Python Coding Questions VI
Python Coding Questions VII
Python Coding Questions VIII
Python Coding Questions IX
Python Coding Questions X
Image processing with Python image library Pillow
Python and C++ with SIP
PyDev with Eclipse
Matplotlib
Redis with Python
NumPy array basics A
NumPy Matrix and Linear Algebra
Pandas with NumPy and Matplotlib
Celluar Automata
Batch gradient descent algorithm
Longest Common Substring Algorithm
Python Unit Test - TDD using unittest.TestCase class
Simple tool - Google page ranking by keywords
Google App Hello World
Google App webapp2 and WSGI
Uploading Google App Hello World
Python 2 vs Python 3
virtualenv and virtualenvwrapper
Uploading a big file to AWS S3 using boto module
Scheduled stopping and starting an AWS instance
Cloudera CDH5 - Scheduled stopping and starting services
Removing Cloud Files - Rackspace API with curl and subprocess
Checking if a process is running/hanging and stop/run a scheduled task on Windows
Apache Spark 1.3 with PySpark (Spark Python API) Shell
Apache Spark 1.2 Streaming
bottle 0.12.7 - Fast and simple WSGI-micro framework for small web-applications ...
Flask app with Apache WSGI on Ubuntu14/CentOS7 ...
Fabric - streamlining the use of SSH for application deployment
Ansible Quick Preview - Setting up web servers with Nginx, configure enviroments, and deploy an App
Neural Networks with backpropagation for XOR using one hidden layer
NLP - NLTK (Natural Language Toolkit) ...
RabbitMQ(Message broker server) and Celery(Task queue) ...
OpenCV3 and Matplotlib ...
Simple tool - Concatenating slides using FFmpeg ...
iPython - Signal Processing with NumPy
iPython and Jupyter - Install Jupyter, iPython Notebook, drawing with Matplotlib, and publishing it to Github
iPython and Jupyter Notebook with Embedded D3.js
Downloading YouTube videos using youtube-dl embedded with Python
Machine Learning : scikit-learn ...
Django 1.6/1.8 Web Framework ...
Ph.D. / Golden Gate Ave, San Francisco / Seoul National Univ / Carnegie Mellon / UC Berkeley / DevOps / Deep Learning / Visualization